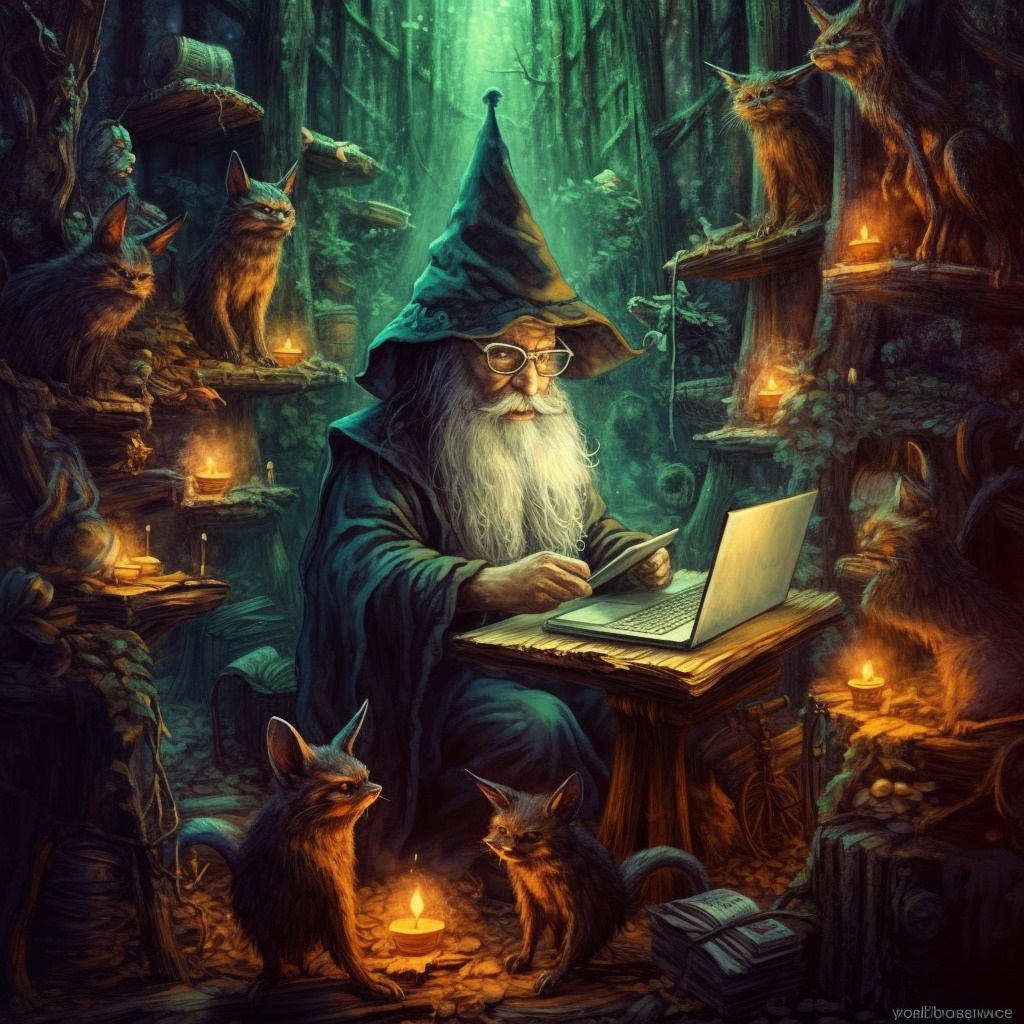
- Basics and Intro: Creating and Manipulating HashMap
- How HashMap Works Under the Hood in JavaScript
- Code Example: Simple HashMap Implementation in JavaScript
- Practical Use Cases for HashMap in JavaScript
- Code Example: Using HashMap for Data Aggregation
- Advanced Techniques: Performance Optimizations Using HashMap
- 1. Load Factor Optimization
- 2. Using Proper Hashing Functions
- 3. Resizing and Rehashing
- 4. Using a TypedArray
- 5. Avoiding Excessive Resizing
- Code Example: High-Performance Data Management with HashMap
- Real-World Applications of JavaScript HashMap
- Code Example: Implementing a Cache System Using HashMap
- Pros and Cons of Using HashMap in JavaScript
- Pros of Using HashMap in JavaScript
- Cons of Using HashMap in JavaScript
- Troubleshooting Common Issues with JavaScript HashMap
- 1. Retrieving a value that does not exist
- 2. Overwriting an existing key-value pair
- 3. Iterating over HashMap entries
- 4. Removing a key-value pair
- 5. Performance considerations
- HashMap Versus Other Data Structures in JavaScript
- Code Example: Comparing HashMap with Arrays and Objects
- Future Trends: The Evolution of HashMap in JavaScript
- ES6 Map and Set
- Typed Arrays
- Immutable HashMaps
- Hash Collision Resolution
Basics and Intro: Creating and Manipulating HashMap
A HashMap is a data structure in JavaScript that allows you to store key-value pairs. It provides fast access to values based on their keys and is commonly used to optimize lookup operations. In this chapter, we will explore the basics of creating and manipulating HashMaps in JavaScript.
