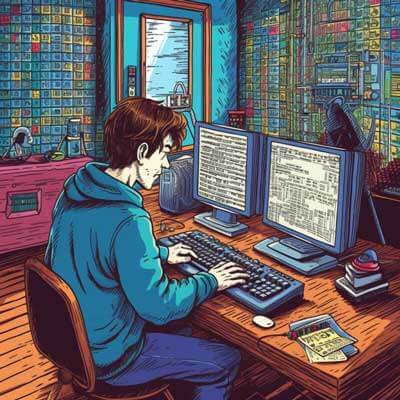
Table of Contents
Appending to a JavaScript Array
Appending to a JavaScript array is a common operation that allows you to add new elements to an existing array. In JavaScript, arrays are dynamic and can grow or shrink in size as needed. Appending to an array can be done using several methods. In this answer, we will explore two common approaches: the push()
method and the array concatenation operator (+
).
Related Article: Resolving Declaration File Issue for Vue-Table-Component
Using the push() method
The push()
method is a built-in JavaScript method that allows you to add one or more elements to the end of an array. Here's how you can use it:
let fruits = ['apple', 'banana', 'orange']; fruits.push('pear'); console.log(fruits); // Output: ['apple', 'banana', 'orange', 'pear']
In the example above, we have an array called fruits
containing three elements: 'apple', 'banana', and 'orange'. We then use the push()
method to append the string 'pear' to the end of the array. The resulting array is ['apple', 'banana', 'orange', 'pear']
.
The push()
method also allows you to append multiple elements at once. Here's an example:
let numbers = [1, 2, 3]; numbers.push(4, 5, 6); console.log(numbers); // Output: [1, 2, 3, 4, 5, 6]
In this example, we have an array called numbers
containing three elements: 1, 2, and 3. We then use the push()
method to append the numbers 4, 5, and 6 to the end of the array. The resulting array is [1, 2, 3, 4, 5, 6]
.
Using the array concatenation operator
Another way to append elements to a JavaScript array is by using the array concatenation operator, which is the plus sign (+
). This operator allows you to concatenate two or more arrays together, effectively appending one array to another. Here's an example:
let animals = ['cat', 'dog']; let newAnimals = animals.concat(['elephant', 'lion']); console.log(newAnimals); // Output: ['cat', 'dog', 'elephant', 'lion']
In this example, we have an array called animals
containing two elements: 'cat' and 'dog'. We then use the array concatenation operator to append a new array containing the strings 'elephant' and 'lion'. The resulting array is ['cat', 'dog', 'elephant', 'lion']
.
It's important to note that the original arrays are not modified when using the array concatenation operator. Instead, a new array is created with the concatenated elements.
Why is this question asked?
The question "How to append to a JavaScript array?" may arise for several reasons. Here are a few potential scenarios:
1. Adding new elements to an existing array**: When working with arrays in JavaScript, you may need to add new elements to an array dynamically. This is common when dealing with user input, data manipulation, or dynamically generated content.
2. **Building an array incrementally**: In some cases, you may want to build an array incrementally by appending elements to it one at a time. This can be useful when processing data or creating a result set.
Related Article: How to Use the JSON.parse() Stringify in Javascript
Suggestions and alternative ideas
While the push()
method and array concatenation operator are common ways to append elements to a JavaScript array, there are other approaches you can consider depending on your specific use case:
1. **Spread syntax**: The spread syntax allows you to expand an array into individual elements. You can use it to append elements from one array to another. Here's an example:
let fruits = ['apple', 'banana', 'orange']; let newFruits = [...fruits, 'pear']; console.log(newFruits); // Output: ['apple', 'banana', 'orange', 'pear']
In this example, we use the spread syntax to create a new array called newFruits
. We expand the fruits
array using the spread syntax and append the string 'pear' to it. The resulting array is ['apple', 'banana', 'orange', 'pear']
.
2. **Array destructuring**: Array destructuring allows you to extract specific elements from an array and assign them to variables. You can use it to append elements to an existing array. Here's an example:
let fruits = ['apple', 'banana', 'orange']; let [first, second, ...rest] = fruits; let newFruits = [first, second, ...rest, 'pear']; console.log(newFruits); // Output: ['apple', 'banana', 'orange', 'pear']
In this example, we use array destructuring to extract the first two elements from the fruits
array and assign them to variables first
and second
. The rest of the elements are collected into an array called rest
. We then create a new array called newFruits
by combining the extracted elements, the rest of the elements, and the string 'pear'. The resulting array is ['apple', 'banana', 'orange', 'pear']
.
Best practices
When appending elements to a JavaScript array, keep the following best practices in mind:
1. **Choose the right method**: The push()
method is a simple and straightforward way to append elements to an array, especially when adding one or more elements at the end. However, if you need more flexibility or want to concatenate multiple arrays together, the array concatenation operator or other methods like spread syntax or array destructuring may be more suitable.
2. **Avoid mutating the original array**: When appending elements to an array, it's generally a good practice to avoid modifying the original array directly. Instead, create a new array with the appended elements using the push()
method, array concatenation, or other methods. This helps prevent unexpected side effects and makes your code more predictable and maintainable.
3. **Consider immutability**: In functional programming, immutability is a key concept. Instead of modifying existing arrays, you can create new arrays that include the existing elements along with the new ones. This promotes cleaner code and helps prevent accidental modification of shared data.
4. **Think about performance: While the push()
method and array concatenation are efficient for appending a few elements, they can become less performant when dealing with large arrays or frequent appends. In such cases, using other data structures like linked lists or considering more specialized libraries or frameworks may be beneficial.