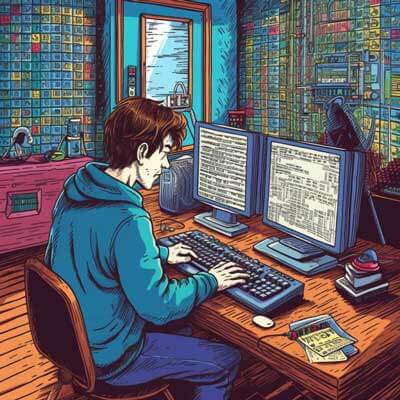
- Custom Elements
- Shadow DOM
- HTML Templates
- Encapsulation in Web Components: Keeping Styles and Behavior Isolated
- Reusability in Web Components: Building Modular and Flexible UI Components
- Interoperability of Web Components with Other JavaScript Frameworks
- Component-based Development: Building Complex Applications with Web Components
- An In-Depth Look at Polymer and Its Relationship to Web Components
- Understanding Lit and Its Connection to Web Components
- Stencil: Exploring Its Role in Web Component Development
- How Web Components Work in JavaScript
- Benefits of Using Web Components in JavaScript
- Reusability
- Encapsulation
- Modularity
- Interoperability
- Performance
- Standardization
- Creating Custom Elements in JavaScript
- The Purpose of the Shadow DOM in Web Components
- HTML Templates and Their Usage in Web Components
- Encapsulating Styles in Web Components
- Compatibility of Web Components with Other JavaScript Frameworks
- The Difference Between Polymer and Web Components
Web Components have emerged as a useful tool in modern JavaScript development, allowing developers to create reusable and encapsulated UI components. They provide a way to build modular and flexible components that can be used across different projects and frameworks. Web Components consist of three main technologies: Custom Elements, Shadow DOM, and HTML Templates.
Custom Elements
Custom Elements allow developers to define their own HTML tags and extend existing elements to create new components. This enables the creation of self-contained and reusable components that can be used just like any other HTML element. Custom Elements can have their own properties and methods, allowing developers to define the behavior and functionality of the component.
To define a custom element, we can use the customElements.define()
method. Let’s take a look at an example:
.my-component { color: red; } <div class="my-component"> Hello, World! </div> class MyComponent extends HTMLElement { constructor() { super(); const template = document.getElementById('my-component-template'); const shadowRoot = this.attachShadow({ mode: 'open' }); shadowRoot.appendChild(template.content.cloneNode(true)); } } customElements.define('my-component', MyComponent);
In this example, we define a custom element called . The
constructor()
method is called when the element is created and attached to the DOM. We use the attachShadow()
method to create a shadow DOM for the component and attach the provided template to it. The template contains the HTML and CSS for the component.
Once the custom element is defined, we can use it in our HTML like this:
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Shadow DOM
The Shadow DOM is a key feature of Web Components that allows for encapsulation of styles and DOM structure within a component. It provides a way to create a scoped DOM tree that is separate from the main document DOM. This ensures that the styles and structure of the component do not interfere with the rest of the page, and vice versa.
To create a shadow DOM for a custom element, we use the attachShadow()
method. Let’s see an example:
.my-component { color: red; } <div class="my-component"> Hello, World! </div> class MyComponent extends HTMLElement { constructor() { super(); const template = document.getElementById('my-component-template'); const shadowRoot = this.attachShadow({ mode: 'open' }); shadowRoot.appendChild(template.content.cloneNode(true)); } } customElements.define('my-component', MyComponent);
In this example, the attachShadow({ mode: 'open' })
method is used to create an open shadow DOM. The open
mode allows the component’s styles to be visible to the outside world. If we use closed
mode instead, the styles will be completely hidden from the outside.
HTML Templates
HTML Templates provide a way to define reusable chunks of HTML that can be cloned and inserted into the DOM when needed. They are particularly useful when combined with Web Components, as they allow for the creation of complex component structures that can be easily reused and shared.