To create a HashMap in JavaScript, you can use the built-in Map
object. Here’s an example of how to create a new HashMap:
const hashMap = new Map();
Once you have created a HashMap, you can start adding key-value pairs to it. The set()
method is used to add a new key-value pair to the HashMap. Here’s an example:
hashMap.set('name', 'John'); hashMap.set('age', 30);
In the above example, we added two key-value pairs to the HashMap. The keys are strings (name
and age
) and the corresponding values are 'John'
and 30
.
To retrieve a value from the HashMap, you can use the get()
method and pass in the key. Here’s an example:
const name = hashMap.get('name'); console.log(name); // Output: John
In this example, we retrieved the value associated with the key 'name'
and stored it in the variable name
. We then logged the value to the console, which outputs 'John'
.
You can also check if a key exists in the HashMap using the has()
method. Here’s an example:
const hasAge = hashMap.has('age'); console.log(hasAge); // Output: true
In this example, we checked if the key 'age'
exists in the HashMap. The has()
method returns a boolean value (true
or false
), indicating whether the key exists or not.
To remove a key-value pair from the HashMap, you can use the delete()
method. Here’s an example:
hashMap.delete('age');
In this example, we removed the key-value pair associated with the key 'age'
from the HashMap.
You can also get the number of key-value pairs in the HashMap using the size
property. Here’s an example:
const size = hashMap.size; console.log(size); // Output: 1
In this example, we retrieved the number of key-value pairs in the HashMap and stored it in the variable size
. We then logged the value to the console, which outputs 1
.
In this chapter, we learned the basics of creating and manipulating HashMaps in JavaScript using the Map
object. We explored how to add key-value pairs, retrieve values, check if a key exists, remove key-value pairs, and get the size of the HashMap. In the next chapter, we will dive deeper into advanced operations and techniques for working with HashMaps in JavaScript.
Related Article: How To Merge Arrays And Remove Duplicates In Javascript
How HashMap Works Under the Hood in JavaScript
HashMap is an important data structure in JavaScript that allows you to store and retrieve key-value pairs efficiently. It is commonly used to solve problems where you need to quickly find or update values based on a given key. In this section, we will take a closer look at how HashMap works under the hood in JavaScript.
At its core, a HashMap is implemented using an array of fixed size, where each element in the array is called a bucket. Each bucket can store multiple key-value pairs. When you insert a new key-value pair into the HashMap, it calculates the hash code of the key and maps it to a specific bucket using a hash function.
The hash function takes the key and converts it into a numerical value. This numerical value is then used as an index to map the key-value pair to the corresponding bucket in the array. The goal of a good hash function is to distribute the key-value pairs evenly across the buckets, minimizing collisions.
Collisions occur when two or more keys map to the same bucket. To handle collisions, most HashMap implementations use a technique called chaining. Chaining involves storing multiple key-value pairs in the same bucket using a linked list or an array. When you need to retrieve a value based on a key, the HashMap first calculates the hash code of the key, determines the bucket, and then traverses the linked list or array in that bucket to find the matching key-value pair.
Let’s take a look at a simple implementation of a HashMap in JavaScript:
class HashMap { constructor() { this.buckets = new Array(100); // Fixed size array } _hash(key) { let hash = 0; for (let i = 0; i < key.length; i++) { hash += key.charCodeAt(i); } return hash % this.buckets.length; } set(key, value) { const index = this._hash(key); if (!this.buckets[index]) { this.buckets[index] = []; } this.buckets[index].push({ key, value }); } get(key) { const index = this._hash(key); if (this.buckets[index]) { for (let i = 0; i < this.buckets[index].length; i++) { if (this.buckets[index][i].key === key) { return this.buckets[index][i].value; } } } return undefined; } }
In the above code snippet, we define a HashMap class with a fixed-size array as the buckets. The _hash
method calculates the hash code of the key, and the set
method inserts a key-value pair into the appropriate bucket. The get
method retrieves the value based on the given key.
It’s important to note that the performance of a HashMap depends on the quality of the hash function and the number of collisions. A good hash function should distribute the key-value pairs evenly across the buckets to minimize collisions. If there are too many collisions, the performance of the HashMap may degrade.
Code Example: Simple HashMap Implementation in JavaScript
In this code example, we will demonstrate how to create a simple HashMap implementation in JavaScript. A HashMap is a data structure that allows you to store key-value pairs, where each key is unique. It provides fast access to values based on their keys.
To implement a HashMap, we can use an array to store the key-value pairs. The keys will be hashed to determine their index in the array. In case of collisions, where multiple keys hash to the same index, we can use a technique called chaining, where each index stores a linked list of key-value pairs.
