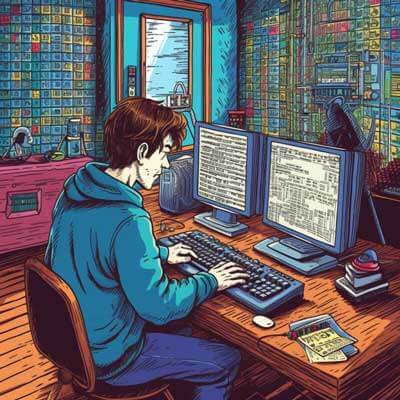
Table of Contents
To generate random strings in PHP, you can use the built-in functions and libraries that PHP provides. There are several methods you can use, depending on the specific requirements of your application. In this answer, we will explore two popular methods for generating random strings in PHP.
Method 1: Using the str_shuffle() Function
One simple method to generate random strings in PHP is by using the str_shuffle()
function. This function shuffles the characters of a string randomly, producing a new string with the same characters but in a different order. Here's an example:
$characters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789'; $randomString = str_shuffle($characters); echo substr($randomString, 0, 10);
In this example, we first define a string of characters that we want to use for generating the random string. The $characters
variable contains all the possible characters that can be used in the random string. Then, we pass this string to the str_shuffle()
function, which shuffles the characters randomly. Finally, we use the substr()
function to extract a portion of the shuffled string. In this case, we extract the first 10 characters.
This method is simple and straightforward, but keep in mind that it relies on the str_shuffle()
function, which may not produce truly random results. If you require a higher level of randomness, consider using a different method.
Related Article: Optimizing Laravel Apps: Deployment and Scaling Techniques
Method 2: Using the random_bytes() or random_int() Functions
For a more secure and truly random approach, PHP provides the random_bytes()
and random_int()
functions, which are available in PHP 7 and later versions. These functions use the operating system's random number generator to generate cryptographically secure random bytes or integers.
To generate a random string using random_bytes()
, you can use the following code:
$length = 10; $randomString = bin2hex(random_bytes($length)); echo substr($randomString, 0, $length);
In this example, we first define the length of the random string we want to generate. We then use the random_bytes()
function to generate a string of random bytes. The bin2hex()
function is used to convert the random bytes to a hexadecimal string representation. Finally, we use the substr()
function to extract a portion of the hexadecimal string, matching the desired length.
If you prefer to generate a random integer instead of a string, you can use the random_int()
function. Here's an example:
$min = 1000; $max = 9999; $randomNumber = random_int($min, $max); echo $randomNumber;
In this example, we specify the minimum and maximum values for the random integer range. The random_int()
function generates a random integer within this range, and we simply output the generated number.
Best Practices and Additional Considerations
Related Article: PHP vs Python: How to Choose the Right Language
When generating random strings in PHP, it's important to consider the following best practices:
1. Use a cryptographically secure random number generator: As mentioned earlier, if you require a high level of randomness, it's recommended to use the random_bytes()
or random_int()
functions, which rely on the operating system's random number generator.
2. Use a sufficient length: The length of the random string should be long enough to provide an adequate level of randomness. Consider the specific requirements of your application and choose an appropriate length.
3. Avoid predictable patterns: When generating random strings, make sure to avoid patterns or sequences that could potentially be guessed or exploited. For example, avoid using sequential numbers or repeating characters.
4. Use character sets wisely: Depending on your application's requirements, choose an appropriate set of characters for generating random strings. You may want to include uppercase letters, lowercase letters, numbers, and special characters, or restrict the character set to a specific subset.
5. Consider the performance impact: Generating random strings can be a computationally intensive task, especially if you need to generate a large number of random strings. Keep in mind the performance implications and optimize your code if necessary.
Overall, generating random strings in PHP is a relatively straightforward task. By using the appropriate functions and following best practices, you can ensure the security and randomness of the generated strings for your application.