To define an HTML template, we use the element. Let’s take a look at an example:
.my-component { color: red; } <div class="my-component"> Hello, World! </div> class MyComponent extends HTMLElement { constructor() { super(); const template = document.getElementById('my-component-template'); const shadowRoot = this.attachShadow({ mode: 'open' }); shadowRoot.appendChild(template.content.cloneNode(true)); } } customElements.define('my-component', MyComponent);
In this example, we define an HTML template with the id my-component-template
. The template contains the HTML structure and styles for our component. We then use the content
property of the template to clone its contents and append them to the shadow DOM of our custom element.
Using HTML templates allows us to define complex component structures that can be easily reused and shared across different parts of our application.
Encapsulation in Web Components: Keeping Styles and Behavior Isolated
One of the key benefits of Web Components is the ability to encapsulate styles and behavior within a component. This ensures that the styles and functionality of a component do not interfere with other parts of the application, and vice versa.
Encapsulation is achieved through the use of the Shadow DOM, which provides a scoped DOM tree for each component. This means that the styles defined within a component are only applied to the elements within its shadow DOM, and not to the rest of the page. Similarly, the behavior and functionality defined within a component are isolated and do not affect other parts of the application.
Let’s take a look at an example to see encapsulation in action:
.my-component { color: red; } <div class="my-component"> Hello, World! </div> class MyComponent extends HTMLElement { constructor() { super(); const template = document.getElementById('my-component-template'); const shadowRoot = this.attachShadow({ mode: 'open' }); shadowRoot.appendChild(template.content.cloneNode(true)); } } customElements.define('my-component', MyComponent);
In this example, we define a custom element called . The styles defined within the component, such as
.my-component
with color: red
, are only applied to the elements within its shadow DOM. This ensures that the styles do not affect other parts of the page.
Similarly, the behavior and functionality defined within the component, such as event listeners and methods, are isolated to the component and do not interfere with other parts of the application.
Encapsulation in Web Components is a useful feature that helps in creating modular and reusable UI components, as well as maintaining code cleanliness and reducing the risk of style and behavior conflicts.
Related Article: How to Use the forEach Loop with JavaScript
Reusability in Web Components: Building Modular and Flexible UI Components
Web Components provide a way to build modular and flexible UI components that can be easily reused across different projects and frameworks. This reusability is achieved through the use of Custom Elements, which allow developers to define their own HTML tags and extend existing elements to create new components.
Let’s take a look at an example to see reusability in action:
.my-component { color: red; } <div class="my-component"> Hello, World! </div> class MyComponent extends HTMLElement { constructor() { super(); const template = document.getElementById('my-component-template'); const shadowRoot = this.attachShadow({ mode: 'open' }); shadowRoot.appendChild(template.content.cloneNode(true)); } static get observedAttributes() { return ['name']; } connectedCallback() { this.render(); } attributeChangedCallback(name, oldValue, newValue) { if (name === 'name') { this.render(); } } render() { const name = this.getAttribute('name') || 'World'; this.shadowRoot.querySelector('.my-component').textContent = `Hello, ${name}!`; } } customElements.define('my-component', MyComponent);
In this example, we define a custom element called . The component has a default template and styles defined within its shadow DOM. It also has a property called
name
, which can be customized by setting the name
attribute on the element.
The component uses the connectedCallback()
method to render its content when it is connected to the DOM. It also uses the attributeChangedCallback()
method to re-render the content whenever the name
attribute changes.
This example demonstrates how Web Components allow for the creation of modular and flexible UI components. The element can be easily reused in different parts of the application by simply adding it to the HTML markup.
Interoperability of Web Components with Other JavaScript Frameworks
Web Components are designed to be interoperable with other JavaScript frameworks, allowing developers to use them alongside existing codebases and frameworks. This interoperability is achieved through the use of standard web technologies such as HTML, CSS, and JavaScript.
Since Web Components are based on standard web technologies, they can be used in any framework or project that supports these technologies. This means that developers can use Web Components in frameworks like React, Angular, and Vue.js, as well as in plain JavaScript projects.
Let’s take a look at an example to see how Web Components can be used alongside other frameworks:
<!-- index.html --> <title>My App</title> const MyComponent = Vue.defineComponent({ template: ` <div> <h1>{{ message }}</h1> <button>Change Message</button> </div> `, data() { return { message: 'Hello, World!' } }, methods: { changeMessage() { this.message = 'Hello, Vue!' } } }) Vue.createApp({ components: { MyComponent } }).mount('my-component')
In this example, we have an index.html file that includes both a Web Component called and a Vue.js component called
MyComponent
. The Web Component is defined using the custom element syntax, and the Vue.js component is defined using the Vue.defineComponent() method.
