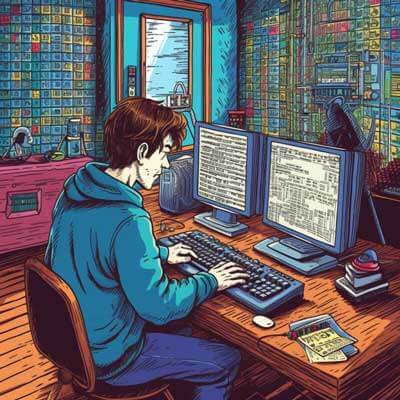
Table of Contents
What are JavaScript Generators?
JavaScript generators are a powerful feature introduced in ECMAScript 6 (ES6) that allows the creation of iterator objects. They provide a convenient way to define an iterable object by writing a function that can be paused and resumed. This is done using the yield
keyword, which allows a generator function to yield multiple values one at a time.
To define a generator function, you use the function*
syntax. Here's an example:
function* fibonacci() { let current = 0; let next = 1; while (true) { yield current; [current, next] = [next, current + next]; } }
In the above example, we define a generator function called fibonacci
that generates the Fibonacci sequence. When called, it returns an iterator object that can be used to iterate over the sequence. The yield
keyword is used to pause the function and return a value. Each time the generator function is called again, it resumes execution from where it left off.
To use the generator function, we can create an iterator object by calling it:
const iterator = fibonacci();
We can then use the iterator to retrieve values from the generator one at a time using the next()
method:
console.log(iterator.next().value); // 0 console.log(iterator.next().value); // 1 console.log(iterator.next().value); // 1 console.log(iterator.next().value); // 2 console.log(iterator.next().value); // 3 // and so on...
The next()
method returns an object with two properties: value
and done
. The value
property contains the yielded value, while the done
property indicates whether the generator function has finished producing values.
Generators can be used to implement lazy evaluation, where values are computed on-demand. They can be particularly useful for generating large sequences of values or when dealing with asynchronous operations.
Related Article: How to Check for Null Values in Javascript
How to Define a Generator Function
To define a generator function in JavaScript, you use the function*
syntax. This syntax distinguishes a generator function from a regular function. Let's take a look at the basic structure of a generator function:
function* myGenerator() { // generator function body }
The function*
keyword is followed by the function name and parentheses, similar to a regular function. However, inside the function body, you will use the yield
keyword to control the generation of values.
The yield
keyword is used to pause the function execution and return a value. It's important to note that each time a generator function encounters a yield
statement, it suspends execution and returns the yielded value. The generator function can then be resumed from where it left off.
Here's an example that demonstrates the use of yield
in a generator function:
function* generateNumbers() { yield 1; yield 2; yield 3; } const generator = generateNumbers(); console.log(generator.next()); // { value: 1, done: false } console.log(generator.next()); // { value: 2, done: false } console.log(generator.next()); // { value: 3, done: false } console.log(generator.next()); // { value: undefined, done: true }
In this example, the generateNumbers
generator function yields the numbers 1, 2, and 3. When we call generateNumbers()
and assign it to the generator
variable, it returns an iterator object. We can then call the next()
method on the iterator to retrieve the next yielded value.
Each time we call generator.next()
, it returns an object with two properties: value
and done
. The value
property represents the yielded value, and the done
property indicates whether the generator function has finished generating values.
As you can see from the example, the generator function execution is paused at each yield
statement, allowing us to control the flow of values being generated.
That's the basic concept of defining a generator function in JavaScript. In the next section, we'll explore how to use generator functions in combination with iterators for more powerful and flexible iteration patterns.
The Yield Keyword in Generators
Generators allow us to create functions that can be paused and resumed, enabling a level of control and flexibility not found in regular functions. One of the key elements of generators is the yield
keyword.
The yield
keyword is used within a generator function to pause the execution of the function and produce a value to the caller. When a generator function encounters a yield
statement, it immediately suspends its execution and returns the yielded value. The function can then be resumed later from where it left off.
Let's take a look at a simple example to understand how the yield
keyword works:
function* generator() { yield 1; yield 2; yield 3; } const gen = generator(); console.log(gen.next()); // { value: 1, done: false } console.log(gen.next()); // { value: 2, done: false } console.log(gen.next()); // { value: 3, done: false } console.log(gen.next()); // { value: undefined, done: true }
In this example, we define a generator function called generator()
using the function*
syntax. Inside the function, we use the yield
keyword to produce three values: 1, 2, and 3.
