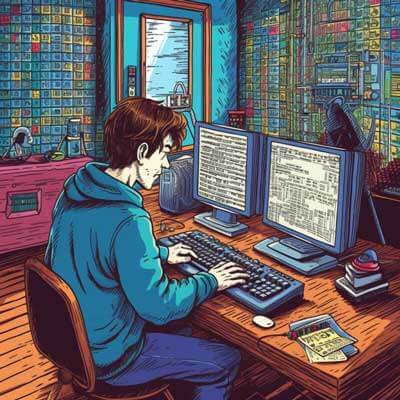
Table of Contents
What is Next.js?
Next.js is a popular framework for building server-side rendered (SSR) React applications. It provides a useful toolset for creating dynamic and performant web applications, with features such as automatic code splitting, server-side rendering, and static site generation. Next.js simplifies the development process by handling many of the complexities associated with building modern web applications, allowing developers to focus on writing code rather than configuring build tools.
Related Article: Javascript Template Literals: A String Interpolation Guide
Why use global CSS imports in Next.js?
Global CSS imports in Next.js allow you to apply CSS styles to your entire application consistently. This is useful when you have CSS rules that need to be shared across multiple components or pages. By importing CSS files globally, you can ensure a consistent look and feel throughout your application and avoid duplicating styles in multiple places.
How can I import a CSS file globally in Next.js?
To import a CSS file globally in Next.js, you can use the built-in CSS support provided by the framework. Next.js supports both CSS modules and global CSS imports. CSS modules allow you to scope CSS styles to specific components, while global CSS imports apply styles globally across the entire application.
To import a CSS file globally, you can use the import
statement in your JavaScript or TypeScript files. This will ensure that the CSS file is included in the final bundle and applied to all pages or components that import it.
Code Snippet: Importing a CSS file globally in Next.js
// styles/global.css body { background-color: #f5f5f5; font-family: Arial, sans-serif; } // pages/index.js import '../styles/global.css'; function HomePage() { return ( <div> <h1>Welcome to my Next.js app</h1> <p>This is an example of a globally styled Next.js page.</p> </div> ); } export default HomePage;
In the above example, we have a global.css
file that contains some global CSS styles. We import this file in our index.js
page using the relative path ../styles/global.css
. The styles defined in global.css
will be applied to all pages in the application.
Related Article: How to Use 'This' Keyword in jQuery
What is the best way to include global CSS in Next.js?
The best way to include global CSS in Next.js is by using a custom _app.js
file. The _app.js
file is a special Next.js file that acts as a wrapper for all pages in your application. It allows you to override the default App
component provided by Next.js and customize how your application is rendered.
To include global CSS in Next.js using a custom _app.js
file, you can import the CSS file within the component and use the Component
prop to render the actual page. This ensures that the CSS styles are applied to all pages in your application.
Code Snippet: Including global CSS in Next.js using a custom _app.js file
// pages/_app.js import '../styles/global.css'; function MyApp({ Component, pageProps }) { return ; } export default MyApp;
In the above example, we import the global.css
file in our custom _app.js
file. The MyApp
component receives the Component
and pageProps
props from Next.js and renders the actual page using the spread operator ({...pageProps}
). This ensures that the CSS styles are applied to all pages in your application.
Is it possible to import a CSS file globally in Next.js?
Yes, it is possible to import a CSS file globally in Next.js. As mentioned earlier, Next.js supports global CSS imports, allowing you to apply CSS styles to your entire application consistently.
Code Snippet: Importing CSS globally in Next.js using a layout component
// styles/global.css body { background-color: #f5f5f5; font-family: Arial, sans-serif; } // components/Layout.js import React from 'react'; import '../styles/global.css'; function Layout({ children }) { return <div>{children}</div>; } export default Layout; // pages/index.js import Layout from '../components/Layout'; function HomePage() { return ( <h1>Welcome to my Next.js app</h1> <p>This is an example of a globally styled Next.js page.</p> ); } export default HomePage;
In the above example, we have a global.css
file that contains some global CSS styles. We import this file in our Layout.js
component, which acts as a layout wrapper for all pages in our application. The styles defined in global.css
will be applied to all pages wrapped by the Layout
component.