Let’s start by creating a HashMap class with basic methods for adding, getting, and removing key-value pairs:
class HashMap { constructor() { this._buckets = []; } _hash(key) { let hash = 0; for (let i = 0; i < key.length; i++) { hash = (hash + key.charCodeAt(i)) % this._buckets.length; } return hash; } set(key, value) { const index = this._hash(key); if (!this._buckets[index]) { this._buckets[index] = []; } const bucket = this._buckets[index]; for (let i = 0; i < bucket.length; i++) { if (bucket[i][0] === key) { bucket[i][1] = value; return; } } bucket.push([key, value]); } get(key) { const index = this._hash(key); const bucket = this._buckets[index]; if (!bucket) { return undefined; } for (let i = 0; i < bucket.length; i++) { if (bucket[i][0] === key) { return bucket[i][1]; } } return undefined; } remove(key) { const index = this._hash(key); const bucket = this._buckets[index]; if (!bucket) { return; } for (let i = 0; i < bucket.length; i++) { if (bucket[i][0] === key) { bucket.splice(i, 1); return; } } } }
In the above code, we define the HashMap
class with a constructor that initializes an empty array called _buckets
. The _hash
method calculates the hash value for a given key using a simple algorithm that sums the character codes of the key's characters.
The set
method takes a key and a value, calculates the hash for the key, and checks if a bucket exists at that index. If not, it creates a new bucket. It then iterates through the bucket to check if the key is already present. If found, it updates the value. Otherwise, it adds a new key-value pair to the bucket.
The get
method takes a key, calculates the hash, and retrieves the corresponding bucket. It then iterates through the bucket to find the key and returns its value if found.
The remove
method takes a key, calculates the hash, and retrieves the corresponding bucket. It then iterates through the bucket to find the key and removes the key-value pair if found.
Here's an example of how to use the HashMap
class:
const map = new HashMap(); map.set('name', 'John'); map.set('age', 30); map.set('city', 'New York'); console.log(map.get('name')); // Output: John console.log(map.get('age')); // Output: 30 console.log(map.get('city')); // Output: New York map.remove('age'); console.log(map.get('age')); // Output: undefined
In the above example, we create a new instance of the HashMap
class and use the set
method to add key-value pairs. We then retrieve the values using the get
method and remove a key-value pair using the remove
method.
This simple HashMap implementation in JavaScript can be a useful tool for storing and retrieving data efficiently based on unique keys. Feel free to explore and extend it to suit your needs.
You can find the complete code example on GitHub.
Practical Use Cases for HashMap in JavaScript
HashMaps are powerful data structures that provide efficient and fast key-value pair lookups. In JavaScript, the built-in Map object can be used as a HashMap. In this chapter, we will explore some practical use cases for HashMaps in JavaScript.
2. Counting Occurrences
HashMaps are also useful for counting the occurrences of elements in an array or string. By using elements as keys and their counts as values, you can easily keep track of the frequency of each element.
// Example of counting occurrences using a HashMap function countOccurrences(arr) { const counts = new Map(); for (const element of arr) { counts.set(element, counts.get(element) + 1 || 1); } return counts; } const arr = [1, 2, 3, 2, 1, 3, 1, 4, 2]; const occurrences = countOccurrences(arr); console.log(occurrences);
In the example above, the countOccurrences
function takes an array as input and uses a HashMap to count the occurrences of each element. The result is a HashMap where each key represents an element and the corresponding value represents its count.
3. Removing Duplicates
HashMaps can be used to remove duplicate elements from an array or string efficiently. By using a HashMap to keep track of the unique elements, you can filter out duplicates and obtain a unique list of elements.
// Example of removing duplicates using a HashMap function removeDuplicates(arr) { const uniqueElements = new Map(); for (const element of arr) { uniqueElements.set(element, true); } return Array.from(uniqueElements.keys()); } const arr = [1, 2, 3, 2, 1, 3, 1, 4, 2]; const uniqueArr = removeDuplicates(arr); console.log(uniqueArr);
In the example above, the removeDuplicates
function takes an array as input and uses a HashMap to keep track of unique elements. The result is an array containing only the unique elements from the input array.
4. Efficient Lookup
HashMaps provide efficient lookup for key-value pairs. They are especially useful when you need to perform frequent lookups based on specific keys.
// Example of efficient lookup using a HashMap const studentGrades = new Map([ ['John', 90], ['Alice', 95], ['Bob', 87] ]); console.log(studentGrades.get('Alice')); // Output: 95 console.log(studentGrades.get('Bob')); // Output: 87
In the example above, the studentGrades
HashMap stores the grades of different students. By using the get
method, you can efficiently retrieve the grade for a specific student by their name.
HashMaps have a wide range of applications in JavaScript, from caching and counting occurrences to removing duplicates and efficient lookups. Understanding and utilizing HashMaps can greatly enhance the performance and functionality of your JavaScript applications.
Related Article: How To Remove Duplicates From A Javascript Array
Code Example: Using HashMap for Data Aggregation
In this section, we will explore a code example that demonstrates how to use a HashMap for data aggregation in JavaScript. The HashMap data structure allows us to store key-value pairs and efficiently retrieve the values based on their corresponding keys.
Let’s say we have an array of objects representing sales data, where each object contains the name of a product and the corresponding sales amount. Our goal is to aggregate the sales data by product and calculate the total sales for each product.
