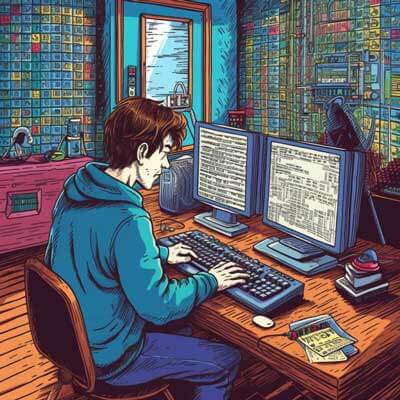
Checking whether a string contains a specific substring is a common task in JavaScript development. There are several methods you can use to accomplish this, depending on your requirements and the version of JavaScript you are working with.
1. Using the includes() method
One of the simplest and most straightforward ways to check if a string contains a substring in JavaScript is by using the includes()
method. This method returns a boolean value indicating whether the target string contains the specified substring.
Here’s an example that demonstrates the usage of the includes()
method:
const str = 'Hello, World!'; const substring = 'Hello'; console.log(str.includes(substring)); // Output: true
In the above example, the includes()
method is called on the str
string with the substring
as the argument. The method returns true
since the substring “Hello” is present in the target string “Hello, World!”.
It’s important to note that the includes()
method is case-sensitive. So if you are looking for a case-insensitive search, you will need to convert both the target string and the substring to lowercase or uppercase before performing the check.
Related Article: How To Check If a Key Exists In a Javascript Object
2. Using the indexOf() method
Another approach to check if a string contains a substring is by using the indexOf()
method. This method returns the index of the first occurrence of the specified substring within the target string. If the substring is not found, it returns -1.
Here’s an example that demonstrates the usage of the indexOf()
method:
const str = 'Hello, World!'; const substring = 'Hello'; console.log(str.indexOf(substring) !== -1); // Output: true
In the above example, the indexOf()
method is called on the str
string with the substring
as the argument. The method returns the index of the first occurrence of the substring “Hello” in the target string “Hello, World!”. Since the returned index is not -1, we can infer that the substring is present in the target string.
If you only need to check if the substring exists in the target string and don’t require the index, you can modify the condition as follows:
console.log(str.indexOf(substring) >= 0); // Output: true
The indexOf()
method is useful when you need to perform additional operations based on the index of the substring, such as extracting a portion of the string or replacing the substring with another value.
Why is this question asked?
This question is commonly asked by JavaScript developers who need to determine whether a string contains a specific substring. By understanding the various methods available to accomplish this task, developers can write more efficient and concise code.
Some potential reasons why this question is asked include:
– Validating user input: When building applications that accept user input, it’s important to validate and sanitize the data. Checking if a string contains a substring can help ensure that the input meets certain criteria or restrictions.
– Searching and filtering data: In scenarios where you need to search or filter data based on specific criteria, checking if a string contains a substring can be extremely useful. This could include searching for keywords in a text document or filtering a list of items based on user-defined criteria.
– String manipulation and formatting: Sometimes, you may need to manipulate or format strings based on certain conditions. Checking if a string contains a substring allows you to conditionally perform operations like adding or removing characters, replacing substrings, or formatting the string in a particular way.
Suggestions and alternative ideas
While the includes()
and indexOf()
methods are the most common approaches to check if a string contains a substring in JavaScript, there are a few alternative ideas and suggestions you can consider depending on your specific use case:
– Regular expressions: JavaScript provides powerful regular expression capabilities through the RegExp
object. By constructing a regular expression pattern, you can perform more complex substring matching and pattern recognition. The test()
method of the RegExp
object can be used to check if a string matches a given pattern.
const str = 'Hello, World!'; const pattern = /hello/i; // Case-insensitive matching console.log(pattern.test(str)); // Output: true
In the above example, the regular expression pattern /hello/i
is used to match the substring “Hello” case-insensitively. The test()
method returns true
since the pattern is found in the target string “Hello, World!”.
– String.prototype.search(): Another option is to use the search()
method, which also accepts regular expressions as the search pattern. This method returns the index of the first match or -1 if no match is found.
const str = 'Hello, World!'; const pattern = /hello/i; console.log(str.search(pattern) !== -1); // Output: true
In the above example, the search()
method is used to determine if the substring “Hello” exists in the target string “Hello, World!”.
– Third-party libraries: If you are working on a larger project or require more advanced string manipulation capabilities, you may consider using third-party libraries such as Lodash or Underscore.js. These libraries provide additional utility functions for working with strings, including substring checks.
Related Article: How To Use the Javascript Void(0) Operator
Best practices
When checking if a string contains a substring in JavaScript, it’s important to consider the following best practices:
– Case sensitivity: By default, the includes()
, indexOf()
, and search()
methods are case-sensitive. If you require a case-insensitive search, you can convert both the target string and the substring to lowercase or uppercase using the toLowerCase()
or toUpperCase()
methods before performing the check.
– Performance considerations: If you need to perform multiple substring checks on the same target string, it is more efficient to use the indexOf()
method and store the index in a variable. This avoids unnecessary repeated searches.
– Error handling: When using the indexOf()
method, keep in mind that it returns -1 when the substring is not found. Make sure to handle this case appropriately in your code to avoid unexpected behavior.
– Regular expressions: If you decide to use regular expressions for substring matching, be aware of their potential complexity and performance implications. Regular expressions can be powerful but may also introduce overhead if used unnecessarily.