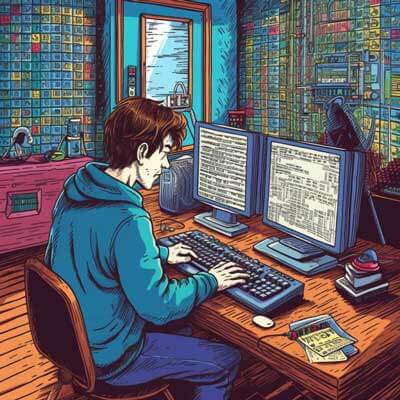
- Introduction
- Efficient Integration of HTMX with React
- Best Practices for Using HTMX with Vue.js
- Compatibility of HTMX with Angular
- HTMX Integration with jQuery
- Examples of Using HTMX with Ember.js
- Using HTMX with Backbone.js
- Configuring Webpack for HTMX Integration
- The Role of Babel in Integrating HTMX with Frontend Libraries
- Compatibility of HTMX with TypeScript
- Examples of Using HTMX with Redux
- Additional Resources
Introduction
HTMX is a lightweight JavaScript library that allows for efficient and seamless integration of AJAX functionality into web applications. With its simple syntax and useful capabilities, HTMX has gained popularity among developers for its ability to enhance user experience and improve performance.
In this article we will discuss best practices for using HTMX with various frontend libraries such as React, Vue.js, Angular, jQuery, Ember.js, and Backbone.js. We will also cover the configuration of Webpack and the role of Babel in integrating HTMX with frontend libraries. Additionally, we will explore the compatibility of HTMX with TypeScript and provide examples of using HTMX with Redux.
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
Efficient Integration of HTMX with React
React is a popular JavaScript library for building user interfaces. It provides a component-based architecture that allows for the creation of reusable UI components. HTMX can be seamlessly integrated with React to enhance the user experience and enable partial page updates.
To integrate HTMX with React, we need to install the HTMX library and import it into our React component. Let’s take a look at an example:
import React from 'react'; import htmx from 'htmx.org'; const App = () => { const handleClick = () => { htmx.ajax('/api/data', 'GET', { target: '#result' }); }; return ( <div> <button>Load Data</button> <div id="result"></div> </div> ); }; export default App;
In this example, we have a React component that fetches data from the server when a button is clicked. The HTMX library is used to send an AJAX request to the server and update the content of the #result
element with the response.
Best Practices for Using HTMX with Vue.js
Vue.js is a progressive JavaScript framework that is often used for building user interfaces. It provides a flexible and intuitive syntax that makes it easy to develop web applications. HTMX can be seamlessly integrated with Vue.js to enhance the user experience and enable partial page updates.
To integrate HTMX with Vue.js, we need to install the HTMX library and import it into our Vue component. Let’s take a look at an example:
<div> <button>Load Data</button> <div id="result"></div> </div> import htmx from 'htmx.org'; export default { methods: { handleClick() { htmx.trigger('#result', 'get'); }, }, };
In this example, we have a Vue component that fetches data from the server when a button is clicked. The HTMX library is used to send an AJAX request to the server and update the content of the #result
element with the response.
Compatibility of HTMX with Angular
Angular is a popular JavaScript framework for building web applications. It provides a comprehensive set of tools and features for creating complex applications. HTMX can be seamlessly integrated with Angular to enhance the user experience and enable partial page updates.
To integrate HTMX with Angular, we need to install the HTMX library and import it into our Angular component. Let’s take a look at an example:
<button>Load Data</button>
In this example, we have an Angular component that fetches data from the server when a button is clicked. The HTMX library is used to send an AJAX request to the server and update the content of the container with the response.
Related Article: How To Use Loop Inside React JSX
HTMX Integration with jQuery
jQuery is a popular JavaScript library that simplifies HTML document traversal, event handling, and animation. HTMX can be seamlessly integrated with jQuery to enhance the user experience and enable partial page updates.
To integrate HTMX with jQuery, we need to include the HTMX library in our HTML file and use the HTMX attributes. Let’s take a look at an example:
<button>Load Data</button> <div id="result"></div>
In this example, we have a button that fetches data from the server when clicked. The HTMX attributes hx-get
and hx-swap
are used to specify the AJAX request and the element to update with the response.
Examples of Using HTMX with Ember.js
Ember.js is a JavaScript framework for building scalable single-page applications. It provides a rich set of tools and features for creating complex applications. HTMX can be seamlessly integrated with Ember.js to enhance the user experience and enable partial page updates.