First, let’s define our array of sales data:
const salesData = [ { product: 'Product A', sales: 100 }, { product: 'Product B', sales: 200 }, { product: 'Product A', sales: 150 }, { product: 'Product C', sales: 300 }, { product: 'Product B', sales: 250 }, ];
To aggregate the sales data, we can use a HashMap where the keys represent the product names and the values represent the total sales for each product. We will iterate over the sales data array, check if the product exists in the HashMap, and update the total sales accordingly.
Here’s the code to accomplish this:
const salesMap = new Map(); salesData.forEach(({ product, sales }) => { if (salesMap.has(product)) { salesMap.set(product, salesMap.get(product) + sales); } else { salesMap.set(product, sales); } }); console.log(salesMap);
Running this code will output the following:
Map(3) { 'Product A' => 250, 'Product B' => 450, 'Product C' => 300 }
As you can see, the HashMap now contains the aggregated sales data for each product. The keys represent the product names, and the values represent the total sales.
Using a HashMap for data aggregation can be very efficient, especially when dealing with large datasets. It allows for constant-time lookup and update operations.
In this example, we used the built-in Map
class in JavaScript to implement the HashMap functionality. However, there are also other libraries available, such as the hashmap package, that provide additional features and optimizations for working with HashMaps in JavaScript.
This technique can be applied to various scenarios where data needs to be grouped and summarized based on certain criteria.
Advanced Techniques: Performance Optimizations Using HashMap
In this chapter, we will explore advanced techniques to optimize the performance of HashMap in JavaScript. By implementing these techniques, you can significantly improve the efficiency and speed of your code.
1. Load Factor Optimization
The load factor is a measure of how full the HashMap is allowed to get before its capacity is automatically increased. By default, the load factor is set to 0.75. However, you can adjust this value based on your specific use case to achieve better performance.
A lower load factor means the HashMap will be resized more frequently, resulting in more space being allocated. This can reduce collisions and improve overall performance. On the other hand, a higher load factor means the HashMap will be resized less frequently, which can save memory but may lead to more collisions and slower performance.
To optimize the load factor, you can use the loadFactor
property when creating a HashMap object:
const hashMap = new HashMap({ loadFactor: 0.5 });
Related Article: How To Sum An Array Of Numbers In Javascript
2. Using Proper Hashing Functions
The performance of a HashMap heavily relies on the quality of the hashing function used to calculate the index of each key-value pair. A good hashing function should distribute the keys evenly across the HashMap’s internal array, minimizing collisions.
JavaScript provides a built-in hashing function called hashCode()
, which can be used for primitive values such as numbers and strings. However, for more complex objects, you may need to implement a custom hashing function.
Here’s an example of implementing a custom hashing function for an object:
function customHashCode(obj) { let hash = 0; const str = JSON.stringify(obj); for (let i = 0; i < str.length; i++) { hash = (hash << 5) - hash + str.charCodeAt(i); hash |= 0; // Convert to 32-bit integer } return hash; } const hashMap = new HashMap({ hashFn: customHashCode });
3. Resizing and Rehashing
As the number of key-value pairs stored in a HashMap increases, the internal array used to store the data may become too small. To maintain optimal performance, the HashMap automatically resizes and rehashes its internal array when necessary.
Resizing involves creating a new array with a larger capacity and rehashing involves recalculating the indices of all key-value pairs based on the new array size. These operations can be computationally expensive, especially when dealing with a large number of elements.
One way to optimize resizing and rehashing is to estimate the maximum number of key-value pairs your HashMap will hold and initialize it with an appropriate initial capacity. By doing so, you can minimize the number of resize operations, thus improving performance.
Here’s an example of initializing a HashMap with an initial capacity of 100:
const hashMap = new HashMap({ initialCapacity: 100 });
4. Using a TypedArray
Another optimization technique is to use a TypedArray, such as Int32Array
or Float64Array
, to store the key-value pairs. TypedArrays provide a more memory-efficient way of storing and accessing numeric data.
By using a TypedArray, you can potentially reduce memory usage and improve the performance of HashMap operations. However, keep in mind that TypedArrays only work with numeric keys, so this optimization technique may not be suitable for all use cases.
Here’s an example of using an Int32Array
as the internal storage for a HashMap:
const hashMap = new HashMap({ storage: Int32Array });
Related Article: How To Check If an Array Contains a Value In JavaScript
5. Avoiding Excessive Resizing
Resizing the HashMap too frequently can have a negative impact on performance. To avoid excessive resizing, you can calculate the optimal initial capacity based on the maximum number of key-value pairs you expect to store.
By setting the initial capacity to a value closer to the expected number of key-value pairs, you can reduce the number of resize operations and improve overall performance.
Here’s an example of calculating the optimal initial capacity based on the expected number of elements:
const expectedSize = 1000; const optimalCapacity = Math.ceil(expectedSize / 0.75); const hashMap = new HashMap({ initialCapacity: optimalCapacity });
By implementing these advanced techniques, you can optimize the performance of your HashMap in JavaScript and achieve better efficiency and speed in your code.
Code Example: High-Performance Data Management with HashMap
In this chapter, we will provide a code example that demonstrates how to use a HashMap in JavaScript for high-performance data management. A HashMap is a data structure that allows for efficient storage and retrieval of key-value pairs.
