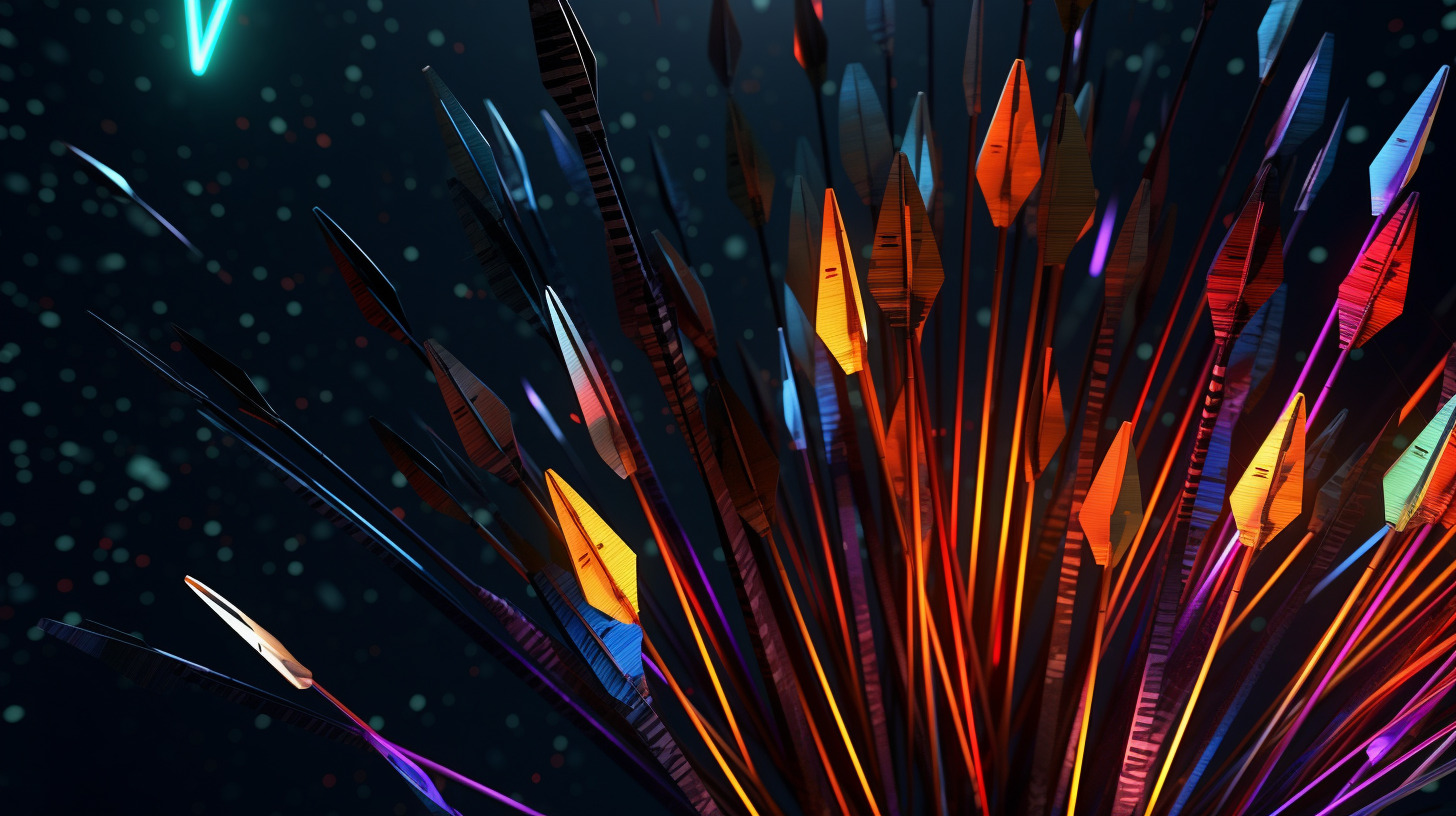
- Syntax and Usage of Arrow Functions
- Basic Syntax
- Implicit Return
- Lexical {{EJS41}} Binding
- When to Use Arrow Functions
- Implicit Return in Arrow Functions
- Using Arrow Functions with Higher Order Functions
- Arrow Functions and Lexical Scope
- The ‘this’ Keyword in Arrow Functions
- Arrow Functions vs Regular Functions
- Syntax
- Binding of {{EJS146}}
- Usage and Considerations
- Using Arrow Functions in Callbacks
- Arrow Functions in Iterators and Generators
- Iterators
- Generators
- Using Arrow Functions with Promises
- Arrow Functions in Event Handlers
- Using Arrow Functions with Object Methods
- Using Arrow Functions in React Components
- Advanced Techniques with Arrow Functions
- Lexical this
- Implicit return
- Using Arrow Functions with Higher-Order Functions
Syntax and Usage of Arrow Functions
ES6 introduced a new way of writing functions called arrow functions. Arrow functions provide a concise syntax for writing anonymous functions and offer some benefits over traditional function expressions. In this chapter, we will explore the syntax and usage of arrow functions in JavaScript.
