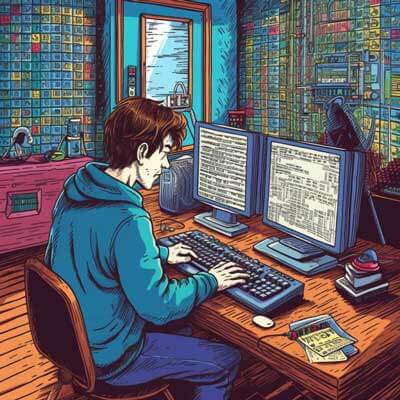
- What is Next.js?
- What does it mean to build a Next.js app locally?
- Why would I want to run a Next.js build locally?
- How do I install Next.js?
- How do I set up a development environment for Next.js?
- How do I build a Next.js app using npm?
- How do I build a Next.js app using yarn?
- Where can I find the build configuration settings in package.json?
- What is the difference between running a Next.js build locally and deploying it?
- What is server-side rendering in Next.js?
What is Next.js?
Next.js is a popular open-source framework for building server-side rendered (SSR) React applications. It provides a set of tools and conventions that make it easier to develop and deploy modern web applications. Next.js is built on top of React and extends it with features like server-side rendering, static site generation, and API routes.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
What does it mean to build a Next.js app locally?
Building a Next.js app locally refers to the process of compiling and bundling all the necessary files and dependencies of a Next.js application on your local machine. This process prepares the application for deployment or running it in a local development environment.
When you build a Next.js app locally, Next.js performs various tasks such as transpiling JavaScript code, optimizing assets, generating static HTML files, and more. The resulting bundle can then be deployed to a web server or used for local development.
Why would I want to run a Next.js build locally?
There are several reasons why you might want to run a Next.js build locally:
1. Testing: Running a Next.js build locally allows you to test your application before deploying it to a production environment. This helps you identify and fix any issues or bugs before they impact your users.
2. Performance Optimization: Building your Next.js app locally enables you to optimize the performance of your application. Next.js automatically optimizes your code and assets during the build process, resulting in faster load times and improved user experience.
3. Development Workflow: Running a Next.js build locally allows you to iterate and develop your application more efficiently. You can quickly see the changes you make to your code without the need for a full deployment cycle.
4. Offline Usage: Building a Next.js app locally enables you to create a static version of your application that can be served offline. This is especially useful for Progressive Web Applications (PWAs) that need to work even when there is no internet connection.
How do I install Next.js?
To install Next.js, you need to have Node.js and npm (Node Package Manager) installed on your machine. Follow these steps to install Next.js:
1. Open your terminal or command prompt.
2. Run the following command to create a new Next.js project:
npx create-next-app my-next-app
This command creates a new directory called “my-next-app” and sets up a basic Next.js project structure inside it.
3. Navigate to the project directory:
cd my-next-app
4. Start the development server:
npm run dev
This command starts the Next.js development server, and you can access your Next.js app at http://localhost:3000 in your web browser.
Related Article: How to Use the forEach Loop with JavaScript
How do I set up a development environment for Next.js?
Setting up a development environment for Next.js involves a few additional steps beyond the initial installation. Here’s how you can set up a development environment for Next.js:
1. Install the necessary dependencies by running the following command:
npm install
This command will install all the required dependencies specified in the package.json file.
2. Create a new file called “.env.local” in the root directory of your Next.js project. This file will contain environment variables that are specific to your development environment. For example, you might want to specify the API endpoint URL or other configuration options.
3. Start the development server:
npm run dev
This command will start the Next.js development server, and you can access your Next.js app at http://localhost:3000 in your web browser.
How do I build a Next.js app using npm?
To build a Next.js app using npm, you can use the following command:
npm run build
This command will build your Next.js app and generate an optimized production-ready bundle in the “.next” directory.
How do I build a Next.js app using yarn?
If you prefer using Yarn as your package manager, you can build a Next.js app using the following command:
yarn build
This command will perform the same build process as the npm command mentioned earlier and generate the production-ready bundle in the “.next” directory.
Related Article: How to Use Javascript Substring, Splice, and Slice
Where can I find the build configuration settings in package.json?
The build configuration settings for a Next.js app can be found in the “scripts” section of the package.json file. Here’s an example:
"scripts": { "dev": "next dev", "build": "next build", "start": "next start" }
In this example, the “build” script is responsible for building the Next.js app. You can customize this script or add additional build steps by modifying the command associated with the “build” key.
What is the difference between running a Next.js build locally and deploying it?
Running a Next.js build locally refers to executing the build process on your local machine to generate a production-ready bundle. This allows you to test and optimize your application before deploying it to a web server or a hosting platform.
On the other hand, deploying a Next.js app involves transferring the built bundle to a server or hosting platform where it can be accessed by users over the internet. Deploying typically involves additional steps such as configuring the server environment, setting up DNS records, and ensuring proper security measures.
What is server-side rendering in Next.js?
Server-side rendering (SSR) is a key feature of Next.js that allows your React components to be rendered on the server before being sent to the client. This means that when a user requests a page, the server generates the HTML content for that page and sends it to the client, pre-rendered and ready to be displayed.
Server-side rendering provides several benefits, including improved performance, better search engine optimization (SEO), and enhanced user experience. By rendering the HTML on the server, Next.js can send meaningful content to the client quickly, resulting in faster page load times. Additionally, search engines can easily crawl and index the pre-rendered HTML, improving the visibility of your application in search results.
To use server-side rendering in Next.js, you can simply create a React component and export it from a file in the “pages” directory. Next.js will automatically handle the server-side rendering for that page, making it easy to build dynamic and SEO-friendly applications.