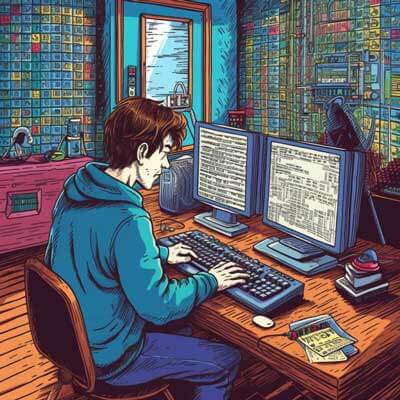
Using async/await with a foreach loop in JavaScript allows you to handle asynchronous operations in a more sequential and readable manner. This combination is particularly useful when dealing with arrays and performing asynchronous tasks on each element. Here are two ways you can use async/await with a foreach loop in JavaScript:
Method 1: Using a regular forEach loop with async/await
async function processArray(array) { array.forEach(async (item) => { await doSomethingAsync(item); }); } async function doSomethingAsync(item) { // Perform asynchronous operation here }
In this method, we define an async function called processArray
that takes an array as a parameter. Within this function, we use the forEach
method to iterate over each item in the array. Inside the callback function, we use the await
keyword to pause the execution until the asynchronous operation in the doSomethingAsync
function is resolved.
However, there is a limitation with this approach. The forEach
method does not wait for the promises returned by the async callback function to be resolved. As a result, the loop will not wait for each asynchronous operation to complete before moving on to the next iteration. If you need the loop to wait for each iteration to finish, you can use a for loop instead.
Related Article: Accessing Parent State from Child Components in Next.js
Method 2: Using a for…of loop with async/await
async function processArray(array) { for (const item of array) { await doSomethingAsync(item); } } async function doSomethingAsync(item) { // Perform asynchronous operation here }
In this method, we define an async function called processArray
that takes an array as a parameter. Using a for...of
loop, we iterate over each item in the array. Inside the loop, we use the await
keyword to pause the execution until the asynchronous operation in the doSomethingAsync
function is resolved.
Using a for...of
loop ensures that each iteration waits for the asynchronous operation to complete before moving on to the next iteration. This makes it more suitable for scenarios where sequential execution is required.
Best Practices
When using async/await with a foreach loop, there are some best practices to keep in mind:
1. Ensure that the callback function inside the loop is marked as async
. This allows you to use the await
keyword inside the callback to handle asynchronous operations.
2. If the order of execution is not important and you want to execute the iterations concurrently, you can use Promise.all
to create an array of promises and then await
the resolution of all the promises at once.
async function processArray(array) { await Promise.all(array.map(async (item) => { await doSomethingAsync(item); })); }
3. Handle errors appropriately within the loop. You can use try/catch blocks to catch and handle any errors that occur during the asynchronous operations.
async function processArray(array) { for (const item of array) { try { await doSomethingAsync(item); } catch (error) { console.error('An error occurred:', error); } } }
4. Make sure to return a promise from the async function if you need to handle the completion of all iterations. You can use Promise.resolve()
to return an empty resolved promise.
async function processArray(array) { for (const item of array) { await doSomethingAsync(item); } return Promise.resolve(); }
Related Article: How to Apply Ngstyle Conditions in Angular