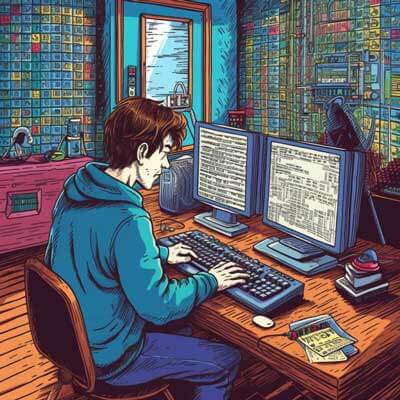
To make a redirect in PHP, you can use the header() function to send a raw HTTP header to the browser. This header tells the browser to navigate to a different URL. Here are two possible ways to achieve a redirect in PHP:
Using the header() function
The header() function allows you to send a raw HTTP header to the browser. To perform a redirect, you need to send a “Location” header with the URL you want to redirect to. Here’s an example:
In this example, the header() function is used to send a “Location” header with the value “https://example.com”. The “exit” statement is then used to stop the execution of the script immediately after the redirect.
It’s important to note that the header() function must be called before any actual output is sent to the browser. If there is any output, including whitespace or HTML tags, before calling the header() function, the redirect will not work. To avoid this, it’s common practice to place the PHP code for the redirect at the very beginning of the script, before any HTML or whitespace.
Related Article: How To Fix the "Array to string conversion" Error in PHP
Using the HTTP status code
Another way to perform a redirect in PHP is by sending an HTTP status code. This method involves sending a “301 Moved Permanently” or “302 Found” status code along with the “Location” header. Here’s an example:
In this example, the header() function is used twice. The first call sets the HTTP status code to “301 Moved Permanently”, indicating that the redirect is permanent. The second call sets the “Location” header to the desired URL. The “exit” statement is then used to stop the execution of the script.
Using an HTTP status code can be useful in certain situations. For example, if you want to redirect to a new URL but also notify search engines that the old URL has permanently moved, you can use a “301 Moved Permanently” status code. On the other hand, if you want to redirect temporarily, you can use a “302 Found” status code.
Why is the question asked?
The question of how to make a redirect in PHP arises when developers need to redirect users to a different page or URL. There can be various reasons for wanting to perform a redirect, such as:
– Redirecting users after a successful form submission.
– Redirecting users to a login page if they are not authenticated.
– Redirecting users to a different page based on their user role or permissions.
– Redirecting users to a different version of a website (e.g., mobile version).
By understanding how to make a redirect in PHP, developers can effectively control the flow of their web applications and provide a seamless user experience.
Suggestions and alternative ideas
While the header() function is a common and straightforward way to make a redirect in PHP, there are alternative approaches and suggestions to consider:
– Use a framework: If you are working with a PHP framework like Laravel or Symfony, they often provide built-in methods or helper functions to handle redirects. These abstractions can simplify the redirect process and offer additional features.
– Use a redirect library: There are several PHP libraries available that provide more advanced features for handling redirects, such as URL routing, URL generation, and redirect tracking. Examples include the “league/route” and “symfony/routing” libraries.
– Consider SEO implications: When performing redirects, especially permanent redirects (301), it’s essential to consider the impact on search engine optimization (SEO). Make sure to redirect to the most relevant and appropriate URL to preserve SEO rankings and avoid duplicate content issues.
– Handle redirects on the client-side: In some cases, it might be more appropriate to handle redirects on the client-side using JavaScript. This can give you more flexibility and control over the redirect logic, especially when dealing with dynamic or interactive web applications.
Related Article: How To Get The Full URL In PHP
Best practices
When making a redirect in PHP, it’s important to follow these best practices:
– Place the redirect code at the very beginning of the script, before any output is sent to the browser.
– Use the appropriate HTTP status code for the type of redirect you want to perform (e.g., 301 for permanent redirects, 302 for temporary redirects).
– Always include the “exit” statement or other means to stop the execution of the script after the redirect. This ensures that the redirect is executed immediately and prevents any further code execution.
– Validate and sanitize any user input used in the redirect URL to prevent security vulnerabilities, such as open redirects or XSS attacks.
– Consider the impact of the redirect on SEO and choose the most appropriate URL to redirect to, especially for permanent redirects (301).
– Test the redirect thoroughly to ensure it works as expected, including different scenarios and edge cases.
By following these best practices, developers can ensure that their redirects are reliable, secure, and provide a smooth user experience.
Overall, making a redirect in PHP is a fundamental skill for web developers. The header() function or HTTP status codes can be used to perform redirects effectively. By understanding the different options and best practices, developers can handle redirects in a secure and user-friendly manner.