To use the generator function, we call it and assign the returned generator object to the variable gen
. We can then call the next()
method on the generator object to get the next value produced by the generator.
Each time we call gen.next()
, the generator function resumes its execution from where it left off and returns the next value. The next()
method returns an object with two properties: value
and done
. The value
property contains the yielded value, while the done
property indicates whether the generator has finished producing values.
After calling gen.next()
three times, the generator function has no more values to yield, so the done
property becomes true
and the value
property becomes undefined
. This signals that the generator has reached its end.
The yield
keyword can also be used to receive values from the caller when resuming the execution of a generator function. By passing a value to the next()
method, we can control the value that will be assigned to the yield
expression.
Here's an example:
function* generator() { const x = yield 'First yield'; const y = yield 'Second yield'; yield x + y; } const gen = generator(); console.log(gen.next()); // { value: 'First yield', done: false } console.log(gen.next(2)); // { value: 'Second yield', done: false } console.log(gen.next(3)); // { value: 5, done: false } console.log(gen.next()); // { value: undefined, done: true }
In this example, we define a generator function called generator()
that receives two values using the yield
keyword. The first time next()
is called, the generator pauses and returns the string 'First yield'. The value passed to the second next()
call is assigned to the variable x
in the generator function, and the second yield
statement produces the string 'Second yield'. Finally, the values of x
and y
are added together and yielded by the third yield
statement.
Understanding the yield
keyword is crucial to working effectively with generators in JavaScript. It allows us to create functions that can produce multiple values and be paused and resumed at any point. This makes generators a powerful tool for handling asynchronous operations, iterating over large datasets, and implementing custom iteration patterns.
Using Generators for Async Operations
Generators offer a great way to handle asynchronous operations. By leveraging the yield keyword, generators can pause and resume execution, allowing for more flexible and readable code when dealing with async tasks.
To understand how generators can be used for async operations, let's consider an example where we need to fetch data from an API. Normally, we would use callbacks or promises to handle the asynchronous nature of the operation. However, with generators, we can take a different approach.
Suppose we have an API endpoint that returns a list of users. We want to fetch this list and then fetch additional details for each user. Here's how we can achieve this using generators:
function* fetchUsers() { const users = yield fetch('https://api.example.com/users'); for (const user of users) { const userDetails = yield fetch(`https://api.example.com/user/${user.id}`); // Do something with userDetails } } const generator = fetchUsers(); const promise = generator.next().value; promise.then(users => { generator.next(users).value.then(userDetails => { generator.next(userDetails); }); });
In the example above, the fetchUsers()
function is a generator function that yields promises. The first yield
statement fetches the list of users, and the second yield
statement fetches the details for each user.
To execute the generator, we create an instance of it using fetchUsers()
, and then call next()
to start the generator. The first next()
call returns a promise that resolves to the list of users. We can then use .then()
to handle the result of the promise and call next()
again with the users as the argument. This process continues until the generator completes.
Using generators for async operations can greatly improve code readability and maintainability. By separating the async logic into generator functions, we can write code that looks synchronous, making it easier to reason about and debug.
In addition to using generators with promises, you can also use them with async/await syntax. The example below demonstrates how the same async operation can be achieved using async/await:
async function fetchUsers() { const users = await fetch('https://api.example.com/users'); for (const user of users) { const userDetails = await fetch(`https://api.example.com/user/${user.id}`); // Do something with userDetails } }
The fetchUsers()
function in the async/await example is essentially the same as the generator function. The await
keyword is used instead of yield
, and we no longer need to manually call next()
to resume the generator's execution.
Related Article: JavaScript Arrow Functions Explained (with examples)
Creating Infinite Sequences with Generators
One interesting use case for generators is creating infinite sequences, which can be extremely useful in certain scenarios.
To create an infinite sequence with generators, we can use a while loop that continues indefinitely. Inside the loop, we use the yield
keyword to generate each value of the sequence. Let's take a look at an example:
function* infiniteSequence() { let i = 0; while (true) { yield i++; } } const generator = infiniteSequence(); console.log(generator.next().value); // 0 console.log(generator.next().value); // 1 console.log(generator.next().value); // 2 // and so on...
In this example, we define a generator function called infiniteSequence
that uses a while loop to generate an infinite sequence of numbers. The yield
keyword is used to return the current value of i
and increment it by one on each iteration.
