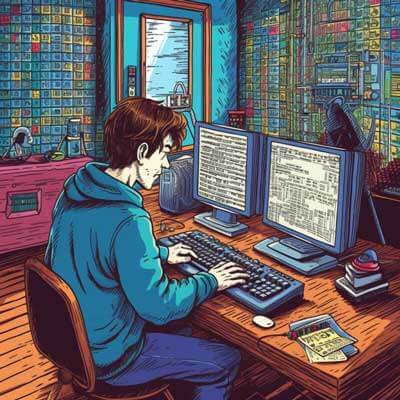
Table of Contents
In Angular applications, JavaScript plays a critical role in defining the behavior and functionality of components. The correct placement of JavaScript code within an Angular component is crucial for ensuring proper execution and maintaining a clean and organized codebase.
One of the key reasons why JavaScript placement is important in Angular components is to ensure that the code is executed at the right time and in the right context. Placing JavaScript code in the wrong location can lead to unexpected errors or undesired behavior. By understanding the Angular component architecture and following best practices for script placement, developers can avoid such issues and enhance the overall performance and maintainability of their Angular applications.
Understanding the Angular Component Architecture
Before diving into the specifics of JavaScript placement in Angular components, it is important to have a clear understanding of the Angular component architecture. In Angular, components are the building blocks of the application. They encapsulate the logic, data, and view of a specific part of the user interface.
Each Angular component consists of three main parts: the template, the class, and the metadata. The template defines the structure and layout of the component's view, while the class contains the JavaScript code that defines the component's behavior. The metadata provides additional information about the component, such as its selector and dependencies.
Related Article: How to Integrate UseHistory from the React Router DOM
Best Practices for Script Placement in Angular Component
To ensure clean and maintainable code, it is important to follow best practices for script placement in Angular components. Here are a few key practices to consider:
1. Separate Concerns: It is important to separate concerns and keep the JavaScript code related to a specific component within that component's class. This helps in better organization and understanding of the codebase.
2. Use Component Lifecycle Hooks: Angular provides several lifecycle hooks that allow developers to execute JavaScript code at specific stages of a component's lifecycle. By leveraging these hooks, developers can ensure that their code is executed at the appropriate time. For example, the ngOnInit hook is commonly used to initialize data and perform setup tasks.
3. Externalize Complex Logic: If a component requires complex JavaScript logic, it is often a good practice to externalize that logic into separate services or helper functions. This helps in keeping the component class clean and focused on its core responsibilities.
4. Follow the Single Responsibility Principle: Each component should have a single responsibility. If a component is responsible for multiple tasks or functionalities, it is recommended to split it into smaller, more focused components. This helps in keeping the codebase modular and maintainable.
Where to Put JavaScript Code in an Angular Component
In Angular components, JavaScript code should be placed within the component class. This is where the behavior and functionality of the component are defined. The component class is typically defined in a TypeScript file with the ".ts" extension.
Here's an example of a simple Angular component with JavaScript code placed within the component class:
import { Component } from '@angular/core'; @Component({ selector: 'app-example', template: '<p>{{ message }}</p>' }) export class ExampleComponent { message: string = 'Hello, World!'; constructor() { this.updateMessage(); } updateMessage() { setTimeout(() => { this.message = 'Updated message!'; }, 2000); } }
In the above example, the JavaScript code is placed within the ExampleComponent class. The message property is initialized with a default value and updated after a delay using the updateMessage method.
Options for Including JavaScript in Angular Component
There are multiple options for including JavaScript code within an Angular component. Here are a few common options:
1. Directly within the Component Class: As shown in the previous example, JavaScript code can be directly placed within the component class. This is the most common and recommended approach for including JavaScript code.
2. External JavaScript Files: In some cases, it may be necessary to include external JavaScript files within an Angular component. This can be achieved by adding a script tag to the component's template or by importing the JavaScript file in the component class.
3. Third-Party Libraries: Angular supports the use of third-party JavaScript libraries. These libraries can be included within an Angular component using various methods, such as installing them via npm and importing them in the component class or adding script tags to the component's template.