The Web Component and the Vue.js component can coexist in the same HTML file and interact with each other seamlessly. The Vue.js component can access the Web Component and vice versa. This demonstrates the interoperability of Web Components with other JavaScript frameworks.
Web Components provide a way to create reusable and encapsulated UI components that can be used in any JavaScript framework. This makes it easier for developers to leverage existing codebases and frameworks while benefiting from the advantages of Web Components.
Component-based Development: Building Complex Applications with Web Components
Component-based development is a popular approach to building complex applications, and Web Components provide a useful foundation for this approach. With Web Components, developers can create reusable and encapsulated UI components that can be easily composed to build complex applications.
Component-based development involves breaking down the user interface into smaller, self-contained components, each responsible for a specific piece of functionality. These components can then be combined and composed to build larger components and ultimately the entire application.
Let’s take a look at an example to see component-based development in action:
.my-button { padding: 10px 20px; background-color: blue; color: white; border: none; border-radius: 5px; cursor: pointer; } <button class="my-button"> </button> class MyButton extends HTMLElement { constructor() { super(); const template = document.getElementById('my-button-template'); const shadowRoot = this.attachShadow({ mode: 'open' }); shadowRoot.appendChild(template.content.cloneNode(true)); } } customElements.define('my-button', MyButton); Click me!
In this example, we define a custom element called . The component encapsulates the styling and functionality of a button. It uses the Shadow DOM to ensure that the styles do not interfere with the rest of the page.
We can then use the element to create buttons throughout our application. By composing and combining these buttons with other components, we can build complex user interfaces.
Component-based development with Web Components allows for better organization, reusability, and maintainability of code. It promotes a modular and flexible architecture, making it easier to develop and maintain complex applications.
Related Article: How to Use Javascript Substring, Splice, and Slice
An In-Depth Look at Polymer and Its Relationship to Web Components
Polymer is a JavaScript library that provides a set of features and tools for building Web Components. It simplifies the process of creating and using Web Components by providing a higher-level API and a set of utility functions.
Polymer is built on top of the core Web Components technologies, such as Custom Elements, Shadow DOM, and HTML Templates. It extends these technologies by adding features like data binding, declarative event handling, and a component lifecycle.
Let’s take a look at an example to see how Polymer simplifies the process of creating and using Web Components:
.my-component { color: red; } <div class="my-component"> Hello, [[name]]! </div> import { PolymerElement, html } from 'https://unpkg.com/@polymer/polymer@3.0.0/lib/legacy/polymer-fn.js'; class MyComponent extends PolymerElement { static get template() { return html` .my-component { color: red; } <div class="my-component"> Hello, [[name]]! </div> `; } static get properties() { return { name: { type: String, value: 'World' } }; } } customElements.define('my-component', MyComponent);
In this example, we define a custom element called using Polymer. We use the
PolymerElement
base class provided by Polymer to define our component.
Polymer simplifies the process of creating Web Components by providing a higher-level API and a set of utility functions. For example, instead of using the attachShadow()
method to create a shadow DOM, we can use the template
property to define the template for our component.
Polymer also provides features like data binding and a component lifecycle, which make it easier to work with Web Components. In this example, we define a name
property with a default value of ‘World’. We then use double square brackets [[name]]
to bind the value of the property to the content of the component.
Polymer is a useful tool for building Web Components, providing a higher-level API and a set of utility functions that simplify the process of creating and using Web Components.
Understanding Lit and Its Connection to Web Components
Lit is a lightweight JavaScript library for building Web Components. It provides a simple and efficient way to create reusable and encapsulated UI components using standard web technologies.
Lit is built on top of the core Web Components technologies, such as Custom Elements, Shadow DOM, and HTML Templates. It extends these technologies by adding features like reactive rendering, declarative templates, and efficient updates.