To use the generator, we create an instance of it by calling the function, and store the result in a variable called generator
. We can then call the next()
method on the generator to retrieve the next value in the sequence. Each time we call next()
, the generator resumes execution from where it left off and returns an object with a value
property that holds the current value of the sequence.
By using the yield
keyword inside a loop, we can create infinite sequences that generate values lazily. This means that values are only generated when they are requested, saving memory and processing power.
In addition to creating infinite sequences, generators can also be used to create sequences with a finite number of values. By adding a termination condition to the while loop, we can control when the sequence should stop generating values. Here's an example:
function* finiteSequence(limit) { let i = 0; while (i < limit) { yield i++; } } const generator = finiteSequence(5); console.log(generator.next().value); // 0 console.log(generator.next().value); // 1 console.log(generator.next().value); // 2 console.log(generator.next().value); // 3 console.log(generator.next().value); // 4 console.log(generator.next().value); // undefined
In this example, we define a generator function called finiteSequence
that takes a limit
parameter. The while loop continues until i
reaches the limit
. Once the limit is reached, the generator stops generating values and subsequent calls to next()
will return an object with a value
property of undefined
.
Creating infinite sequences with generators can be a powerful technique in JavaScript. It allows us to generate values on demand, saving resources and enabling us to work with sequences of any length without worrying about memory limitations.
Using Generators for Iteration Control
By using generators, we can pause and resume the execution of a function, which gives us fine-grained control over the iteration process. In this section, we will explore how to use generators for iteration control.
To create a generator function, we use the function*
syntax. Inside the generator function, we use the yield
keyword to pause the execution and return a value. Let's take a look at a simple example:
function* myGenerator() { yield 1; yield 2; yield 3; } const generator = myGenerator(); console.log(generator.next()); // { value: 1, done: false } console.log(generator.next()); // { value: 2, done: false } console.log(generator.next()); // { value: 3, done: false } console.log(generator.next()); // { value: undefined, done: true }
In the above example, we define a generator function myGenerator
that yields three values: 1, 2, and 3. We then create a generator instance generator
by calling the generator function. We can use the next()
method to iterate through the values generated by the generator. Each call to next()
returns an object with two properties: value
and done
. The value
property contains the yielded value, and the done
property indicates whether the generator has finished or not.
Generators can also receive values from the caller by using the yield
keyword as an expression. Let's see an example:
function* greetingGenerator() { const name = yield 'What is your name?'; yield `Hello, ${name}!`; } const generator = greetingGenerator(); console.log(generator.next()); // { value: 'What is your name?', done: false } console.log(generator.next('John')); // { value: 'Hello, John!', done: false } console.log(generator.next()); // { value: undefined, done: true }
In this example, the generator function greetingGenerator
asks for a name by yielding the string 'What is your name?'. The caller can then send a value to the generator by calling next()
with an argument. The value passed as an argument to next()
becomes the result of the yield
expression. In this case, the name 'John' is passed as an argument to the second next()
call, and it becomes the value of the name
variable.
By using generators, we can create custom iterators that provide more control over the iteration process. For example, we can define a generator function that yields values one by one from an array:
function* arrayIterator(arr) { for (let i = 0; i < arr.length; i++) { yield arr[i]; } } const array = [1, 2, 3]; const iterator = arrayIterator(array); console.log(iterator.next()); // { value: 1, done: false } console.log(iterator.next()); // { value: 2, done: false } console.log(iterator.next()); // { value: 3, done: false } console.log(iterator.next()); // { value: undefined, done: true }
In this example, we define a generator function arrayIterator
that takes an array as a parameter. The function uses a for
loop to iterate through the elements of the array and yields each element one by one. We can then create an iterator iterator
by calling the generator function with an array. Each call to next()
returns the next value from the array.
By using generators, we can pause and resume the execution of a function, allowing us to create custom iteration patterns.
Combining Generators with Promises
Generators provide a way to define functions that can be paused and resumed, allowing for the creation of asynchronous iterators. Promises, on the other hand, represent the eventual completion (or failure) of an asynchronous operation, allowing us to easily handle success and error cases.
By combining generators with promises, we can create a powerful tool for managing asynchronous flows. Generators can yield promises, which can then be resolved and resumed when the promise is fulfilled. This allows us to write asynchronous code that looks and behaves like synchronous code, making it easier to reason about and maintain.