Related Article: How To Generate Random String Characters In Javascript
Basic Syntax
The syntax of an arrow function is simple and straightforward. It consists of a parameter list (optional) wrapped in parentheses, followed by the arrow operator (=>
), and then the function body. Here is an example of a basic arrow function that takes no parameters and returns a hardcoded value:
const sayHello = () => { return "Hello, World!"; };
In the above example, the arrow function sayHello
takes no parameters and returns the string “Hello, World!”. The function body is enclosed in curly braces {}
, and the return statement is used to specify the value to be returned.
Implicit Return
Arrow functions provide a shorthand syntax for functions that have a single expression in the function body. If the function body consists of a single statement, you can omit the curly braces {}
and the return
keyword. The result of the expression will be automatically returned. Here’s an example:
const square = (num) => num * num;
In the above example, the arrow function square
takes a single parameter num
and returns the square of that number. Since the function body is a single expression (num * num
), we can omit the curly braces and the return
keyword.
Lexical this
Binding
One of the most significant advantages of arrow functions is their lexical this
binding. In regular functions, the value of this
is determined by how a function is called. However, arrow functions do not bind their own this
value but inherit it from the surrounding code. Let’s consider an example to better understand this concept:
function Person() { this.age = 0; setInterval(() => { this.age++; // 'this' refers to the Person object }, 1000); }
In the above example, we have a constructor function Person
that initializes an age
property with a value of 0. We then use setInterval
to increment the age
property every second. Inside the arrow function, this
refers to the Person
object, allowing us to access and modify its properties.
Related Article: How to Work with Async Calls in JavaScript
When to Use Arrow Functions
Arrow functions are especially useful in certain scenarios, such as:
– Callback functions: Arrow functions provide a concise syntax for callback functions, making the code more readable and reducing the need for bind()
or self
tricks.
– Iteration methods: Arrow functions can be used with iteration methods like map()
, filter()
, and reduce()
to write more concise and expressive code.
– Lexical scoping: The lexical this
binding of arrow functions makes them suitable for certain use cases, particularly when dealing with nested functions or asynchronous operations.
It’s important to note that arrow functions have some limitations, such as not having their own arguments
object and not being suitable for defining object methods due to their lack of a this
context.
In this chapter, we learned about the syntax and usage of arrow functions in JavaScript. We explored the basic syntax, implicit return, and the lexical this
binding feature. We also discussed when and where arrow functions can be beneficial.
Implicit Return in Arrow Functions
ES6 arrow functions provide a concise syntax for writing functions in JavaScript. One of the features of arrow functions is the ability to implicitly return a value without using the return
keyword. This can make the code more readable and reduce the amount of code you need to write.
To understand how implicit return works, let’s take a look at an example. Consider the following traditional function that adds two numbers:
function add(a, b) { return a + b; }
Using an arrow function, we can rewrite this code in a more concise way:
const add = (a, b) => a + b;
In this example, the arrow function automatically returns the sum of a
and b
. The return
keyword is not needed because the expression a + b
is implicitly returned.
Implicit return also works for single-line arrow functions that do not have curly braces. For example:
const square = x => x * x;
In this case, the arrow function takes a single parameter x
and returns the square of x
without using the return
keyword.
It’s worth noting that when using implicit return with arrow functions, you need to be careful with complex expressions or multiple statements. Implicit return is most suitable for simple, one-line expressions. If you need to write more complex logic or include multiple statements, it’s best to use the traditional function syntax.
Here’s an example where implicit return may not be suitable:
const divide = (a, b) => { if (b === 0) { throw new Error("Cannot divide by zero"); } return a / b; };
In this case, we have a conditional statement and a throw
statement, which makes the code more complex. Using explicit return with curly braces allows us to handle the error case and return the result when the division is possible.
