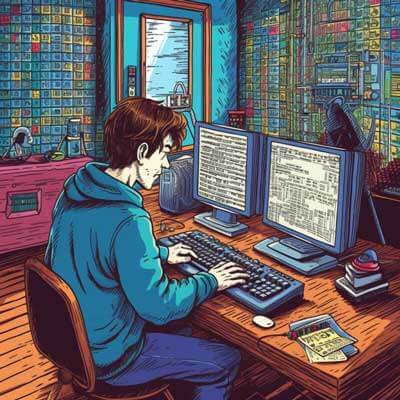
Table of Contents
How to check the number of active connections?
To check the number of active connections to a PostgreSQL query, you can use the following SQL query:
SELECT count(*) FROM pg_stat_activity WHERE state = 'active';
This query retrieves the count of all active connections from the pg_stat_activity
system view. The state
column is used to filter only the active connections.
Here's an example of how to execute the query using the psql
command-line tool:
$ psql -U postgres -c "SELECT count(*) FROM pg_stat_activity WHERE state = 'active';"
The output will be a single row with the count of active connections.
Related Article: How to Convert Columns to Rows in PostgreSQL
What is the maximum number of connections allowed?
The maximum number of connections allowed in PostgreSQL is determined by the max_connections
configuration parameter. This parameter specifies the maximum number of concurrent connections that can be made to the database server.
To check the current value of max_connections
, you can run the following query:
SHOW max_connections;
Here's an example of how to execute the query using the psql
command-line tool:
$ psql -U postgres -c "SHOW max_connections;"
The output will be a single row with the current value of max_connections
.
How to increase the maximum number of connections?
To increase the maximum number of connections in PostgreSQL, you need to modify the max_connections
configuration parameter in the postgresql.conf
file.
Here are the steps to increase the maximum number of connections:
1. Open the postgresql.conf
file in a text editor. The location of this file may vary depending on your operating system and PostgreSQL installation.
2. Search for the max_connections
parameter in the file.
3. Modify the value of max_connections
to the desired number of maximum connections.
4. Save the changes to the postgresql.conf
file.
5. Restart the PostgreSQL server for the changes to take effect.
After increasing the max_connections
parameter, PostgreSQL will allow a higher number of concurrent connections to the database server.
How can I monitor the number of open connections?
There are several ways to monitor the number of open connections in PostgreSQL.
One way is to use the pg_stat_activity
system view, which provides information about the current connections to the database server. You can run the following query to view the details of open connections:
SELECT * FROM pg_stat_activity;
This query will display information such as the process ID, username, application name, client IP address, and state of each connection.
Another method is to use monitoring tools such as pgAdmin or DataDog, which provide graphical interfaces to monitor PostgreSQL connections and other performance metrics.
Related Article: How to Use the ISNULL Function in PostgreSQL
What happens when the maximum number of connections is reached?
When the maximum number of connections is reached in PostgreSQL, any new connection attempts will be rejected until an existing connection is closed or the maximum number of connections is increased.
PostgreSQL will return an error message to the client indicating that the connection limit has been reached. The exact error message will depend on the client library or application you are using to connect to the database.
To handle this situation, you can implement connection pooling, which allows you to reuse existing connections instead of creating new ones for each query. This can help optimize resource usage and avoid reaching the maximum number of connections.
How to close idle connections?
Idle connections in PostgreSQL refer to connections that are open but not actively executing any queries. These connections consume server resources and can affect the overall performance of the database.
To close idle connections in PostgreSQL, you can use the pg_terminate_backend
function to terminate individual connections. Here's an example of how to close idle connections using the pg_terminate_backend
function:
SELECT pg_terminate_backend(pid)FROM pg_stat_activityWHERE state = 'idle' AND backend_type = 'client backend';
This query selects all idle connections of type "client backend" and terminates them using the pg_terminate_backend
function.
You can schedule this query to run periodically or integrate it into your application's logic to ensure that idle connections are closed automatically.
How to limit the number of connections per user?
To limit the number of connections per user in PostgreSQL, you can use the pg_hba.conf
file to define connection limits based on user roles.
Here's an example of how to set a connection limit for a specific user:
1. Open the pg_hba.conf
file in a text editor. The location of this file may vary depending on your operating system and PostgreSQL installation.
2. Add a line to the pg_hba.conf
file specifying the user, database, and connection limit. For example:
host mydb myuser 0/32 reject
This line specifies that the user myuser
is only allowed to have a maximum of 32 connections to the mydb
database. If the user exceeds this limit, the connection will be rejected.
3. Save the changes to the pg_hba.conf
file.
4. Restart the PostgreSQL server for the changes to take effect.
How to find the current number of connections?
To find the current number of connections in PostgreSQL, you can use the following SQL query:
SELECT count(*) FROM pg_stat_activity;
This query retrieves the count of all connections from the pg_stat_activity
system view.
Here's an example of how to execute the query using the psql
command-line tool:
$ psql -U postgres -c "SELECT count(*) FROM pg_stat_activity;"
The output will be a single row with the count of all connections.
Related Article: Integrating Fluent Bit with PostgreSQL Databases
How to terminate a specific connection?
To terminate a specific connection in PostgreSQL, you can use the pg_terminate_backend
function along with the process ID (PID) of the connection.
Here's an example of how to terminate a specific connection using the pg_terminate_backend
function:
SELECT pg_terminate_backend(pid)FROM pg_stat_activityWHERE pid = <process_id>;
Replace <process_id>
with the actual process ID of the connection you want to terminate. You can find the process ID in the pg_stat_activity
system view.
Once the query is executed, the specified connection will be terminated.
What is a database connection pool?
A database connection pool is a cache of database connections maintained by an application server or middleware. It allows multiple clients to share a set of reusable connections to a database, reducing the overhead of creating and closing connections for each client request.
When a client requests a connection from the pool, it is provided with an available connection from the pool. After the client finishes using the connection, it is returned to the pool instead of being closed. This enables the connection to be reused by other clients, improving performance and scalability.
Connection pooling helps optimize resource usage and can reduce the number of active connections to the database server. It is especially useful in scenarios where the number of client connections is high and the overhead of creating new connections is significant.
Many programming languages and frameworks provide built-in support for connection pooling, making it easier to implement and manage in your applications.
Additional Resources
- How to check the number of active connections in PostgreSQL?
- What is the maximum number of connections allowed in PostgreSQL?