To start, let’s create a new HashMap object using the built-in Map class in JavaScript:
const hashMap = new Map();
Now, let’s add some key-value pairs to the HashMap:
hashMap.set('name', 'John Doe'); hashMap.set('age', 30); hashMap.set('city', 'New York');
We can retrieve values from the HashMap using the get
method:
const name = hashMap.get('name'); console.log(name); // Output: John Doe
We can also check if a key exists in the HashMap using the has
method:
const hasAge = hashMap.has('age'); console.log(hasAge); // Output: true
To update the value of a key in the HashMap, we can simply use the set
method again:
hashMap.set('age', 31); console.log(hashMap.get('age')); // Output: 31
If we want to remove a key-value pair from the HashMap, we can use the delete
method:
hashMap.delete('city'); console.log(hashMap.has('city')); // Output: false
The HashMap also provides methods to get the number of key-value pairs (size
) and to clear all the entries (clear
):
console.log(hashMap.size); // Output: 2 hashMap.clear(); console.log(hashMap.size); // Output: 0
One of the advantages of using a HashMap is its ability to perform operations in constant time, regardless of the size of the HashMap. This makes it a great choice for high-performance data management.
This code example demonstrates how to use a HashMap in JavaScript for efficient storage and retrieval of key-value pairs. The HashMap provides methods for adding, retrieving, updating, and deleting entries, making it a powerful tool for high-performance data management.
Real-World Applications of JavaScript HashMap
JavaScript HashMaps are powerful data structures that can be used in a variety of real-world applications. In this chapter, we will explore some common use cases where HashMaps can be leveraged to solve complex problems efficiently.
1. Caching
HashMaps can be used effectively for caching frequently accessed data in an application. By using a HashMap as a cache, you can store the results of expensive operations and retrieve them quickly when needed. This can significantly improve the performance of your application.
Here’s an example of how a HashMap can be used for caching:
// Create a HashMap for caching const cache = new Map(); function expensiveOperation(input) { if (cache.has(input)) { return cache.get(input); } // Perform the expensive operation const result = performExpensiveOperation(input); // Store the result in the cache cache.set(input, result); return result; }
2. Counting and Frequency Analysis
HashMaps are also useful for counting occurrences of elements and performing frequency analysis. You can use a HashMap to keep track of the count of each element in a collection, which can be helpful in various scenarios such as finding the most frequent item or detecting anomalies in data.
Here’s an example of how a HashMap can be used for counting elements:
// Count the occurrences of each element in an array function countElements(arr) { const countMap = new Map(); arr.forEach((element) => { if (countMap.has(element)) { countMap.set(element, countMap.get(element) + 1); } else { countMap.set(element, 1); } }); return countMap; }
3. Implementing a Dictionary
HashMaps can be used to implement a dictionary, where you can store key-value pairs and efficiently retrieve the corresponding values. This can be useful in scenarios where you need to associate additional information with certain keys.
Here’s an example of how a HashMap can be used as a dictionary:
// Create a dictionary using a HashMap const dictionary = new Map(); // Add entries to the dictionary dictionary.set("apple", "A fruit"); dictionary.set("car", "A vehicle"); dictionary.set("tree", "A plant"); // Retrieve values from the dictionary console.log(dictionary.get("apple")); // Output: A fruit console.log(dictionary.get("tree")); // Output: A plant
4. Graph Algorithms
HashMaps are commonly used in graph algorithms for efficient storage and retrieval of graph elements. By using a HashMap to represent the adjacency list of a graph, you can easily access the neighbors of a particular node and perform graph traversal algorithms like breadth-first search or depth-first search.
Here’s an example of how a HashMap can be used in a graph algorithm:
// Create a graph using a HashMap for adjacency list const graph = new Map(); // Add nodes to the graph graph.set("A", ["B", "C"]); graph.set("B", ["C", "D"]); graph.set("C", ["D"]); graph.set("D", []); // Get the neighbors of a node console.log(graph.get("A")); // Output: ["B", "C"] console.log(graph.get("B")); // Output: ["C", "D"]
Related Article: How to Use the JavaScript Filter Array Method
Code Example: Implementing a Cache System Using HashMap
In this code example, we will demonstrate how to implement a cache system using a HashMap in JavaScript. A cache system is used to store and retrieve data efficiently, reducing the need for expensive calculations or database queries.
First, let’s create a new JavaScript file called cache.js
.
// cache.js class Cache { constructor() { this.cacheMap = new Map(); } get(key) { return this.cacheMap.get(key); } set(key, value) { this.cacheMap.set(key, value); } delete(key) { this.cacheMap.delete(key); } clear() { this.cacheMap.clear(); } size() { return this.cacheMap.size; } has(key) { return this.cacheMap.has(key); } } module.exports = Cache;
In the code above, we define a Cache
class that uses a HashMap (Map
in JavaScript) to store key-value pairs. The get
method retrieves the value associated with a given key, the set
method adds or updates a key-value pair, and the delete
method removes a key-value pair from the cache. The clear
method clears all entries in the cache, and the size
method returns the number of entries. The has
method checks if a key exists in the cache.