In summary, implicit return in arrow functions is a powerful feature that allows you to write concise and readable code. It’s especially useful for simple, one-line expressions. However, for more complex logic or multiple statements, it’s best to use the traditional function syntax.
Using Arrow Functions with Higher Order Functions
Arrow functions are particularly useful when working with higher order functions. Higher order functions are functions that take one or more functions as arguments or return a function as a result. In this chapter, we will explore how arrow functions can be used effectively with higher order functions.
One common higher order function is map()
. The map()
function takes an array and applies a function to each element, creating a new array with the results. With arrow functions, we can write concise and readable code when using map()
. Here’s an example:
const numbers = [1, 2, 3, 4, 5]; const doubledNumbers = numbers.map((num) => num * 2); console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
In the example above, we use an arrow function to double each number in the numbers
array. The arrow function (num) => num * 2
takes a single argument num
and returns num * 2
. This concise syntax allows us to easily define the transformation logic for each element in the array.
Another higher order function where arrow functions shine is filter()
. The filter()
function takes an array and returns a new array with only the elements that pass a certain condition. Arrow functions can be used to write expressive and compact code when using filter()
. Here’s an example:
const numbers = [1, 2, 3, 4, 5]; const evenNumbers = numbers.filter((num) => num % 2 === 0); console.log(evenNumbers); // Output: [2, 4]
In this example, we use an arrow function (num) => num % 2 === 0
to filter out the odd numbers from the numbers
array. The arrow function takes a single argument num
and returns true
if num % 2 === 0
, indicating that the number is even.
Arrow functions can also be used with other higher order functions like reduce()
, forEach()
, and sort()
. When using arrow functions with these higher order functions, we can write more concise and readable code, making our intentions clear.
It’s worth noting that arrow functions have some differences in behavior compared to regular functions, especially when it comes to the this
keyword. When using arrow functions with higher order functions, it’s important to understand these differences and consider the implications. For more information on the behavior of arrow functions, refer to the previous chapters of this article.
Related Article: Understanding JavaScript Execution Context and Hoisting
Arrow Functions and Lexical Scope
One of the key benefits of arrow functions is their lexical scoping behavior, which differs from regular functions.
Arrow functions do not have their own this
value, arguments
object, super
keyword, or new.target
keyword. Instead, they inherit these values from the containing lexical scope. This means that the value of this
inside an arrow function is determined by the context in which the function is defined, not when it is called.
Let’s take a look at an example to understand this concept better:
// Regular function function regularFunction() { console.log(this); // logs the global object (window in a browser) } // Arrow function const arrowFunction = () => { console.log(this); // logs the global object (window in a browser) }; regularFunction(); arrowFunction();
In this code snippet, both the regular function regularFunction
and the arrow function arrowFunction
log the global object (window
in a browser). This is because arrow functions inherit the this
value from the containing scope, which in this case is the global scope.
Arrow functions are particularly useful when working with callbacks or event handlers, where maintaining the lexical scope is important. Let’s see an example:
const button = document.querySelector('button'); button.addEventListener('click', () => { console.log(this); // logs the button element });
In this code, the arrow function used as the event listener for the button’s click event logs the button element itself as the value of this
. This is because the arrow function inherits the this
value from its containing scope, which in this case is the event listener’s lexical scope.
Arrow functions also have a shorter syntax compared to regular functions, making them more concise and readable. They are especially useful when used in combination with array methods like map
, filter
, and reduce
. Here’s an example of using an arrow function with the map
method:
const numbers = [1, 2, 3, 4, 5]; const squaredNumbers = numbers.map((num) => num ** 2); console.log(squaredNumbers); // [1, 4, 9, 16, 25]
In this code, the arrow function passed to the map
method squares each number in the numbers
array. The result is a new array squaredNumbers
containing the squared values.
Arrow functions provide a concise and convenient way to write functions in JavaScript, with their lexical scoping behavior making them particularly useful in certain scenarios. Understanding how arrow functions interact with lexical scope is crucial for writing effective and maintainable code.
The ‘this’ Keyword in Arrow Functions
In JavaScript, the keyword this
is used to refer to the object that is executing the current code. It is a special keyword that can have different values depending on how a function is called.
Traditional JavaScript functions bind their own this
value, which means that the value of this
within a function can change depending on how the function is called. This behavior can be confusing and lead to unexpected bugs, especially when dealing with callbacks or event handlers.