Here's an example that demonstrates how to combine generators with promises:
function* asyncGenerator() { try { const result1 = yield new Promise((resolve) => setTimeout(() => resolve('First result'), 2000)); console.log(result1); const result2 = yield new Promise((resolve) => setTimeout(() => resolve('Second result'), 1000)); console.log(result2); const result3 = yield new Promise((resolve) => setTimeout(() => resolve('Third result'), 3000)); console.log(result3); } catch (error) { console.error('Error:', error); } } function runAsync(generator) { const iterator = generator(); function iterate({ value, done }) { if (done) return; Promise.resolve(value) .then((result) => iterate(iterator.next(result))) .catch((error) => iterate(iterator.throw(error))); } iterate(iterator.next()); } runAsync(asyncGenerator);
In this example, the asyncGenerator
function is a generator that yields promises. Each promise represents an asynchronous operation that takes some time to complete. The runAsync
function takes a generator function as an argument and runs it, iterating over the generator's values until it completes.
When the generator yields a promise, the runAsync
function waits for the promise to be resolved and then passes the result back to the generator by calling iterator.next(result)
. If the promise is rejected, the error is caught and passed to the generator by calling iterator.throw(error)
.
By combining generators with promises, we can create more concise and readable code that handles asynchronous operations in a synchronous-like manner. This can greatly improve the overall quality and maintainability of our JavaScript code.
Working with Error Handling in Generators
When working with JavaScript generators, it is important to handle errors properly. Errors can occur when a generator function is executed or when values are consumed from the generator using the iterator protocol. In this section, we will explore how to handle errors in generators effectively.
To handle errors within a generator function, we can use the try...catch statement. This allows us to catch and handle any errors that occur during the execution of the generator. Let's take a look at an example:
function* myGenerator() { try { yield 'Hello'; yield 'World'; throw new Error('Something went wrong'); yield '!'; } catch (e) { yield 'Error: ' + e.message; } } const generator = myGenerator(); console.log(generator.next().value); // Output: Hello console.log(generator.next().value); // Output: World console.log(generator.next().value); // Output: Error: Something went wrong console.log(generator.next().value); // Output: undefined
In the above example, we have a generator function myGenerator()
that yields three values: "Hello", "World", and an error is thrown with the message "Something went wrong". The try...catch
statement within the generator catches the error and yields an error message.
When consuming values from the generator using the iterator protocol, errors can also be thrown. We can handle these errors by using the try...catch
statement around the iterator's next()
method. Here's an example:
function* myGenerator() { yield 'Hello'; yield 'World'; throw new Error('Something went wrong'); yield '!'; } const generator = myGenerator(); let result; try { result = generator.next(); console.log(result.value); // Output: Hello result = generator.next(); console.log(result.value); // Output: World result = generator.next(); console.log(result.value); // This line will not be reached } catch (e) { console.log('Error:', e.message); // Output: Error: Something went wrong }
In the above example, we use the try...catch
statement to catch any errors that occur while consuming values from the generator. If an error is thrown, we can handle it within the catch
block and display an appropriate error message.
Handling errors in generators is crucial for ensuring that our code behaves as expected and provides meaningful feedback when errors occur. By using the try...catch
statement both within the generator function and when consuming values from the generator, we can effectively handle errors and maintain control over the flow of our program.
Related Article: How To Loop Through An Array In Javascript
Understanding JavaScript Iterators
An iterator is an object that provides a way to access the elements of a collection one by one. It allows us to loop over any iterable object, such as arrays, strings, or even custom objects. Iterators provide a common interface for iterating through different types of data structures.
The Iterator Protocol
The iterator protocol is a set of rules that JavaScript objects can implement to become iterable. An iterable object must have a method called Symbol.iterator
that returns an iterator object.
Here's an example of how to create an iterator for an array:
const myArray = [1, 2, 3]; const iterator = myArray[Symbol.iterator](); console.log(iterator.next()); // { value: 1, done: false } console.log(iterator.next()); // { value: 2, done: false } console.log(iterator.next()); // { value: 3, done: false } console.log(iterator.next()); // { value: undefined, done: true }
In the example above, we obtain the iterator for the myArray
array using the Symbol.iterator
method. We can then use the iterator's next
method to access the elements of the array one by one. Each call to next
returns an object with two properties: value
, which is the current element, and done
, which indicates whether there are more elements to iterate over.
The Iterable Protocol
The iterable protocol is a set of rules that iterable objects must follow. An iterable object is any object that can be iterated over using a loop or the spread operator.