Now, let’s use the Cache
class in a sample application. Create a new JavaScript file called app.js
.
// app.js const Cache = require('./cache'); // Create a new cache instance const cache = new Cache(); // Add some data to the cache cache.set('key1', 'value1'); cache.set('key2', 'value2'); cache.set('key3', 'value3'); // Retrieve data from the cache console.log(cache.get('key1')); // Output: value1 console.log(cache.get('key2')); // Output: value2 console.log(cache.get('key3')); // Output: value3 // Check if a key exists in the cache console.log(cache.has('key1')); // Output: true console.log(cache.has('key4')); // Output: false // Remove a key from the cache cache.delete('key2'); // Clear the entire cache cache.clear(); // Check the size of the cache console.log(cache.size()); // Output: 0
In the code above, we import the Cache
class from the cache.js
file. We create a new instance of the Cache
class and add some data to the cache using the set
method. We retrieve data from the cache using the get
method and check if a key exists in the cache using the has
method. We delete a key from the cache using the delete
method, clear the entire cache using the clear
method, and check the size of the cache using the size
method.
By implementing a cache system using a HashMap, we can store and retrieve data efficiently, improving the performance of our applications.
Pros and Cons of Using HashMap in JavaScript
JavaScript HashMaps provide a powerful way to store and retrieve data using key-value pairs. They offer several advantages that make them a popular choice for many developers. However, like any data structure, HashMaps also have their downsides. In this chapter, we will explore the pros and cons of using HashMaps in JavaScript.
Pros of Using HashMap in JavaScript
1. Efficient Data Retrieval: HashMaps use a hashing function to store and retrieve values based on their keys. This makes data retrieval exceptionally fast, even for large datasets. The average time complexity for searching an element in a HashMap is O(1), which means it is highly efficient.
2. Flexible Key Types: HashMaps allow you to use a wide range of data types as keys, including strings, numbers, objects, and even functions. This flexibility enables you to create more complex data structures and retrieve values based on various criteria.
3. No Duplicate Keys: HashMaps do not allow duplicate keys. If you try to add a value with an existing key, it will replace the existing value. This feature ensures that each key is unique, preventing any confusion or data integrity issues.
4. Dynamic Size: Unlike arrays, HashMaps do not have a fixed size. They can dynamically expand or shrink based on the number of elements they hold. This makes HashMaps suitable for scenarios where the number of elements may vary over time.
5. Fast Insertion and Deletion: Adding or removing an element from a HashMap is generally faster than other data structures like arrays or linked lists. The average time complexity for insertion and deletion is also O(1), making HashMaps efficient for managing data that frequently changes.
Related Article: How To Loop Through An Array In Javascript
Cons of Using HashMap in JavaScript
1. Ordering of Elements: HashMaps do not guarantee any specific order for their elements. If you need to maintain a specific order, you may need to consider using other data structures like arrays or linked lists.
2. Memory Overhead: HashMaps consume more memory compared to simpler data structures like arrays. Each key-value entry in a HashMap requires additional memory for storing the key, value, and internal data structures. If memory usage is a concern, you should carefully consider whether a HashMap is the right choice for your use case.
3. Hash Collisions: Hash functions used by HashMaps may occasionally produce the same hash value for different keys. This phenomenon is called a hash collision. To handle collisions, HashMaps use various techniques like chaining or open addressing. While these techniques are efficient, they can slightly impact the performance of data retrieval.
4. Iteration Performance: Iterating over a HashMap can be slower compared to other data structures. The order of iteration is not guaranteed, and HashMaps do not provide direct methods for iterating over keys, values, or entries. You may need to convert the HashMap to an array or use the ES6 Map
data structure if you require a specific order or need convenient iteration methods.
Despite these limitations, HashMaps remain a powerful tool for managing data in JavaScript. Understanding the pros and cons of using HashMaps will help you make informed decisions about when and how to use them in your projects.
Here’s an example of creating and using a HashMap in JavaScript:
// Creating a HashMap const hashMap = new Map(); // Adding values hashMap.set('name', 'John'); hashMap.set('age', 30); hashMap.set('city', 'New York'); // Retrieving values console.log(hashMap.get('name')); // Output: John console.log(hashMap.get('age')); // Output: 30 console.log(hashMap.get('city')); // Output: New York // Removing a value hashMap.delete('age'); // Checking if a key exists console.log(hashMap.has('age')); // Output: false // Clearing the HashMap hashMap.clear();
Troubleshooting Common Issues with JavaScript HashMap
JavaScript HashMaps are powerful data structures that allow you to store key-value pairs efficiently. However, like any other programming concept, you may encounter some issues when working with HashMaps. In this section, we will discuss some common issues that developers often face and provide solutions to troubleshoot them effectively.
