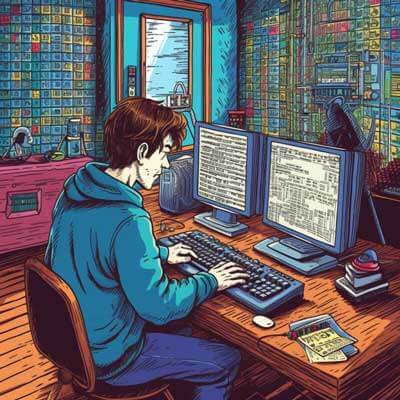
Table of Contents
Python does not have a built-in switch case statement like some other programming languages, such as C++ or Java. However, there are several ways to achieve similar functionality in Python. In this answer, we will explore different approaches to implement a switch case statement in Python.
Why is the question asked?
The question of how to use Python's equivalent for a case switch statement arises because developers are often familiar with switch case statements from other programming languages and want to achieve similar functionality in Python. Switch case statements provide a concise and readable way to handle multiple conditions or branches of code execution based on the value of a variable. Understanding how to implement a switch case-like behavior in Python can improve code readability and maintainability.
Related Article: How to Work with CSV Files in Python: An Advanced Guide
Approach 1: Using if...elif...else statements
One way to emulate a switch case statement in Python is by using a series of if...elif...else statements. Each condition is checked sequentially, and the corresponding block of code is executed if the condition is true. Here's an example:
def switch_case_example(argument): if argument == 1: print("You chose option 1") elif argument == 2: print("You chose option 2") elif argument == 3: print("You chose option 3") else: print("Invalid option") # Usage switch_case_example(2)
In this example, the switch_case_example
function takes an argument and executes different code blocks based on its value. The if...elif...else
statements act as the cases, and the code inside each block is executed if the corresponding condition is true.
This approach can be useful for handling a small number of cases. However, if there are many cases or the conditions become complex, this approach can become verbose and less readable.
Approach 2: Using a dictionary
Another approach to achieve a switch case-like behavior in Python is by using a dictionary. In this approach, the keys of the dictionary represent the different cases, and the values represent the corresponding code blocks. Here's an example:
def switch_case_example(argument): switch = { 1: "You chose option 1", 2: "You chose option 2", 3: "You chose option 3" } result = switch.get(argument, "Invalid option") print(result) # Usage switch_case_example(2)
In this example, the switch_case_example
function uses a dictionary called switch
to map the different cases to their corresponding code blocks. The switch.get(argument, "Invalid option")
statement retrieves the value associated with the argument
key from the dictionary. If the key does not exist, it returns the default value "Invalid option"
. This approach provides a more concise and readable way to handle multiple cases compared to the if...elif...else approach.
Using a dictionary for switch case-like behavior can be particularly useful when the cases involve more complex code blocks or when the mapping between cases and code blocks needs to be dynamic.
Alternative Ideas
While the if...elif...else and dictionary approaches are commonly used to emulate switch case statements in Python, there are other alternative ideas to consider:
- Using a class-based approach: Python allows you to define classes, and each class can have methods that represent different cases. By instantiating an object of the class and calling the corresponding method based on the case, you can achieve switch case-like behavior. This approach can lead to more modular and object-oriented code.
- Using the match statement (Python 3.10+): Starting from Python 3.10, a new match statement was introduced as part of the language. The match statement provides a more concise and readable way to handle multiple cases. It can be a good choice for projects that use Python 3.10 or newer versions.
Related Article: How to Implement Line Break and Line Continuation in Python
Best Practices
When emulating switch case statements in Python, it's important to follow some best practices to ensure code readability and maintainability:
- Use meaningful variable and case names: Choose variable and case names that clearly describe their purpose. This makes the code more understandable and reduces the chances of introducing bugs.
- Keep code blocks concise: Each code block associated with a case should be kept concise and focused. Extract complex logic into separate functions or methods to improve code readability.
- Consider using the default case: Always include a default case or handle cases where the value does not match any of the defined cases. This prevents unexpected behavior or silent failures.
- Be consistent with your chosen approach: If you are working on a team or collaborating on a project, ensure that everyone follows the same approach to emulate switch case behavior. Consistency improves code maintainability and reduces confusion.