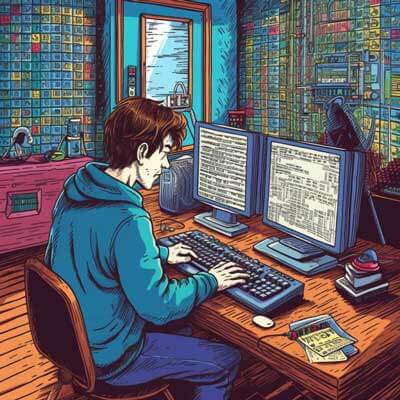
Defining a function with optional arguments allows you to create flexible and versatile functions that can be called with different sets of inputs. In Python, you can define optional arguments by providing default values for parameters in the function definition. This means that if an argument is not provided when the function is called, the default value will be used instead. Here’s how you can define a function with optional arguments in Python:
Syntax
To define a function with optional arguments, you can use the following syntax:
def function_name(parameter1, parameter2=default_value): # function body
In this syntax, parameter1
is a required argument that must be provided when calling the function, while parameter2
is an optional argument that has a default value. If parameter2
is not provided when calling the function, the default value will be used.
Related Article: How To Limit Floats To Two Decimal Points In Python
Example 1: Function with Optional Argument
Let’s say you want to define a function that greets a person by their name. You want the function to be able to handle cases where the name is not provided, in which case it should greet the person with a generic message. Here’s how you can define such a function:
def greet(name="stranger"): print("Hello, " + name + "!")
In this example, the greet()
function has an optional argument name
with a default value of “stranger”. If the name
argument is not provided when calling the function, it will default to “stranger”. Here are some examples of how you can call the greet()
function:
greet("Alice") # Output: Hello, Alice! greet() # Output: Hello, stranger!
As you can see, if the name
argument is provided, the function will use it in the greeting. If the name
argument is not provided, the function will use the default value and greet the person as “stranger”.
Example 2: Function with Multiple Optional Arguments
You can also define a function with multiple optional arguments by providing default values for each argument. Here’s an example:
def calculate_discount(price, discount_percent=0, additional_discount=0): discounted_price = price - (price * discount_percent / 100) - additional_discount return discounted_price
In this example, the calculate_discount()
function takes a required argument price
, as well as two optional arguments discount_percent
and additional_discount
with default values of 0. The function calculates the discounted price based on the provided arguments and returns it. Here are some examples of how you can call the calculate_discount()
function:
price1 = calculate_discount(100) # Output: 100 (no discount applied) price2 = calculate_discount(100, 10) # Output: 90 (10% discount applied) price3 = calculate_discount(100, 10, 5) # Output: 85 (10% discount + $5 additional discount applied)
As you can see, the function can be called with different combinations of arguments. If an argument is not provided, the default value will be used.
Best Practices
When defining functions with optional arguments in Python, it’s important to follow some best practices to ensure clarity and maintainability of your code:
1. Use meaningful default values: Choose default values that make sense in the context of your function. This will help users of your function understand the behavior and intent of the optional arguments.
2. Document the function: Clearly document the function and its optional arguments, including their default values, in the function’s docstring. This will make it easier for others (including yourself) to understand and use the function correctly.
3. Avoid mutable default arguments: Be cautious when using mutable objects (such as lists or dictionaries) as default values for optional arguments. Mutable default arguments can lead to unexpected behavior due to their mutable nature. Instead, use immutable objects (such as None or a string) as default values, and handle mutable objects within the function body if needed.
4. Keep the order of arguments consistent: If you have multiple optional arguments, make sure to keep their order consistent across different versions of your function. Changing the order of arguments can break existing code that relies on the default values.
5. Use keyword arguments for clarity: When calling a function with optional arguments, consider using keyword arguments to make the code more readable and self-explanatory. This way, it’s clear which arguments are being passed and what their values are.
Related Article: How To Rename A File With Python