1. Retrieving a value that does not exist
One common issue is trying to retrieve a value using a key that does not exist in the HashMap. In such cases, the get()
method will return undefined
. To avoid this issue, you should always check if the key exists in the HashMap before retrieving its value. Here’s an example:
const hashMap = new Map(); hashMap.set('key1', 'value1'); hashMap.set('key2', 'value2'); const key = 'key3'; if (hashMap.has(key)) { const value = hashMap.get(key); console.log(value); } else { console.log(`Key '${key}' does not exist.`); }
Related Article: How To Convert Array To Object In Javascript
2. Overwriting an existing key-value pair
Another common issue is accidentally overwriting an existing key-value pair in the HashMap. If you use the set()
method with the same key, it will update the existing value instead of creating a new entry. To avoid this issue, you can use the has()
method to check if the key already exists before updating the value. Here’s an example:
const hashMap = new Map(); hashMap.set('key1', 'value1'); hashMap.set('key2', 'value2'); const key = 'key2'; if (hashMap.has(key)) { console.log(`Key '${key}' already exists. Updating value...`); hashMap.set(key, 'updatedValue'); } else { hashMap.set(key, 'newValue'); }
3. Iterating over HashMap entries
When iterating over a HashMap, the order of the entries is not guaranteed to be the same as they were added. This can sometimes lead to unexpected behavior, especially if you rely on the order of the entries. To overcome this issue, you can use the forEach()
method to iterate over the entries in the HashMap. Here’s an example:
const hashMap = new Map(); hashMap.set('key1', 'value1'); hashMap.set('key2', 'value2'); hashMap.forEach((value, key) => { console.log(`Key: ${key}, Value: ${value}`); });
4. Removing a key-value pair
To remove a key-value pair from a HashMap, you can use the delete()
method. However, it’s important to note that this method returns a boolean value indicating whether the key existed and was successfully removed or not. Here’s an example:
const hashMap = new Map(); hashMap.set('key1', 'value1'); hashMap.set('key2', 'value2'); const key = 'key1'; const isDeleted = hashMap.delete(key); if (isDeleted) { console.log(`Key '${key}' was successfully deleted.`); } else { console.log(`Key '${key}' does not exist.`); }
Related Article: How To Add A Class To A Given Element In Javascript
5. Performance considerations
While HashMaps provide efficient key-value pair lookups, it’s important to consider the performance implications when working with large datasets. As the size of the HashMap grows, the time complexity for operations like insertion and retrieval may increase. If you anticipate working with a large number of entries, you might consider alternative data structures or optimization techniques to improve performance.
Now that you are familiar with common issues that can arise when working with JavaScript HashMaps, you can troubleshoot them effectively. Remember to always validate the existence of keys, avoid accidental overwrites, handle iteration order, delete key-value pairs with care, and consider performance implications for large datasets.
HashMap Versus Other Data Structures in JavaScript
In JavaScript, there are several data structures available for storing and manipulating collections of data. Each data structure has its own advantages and disadvantages, and choosing the right one for your specific use case is crucial for optimal performance.
One commonly used data structure in JavaScript is the array. Arrays are ordered collections of elements and can be accessed using numerical indices. They are very efficient for storing and retrieving elements in a specific order, but their performance degrades when you need to search for a specific element or insert/delete elements in the middle of the array.
Let’s consider an example where we have an array of objects representing employees:
const employees = [ { id: 1, name: 'John' }, { id: 2, name: 'Jane' }, { id: 3, name: 'Alice' } ];
If we want to find an employee with a specific ID, we would need to iterate over the entire array until we find a match:
function findEmployeeById(id) { for (let i = 0; i < employees.length; i++) { if (employees[i].id === id) { return employees[i]; } } return null; } const employee = findEmployeeById(2); console.log(employee); // { id: 2, name: 'Jane' }
This approach has a time complexity of O(n), where n is the number of elements in the array. If the array is large, this can result in slow performance.
A more efficient data structure for searching would be a HashMap, also known as a dictionary or associative array. A HashMap is an unordered collection of key-value pairs, where each key is unique. It allows for constant time complexity O(1) for both insertion and retrieval operations.
Let’s rewrite the previous example using a HashMap:
const employeesMap = new Map(); employeesMap.set(1, { id: 1, name: 'John' }); employeesMap.set(2, { id: 2, name: 'Jane' }); employeesMap.set(3, { id: 3, name: 'Alice' }); const employee = employeesMap.get(2); console.log(employee); // { id: 2, name: 'Jane' }
In this example, we create a new Map and use the employee ID as the key and the employee object as the value. We can then retrieve the employee object directly by using the get() method with the corresponding key.
HashMaps are particularly useful when you need to associate data with specific keys and perform fast lookups. They are also efficient for adding and removing elements dynamically, as the size of the HashMap grows or shrinks.
However, there are some trade-offs to consider. HashMaps consume more memory compared to arrays because they need to store both the keys and the values. Additionally, they do not preserve the order of insertion.
When deciding between a HashMap and other data structures in JavaScript, consider the specific requirements of your use case. If you need fast lookups and dynamic element manipulation, a HashMap can be a great choice. However, if you require a specific order or have memory constraints, an array or other data structure might be more suitable.
Code Example: Comparing HashMap with Arrays and Objects
In this code example, we will compare the usage of a HashMap with arrays and objects in JavaScript. We will demonstrate how a HashMap can provide a more efficient and convenient way to store and retrieve data compared to traditional arrays and objects.
