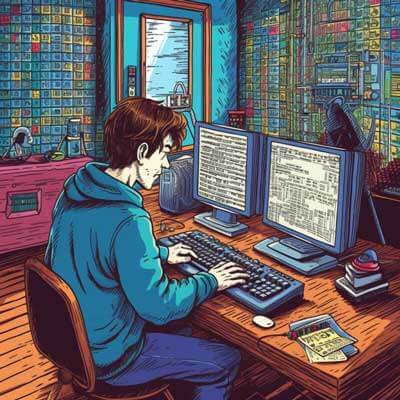
Table of Contents
To scroll to an element with jQuery, you can use the scrollTop
method along with the offset
method to calculate the position of the element relative to the top of the page. Here are two possible approaches:
Approach 1: Smooth Scroll
To achieve a smooth scrolling effect, you can use the animate
method in jQuery to gradually scroll to the desired element. Here's an example:
$('html, body').animate({ scrollTop: $('#target-element').offset().top }, 1000); // Adjust the duration (in milliseconds) as needed
In this example, $('html, body')
selects both the html
and body
elements, which covers most scenarios for scrolling. The animate
method is then called with the scrollTop
property set to the offset of the target element's top position. The 1000
parameter specifies the duration of the animation in milliseconds.
Related Article: How to Parse a String to a Date in JavaScript
Approach 2: Instant Scroll
If you prefer an instant scroll without animation, you can use the scrollTop
method directly. Here's an example:
$(window).scrollTop($('#target-element').offset().top);
In this example, $(window)
selects the window object, and the scrollTop
method is called with the offset of the target element's top position.
Best Practices and Alternative Ideas
Related Article: How to Convert a Number to a String in Javascript
- It is recommended to wrap the scrolling code inside a function and attach it to a click event or any other appropriate user action.
- To ensure smooth scrolling on all browsers, consider including a polyfill for the requestAnimationFrame
method. This can help improve performance and user experience.
- If your page has a fixed header or other elements that may obstruct the target element, you can subtract the height of those elements from the scroll position. This can be done using the outerHeight
method. Example:
var headerHeight = $('header').outerHeight(); var targetOffset = $('#target-element').offset().top - headerHeight; $('html, body').animate({ scrollTop: targetOffset }, 1000);
- If you need to scroll to a specific position on the page rather than an element, you can pass the desired pixel value to the scrollTop
method directly.
- If you are working with a single-page application (SPA) or have dynamically loaded content, you may need to wait for the content to be fully loaded before scrolling. You can achieve this by placing the scrolling code inside the window.onload
event or using the $(document).ready()
function.
- If you prefer a more lightweight solution without jQuery, you can achieve the same result using vanilla JavaScript by manipulating the scrollTo
or scrollIntoView
methods.