Here's an example of how to create an iterable object:
const myObject = { data: [1, 2, 3], [Symbol.iterator]() { let index = 0; const data = this.data; return { next() { if (index < data.length) { return { value: data[index++], done: false }; } else { return { value: undefined, done: true }; } } }; } }; for (const element of myObject) { console.log(element); }
In this example, we define an iterable object myObject
that has a Symbol.iterator
method. The Symbol.iterator
method returns an iterator object with a next
method. Each call to next
returns the next element in the data
array until all elements have been iterated over.
Using Iterators with Generators
Generators in JavaScript provide an easier way to create iterators. A generator function is a special kind of function that can be paused and resumed. It allows us to write code that looks synchronous but behaves asynchronously.
Here's an example of a generator function that generates an infinite sequence of numbers:
function* numberGenerator() { let number = 1; while (true) { yield number++; } } const iterator = numberGenerator(); console.log(iterator.next()); // { value: 1, done: false } console.log(iterator.next()); // { value: 2, done: false } console.log(iterator.next()); // { value: 3, done: false }
In this example, the numberGenerator
function is defined using the function*
syntax. It contains a yield
statement that pauses the generator and returns a value. Each call to iterator.next()
resumes the generator and returns the next value in the sequence.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Benefits of Iterators
Iterators provide several benefits in JavaScript. They allow us to iterate over collections in a consistent and predictable way, regardless of their underlying data structure. They also provide a way to process large datasets efficiently, as we can lazily generate the next value in the sequence.
By using iterators, we can write more expressive and reusable code. They enable us to create custom iteration logic for our own objects, making them iterable and compatible with built-in JavaScript features like the for...of
loop and the spread operator.
Creating Custom Iterables
In JavaScript, we can create custom iterables by implementing the iterator protocol. This allows us to define our own iteration behavior for objects. To create a custom iterable, we need to define an iterator object that includes a next()
method.
The next()
method should return an object with two properties: value
and done
. The value
property represents the next value in the iteration, while the done
property indicates whether the iteration is complete or not.
Let's create a simple example of a custom iterable. Suppose we want to create an iterable that iterates over the even numbers up to a given limit. We can define an object with an iterator method that returns the next even number in the iteration:
const evenNumbers = { [Symbol.iterator]() { let current = 0; const limit = 10; return { next() { if (current <= limit) { const value = current; current += 2; return { value, done: false }; } else { return { done: true }; } } }; } }; for (const number of evenNumbers) { console.log(number); }
In the example above, we define a custom iterable evenNumbers
that uses the symbol Symbol.iterator
to define an iterator method. The iterator method returns an object with a next()
method.
The next()
method checks if the current value is less than or equal to the limit. If it is, it returns an object with the current value and done
set to false
, indicating that the iteration is not yet complete. Otherwise, it returns an object with done
set to true
, indicating that the iteration is complete.
We can then use a for...of
loop to iterate over the even numbers up to the limit defined in the iterator.
Creating custom iterables allows us to define our own iteration logic for objects, providing more flexibility and control over how we iterate over data. It can be particularly useful when working with complex data structures or when we want to iterate over values in a specific order.
To learn more about custom iterables and iterators, you can refer to the MDN documentation on Iterators and Generators.
Using Iterators with Generators
Generators and iterators are closely related concepts in JavaScript. While generators are functions that can be paused and resumed, iterators help in traversing data structures like arrays or strings.
By using the yield*
keyword, we can delegate the iteration to another iterator or generator. This allows us to easily combine multiple iterators or generators into a single sequence. Here's an example:
function* combinedGenerator() { yield* numberGenerator(); yield* ['a', 'b', 'c']; } const combined = combinedGenerator(); console.log(combined.next()); // { value: 1, done: false } console.log(combined.next()); // { value: 2, done: false } console.log(combined.next()); // { value: 3, done: false } console.log(combined.next()); // { value: 'a', done: false } console.log(combined.next()); // { value: 'b', done: false } console.log(combined.next()); // { value: 'c', done: false } // ...
In the example above, we combined the numberGenerator
with an array to produce a sequence of numbers followed by the elements of the array. Remember to use the yield*
keyword to delegate the iteration and make sure to implement the Symbol.iterator
method in objects that need to be iterated.