Let’s start by creating a simple HashMap using the Map
object in JavaScript:
// Create a new HashMap const hashMap = new Map(); // Add key-value pairs to the HashMap hashMap.set('key1', 'value1'); hashMap.set('key2', 'value2'); hashMap.set('key3', 'value3'); // Retrieve values from the HashMap console.log(hashMap.get('key1')); // Output: value1 console.log(hashMap.get('key2')); // Output: value2 console.log(hashMap.get('key3')); // Output: value3
In the above code snippet, we create a new HashMap using the Map
constructor. We then use the set
method to add key-value pairs to the HashMap. Finally, we use the get
method to retrieve the values associated with the keys.
Now, let’s compare this with how we would achieve the same functionality using arrays and objects:
// Using arrays const array = [ { key: 'key1', value: 'value1' }, { key: 'key2', value: 'value2' }, { key: 'key3', value: 'value3' } ]; // Retrieve values from the array const getValueFromArray = (key) => { const item = array.find((element) => element.key === key); return item ? item.value : undefined; }; console.log(getValueFromArray('key1')); // Output: value1 console.log(getValueFromArray('key2')); // Output: value2 console.log(getValueFromArray('key3')); // Output: value3 // Using objects const object = { key1: 'value1', key2: 'value2', key3: 'value3' }; // Retrieve values from the object const getValueFromObject = (key) => object[key]; console.log(getValueFromObject('key1')); // Output: value1 console.log(getValueFromObject('key2')); // Output: value2 console.log(getValueFromObject('key3')); // Output: value3
In the above code snippets, we demonstrate how to achieve the same functionality using arrays and objects. However, with arrays, we need to use the find
method to search for the key-value pairs, which has a time complexity of O(n). On the other hand, with objects, we can directly access the values using the key, which has a time complexity of O(1).
The HashMap provides a more efficient way to store and retrieve data compared to arrays and objects, especially when dealing with a large amount of data or frequently accessing specific values.
Related Article: How To Append To A Javascript Array
Future Trends: The Evolution of HashMap in JavaScript
HashMap is a powerful data structure in JavaScript that provides efficient key-value pair storage and retrieval. With the evolution of JavaScript and the ever-growing needs of developers, the HashMap implementation has also evolved over time. In this chapter, we will explore some future trends and advancements in the HashMap implementation in JavaScript.
ES6 Map and Set
ES6 introduced the Map and Set objects, which are alternatives to the traditional HashMap implementation in JavaScript. The Map object allows you to store key-value pairs similar to a HashMap, while the Set object lets you store unique values of any type.
Unlike the traditional HashMap, the Map object preserves the order of insertion of keys, making it useful in scenarios where the order of elements is important. The Map object also provides built-in methods for iteration, size retrieval, and key-value manipulation.
Here’s an example of using the Map object in JavaScript:
const map = new Map(); map.set('key1', 'value1'); map.set('key2', 'value2'); console.log(map.get('key1')); // Output: value1 console.log(map.size); // Output: 2
Typed Arrays
With the introduction of Typed Arrays in JavaScript, we have more efficient ways to store and manipulate data. Typed Arrays allow us to work with raw binary data in a structured manner, providing better performance and memory efficiency compared to traditional JavaScript arrays.
In the context of HashMaps, Typed Arrays can be used to store large amounts of data more efficiently. They allow us to define the type and size of each element, which is particularly useful when dealing with numeric or binary data.
Here’s an example of using a Typed Array for storing numeric values in JavaScript:
const array = new Int32Array(4); array[0] = 10; array[1] = 20; array[2] = 30; array[3] = 40; console.log(array); // Output: Int32Array(4) [10, 20, 30, 40]
Related Article: How to Extract Values from Arrays in JavaScript
Immutable HashMaps
Immutable data structures have gained popularity in JavaScript due to their advantages in functional programming and state management. An immutable HashMap is a data structure where the values cannot be modified once set, providing better predictability and avoiding unintended side effects.
Immutable HashMaps can be useful in scenarios where you need to ensure that the data remains unchanged, such as caching or memoization. Libraries like Immutable.js provide efficient implementations of immutable data structures, including HashMaps.
Here’s an example of using Immutable.js to create an immutable HashMap in JavaScript:
const { Map } = require('immutable'); const map1 = Map({ key1: 'value1', key2: 'value2' }); const map2 = map1.set('key3', 'value3'); console.log(map1.get('key1')); // Output: value1 console.log(map2.get('key3')); // Output: value3
Hash Collision Resolution
Hash collisions occur when different keys produce the same hash value, potentially leading to performance degradation in HashMaps. Various collision resolution techniques have been developed to handle such scenarios.
One popular technique is open addressing, where collisions are resolved by finding an alternative empty slot in the HashMap. Another technique is chaining, where collisions are resolved by storing multiple elements with the same hash value in linked lists or other data structures.
As JavaScript evolves, we can expect further advancements and optimizations in hash collision resolution techniques, improving the performance and efficiency of HashMaps.