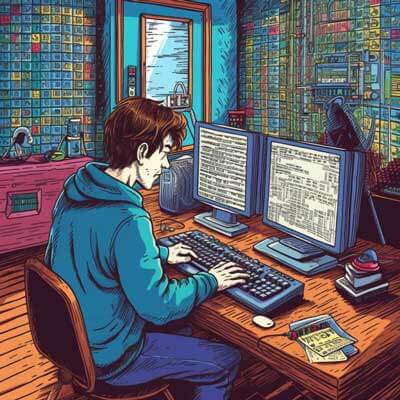
How to Download a File with JavaScript and jQuery
Downloading a file with JavaScript and jQuery can be accomplished using various techniques. In this answer, we will explore two common methods to download files using JavaScript and jQuery.
Related Article: How To Fix 'Maximum Call Stack Size Exceeded' Error
Method 1: Using the HTML5 download Attribute
The HTML5 download
attribute allows you to specify the filename when triggering a file download. This attribute can be used with an anchor (<a>
) element to create a link that triggers the file download when clicked.
Here’s an example of how to use the download
attribute to download a file with JavaScript and jQuery:
<a href="path/to/file.pdf" id="downloadLink">Download File</a> // Using jQuery to trigger the download $('#downloadLink').click();
In the example above, the href
attribute specifies the path to the file you want to download (path/to/file.pdf
), while the download
attribute specifies the desired filename (filename.pdf
). The id
attribute is used to select the anchor element using jQuery.
When the link is clicked, the file will be downloaded with the specified filename. This method works well for files that are publicly accessible via a URL.
Method 2: Using JavaScript to Programmatically Trigger the Download
If you need more control over the download process or want to download a file that is not publicly accessible via a URL, you can use JavaScript to programmatically trigger the download.
Here’s an example of how to use JavaScript and jQuery to programmatically download a file:
<button id="downloadButton">Download File</button> $('#downloadButton').click(function() { var fileUrl = 'path/to/file.pdf'; var fileName = 'filename.pdf'; var link = document.createElement('a'); link.href = fileUrl; link.download = fileName; document.body.appendChild(link); link.click(); document.body.removeChild(link); });
In the example above, we create a button element with the id downloadButton
. When the button is clicked, the JavaScript code inside the click
event handler is executed.
Inside the event handler, we create a new <a>
element using document.createElement('a')
. We set the href
attribute to the URL of the file (path/to/file.pdf
) and the download
attribute to the desired filename (filename.pdf
).
Next, we append the link element to the document body using document.body.appendChild(link)
. This is necessary to trigger the file download.
We then simulate a click on the link element using link.click()
, which triggers the file download. Finally, we remove the link element from the document using document.body.removeChild(link)
.
This method allows you to dynamically generate the file URL and filename based on your application’s logic.
Reasons for Downloading Files with JavaScript and jQuery
There are several potential reasons why you may need to download files using JavaScript and jQuery:
1. Generating dynamic files: You may need to generate files on the fly based on user input or server-side data. For example, you might want to generate a PDF report or export data as a CSV file.
2. Enhancing user experience: By allowing users to download files directly from your web application, you can provide a seamless and convenient experience.
3. Handling file dependencies: You may have files that need to be downloaded together or in a specific order. JavaScript and jQuery can help facilitate this process.
Related Article: How to Run 100 Queries Simultaneously in Nodejs & PostgreSQL
Suggestions and Best Practices
When downloading files with JavaScript and jQuery, keep the following suggestions and best practices in mind:
1. Ensure file availability: Make sure that the file you are trying to download is accessible and available at the specified URL.
2. Properly handle errors: Implement error handling to handle cases where the file cannot be downloaded or the download fails. This will help provide a better user experience.
3. Provide feedback to the user: Consider displaying a loading indicator or a success message to inform the user that the download is in progress or has completed successfully.
4. Consider security implications: Be cautious when downloading files based on user input, as it could potentially introduce security vulnerabilities. Always validate and sanitize user input before using it to generate file URLs or filenames.
5. Test cross-browser compatibility: Test your file download functionality across different browsers to ensure consistent behavior and compatibility.
6. Consider server-side alternatives: Depending on your specific use case, it may be more appropriate to handle file downloads on the server-side instead of using JavaScript and jQuery. Evaluate your requirements and choose the approach that best fits your needs.