Let’s take a look at an example to see how Lit simplifies the process of creating and using Web Components:
.my-component { color: red; } <div class="my-component"> Hello, {{name}}! </div> import { html, css, LitElement } from 'https://unpkg.com/lit@2.0.0/lit.js'; class MyComponent extends LitElement { static styles = css` .my-component { color: red; } `; static properties = { name: { type: String } }; render() { return html` <div class="my-component"> Hello, ${this.name}! </div> `; } } customElements.define('my-component', MyComponent);
In this example, we define a custom element called using Lit. We use the
LitElement
base class provided by Lit to define our component.
Lit simplifies the process of creating Web Components by providing a simple and efficient API. Instead of using the attachShadow()
method to create a shadow DOM, we can use the styles
property to define the styles for our component. We can use the properties
property to define the properties of our component.
Lit also provides features like reactive rendering and declarative templates, which make it easier to work with Web Components. In the render()
method, we use the html
template tag to define the template for our component. We can use JavaScript expressions within the template using the ${}
syntax.
Lit is a lightweight and efficient library for building Web Components, providing a simple and efficient way to create reusable and encapsulated UI components using standard web technologies.
Stencil: Exploring Its Role in Web Component Development
Stencil is a toolchain for building Web Components that combines the simplicity of JSX with the performance of pure JavaScript. It provides a way to write components using a syntax similar to JSX and compiles them into highly optimized Web Components.
Stencil is designed to be efficient and scalable, making it suitable for building complex applications. It focuses on performance and optimization, allowing developers to create fast and responsive Web Components.
Let’s take a look at an example to see how Stencil simplifies the process of building Web Components:
import { Component, h } from '@stencil/core'; @Component({ tag: 'my-component', styleUrl: 'my-component.css', shadow: true, }) export class MyComponent { render() { return ( <div class="my-component"> Hello, World! </div> ); } }
In this example, we define a Stencil component called . Stencil components are written using a syntax similar to JSX, allowing for easy and familiar development. We use the
h
function provided by Stencil to define the template for our component.
Stencil provides a useful toolchain for building Web Components. It includes features like automatic code splitting, lazy loading, and prerendering, which improve performance and user experience. It also supports TypeScript out of the box, making it easier to write type-safe and maintainable code.
Stencil is a useful tool for building Web Components, providing a simple and efficient way to write highly optimized components using a syntax similar to JSX.
Related Article: JavaScript Arrays: Learn Array Slice, Array Reduce, and String to Array Conversion
How Web Components Work in JavaScript
Web Components are a set of web platform APIs and standards that allow developers to create reusable and encapsulated UI components using standard web technologies such as HTML, CSS, and JavaScript.
Web Components consist of three main technologies: Custom Elements, Shadow DOM, and HTML Templates.
Custom Elements allow developers to define their own HTML tags and extend existing elements to create new components. This enables the creation of self-contained and reusable components that can be used just like any other HTML element.
Shadow DOM provides a way to create a scoped DOM tree that is separate from the main document DOM. This ensures that the styles and structure of the component do not interfere with the rest of the page, and vice versa.
HTML Templates provide a way to define reusable chunks of HTML that can be cloned and inserted into the DOM when needed. They are particularly useful when combined with Web Components, as they allow for the creation of complex component structures that can be easily reused and shared.
Web Components work by leveraging these technologies to create reusable and encapsulated UI components. Developers can define their own custom elements using the Custom Elements API, encapsulate styles and structure using the Shadow DOM, and define complex component structures using HTML Templates.
Once a Web Component is defined, it can be used in HTML markup just like any other HTML element. The component’s behavior and functionality can be customized using properties and methods defined within the component.
Web Components are supported by all major browsers, making them a useful and cross-platform solution for building UI components.
Benefits of Using Web Components in JavaScript
Using Web Components in JavaScript offers several benefits that can greatly improve the development process and the quality of web applications. Here are some of the key benefits:
Reusability
Web Components enable the creation of reusable UI components that can be easily shared and used across different projects and frameworks. Components can be defined once and used in multiple places, reducing code duplication and improving maintainability.
Related Article: JavaScript HashMap: A Complete Guide
Encapsulation
Web Components provide a way to encapsulate the styles and behavior of a component, ensuring that they do not interfere with other parts of the application. Styles defined within a component’s Shadow DOM are scoped to that component, preventing conflicts with other styles on the page.
Modularity
Web Components promote a modular architecture, allowing developers to break down the user interface into smaller, self-contained components. This makes it easier to understand, test, and maintain the codebase, leading to better overall code quality.