However, arrow functions have a different behavior when it comes to the this
keyword. Unlike traditional functions, arrow functions do not bind their own this
value. Instead, they inherit the this
value from the surrounding scope.
This means that when you use this
inside an arrow function, it will always refer to the value of this
in the enclosing scope, regardless of how the function is called. This behavior is often referred to as “lexical scoping” or “lexical this”.
Let’s take a look at an example to better understand the difference between traditional functions and arrow functions when it comes to the this
keyword:
// Traditional function const obj1 = { name: 'John', sayHello: function() { console.log(`Hello, ${this.name}!`); } }; // Arrow function const obj2 = { name: 'Jane', sayHello: () => { console.log(`Hello, ${this.name}!`); } }; obj1.sayHello(); // Output: Hello, John! obj2.sayHello(); // Output: Hello, undefined!
In this example, obj1
is an object with a sayHello
method that uses a traditional function. When sayHello
is called on obj1
, the value of this
inside the function refers to obj1
, so it correctly logs “Hello, John!”.
On the other hand, obj2
is an object with a sayHello
method that uses an arrow function. When sayHello
is called on obj2
, the value of this
inside the function does not refer to obj2
. Instead, it refers to the this
value of the surrounding scope, which is the global object (window
in a browser or global
in Node.js) in this case. Since the global object does not have a name
property, the log statement outputs “Hello, undefined!”.
It’s important to note that arrow functions are not suitable for all scenarios. They are best used in situations where you want to preserve the value of this
from the surrounding scope, such as when defining methods within objects or when working with callbacks or event handlers.
To recap, arrow functions inherit the this
value from the surrounding scope, while traditional functions bind their own this
value. Understanding this difference is crucial when working with arrow functions to avoid unexpected behavior related to the this
keyword.
Arrow Functions vs Regular Functions
Arrow functions provide a more concise syntax compared to regular functions and offer some additional benefits. Let’s explore the differences between arrow functions and regular functions.
Related Article: How to Use Closures with JavaScript
Syntax
The syntax for arrow functions is quite different from regular functions. Arrow functions are defined using the arrow (=>
) syntax, which is where they get their name. Here’s an example of a simple arrow function that adds two numbers:
const add = (a, b) => a + b;
In the example above, the arrow function add
takes two parameters a
and b
and returns their sum using the concise arrow syntax. The curly braces {}
and the return
keyword are omitted because the arrow function implicitly returns the result of the expression.
On the other hand, regular functions are defined using the function
keyword and have a more verbose syntax. Here’s the equivalent regular function for the above arrow function:
function add(a, b) { return a + b; }
As you can see, regular functions require the function
keyword, parentheses ()
, curly braces {}
, and the return
keyword to define and return the result.
Binding of this
One of the significant differences between arrow functions and regular functions is how they handle the binding of the this
keyword.
In regular functions, the value of this
is determined by how the function is called. It can be influenced by factors like how the function is invoked, whether it is bound to a specific object, or if it is used as a method of an object. The value of this
can change dynamically.
On the other hand, arrow functions do not bind their own this
value. Instead, they inherit the this
value from the enclosing scope. This behavior can be beneficial in scenarios where you need to access the outer this
value inside a nested function.
Here’s an example that demonstrates the difference in the binding of this
between arrow functions and regular functions:
const person = { name: "John", sayHi: function() { console.log(`Hi, my name is ${this.name}.`); }, sayHiArrow: () => { console.log(`Hi, my name is ${this.name}.`); } }; person.sayHi(); // Output: Hi, my name is John. person.sayHiArrow(); // Output: Hi, my name is undefined.
In the example above, the sayHi
regular function correctly accesses the name
property of the person
object using this
. However, the sayHiArrow
arrow function does not bind its own this
value and ends up printing undefined
.
Usage and Considerations
Arrow functions are particularly useful in scenarios where you need to write shorter and more concise code. They are especially handy when working with array methods like map
, filter
, and reduce
, as they allow you to write more expressive code with fewer keystrokes.
However, there are some considerations to keep in mind when using arrow functions. For example, arrow functions cannot be used as constructor functions to create new objects, and they do not have their own arguments
object. Additionally, arrow functions are not suitable for methods that require the binding of this
to the object they are defined on.
It’s important to understand these differences and use arrow functions appropriately based on the specific requirements of your code.
