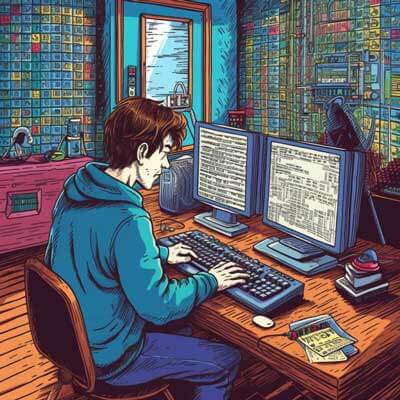
The ‘Maximum Call Stack Size Exceeded’ error is a common issue in JavaScript that occurs when a function calls itself repeatedly without an exit condition. This leads to the call stack becoming full and exceeding its maximum capacity. In this answer, we will discuss the potential reasons for this error, how to fix it, and some best practices to avoid it in the future.
Potential Reasons for the Error
There are several potential reasons why you might encounter the ‘Maximum Call Stack Size Exceeded’ error in JavaScript. Here are a few common scenarios:
1. Recursive Function Calls: Recursive functions are functions that call themselves. If a recursive function does not have a proper exit condition, it can lead to an infinite loop and eventually cause the call stack to overflow.
2. Unintentional Infinite Loops: In some cases, you may have unintentionally created an infinite loop without using recursion. This can happen when you have a loop that never terminates due to a logic error or incorrect loop conditions.
3. Deeply Nested Function Calls: If you have a large number of nested function calls, it can also lead to the call stack being exhausted. This can happen when working with complex algorithms or data structures.
Related Article: How To Download a File With jQuery
Fixing the Error
To fix the ‘Maximum Call Stack Size Exceeded’ error, you need to identify the root cause and make the necessary changes to prevent excessive function calls or infinite loops. Here are a few approaches you can take:
1. Check Recursive Functions: If you are using recursive functions, ensure that you have a proper exit condition that will stop the recursion at some point. Make sure that the exit condition is reachable and that it will eventually be met.
2. Review Loop Conditions: If you suspect that an unintentional infinite loop is causing the error, review the conditions of your loops carefully. Check for logic errors or incorrect loop conditions that prevent the loop from terminating.
3. Optimize Code Logic: Analyze your code to identify any areas where you might be making unnecessary or redundant function calls. Look for opportunities to optimize your code logic and reduce the number of function calls where possible.
4. Increase Stack Size Limit: In some cases, you may encounter the error due to a genuinely large call stack caused by a legitimate use case. If this is the case, you can try increasing the stack size limit using the ‘–stack-size’ flag when running your JavaScript code. However, be cautious with this approach as it is not a recommended long-term solution.
Best Practices to Avoid the Error
To prevent encountering the ‘Maximum Call Stack Size Exceeded’ error in the future, here are some best practices to follow when writing JavaScript code:
1. Use Proper Exit Conditions: When using recursive functions, ensure that you have a proper exit condition that will eventually be met. This will prevent infinite recursion and help you avoid the error.
2. Test and Validate Loops: Always test and validate your loops to ensure that they terminate as expected. Double-check the loop conditions and verify that the loop will terminate under all possible scenarios.
3. Optimize Code Efficiency: Write efficient code that minimizes unnecessary function calls and computations. Look for opportunities to optimize your algorithms and data structures to avoid excessive function calls.
4. Use Tail Call Optimization: Consider using tail call optimization (TCO) techniques when working with recursive functions. TCO allows the JavaScript engine to optimize recursive calls and avoid unnecessary stack growth.
5. Use Iterative Solutions: In some cases, you can replace recursive solutions with iterative ones to avoid excessive function calls. Iterative solutions often consume less memory and are less prone to stack overflow errors.
Example
Here’s an example that demonstrates a recursive function with a proper exit condition to avoid the ‘Maximum Call Stack Size Exceeded’ error:
function countdown(n) { if (n === 0) { console.log("Done!"); } else { console.log(n); countdown(n - 1); } } countdown(5);
In this example, the ‘countdown’ function recursively calls itself with a decreasing value of ‘n’ until ‘n’ reaches 0. At that point, it prints “Done!” and stops the recursion. This ensures that the function will eventually terminate and prevents the stack from overflowing.
Related Article: How to Run 100 Queries Simultaneously in Nodejs & PostgreSQL