Related Article: How to Use the JavaScript Event Loop
Recommended Location for JavaScript in Angular Component
The recommended location for JavaScript code in an Angular component is within the component class. Placing the JavaScript code within the class ensures that it is directly associated with the component, making the code more organized, maintainable, and easier to understand.
Handling JavaScript Code in an Angular Component
When handling JavaScript code in an Angular component, it is important to follow certain conventions and guidelines to ensure consistency and maintainability. Here are some guidelines to consider:
1. Use TypeScript: Angular recommends using TypeScript for writing JavaScript code in Angular applications. TypeScript provides additional features such as static typing, interfaces, and classes, which enhance the development experience and help catch errors at compile-time.
2. Follow the Angular Style Guide: Following the Angular Style Guide helps maintain a consistent coding style across the project and makes the codebase more readable and maintainable. The style guide provides guidelines for various aspects of Angular development, including JavaScript placement within components.
3. Keep the Code Clean and Readable: It is important to write clean and readable JavaScript code within Angular components. This includes using meaningful variable and function names, following proper indentation and formatting, and adding comments when necessary.
4. Test the JavaScript Code: Writing unit tests for the JavaScript code within Angular components is essential to ensure its correctness and reliability. Unit tests can help catch bugs and regressions early in the development process and provide confidence in the behavior of the code.
Conventions for Script Placement within Angular Components
To maintain consistency and improve code readability, there are certain conventions for script placement within Angular components. Here are some common conventions to follow:
1. Place Imports at the Top: In the component class, imports should be placed at the top of the file, before the component decorator. This makes it easier to identify and manage the dependencies of the component.
2. Group Related Code: Within the component class, it is recommended to group related code together. For example, properties and methods related to data binding can be grouped separately from methods related to component lifecycle hooks.
3. Use Constructor for Dependency Injection: If the component requires any dependencies, it is recommended to use the constructor for dependency injection. This helps in maintaining clean and testable code by clearly defining the dependencies of the component.
Positioning Scripts within an Angular Component
When positioning scripts within an Angular component, it is important to consider the order of execution and the context in which the code will be executed. Here are some guidelines for positioning scripts within an Angular component:
1. Initialization Code: Initialization code, such as setting default values or initializing variables, should be placed within the constructor or the ngOnInit lifecycle hook. This ensures that the code is executed when the component is initialized.
2. Event Handlers: Event handlers should be placed within the component class, usually as methods. These methods can be called directly from the template or bound to specific events using Angular's event binding syntax.
3. Component Lifecycle Hooks: Angular provides several lifecycle hooks that allow developers to execute code at specific stages of a component's lifecycle. Depending on the specific requirements, code can be placed within hooks such as ngOnInit, ngOnChanges, ngOnDestroy, etc.
Related Article: How to Extract Values from Arrays in JavaScript
Including JavaScript Code in an Angular Component
To include JavaScript code within an Angular component, developers have multiple options. Here are a few common methods:
1. Directly within the Component Class: JavaScript code can be directly included within the component class, as shown in the previous examples. This is the most common and recommended method for including JavaScript code.
2. External JavaScript Files: If the JavaScript code is large or needs to be shared across multiple components, it can be placed in external JavaScript files. These files can be imported into the component class using the import statement.
3. Inline JavaScript: In some cases, it may be necessary to include inline JavaScript code within the component's template. This can be achieved using the script tag within the template, similar to how JavaScript code is included in HTML files.
Specific Location to Include Scripts for Angular Components
The specific location to include scripts for Angular components depends on the specific requirements and context of the application. However, there are some common locations where scripts are typically included:
1. Within the Component Class: The primary location for including JavaScript code within an Angular component is within the component class. This ensures that the code is directly associated with the component and follows the principles of encapsulation and modularity.
2. External JavaScript Files: If the JavaScript code is large or needs to be shared across multiple components, it can be placed in external JavaScript files. These files can be imported into the component class or included directly in the component's template, depending on the specific requirements.
3. Inline within the Template: In some cases, it may be necessary to include inline JavaScript code within the component's template. This can be achieved using the script tag within the template. However, it is generally recommended to keep the template focused on the presentation logic and move complex JavaScript code to the component class or external files.
Additional Resources
- Angular - Including External JavaScript Libraries