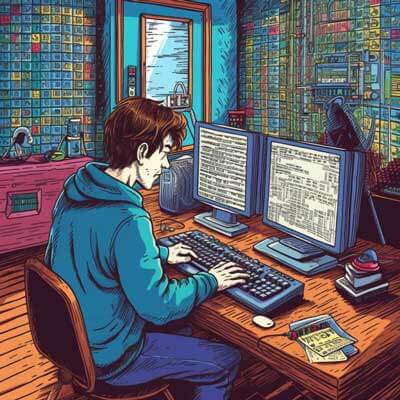
There are several ways to empty an array in Javascript. In this article, we will explore different methods and provide examples for each approach.
Method 1: Setting the length property to 0
One simple way to empty an array in Javascript is by setting the length property of the array to 0. This method works by truncating the array at index 0, effectively removing all elements.
Here’s an example:
let myArray = [1, 2, 3, 4, 5]; myArray.length = 0; console.log(myArray); // Output: []
In the example above, we first create an array called myArray
with some elements. Then, we set the length property to 0, which removes all elements from the array. Finally, we log the contents of the array to the console and verify that it is now empty.
Related Article: How To Check If a Key Exists In a Javascript Object
Method 2: Reassigning an empty array
Another way to empty an array is by reassigning it to a new empty array. This method creates a new array and assigns it to the variable, effectively replacing the old array with a new empty one.
Here’s an example:
let myArray = [1, 2, 3, 4, 5]; myArray = []; console.log(myArray); // Output: []
In the example above, we assign an empty array []
to the variable myArray
, replacing the original array with a new empty one. The console log verifies that the array is now empty.
Why would you want to empty an array?
There are several reasons why you might want to empty an array in Javascript. Some common use cases include:
– Resetting the state: If you are working on an application that uses arrays to store data or maintain state, you may need to reset the array to its initial state or clear it before populating it with new data.
– Memory management: In some cases, emptying an array can help free up memory resources, especially if the array contains a large number of elements. By removing the elements from the array, you can ensure that the memory used by those elements is released.
Alternative ideas and suggestions
While the methods described above are commonly used to empty an array in Javascript, there are also alternative approaches you can consider:
– Using the splice()
method: The splice()
method can be used to remove elements from an array by specifying the starting index and the number of elements to remove. To empty an array using splice()
, you can pass 0
as the starting index and the length of the array as the number of elements to remove. Here’s an example:
let myArray = [1, 2, 3, 4, 5]; myArray.splice(0, myArray.length); console.log(myArray); // Output: []
– Using the pop()
method in a loop: If you want to remove all elements from an array one by one, you can use a loop in combination with the pop()
method. The pop()
method removes the last element from an array and returns it. By repeatedly calling pop()
until the array is empty, you can effectively empty the array. Here’s an example:
let myArray = [1, 2, 3, 4, 5]; while (myArray.length > 0) { myArray.pop(); } console.log(myArray); // Output: []
Related Article: How To Use the Javascript Void(0) Operator
Best practices
When emptying an array in Javascript, it is important to keep a few best practices in mind:
– Be cautious of references: If there are other variables or references pointing to the same array, emptying the array using any of the methods described above will also affect those references. Make sure to consider the impact on other parts of your code.
– Consider performance implications: The performance of different methods may vary depending on the size of the array. For small arrays, the performance difference is negligible. However, for large arrays, methods like splice()
or pop()
in a loop may be less efficient compared to setting the length property to 0 or reassigning an empty array.
– Choose the method that suits your needs: Different methods have different implications. Consider factors like readability, maintainability, and the specific requirements of your application when choosing the method to empty an array.