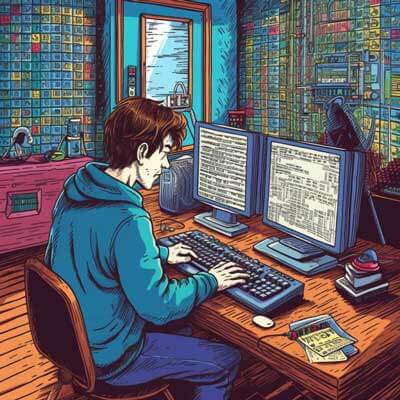
- What is a null matrix in Python?
- How to create a null matrix in Python using NumPy?
- What is the difference between a null matrix and a zero matrix?
- How to initialize a null matrix in Python?
- How to create a 2D null matrix in Python?
- Is it possible to create a null matrix with non-zero dimensions?
- What are some alternative ways to create a null matrix in Python?
- Can a null matrix contain non-null values?
- How to check if a matrix is null in Python?
- What are some use cases for null matrices in Python?
- Additional Resources
What is a null matrix in Python?
A null matrix, also known as a zero matrix, is a matrix where all the elements are zero. In Python, a null matrix can be represented using various libraries and data structures. One of the popular libraries for matrix operations in Python is NumPy. NumPy provides a multidimensional array object that can be used to create and manipulate matrices efficiently.
Related Article: 16 Amazing Python Libraries You Can Use Now
How to create a null matrix in Python using NumPy?
To create a null matrix using NumPy, we can use the numpy.zeros()
function. This function takes the shape of the desired matrix as an argument and returns a new matrix with all elements initialized to zero.
Here is an example of creating a 3×3 null matrix using NumPy:
import numpy as np null_matrix = np.zeros((3, 3)) print(null_matrix)
Output:
[[0. 0. 0.] [0. 0. 0.] [0. 0. 0.]]
In the above example, we imported the NumPy library and used the zeros()
function to create a 3×3 null matrix. The shape of the matrix is specified as (3, 3)
, which means it has 3 rows and 3 columns. The resulting matrix is then printed to the console.
What is the difference between a null matrix and a zero matrix?
In Python, a null matrix and a zero matrix are often used interchangeably, as both refer to a matrix where all the elements are zero. However, in some mathematical contexts, a null matrix may refer to a matrix with no elements at all (i.e., an empty matrix), whereas a zero matrix is a matrix with zero elements.
In practical terms, when working with libraries like NumPy, a null matrix and a zero matrix are essentially the same thing, and the terms can be used interchangeably.
How to initialize a null matrix in Python?
To initialize a null matrix in Python, we can use the numpy.zeros()
function from the NumPy library. This function takes the shape of the desired matrix as an argument and returns a new matrix with all elements initialized to zero.
Here is an example of initializing a 2×2 null matrix using NumPy:
import numpy as np null_matrix = np.zeros((2, 2)) print(null_matrix)
Output:
[[0. 0.] [0. 0.]]
In the above example, we used the zeros()
function to initialize a 2×2 null matrix. The shape of the matrix is specified as (2, 2)
, which means it has 2 rows and 2 columns. The resulting matrix is then printed to the console.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
How to create a 2D null matrix in Python?
To create a 2D null matrix in Python, we can use the numpy.zeros()
function from the NumPy library. This function takes the shape of the desired matrix as an argument and returns a new matrix with all elements initialized to zero.
Here is an example of creating a 2D null matrix with dimensions 3×4 using NumPy:
import numpy as np null_matrix = np.zeros((3, 4)) print(null_matrix)
Output:
[[0. 0. 0. 0.] [0. 0. 0. 0.] [0. 0. 0. 0.]]
In the above example, we used the zeros()
function to create a 2D null matrix with dimensions 3×4. The shape of the matrix is specified as (3, 4)
, which means it has 3 rows and 4 columns. The resulting matrix is then printed to the console.
Is it possible to create a null matrix with non-zero dimensions?
No, it is not possible to create a null matrix with non-zero dimensions. A null matrix, by definition, is a matrix where all the elements are zero. Therefore, a null matrix will always have zero dimensions.
If you need to create a matrix with non-zero dimensions but with all elements initialized to zero, you can use the numpy.zeros()
function from the NumPy library, as shown in the previous examples.
What are some alternative ways to create a null matrix in Python?
In addition to using the numpy.zeros()
function from the NumPy library, there are a few alternative ways to create a null matrix in Python.
1. Using a nested list comprehension:
null_matrix = [[0 for _ in range(num_columns)] for _ in range(num_rows)]
2. Using a nested for loop:
null_matrix = [] for i in range(num_rows): null_matrix.append([]) for j in range(num_columns): null_matrix[i].append(0)
Both of these methods create a null matrix by initializing each element to zero. However, using the NumPy library’s zeros()
function is generally more efficient and recommended for working with large matrices.
Related Article: Django 4 Best Practices: Leveraging Asynchronous Handlers for Class-Based Views
Can a null matrix contain non-null values?
No, a null matrix cannot contain non-null (non-zero) values. By definition, a null matrix is a matrix where all the elements are zero. If a matrix contains any non-zero elements, it is not a null matrix.
If you need to create a matrix with some non-zero values, you can use the numpy.zeros()
function from the NumPy library to create a null matrix and then modify specific elements to the desired non-zero values.
How to check if a matrix is null in Python?
To check if a matrix is null (i.e., all elements are zero) in Python, we can use NumPy’s numpy.all()
function along with the ==
operator.
Here is an example of checking if a matrix is null using NumPy:
import numpy as np matrix = np.zeros((3, 3)) is_null = np.all(matrix == 0) print(is_null)
Output:
True
In the above example, we created a 3×3 null matrix using numpy.zeros()
and then used the numpy.all()
function along with the ==
operator to check if all elements of the matrix are zero. The resulting boolean value (True
or False
) is then printed to the console.
What are some use cases for null matrices in Python?
Null matrices can be used in various applications and scenarios in Python. Here are a few use cases:
1. Initialization: Null matrices can be used as a starting point for initializing matrices with specific values. By creating a null matrix and then modifying specific elements, you can efficiently initialize a matrix with desired values.
2. Mathematical operations: Null matrices can be used in mathematical operations such as matrix addition, multiplication, and inverse calculations. They serve as a neutral element in these operations and can help simplify calculations.
3. Data analysis: In data analysis and machine learning, null matrices can be used to represent missing or incomplete data in a dataset. By replacing missing values with zeros, null matrices can facilitate further analysis and modeling.
4. Image processing: Null matrices can be used in image processing algorithms for tasks such as image filtering, noise reduction, and convolution operations. They provide a blank canvas to perform various operations on the image.
These are just a few examples of the use cases for null matrices in Python. The versatility of matrices makes them a fundamental data structure in many scientific and computational applications.
Related Article: String Comparison in Python: Best Practices and Techniques
Additional Resources
– Python Numpy: Create a null matrix
– Creating a null matrix using numpy
– Python numpy.zeros() function to create a zero matrix