In summary, arrow functions provide a concise syntax and offer benefits like lexical scoping of this
. However, they also have some limitations compared to regular functions. Understanding the differences between these two types of functions will help you make informed decisions when writing JavaScript code.
Now that we have explored the differences between arrow functions and regular functions, let’s move on to the next chapter where we will delve deeper into the various ways arrow functions can be used.
Related Article: How to Implement Functional Programming in JavaScript: A Practical Guide
Using Arrow Functions in Callbacks
Arrow functions are especially useful when working with callbacks, as they provide a more concise syntax compared to traditional function expressions.
Traditionally, when passing a function as a callback, you would use the function
keyword:
// Using a traditional function expression as a callback function myCallback(value) { console.log(value); } myArray.forEach(function (element) { myCallback(element); });
With arrow functions, the syntax becomes much simpler:
// Using an arrow function as a callback myArray.forEach((element) => { console.log(element); });
In this example, the arrow function (element) => { console.log(element); }
serves as the callback for the forEach
method. The arrow function takes one parameter, element
, and logs it to the console.
One of the main benefits of using arrow functions as callbacks is that they automatically inherit the this
value from their surrounding scope. This means that you don’t need to use the bind
method or create a closure to access the correct this
value within the callback.
Let’s take a look at an example:
const myObject = { name: 'John', sayHello: function () { setTimeout(() => { console.log(`Hello, ${this.name}!`); }, 1000); } }; myObject.sayHello(); // Output: Hello, John!
In this example, the arrow function () => { console.log(
, ${this.name}!); }
is used as the callback for the setTimeout
function. Since arrow functions don’t bind their own this
, they are able to access the this
value of the surrounding scope, which is the myObject
object in this case.
It’s important to note that arrow functions are not suitable for all callback scenarios. For example, if you need to dynamically bind the this
value or access the arguments object, you should use a traditional function expression instead.
Arrow functions provide a concise syntax for working with callbacks. They automatically inherit the this
value from their surrounding scope, making it easier to access the correct this
value within the callback. However, they are not a one-size-fits-all solution and should be used judiciously depending on the specific requirements of the callback.
Arrow Functions in Iterators and Generators
Arrow functions in JavaScript are not only useful for simplifying code syntax, but they also have specific advantages when used in iterators and generators. In this chapter, we will explore how arrow functions can be used in these contexts and the benefits they offer.
Iterators
Iterators are objects that define a sequence and potentially a return value upon reaching the end of that sequence. They are used in various JavaScript constructs, such as the for...of
loop, the Array.from()
method, and the spread operator.
Arrow functions are particularly useful when creating iterators. Let’s take a look at an example:
const numbers = [1, 2, 3, 4, 5]; const iterator = { [Symbol.iterator]: () => ({ next: () => ({ done: numbers.length === 0, value: numbers.shift() }) }) }; for (const number of iterator) { console.log(number); }
In this example, we create an iterator object that returns the next value from the numbers
array until it reaches the end. The arrow function syntax simplifies the code by removing the need for the function
keyword and allowing us to define the behavior of the iterator more concisely.
Related Article: How to Work with Big Data using JavaScript
Generators
Generators are a special type of iterator that allow pausing and resuming the execution of a function. They are denoted by the function*
syntax and make use of the yield
keyword.
Arrow functions can also be used in generators, making the code more concise and easier to read. Consider the following example:
function* fibonacci() { let current = 0; let next = 1; while (true) { yield current; [current, next] = [next, current + next]; } } const fib = fibonacci(); const fibSequence = Array.from(Array(10), () => fib.next().value); console.log(fibSequence);
In this example, we define a generator function fibonacci
that generates the Fibonacci sequence. We use the arrow function syntax within the Array.from()
method to create an array of the first 10 Fibonacci numbers. The arrow function simplifies the code and makes it easier to understand the intent of the Array.from()
method.
Arrow functions have truly enhanced the way we write iterators and generators in JavaScript. Their concise syntax and ability to capture the surrounding context make them a powerful tool for working with sequences of values.
Using Arrow Functions with Promises
Arrow functions are especially useful when working with promises, as they simplify the syntax and make the code more readable.
Promises are a JavaScript feature introduced in ES6 that allow you to work with asynchronous code in a more structured way. They represent the eventual completion or failure of an asynchronous operation and allow you to chain multiple asynchronous operations together.