Related Article: JavaScript Prototype Inheritance Explained (with Examples)
How do I style my Next.js components using global CSS?
To style your Next.js components using global CSS, you can import the CSS file within the component and apply the styles directly to the elements using CSS classes or inline styles.
Code Snippet: Styling Next.js components using global CSS
// styles/global.css .button { background-color: #f5f5f5; color: #333; padding: 10px; border-radius: 4px; } // components/Button.js import React from 'react'; import '../styles/global.css'; function Button({ text }) { return <button>{text}</button>; } export default Button; // pages/index.js import Button from '../components/Button'; function HomePage() { return ( <div> <h1>Welcome to my Next.js app</h1> <Button /> </div> ); } export default HomePage;
In the above example, we have a global.css
file that contains a CSS class called .button
. We import this file in our Button.js
component and apply the class to the button element. The styles defined in global.css
will be applied to the button component.
Can I import a CSS file in Next.js that will be applied to all pages?
Yes, you can import a CSS file in Next.js that will be applied to all pages by using the custom _app.js
file. As mentioned earlier, the _app.js
file acts as a wrapper for all pages in your application and allows you to customize the rendering process.
Code Snippet: Importing a CSS file in Next.js to apply to all pages
// styles/global.css body { background-color: #f5f5f5; font-family: Arial, sans-serif; } // pages/_app.js import '../styles/global.css'; function MyApp({ Component, pageProps }) { return ; } export default MyApp;
In the above example, we import the global.css
file in our custom _app.js
file. The styles defined in global.css
will be applied to all pages in your application.
Related Article: Executing a React Component in a JavaScript File
What are the different methods to import CSS globally in Next.js?
There are multiple methods to import CSS globally in Next.js. Some of the common methods include:
1. Importing the CSS file directly in the JavaScript or TypeScript files using the import
statement.
2. Using a custom _app.js
file to import the CSS file and apply the styles to all pages.
3. Importing the CSS file in a layout component and wrapping all pages with the layout component.
Code Snippet: Different methods to import CSS globally in Next.js
// Method 1: Importing CSS directly in JavaScript/TypeScript files import '../styles/global.css'; // Method 2: Using a custom _app.js file // pages/_app.js import '../styles/global.css'; function MyApp({ Component, pageProps }) { return ; } export default MyApp; // Method 3: Importing CSS in a layout component // styles/global.css body { background-color: #f5f5f5; font-family: Arial, sans-serif; } // components/Layout.js import React from 'react'; import '../styles/global.css'; function Layout({ children }) { return <div>{children}</div>; } export default Layout; // pages/index.js import Layout from '../components/Layout'; function HomePage() { return ( <h1>Welcome to my Next.js app</h1> <p>This is an example of a globally styled Next.js page.</p> ); } export default HomePage;
In the above example, we have demonstrated three different methods to import CSS globally in Next.js. Method 1 shows how to import CSS directly in JavaScript or TypeScript files. Method 2 demonstrates using a custom _app.js
file to import the CSS file and apply styles to all pages. Method 3 showcases importing CSS in a layout component and wrapping all pages with the layout component.
Does Next.js support global CSS imports?
Yes, Next.js supports global CSS imports out of the box. You can import CSS files globally and have them applied to all pages in your Next.js application.
Code Snippet: Checking if Next.js supports global CSS imports
// pages/index.js import '../styles/global.css'; function HomePage() { return ( <div> <h1>Welcome to my Next.js app</h1> <p>This is an example of a globally styled Next.js page.</p> </div> ); } export default HomePage;
In the above example, we import a CSS file globally in our index.js
page using the relative path ../styles/global.css
. The styles defined in global.css
will be applied to all pages in the Next.js application.
