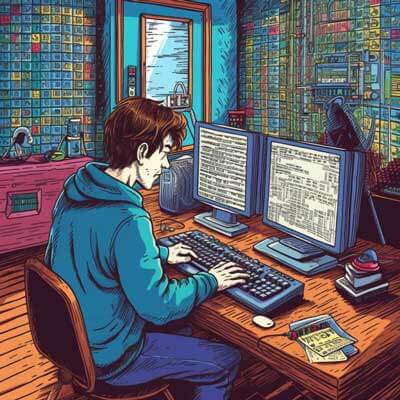
To redirect to another webpage in Javascript, you can use the window.location
object or the window.location.href
property. These methods allow you to navigate to a different URL within the same window or frame. In this answer, I will explain how to use these methods and provide some examples of best practices.
Using window.location
The window.location
object provides several properties and methods that allow you to manipulate the current URL and navigate to a different webpage. One of the most commonly used properties is window.location.href
, which returns the URL of the current webpage and can also be used to navigate to a new URL.
To redirect to another webpage, you can simply assign the new URL to window.location.href
. Here’s an example:
window.location.href = "https://www.example.com";
This will immediately redirect the user to the specified URL. It is important to note that the new URL must be a fully qualified URL, including the protocol (e.g., https://
).
Alternatively, you can also use window.location.assign()
, which has the same effect as assigning a new URL to window.location.href
. Here’s an example:
window.location.assign("https://www.example.com");
Both window.location.href
and window.location.assign()
are widely supported by all major browsers and are the recommended methods for redirecting to another webpage in Javascript.
Related Article: How To Fix 'Maximum Call Stack Size Exceeded' Error
Reasons for Redirecting to Another Webpage
There are several reasons why you might want to redirect to another webpage in Javascript. Some common use cases include:
1. After a form submission: When a user submits a form, you may want to redirect them to a “thank you” page or another relevant page.
2. After a successful login: After a user successfully logs in to your website, you may want to redirect them to their dashboard or a specific landing page.
3. URL redirection: You may need to redirect users from an old URL to a new URL, especially when migrating or restructuring your website.
Best Practices
When redirecting to another webpage in Javascript, it is important to follow some best practices to ensure a smooth user experience and maintain SEO friendliness. Here are some recommendations:
1. Use the appropriate HTTP status code: When redirecting, make sure to use the appropriate HTTP status code. For permanent redirects, use a 301 status code, and for temporary redirects, use a 302 status code. This helps search engines understand the intent of the redirect and properly handle indexing and ranking.
2. Provide alternative options for non-Javascript users: Not all users have Javascript enabled in their browsers. To ensure accessibility, provide an alternative option or a fallback mechanism for users who have Javascript disabled.
3. Consider using server-side redirection: In some cases, it may be more appropriate to handle the redirect on the server-side rather than relying solely on Javascript. This can help improve performance and ensure consistent behavior across different devices and browsers.
4. Test and handle edge cases: Test your redirection logic thoroughly and handle edge cases such as invalid URLs or unexpected user input. Make sure to handle errors gracefully and provide meaningful error messages when necessary.
Alternative Ideas
While using window.location.href
or window.location.assign()
is the most common way to redirect to another webpage in Javascript, there are alternative methods that you can consider depending on your specific requirements:
1. window.location.replace()
: This method replaces the current webpage in the browser’s history with the new URL, effectively preventing the user from navigating back to the original page using the browser’s back button. Use this method when you want to enforce a one-way navigation flow.
2. window.location.reload()
: This method reloads the current webpage. You can use this method in combination with window.location.href
or window.location.assign()
to redirect the user to another webpage and then automatically refresh the page.
Related Article: How To Download a File With jQuery