To integrate HTMX with Ember.js, we need to install the HTMX library and import it into our Ember component. Let’s take a look at an example:
import Component from '@glimmer/component'; import htmx from 'htmx.org'; export default class MyComponent extends Component { handleClick() { htmx.ajax('/api/data', 'GET', { target: '#result' }); } }
In this example, we have an Ember component that fetches data from the server when a button is clicked. The HTMX library is used to send an AJAX request to the server and update the content of the #result
element with the response.
Using HTMX with Backbone.js
Backbone.js is a lightweight JavaScript library that provides a simple and scalable way to structure web applications. It provides models, collections, and views to handle data and UI components. HTMX can be seamlessly integrated with Backbone.js to enhance the user experience and enable partial page updates.
To integrate HTMX with Backbone.js, we need to include the HTMX library in our HTML file and use the HTMX attributes. Let’s take a look at an example:
<button id="loadButton">Load Data</button> <div id="result"></div> const MyView = Backbone.View.extend({ el: '#loadButton', events: { 'click': 'handleClick', }, handleClick() { htmx.trigger('#result', 'get'); }, }); new MyView();
In this example, we have a Backbone.js view that fetches data from the server when a button is clicked. The HTMX attributes hx-get
and hx-swap
are used to specify the AJAX request and the element to update with the response.
Related Article: How To Upgrade Node.js To The Latest Version
Configuring Webpack for HTMX Integration
Webpack is a popular module bundler that is often used in modern web development. It allows developers to bundle and optimize their JavaScript code for production. To configure Webpack for HTMX integration, we need to install the necessary plugins and loaders.
First, let’s install the required packages:
npm install webpack webpack-cli html-webpack-plugin --save-dev
Next, we need to configure Webpack to handle HTMX files. In your Webpack configuration file, add the following code:
const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { // ...other configuration options plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', }), ], module: { rules: [ { test: /\.htmx$/, use: 'htmx-loader', }, ], }, };
In this example, we use the html-webpack-plugin
to generate an HTML file with the necessary script tags. We also configure Webpack to use the htmx-loader
for files with the .htmx
extension.
The Role of Babel in Integrating HTMX with Frontend Libraries
Babel is a popular JavaScript compiler that allows developers to write modern JavaScript code and transpile it to a compatible version that can run in older browsers. When integrating HTMX with frontend libraries, Babel plays a crucial role in ensuring compatibility and enabling the use of modern JavaScript features.
To configure Babel for HTMX integration, we need to install the necessary plugins and presets. Let’s install the required packages:
npm install @babel/core @babel/preset-env babel-loader --save-dev
Next, we need to configure Babel in our Webpack configuration file. Add the following code:
module.exports = { // ...other configuration options module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env'], }, }, }, ], }, };
In this example, we configure Babel to use the babel-loader
for JavaScript files and apply the @babel/preset-env
preset to transpile the code.
Compatibility of HTMX with TypeScript
TypeScript is a typed superset of JavaScript that compiles to plain JavaScript. It provides static typing and advanced features that make it easier to develop and maintain large-scale applications. HTMX is compatible with TypeScript and can be seamlessly integrated into TypeScript projects.
To use HTMX with TypeScript, we need to install the HTMX types and configure TypeScript to recognize HTMX attributes. Let’s install the required packages:
npm install htmx @types/htmx --save
Next, we can use HTMX in our TypeScript code. Here’s an example:
import htmx from 'htmx'; const handleClick = () => { htmx.ajax('/api/data', 'GET', { target: '#result' }); }; document.getElementById('loadButton').addEventListener('click', handleClick);
In this example, we import the HTMX library and use it to send an AJAX request when a button is clicked. TypeScript recognizes the HTMX types and provides autocompletion and type checking.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Examples of Using HTMX with Redux
Redux is a predictable state container for JavaScript applications. It provides a central store to manage application state and enables predictable state updates through actions and reducers. HTMX can be seamlessly integrated with Redux to enhance the user experience and enable partial page updates.
To integrate HTMX with Redux, we need to install the HTMX library and import it into our Redux actions or components. Let’s take a look at an example:
import htmx from 'htmx.org'; export const fetchData = () => { return (dispatch) => { htmx.ajax('/api/data', 'GET', { target: '#result', onSuccess: () => { dispatch({ type: 'FETCH_SUCCESS' }); }, onError: () => { dispatch({ type: 'FETCH_ERROR' }); }, }); }; };
In this example, we have a Redux action that fetches data from the server using HTMX. The HTMX library is used to send an AJAX request to the server and update the content of the #result
element with the response. The Redux store is updated based on the success or error of the AJAX request.
Additional Resources
– Integrating HTMX with React
– Best Practices for Integrating HTMX with Angular
– HTMX with Vue.js – AJAX-driven web applications