Interoperability
Web Components are designed to be interoperable with other JavaScript frameworks and libraries. They can be used alongside existing codebases and frameworks, allowing developers to leverage their existing knowledge and infrastructure.
Related Article: Conditional Flow in JavaScript: Understand the 'if else' and 'else if' Syntax and More
Performance
Web Components can offer improved performance compared to traditional JavaScript frameworks, as they are based on native browser technologies. They can be optimized for rendering and take advantage of the browser’s rendering engine, resulting in faster and more efficient UI components.
Standardization
Web Components are based on standard web technologies such as HTML, CSS, and JavaScript. They are supported by all major browsers and follow a set of standards and specifications, ensuring compatibility and future-proofing.
Creating Custom Elements in JavaScript
Creating custom elements in JavaScript allows developers to define their own HTML tags and extend existing elements to create new components. This enables the creation of self-contained and reusable components that can be used just like any other HTML element.
To create a custom element, we can use the customElements.define()
method. Let’s take a look at an example:
class MyComponent extends HTMLElement { constructor() { super(); } connectedCallback() { this.innerHTML = 'Hello, World!'; } } customElements.define('my-component', MyComponent);
In this example, we define a custom element called . The
constructor()
method is called when the element is created and attached to the DOM. We use the connectedCallback()
method to set the content of the element to ‘Hello, World!’.
Once the custom element is defined, we can use it in our HTML like this:
This will create an instance of the custom element and render the content defined in the connectedCallback()
method.
Creating custom elements in JavaScript allows for the creation of self-contained and reusable components that can be easily used in HTML markup.
Related Article: JavaScript Arrow Functions Explained (with examples)
The Purpose of the Shadow DOM in Web Components
The Shadow DOM is a key feature of Web Components that allows for encapsulation of styles and DOM structure within a component. It provides a way to create a scoped DOM tree that is separate from the main document DOM. This ensures that the styles and structure of the component do not interfere with the rest of the page, and vice versa.
The main purpose of the Shadow DOM is to encapsulate the styles and structure of a component, making it self-contained and isolated from the rest of the page. This prevents styles from leaking out of the component and affecting other parts of the page, and also prevents styles from the page from affecting the component.
The Shadow DOM also provides a way to create a separate DOM tree for a component, allowing for easy manipulation and rendering of the component’s content. This makes it easier to manage the state and behavior of the component, as well as improve performance by limiting the scope of rendering and updates.
To create a shadow DOM for a custom element, we use the attachShadow()
method. Let’s see an example:
class MyComponent extends HTMLElement { constructor() { super(); const shadowRoot = this.attachShadow({ mode: 'open' }); shadowRoot.innerHTML = '.my-component { color: red; }<div class="my-component">Hello, World!</div>'; } } customElements.define('my-component', MyComponent);
In this example, the attachShadow({ mode: 'open' })
method is used to create an open shadow DOM. The open
mode allows the component’s styles to be visible to the outside world. If we use closed
mode instead, the styles will be completely hidden from the outside.
The Shadow DOM is a useful feature of Web Components that enables encapsulation, isolation, and improved performance. It plays a crucial role in creating self-contained and reusable UI components.
HTML Templates and Their Usage in Web Components
HTML Templates provide a way to define reusable chunks of HTML that can be cloned and inserted into the DOM when needed. They are particularly useful when combined with Web Components, as they allow for the creation of complex component structures that can be easily reused and shared.
To define an HTML template, we use the element. Let’s take a look at an example:
.my-component { color: red; } <div class="my-component"> Hello, World! </div> class MyComponent extends HTMLElement { constructor() { super(); const template = document.getElementById('my-component-template'); const shadowRoot = this.attachShadow({ mode: 'open' }); shadowRoot.appendChild(template.content.cloneNode(true)); } } customElements.define('my-component', MyComponent);
In this example, we define an HTML template with the id my-component-template
. The template contains the HTML structure and styles for our component. We then use the content
property of the template to clone its contents and append them to the shadow DOM of our custom element.
Using HTML templates allows us to define complex component structures that can be easily reused and shared across different parts of our application.
