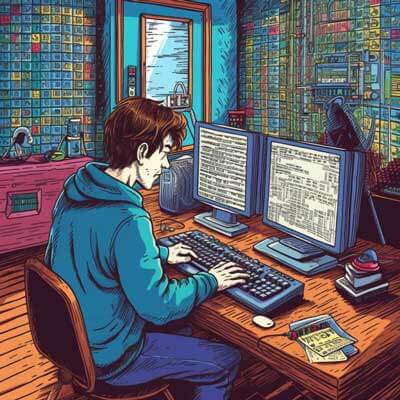
Converting JSON data to CSV format in Python can be achieved using various libraries and techniques. In this answer, we will explore two popular methods to accomplish this task.
Method 1: Using the json and csv libraries
The json and csv libraries in Python provide built-in functions that make it easy to convert JSON data to CSV format. Here is a step-by-step guide on how to do this:
1. Import the required libraries:
import json import csv
2. Load the JSON data from a file or a string:
data = ''' [ { "name": "John", "age": 30, "city": "New York" }, { "name": "Jane", "age": 25, "city": "San Francisco" } ] ''' json_data = json.loads(data)
3. Define the CSV file path and open it in write mode:
csv_file = 'output.csv' csv_obj = open(csv_file, 'w')
4. Create a CSV writer object and write the header row:
csv_writer = csv.writer(csv_obj) header = json_data[0].keys() csv_writer.writerow(header)
5. Iterate over the JSON data and write each row to the CSV file:
for item in json_data: csv_writer.writerow(item.values())
6. Close the CSV file:
csv_obj.close()
The resulting CSV file will contain the converted data from the JSON file or string.
Related Article: How To Read JSON From a File In Python
Method 2: Using the pandas library
The pandas library in Python provides useful data manipulation capabilities, including the ability to convert JSON data to CSV format. Here is how you can do it:
1. Install the pandas library if you haven’t already:
pip install pandas
2. Import the required libraries:
import pandas as pd
3. Load the JSON data from a file or a string:
data = ''' [ { "name": "John", "age": 30, "city": "New York" }, { "name": "Jane", "age": 25, "city": "San Francisco" } ] ''' json_data = json.loads(data)
4. Create a pandas DataFrame from the JSON data:
df = pd.DataFrame(json_data)
5. Define the CSV file path and save the DataFrame as a CSV file:
csv_file = 'output.csv' df.to_csv(csv_file, index=False)
The resulting CSV file will contain the converted data from the JSON file or string.
Alternative Ideas
Apart from the methods mentioned above, there are other libraries and techniques available for converting JSON to CSV in Python. Some popular alternatives include:
– Using the json2csv library: The json2csv library provides a command-line interface for converting JSON data to CSV format. It offers various options and configurations to customize the conversion process. You can install it using the following command:
pip install json2csv
Once installed, you can use the json2csv
command to convert JSON data to CSV.
– Writing a custom conversion function: If you have complex JSON data or specific requirements, you can write a custom function to convert the JSON data to CSV format. This approach gives you full control over the conversion process and allows you to handle edge cases or perform additional data transformations.
Best Practices
When converting JSON to CSV in Python, consider the following best practices:
– Validate the JSON data: Before converting the JSON data to CSV, ensure that it is valid JSON by using libraries like jsonschema
or by validating against a JSON schema.
– Handle missing or inconsistent data: JSON data may have missing or inconsistent fields. Handle these cases gracefully to avoid errors during the conversion process.
– Define a consistent structure: If your JSON data has a variable structure, consider flattening it or defining a consistent structure before converting it to CSV. This will ensure that the resulting CSV file has a consistent format.
– Use appropriate data types: When converting JSON data to CSV, ensure that the data types are preserved. For example, numeric values should be converted to numbers in the CSV file, and date/time values should be converted to the appropriate format.
– Handle large datasets: If you are working with large JSON datasets, consider using libraries like ijson
or jsonlines
to handle the data incrementally and avoid memory issues.
Related Article: How to Read Xlsx File Using Pandas Library in Python