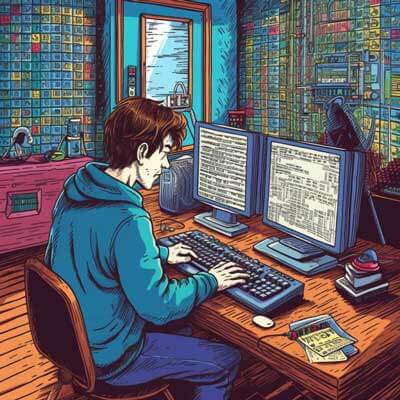
NgClass is a built-in directive in Angular that allows you to conditionally apply CSS classes to HTML elements based on certain conditions. It is a powerful tool that helps you dynamically change the appearance of your application based on different states or user interactions. In this guide, we will explore how to use NgClass for Angular conditional classes.
Why is this question asked?
The question “How to use NgClass for Angular conditional classes?” is commonly asked by developers who are new to Angular or are looking for a way to dynamically apply classes to their HTML elements based on specific conditions. By understanding how to use NgClass, developers can enhance the user experience by altering the styling of elements in response to different events or states.
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
How to use NgClass for Angular conditional classes
To use NgClass for conditional classes in Angular, follow these steps:
Step 1: Import the NgClass directive
First, make sure you have imported the NgClass directive from the @angular/common module in your component file. This allows you to use the directive in your HTML template.
import { NgClass } from '@angular/common';
Step 2: Define the conditions
Next, define the conditions that determine whether a specific class should be applied or not. You can use any expression that evaluates to a boolean value as a condition. For example, you can use properties from your component’s class or expressions that rely on user interactions.
isActive: boolean = true; isHighlighted: boolean = false;
Step 3: Apply the NgClass directive
In your HTML template, apply the NgClass directive to the element you want to conditionally style. Use the NgClass directive as an attribute and provide it with an object that maps the CSS class name to the condition.
<div>...</div>
In this example, the CSS class ‘active’ will be applied to the div if the isActive property is true. Similarly, the CSS class ‘highlighted’ will be applied if the isHighlighted property is true.
Step 4: Add additional classes
You can also include additional classes that are always applied to the element by specifying them as regular class attributes.
<div class="default-class">...</div>
In this example, the ‘default-class’ will always be applied to the div, regardless of the conditions specified in the NgClass directive.
Step 5: Toggling classes dynamically
To dynamically toggle the classes based on user interactions or other events, you can update the values of the properties used in the NgClass directive.
toggleActive() { this.isActive = !this.isActive; } toggleHighlighted() { this.isHighlighted = !this.isHighlighted; }
In this example, the toggleActive() and toggleHighlighted() methods can be called to toggle the values of the isActive and isHighlighted properties, respectively. As a result, the classes will be added or removed accordingly.
Best Practices and Suggestions
When using NgClass for conditional classes in Angular, consider the following best practices and suggestions:
– Use meaningful class names: Choose class names that accurately describe the purpose or state of the element you are styling. This makes your code more readable and maintainable.
– Keep the conditions simple: Avoid complex expressions in the NgClass directive. If a condition becomes too complex, consider moving it to a method in your component’s class and use the method’s return value as the condition.
– Combine with ngStyle: NgClass can be combined with ngStyle to apply inline styles conditionally. This allows you to dynamically change both classes and styles based on specific conditions.
– Use class binding syntax: Instead of using the NgClass directive, you can also use the class binding syntax to conditionally apply classes. This can be useful when you have multiple conditions or want to apply multiple classes based on a single condition.
<div>...</div>
Related Article: How To Use Loop Inside React JSX