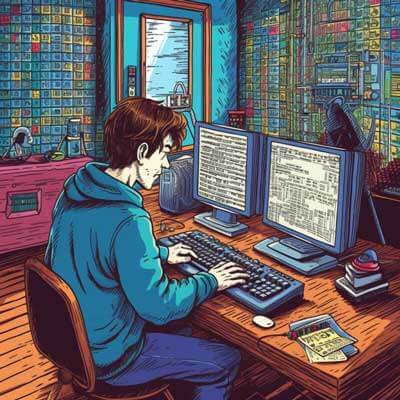
Table of Contents
How to Capitalize the First Letter in JavaScript
To capitalize the first letter of a string in JavaScript, you can use various approaches. Here are two possible ways to achieve this:
Related Article: Quick Intro on JavaScript Objects
Method 1: Using the toUpperCase() and slice() methods
One straightforward way to capitalize the first letter of a string is by using the toUpperCase() and slice() methods. The toUpperCase() method converts a string to uppercase, while the slice() method extracts a portion of a string.
Here's an example code snippet that demonstrates how to capitalize the first letter of a string using this method:
function capitalizeFirstLetter(string) { return string.charAt(0).toUpperCase() + string.slice(1); } const myString = "hello world"; const capitalizedString = capitalizeFirstLetter(myString); console.log(capitalizedString); // Output: "Hello world"
In the above code, the capitalizeFirstLetter()
function takes a string as input. It uses the charAt(0)
method to get the first character of the string and then applies the toUpperCase()
method to convert it to uppercase. Finally, the slice(1)
method is used to extract the remaining characters of the string, starting from the second character. The function returns the capitalized string.
You can use this method to capitalize the first letter of any string in JavaScript.
Method 2: Using the replace() method with a regular expression
Another way to capitalize the first letter of a string is by using the replace()
method with a regular expression.
Here's an example code snippet that demonstrates how to capitalize the first letter of a string using this method:
function capitalizeFirstLetter(string) { return string.replace(/^\w/, (c) => c.toUpperCase()); } const myString = "hello world"; const capitalizedString = capitalizeFirstLetter(myString); console.log(capitalizedString); // Output: "Hello world"
In the above code, the capitalizeFirstLetter()
function takes a string as input. It uses the replace()
method with the regular expression /^\w/
to match the first character of the string. The replace()
method then replaces the matched character with its uppercase version using the arrow function (c) => c.toUpperCase()
. The function returns the capitalized string.
This method is useful when you want to capitalize the first letter of a string without modifying the rest of the string.
Why is this question asked?
The question of how to capitalize the first letter in JavaScript is commonly asked because capitalizing the first letter of a string is a common requirement in many applications. For example, when displaying names or titles, it is often desirable to capitalize the first letter for better readability and visual appeal.
Furthermore, the question may arise when dealing with user input, where it is necessary to enforce proper capitalization of names or other input fields.
Related Article: How to Use Jquery Click Vs Onclick in Javascript
Potential reasons for asking this question:
1. User Interface: When developing a user interface, it is essential to present text in a visually appealing manner. Capitalizing the first letter of a string can help improve readability and provide a more polished appearance.
2. Data Validation: In certain scenarios, it may be necessary to ensure that specific fields, such as names or titles, are properly capitalized. By capitalizing the first letter, you can enforce data validation rules and maintain consistency in the application.
Suggestions and alternative ideas:
While the methods described above are commonly used to capitalize the first letter of a string in JavaScript, there are alternative approaches you can consider based on your specific requirements. Here are a few suggestions:
1. Using CSS: If you are working with a web application and the capitalization is purely for visual purposes, you can achieve this using CSS. By applying the text-transform: capitalize;
style to the relevant HTML element, you can automatically capitalize the first letter of each word in the element's text content.
2. Using a JavaScript library or utility function: If you frequently encounter the need to capitalize strings or perform other string manipulations, you may consider using a JavaScript library or utility function. Libraries like lodash or string.js provide convenient functions for string manipulation tasks, including capitalizing the first letter.
Best practices:
When capitalizing the first letter of a string in JavaScript, consider the following best practices:
1. Handle empty strings: Ensure that your implementation handles empty strings gracefully. For example, you can add a check to return an empty string if the input string is empty.
2. Consider internationalization: Be mindful that capitalizing the first letter of a string may not be sufficient in all languages. Some languages have specific capitalization rules or characters where the capitalization may differ. If your application supports multiple languages, consider using appropriate internationalization (i18n) libraries or functions to handle capitalization correctly.
3. Performance considerations: Depending on your specific use case, it is important to consider the performance implications of the chosen capitalization method. In most cases, the performance difference between the methods mentioned above is negligible, but if you are dealing with a large number of strings, it may be worth benchmarking and comparing the performance of different approaches.