When using arrow functions with promises, you can take advantage of the compact syntax to make your code more concise and easier to understand. Here’s an example that demonstrates how arrow functions can be used with promises:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Do something with the data }) .catch(error => { // Handle any errors });
In the code snippet above, we use the fetch()
function to make an HTTP request to an API endpoint. The fetch()
function returns a promise, which we can chain with the then()
method. The arrow function passed to the then()
method is executed when the promise is fulfilled, and it receives the response as a parameter.
Inside the arrow function, we use the json()
method of the response object to parse the response body as JSON data. The json()
method also returns a promise, so we can chain another then()
method to handle the parsed data.
If any errors occur during the promise chain, they are caught by the catch()
method. The arrow function passed to catch()
is executed when an error occurs, and it receives the error as a parameter.
By using arrow functions with promises, we can clearly see the flow of the asynchronous code and handle any errors in a concise and readable way. Additionally, arrow functions automatically bind the this
value to the surrounding context, which can be very helpful when working with promises.
It’s worth noting that arrow functions are not always the best choice when working with promises, especially when you need to access the this
value of the surrounding context. In those cases, a regular function expression may be more appropriate.
Overall, using arrow functions with promises can greatly improve the readability and maintainability of your code. They provide a concise syntax that allows you to express your intent more clearly and handle asynchronous operations in a structured way.
Arrow Functions in Event Handlers
One common use case for arrow functions is in event handlers.
In traditional JavaScript, event handlers are often written as anonymous functions. For example, let’s say we have a button with an id of “myButton” that we want to add a click event to:
document.getElementById("myButton").addEventListener("click", function() { console.log("Button clicked"); });
With arrow functions, we can rewrite this event handler in a more concise way:
document.getElementById("myButton").addEventListener("click", () => { console.log("Button clicked"); });
Arrow functions have a few advantages when it comes to event handlers. First, they have a shorter syntax, which can make your code more readable and maintainable. Second, arrow functions do not bind their own “this” value. Instead, they inherit the “this” value from the surrounding context. This means that you can access the correct “this” value without having to use workarounds like creating a reference to “this” with a variable or using the “bind” method.
Let’s take a look at an example to demonstrate this:
const myObject = { name: "John", sayHello: function() { document.getElementById("myButton").addEventListener("click", () => { console.log("Hello, " + this.name); }); } }; myObject.sayHello();
In the example above, we have an object called “myObject” with a property called “name” and a method called “sayHello”. Inside the “sayHello” method, we add a click event listener to the button with an id of “myButton”. The event handler is an arrow function that logs a greeting using the “name” property of “myObject”. Since arrow functions inherit the “this” value from the surrounding context, we can access the correct value of “this” inside the event handler.
Arrow functions in event handlers can be a powerful tool for writing concise and maintainable code in JavaScript. They provide a more elegant way to define event handlers and automatically capture the correct “this” value. However, it’s worth noting that arrow functions are not always the best choice for event handlers, especially if you need to access the event object or remove the event listener later on.
Related Article: How to Use Classes in JavaScript
Using Arrow Functions with Object Methods
ES6 arrow functions can also be used effectively with object methods. Object methods are functions that are defined as properties of an object.
When using arrow functions as object methods, there are a few things to keep in mind. First, arrow functions do not have their own this
value. Instead, they inherit the this
value from the surrounding scope. This means that when an arrow function is used as an object method, this
inside the arrow function refers to the object itself, rather than the function.
Let’s take a look at an example to see how arrow functions can be used with object methods:
const person = { name: 'John', age: 30, greet: () => { console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`); } }; person.greet(); // Output: Hello, my name is undefined and I am undefined years old.
In the above example, the greet
method is defined as an arrow function. However, when we try to access the name
and age
properties using this
, we get undefined
because arrow functions do not have their own this
value.
To overcome this issue, it is recommended to use regular functions instead of arrow functions when defining object methods that need to access the object’s properties or methods. Regular functions have their own this
value, which is automatically set to the object that the method is called on.
Here’s an updated version of the previous example using a regular function:
const person = { name: 'John', age: 30, greet: function() { console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`); } }; person.greet(); // Output: Hello, my name is John and I am 30 years old.
In this updated example, greet
is defined as a regular function. When we call person.greet()
, this
inside the function refers to the person
object, allowing us to access the name
and age
properties correctly.
While arrow functions can be used with object methods, it is important to consider the behavior of this
. If you need to access the object’s properties or methods within the method, it is recommended to use regular functions instead of arrow functions.