Encapsulating Styles in Web Components
Encapsulating styles in Web Components is a key feature that ensures the styles of a component do not interfere with other parts of the application, and vice versa. This is achieved through the use of the Shadow DOM, which provides a scoped DOM tree for each component.
To encapsulate styles within a component, we define the styles within the component’s Shadow DOM. This ensures that the styles are only applied to the elements within the component and do not affect the rest of the page.
Let’s take a look at an example to see how styles can be encapsulated in Web Components:
.my-component { color: red; } <div class="my-component"> Hello, World! </div> class MyComponent extends HTMLElement { constructor() { super(); const template = document.getElementById('my-component-template'); const shadowRoot = this.attachShadow({ mode: 'open' }); shadowRoot.appendChild(template.content.cloneNode(true)); } } customElements.define('my-component', MyComponent);
In this example, the styles defined within the tags are encapsulated within the component’s Shadow DOM. The
.my-component
class is only applied to the elements within the component and does not affect other parts of the page.
Encapsulating styles in Web Components ensures that the styles of a component are isolated and do not interfere with other parts of the application. This promotes code cleanliness, reduces the risk of style conflicts, and improves the maintainability of the codebase.
Related Article: JavaScript Modules & How to Reuse Code in JavaScript
Compatibility of Web Components with Other JavaScript Frameworks
Web Components are designed to be compatible with other JavaScript frameworks, allowing developers to use them alongside existing codebases and frameworks. This compatibility is achieved through the use of standard web technologies and the adherence to web standards.
Web Components are based on the core web technologies such as HTML, CSS, and JavaScript, which are supported by all major browsers and frameworks. This means that Web Components can be used in any framework or project that supports these technologies.
Let’s take a look at an example to see how Web Components can be used alongside other JavaScript frameworks:
<!-- index.html --> <title>My App</title> const MyComponent = () => { return <div>Hello, World!</div>; }; ReactDOM.render(, document.querySelector('my-component'));
In this example, we have an index.html file that includes both a Web Component called and a React component called
MyComponent
. The Web Component is defined using the custom element syntax, and the React component is defined using JSX.
The Web Component and the React component can coexist in the same HTML file and interact with each other seamlessly. The React component can access the Web Component and vice versa. This demonstrates the compatibility of Web Components with other JavaScript frameworks.
Web Components provide a way to create reusable and encapsulated UI components that can be used in any JavaScript framework. This makes it easier for developers to leverage existing codebases and frameworks while benefiting from the advantages of Web Components.
The Difference Between Polymer and Web Components
Polymer and Web Components are closely related but distinct concepts in JavaScript development. While Web Components are a set of web platform APIs and standards that allow developers to create reusable and encapsulated UI components, Polymer is a JavaScript library that provides a set of features and tools for building Web Components.
Web Components are based on standard web technologies such as HTML, CSS, and JavaScript. They consist of three main technologies: Custom Elements, Shadow DOM, and HTML Templates. Web Components can be used in any JavaScript framework or project that supports these technologies.
Polymer, on the other hand, is built on top of the core Web Components technologies. It provides a higher-level API and a set of utility functions that simplify the process of creating and using Web Components. Polymer extends the capabilities of Web Components by adding features like data binding, declarative event handling, and a component lifecycle.
Let’s take a look at an example to see the difference between Polymer and Web Components:
.my-component { color: red; } <div class="my-component"> Hello, [[name]]! </div> import { PolymerElement, html } from 'https://unpkg.com/@polymer/polymer@3.0.0/lib/legacy/polymer-fn.js'; class MyComponent extends PolymerElement { static get template() { return html` .my-component { color: red; } <div class="my-component"> Hello, [[name]]! </div> `; } static get properties() { return { name: { type: String, value: 'World' } }; } } customElements.define('my-component', MyComponent);
In this example, we define a custom element called using both Polymer and Web Components. The component is defined using the PolymerElement base class provided by Polymer. We use the
template
and properties
static properties to define the template and properties of the component, respectively.
Polymer simplifies the process of creating and using Web Components by providing a higher-level API and a set of utility functions. It extends the capabilities of Web Components by adding features like data binding and a component lifecycle.
Web Components, on the other hand, are the underlying technologies and standards that enable the creation of reusable and encapsulated UI components. They can be used independently or with the help of libraries like Polymer.