Iterating Over Arrays with for...of Loop
One of the most common use cases for generators and iterators in JavaScript is to iterate over arrays. The for...of
loop provides a simple and concise syntax for looping through the elements of an array.
To understand how the for...of
loop works with arrays, let's consider a simple example. Suppose we have an array of numbers:
const numbers = [1, 2, 3, 4, 5];
We can use the for...of
loop to iterate over this array and print each element to the console:
for (const number of numbers) { console.log(number); }
When we run this code, it will output:
1 2 3 4 5
As you can see, the for...of
loop iterates over each element of the array and assigns it to the variable number
. Inside the loop body, we can perform any desired operations with the current element.
The for...of
loop is particularly useful when we don't need to access the index of each element. It provides a cleaner and more readable alternative to the traditional for
loop.
In addition to arrays, the for...of
loop can be used with any iterable object, such as strings, sets, and maps. Iterables are objects that implement the iterable protocol and have a built-in iterator.
Here's an example of using the for...of
loop with a string:
const message = "Hello, World!"; for (const character of message) { console.log(character); }
This code will output:
H e l l o , W o r l d !
As you can see, the for...of
loop iterates over each character of the string and assigns it to the variable character
.
The for...of
loop provides a convenient way to iterate over the elements of an array or any other iterable object. It simplifies the code and improves readability, especially when we don't need to access the index of each element.
Related Article: How to Remove Spaces from a String in Javascript
Using Iterators for Object Iteration
To begin, let's explore what object iteration is. Object iteration refers to the process of iterating over the properties of an object. In JavaScript, objects can have both enumerable and non-enumerable properties. Enumerable properties are those that can be iterated over, while non-enumerable properties cannot.
One way to iterate over object properties is by using a for...in loop. This loop allows us to loop over the enumerable properties of an object, including properties inherited from its prototype chain. However, it does not include non-enumerable properties.
const person = { name: 'John', age: 30, city: 'New York' }; for (const key in person) { console.log(key, person[key]); }
In the above example, the for...in loop iterates over the properties of the person
object and logs each property key-value pair to the console. The output would be:
name John age 30 city New York
While the for...in loop is useful for iterating over enumerable properties, it does not provide a way to iterate over non-enumerable properties. This is where iterators come in.
JavaScript provides the Object.keys()
method, which returns an array of all enumerable property names of an object. We can then use this array to create an iterator and iterate over the object's properties.
const person = { name: 'John', age: 30, city: 'New York' }; const propertyNames = Object.keys(person); const iterator = propertyNames[Symbol.iterator](); let next = iterator.next(); while (!next.done) { const key = next.value; console.log(key, person[key]); next = iterator.next(); }
In the above example, we use Object.keys()
to get an array of property names from the person
object. We then create an iterator from the array using propertyNames[Symbol.iterator]()
. The iterator's next()
method is called in a loop until next.done
is true
, indicating that all properties have been iterated over.
The output would be the same as before:
name John age 30 city New York
Using iterators for object iteration gives us more control and flexibility, allowing us to iterate over both enumerable and non-enumerable properties. Additionally, iterators can be used with other looping constructs, such as for...of
loops, to further simplify the iteration process.
In this chapter, we have learned how to use iterators for object iteration in JavaScript. We explored how to use a for...in loop to iterate over enumerable properties and how to create an iterator from an array of property names using Object.keys()
. By leveraging iterators, we can iterate over all properties of an object, including both enumerable and non-enumerable ones.
Iterating Over Maps
Maps in JavaScript are key-value pairs where the keys can be any data type. To iterate over a map, we can use the entries()
method, which returns an iterator object containing an array of [key, value]
pairs.
Here's an example of how to iterate over a map using a generator function:
function* iterateMap(map) { for (let entry of map.entries()) { yield entry; } } let myMap = new Map(); myMap.set('key1', 'value1'); myMap.set('key2', 'value2'); myMap.set('key3', 'value3'); for (let [key, value] of iterateMap(myMap)) { console.log(`Key: ${key}, Value: ${value}`); }
This code creates a generator function iterateMap()
that uses a for...of
loop to iterate over the entries of the map. The generator function yields each entry, which can then be accessed in the for...of
loop.
The output of this code will be:
Key: key1, Value: value1 Key: key2, Value: value2 Key: key3, Value: value3
Iterating Over Sets
Sets in JavaScript are collections of unique values. To iterate over a set, we can use the values()
method, which returns an iterator object containing the set's values.