Using Arrow Functions in React Components
React is a popular JavaScript library for building user interfaces, and it provides a way to create reusable components. Arrow functions have some advantages over regular functions, especially when it comes to using them in React components.
One major benefit of using arrow functions in React components is that they automatically bind the context of the component to the function. This means that you don’t need to use the bind()
method or the this
keyword to access the component’s properties and methods within the function. Here’s an example:
class MyComponent extends React.Component { handleClick = () => { console.log(this.props.someProp); } render() { return ( <button>Click me</button> ); } }
In the above example, the handleClick
function is defined as an arrow function. When the button is clicked, the function will be called with the correct context, so we can access the this.props.someProp
property without any issues.
Another advantage of arrow functions in React components is that they provide a more concise syntax. Arrow functions allow you to omit the function
keyword and use an implicit return if the function body consists of a single expression. This can make your code cleaner and easier to read. Here’s an example:
class MyComponent extends React.Component { render() { return ( <div> {this.props.items.map(item => <div>{item}</div>)} </div> ); } }
In the above example, we use an arrow function as the callback for the map()
method. The arrow function takes each item in the items
array and returns a <div>
element with the item as its content. This concise syntax makes it clear what the function does without the need for extra boilerplate code.
It’s worth noting that arrow functions are not always the best choice in every situation. They have some limitations, such as not having their own this
value and not being able to be used as constructors. It’s important to understand these limitations and use arrow functions appropriately in your React components.
In this chapter, we explored the benefits of using arrow functions in React components. We saw how arrow functions automatically bind the context of the component, making it easier to access properties and methods. We also learned how arrow functions provide a more concise syntax, making our code cleaner and easier to read. However, it’s important to be aware of the limitations of arrow functions and use them appropriately in our code.
Advanced Techniques with Arrow Functions
While arrow functions are generally used as a replacement for traditional function expressions, they also offer some advanced techniques that can be useful in certain scenarios. In this chapter, we will explore some of these advanced techniques with arrow functions.
Related Article: JavaScript Spread and Rest Operators Explained
Lexical this
One of the most significant advantages of arrow functions is their lexical scoping of the this
keyword. Unlike traditional functions, arrow functions do not bind their own this
value. Instead, they inherit the this
value from the surrounding scope.
Consider the following code snippet:
const obj = { name: 'John', sayHello: function() { setTimeout(() => { console.log(`Hello, ${this.name}!`); }, 1000); } }; obj.sayHello(); // Output: Hello, John!
In this example, the arrow function inside the setTimeout
callback will correctly reference the name
property of the obj
object. If we had used a traditional function expression instead, the this
value would have been bound to the setTimeout
function itself, resulting in an error or an incorrect output.
This lexical scoping of this
is especially useful when working with event handlers or asynchronous operations, as it eliminates the need for explicit binding or using the bind
method.
Implicit return
Arrow functions also have an implicit return behavior, which means that if the function body consists of a single expression, that expression is implicitly returned without needing the return
keyword.
Consider the following code snippet:
const numbers = [1, 2, 3, 4, 5]; const doubled = numbers.map((num) => num * 2); console.log(doubled); // Output: [2, 4, 6, 8, 10]
In this example, the arrow function passed to the map
method implicitly returns the result of multiplying each number by 2. This concise syntax can greatly simplify code and make it more readable.
However, it’s important to note that the implicit return behavior only applies when the function body is a single expression. If you need to perform multiple statements or have a more complex function body, you should use curly braces and explicitly return the value.
Using Arrow Functions with Higher-Order Functions
Arrow functions can be particularly useful when working with higher-order functions, such as map
, filter
, and reduce
. Their concise syntax and lexical scoping make them a natural fit for these functional programming paradigms.
For example, let’s say we have an array of objects representing books, and we want to filter out the books written by a specific author. We can achieve this using the filter
method and an arrow function:
const books = [ { title: 'The Great Gatsby', author: 'F. Scott Fitzgerald' }, { title: 'To Kill a Mockingbird', author: 'Harper Lee' }, { title: 'Pride and Prejudice', author: 'Jane Austen' }, ]; const author = 'F. Scott Fitzgerald'; const booksByAuthor = books.filter((book) => book.author === author); console.log(booksByAuthor);
In this example, the arrow function filters out the books whose author matches the specified author
variable. The result is an array containing only the books written by F. Scott Fitzgerald.
Arrow functions can also be used with other higher-order functions like map
and reduce
to perform transformations and aggregations on arrays.