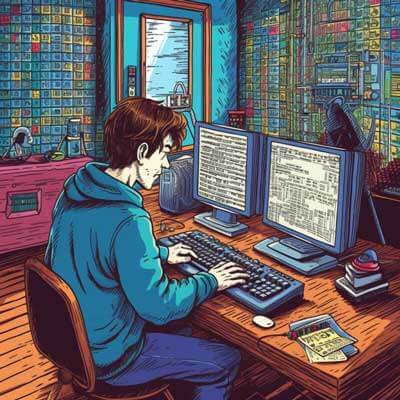
Table of Contents
To reverse a string in-place in JavaScript, you can follow these steps:
Step 1: Convert the string into an array
The first step is to convert the string into an array of characters. This can be done using the split()
method with an empty string as the separator. Here's an example:
let str = "Hello, World!"; let arr = str.split('');
Related Article: How To Add Days To a Date In Javascript
Step 2: Reverse the array
Once you have the array of characters, you can reverse it using the reverse()
method. This method modifies the original array in-place, reversing the order of its elements. Here's how you can do it:
arr.reverse();
Step 3: Convert the array back into a string
After reversing the array, you can convert it back into a string using the join()
method. This method joins all elements of an array into a string, with an optional separator between them. In this case, we'll use an empty string as the separator to join the characters together. Here's the code:
let reversedStr = arr.join('');
Alternative Approach
Another approach to reversing a string in-place is by using two pointers, one starting from the beginning of the string and the other starting from the end. You can swap the characters at the two pointers and move the pointers towards each other until they meet in the middle. Here's an example implementation:
function reverseStringInPlace(str) { let arr = str.split(''); let start = 0; let end = arr.length - 1; while (start < end) { // Swap characters let temp = arr[start]; arr[start] = arr[end]; arr[end] = temp; // Move pointers start++; end--; } return arr.join(''); } let reversedStr = reverseStringInPlace("Hello, World!");
This approach avoids creating a new array and directly modifies the original string in-place.
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
Best Practices
When reversing a string in-place in JavaScript, it's important to consider the following best practices:
1. Use the reverse()
method: The reverse()
method is the most straightforward way to reverse an array in JavaScript. It's a built-in method that efficiently handles the reversal process.
2. Convert string to an array: Converting the string into an array of characters allows you to take advantage of array manipulation methods like reverse()
and join()
.
3. Avoid unnecessary memory usage: Reversing a string in-place helps to minimize memory usage and improve performance. Instead of creating a new string or array, modify the existing data structure directly.
4. Consider edge cases: When implementing the reverse algorithm, consider edge cases such as empty strings or strings with a single character. Handle these cases appropriately to ensure your code works correctly in all scenarios.
Overall, the process of reversing a string in-place in JavaScript is straightforward. By converting the string to an array, reversing the array, and converting it back to a string, you can achieve the desired result efficiently.