Here's an example of how to iterate over a set using a generator function:
function* iterateSet(set) { for (let value of set.values()) { yield value; } } let mySet = new Set(); mySet.add('value1'); mySet.add('value2'); mySet.add('value3'); for (let value of iterateSet(mySet)) { console.log(`Value: ${value}`); }
This code creates a generator function iterateSet()
that uses a for...of
loop to iterate over the values of the set. The generator function yields each value, which can then be accessed in the for...of
loop.
The output of this code will be:
Value: value1 Value: value2 Value: value3
Real World Examples of Generators and Iterators
Generators and iterators are powerful features in JavaScript that allow us to iterate over collections and generate values on the fly. They provide a way to control the flow of execution and manage resources efficiently. In this chapter, we will explore some real-world examples where generators and iterators can be used effectively.
Related Article: How To Append To A Javascript Array
1. Generating Fibonacci Sequence
One classic example of using generators is to generate the Fibonacci sequence. The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. Let's take a look at how we can use a generator to generate the Fibonacci sequence:
function* fibonacci() { let [prev, curr] = [0, 1]; while (true) { yield curr; [prev, curr] = [curr, prev + curr]; } } const fib = fibonacci(); console.log(fib.next().value); // 1 console.log(fib.next().value); // 1 console.log(fib.next().value); // 2 console.log(fib.next().value); // 3 console.log(fib.next().value); // 5
By using a generator, we can generate Fibonacci numbers on the fly without having to calculate the entire sequence upfront. This is especially useful when we only need a specific number of Fibonacci numbers or when memory is a concern.
2. Iterating over Infinite Streams
Iterators can be used to iterate over infinite streams of data, where the data is generated on demand. Let's consider the example of generating an infinite stream of random numbers:
function* randomNumbers() { while (true) { yield Math.random(); } } const numbers = randomNumbers(); console.log(numbers.next().value); // 0.123456789 console.log(numbers.next().value); // 0.987654321 console.log(numbers.next().value); // 0.543210987 // ...
In this example, we can see that the generator function randomNumbers()
generates an infinite stream of random numbers. We can consume this stream using the iterator interface provided by generators.
3. Asynchronous Control Flow
Generators can also be used to simplify asynchronous control flow. By using the yield
keyword with promises, we can pause and resume the execution of asynchronous tasks. Let's take a look at an example where we fetch data from an API asynchronously:
function* fetchData() { const data1 = yield fetch('https://api.example.com/data1'); const data2 = yield fetch('https://api.example.com/data2'); const data3 = yield fetch('https://api.example.com/data3'); // process data... } function run(generator) { const iterator = generator(); function iterate(value) { const { value: result, done } = iterator.next(value); if (!done) { result.then(iterate); } } iterate(); } run(fetchData);
In this example, the fetchData()
generator function fetches data from three different URLs asynchronously. The run()
function takes care of executing the generator and handling the asynchronous control flow using promises.
Optimizing Performance with Generators and Iterators
Generators and iterators in JavaScript provide powerful ways to work with sequences of values. They can also be optimized to improve performance in certain scenarios. In this chapter, we will explore some techniques to optimize the performance of generators and iterators.
Related Article: How To Redirect To Another Webpage In Javascript
Lazy Evaluation
One key advantage of generators is their ability to perform lazy evaluation. Lazy evaluation means that values are generated only when they are needed, rather than generating the entire sequence upfront. This can significantly improve performance when working with large sequences.
Consider the following example where we have a generator function that generates an infinite sequence of numbers:
function* generateNumbers() { let number = 1; while (true) { yield number; number++; } }
If we were to iterate over this generator and print the first 5 numbers, the generator would only generate as many numbers as needed:
const numbers = generateNumbers(); for (let i = 0; i < 5; i++) { console.log(numbers.next().value); }
This lazy evaluation prevents unnecessary computations and allows us to work with potentially infinite sequences efficiently.
Optimizing Memory Usage
Generators and iterators can also be optimized to reduce memory usage. By using generators, we can avoid storing large sequences in memory and generate values on the fly.
For example, let's say we have a generator function that generates all prime numbers:
function* generatePrimes() { let number = 2; while (true) { if (isPrime(number)) { yield number; } number++; } }
By using this generator, we can iterate over prime numbers without storing them in an array:
const primes = generatePrimes(); for (let i = 0; i < n; i++) { console.log(primes.next().value); }
This approach saves memory and allows us to work with large sequences efficiently.