Related Article: Utilizing Debugger Inside GetInitialProps in Next.js
Are there any limitations when importing CSS files globally in Next.js?
When importing CSS files globally in Next.js, there are a few limitations to keep in mind:
1. CSS files imported globally may increase the size of your application bundle, leading to longer load times.
2. Global CSS styles can potentially interfere with the styles of third-party libraries or components used in your application.
3. Hot module replacement (HMR) may not work as expected when using global CSS imports, requiring a full page refresh to see style changes.
4. CSS rules imported globally may not be properly scoped, leading to potential clashes or unintended styling effects.
It's important to consider these limitations and use global CSS imports judiciously in your Next.js application.
Code Snippet: Handling limitations when importing CSS files globally in Next.js
// styles/global.css body { background-color: #f5f5f5; font-family: Arial, sans-serif; } // pages/index.js import Head from 'next/head'; function HomePage() { return ( <div> <h1>Welcome to my Next.js app</h1> <p>This is an example of a globally styled Next.js page.</p> </div> ); } export default HomePage;
In the above example, we use the Head
component from the next/head
package to import the CSS file globally via a link tag. This allows us to mitigate some of the limitations of global CSS imports in Next.js, such as reducing bundle size and scoping CSS rules properly.
How does Next.js handle global CSS imports during server-side rendering?
Next.js handles global CSS imports during server-side rendering (SSR) by injecting the CSS styles into the generated HTML markup. This ensures that the styles are applied correctly when a page is initially loaded.
During the SSR process, Next.js collects all the CSS imports from your application and includes them in the generated HTML markup. This allows the styles to be applied before the page is rendered on the client side.
It's important to note that Next.js optimizes the CSS handling during SSR by only including the CSS that is actually used on each page. This helps to minimize the size of the generated HTML and improve performance.
Code Snippet: Handling global CSS imports during server-side rendering in Next.js
// styles/global.css body { background-color: #f5f5f5; font-family: Arial, sans-serif; } // pages/index.js import '../styles/global.css'; function HomePage() { return ( <div> <h1>Welcome to my Next.js app</h1> <p>This is an example of a globally styled Next.js page.</p> </div> ); } export default HomePage;
In the above example, we import a CSS file globally in our index.js
page using the relative path ../styles/global.css
. Next.js will handle the global CSS import during server-side rendering and inject the styles into the generated HTML markup. This ensures that the styles are applied correctly when the page is initially loaded.
Related Article: How to Check if a String Matches a Regex in JS
Can I use CSS modules for global styles in Next.js?
While CSS modules are primarily designed for scoping CSS styles to specific components, you can use them for global styles in Next.js as well. However, it's important to note that using CSS modules for global styles may not be the best approach, as it goes against the intended use case of CSS modules.
CSS modules are typically used to encapsulate styles within a component, preventing global style conflicts and making it easier to manage styles at a component level. When used for global styles, CSS modules may introduce unnecessary complexity and make it harder to maintain and update styles across your application.
It's recommended to use global CSS imports for global styles in Next.js, as it provides a simpler and more straightforward approach to applying styles globally.
Code Snippet: Using CSS modules for global styles in Next.js
// styles/global.module.css body { background-color: #f5f5f5; font-family: Arial, sans-serif; } // pages/index.js import styles from '../styles/global.module.css'; function HomePage() { return ( <div> <h1>Welcome to my Next.js app</h1> <p>This is an example of a globally styled Next.js page.</p> </div> ); } export default HomePage;
In the above example, we define a CSS module called global.module.css
, which contains a CSS class called body
. We import this CSS module in our index.js
page and apply the body
class to the root element of the page. While this approach technically works, it's not recommended for global styles in Next.js.
Additional Resources
- Next.js GitHub Repository: Styling and CSS