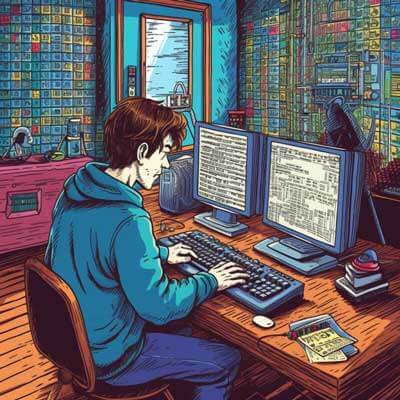
Table of Contents
In Python, exceptions are a way to handle errors or exceptional events that occur during the execution of a program. An exception is an object that represents an error or exceptional condition. By raising an exception, you can interrupt the normal flow of execution and handle the error in a controlled manner.
Why would you raise an exception?
There are several reasons why you might want to manually raise an exception in Python:
1. Error handling: You can raise an exception when you encounter an error or an exceptional condition that you want to handle in a specific way. By raising an exception, you can transfer control to an exception handler that can take appropriate actions, such as logging the error, displaying an error message to the user, or rolling back a transaction.
2. Validation: You can raise an exception to validate input or enforce certain conditions. For example, if you have a function that requires a positive integer as input, you can raise an exception if the input is not valid.
3. Custom exceptions: Python provides a set of built-in exceptions that cover common error scenarios. However, in some cases, you may need to define your own custom exception types to handle specific application-specific errors. By raising a custom exception, you can communicate the specific error condition to the calling code.
Related Article: How to Implement a Python Foreach Equivalent
How to manually raise an exception
In Python, you can manually raise an exception by using the raise
statement followed by an instance of an exception class or a built-in exception type. The general syntax for raising an exception is as follows:
raise ExceptionType("Error message")
Here, ExceptionType
can be either a built-in exception type (such as ValueError
, TypeError
, etc.) or a custom exception class that you have defined. The "Error message"
is an optional string that provides additional information about the error.
Let's look at some examples to see how to manually raise exceptions in Python:
Raising a built-in exception
# Raising a ValueError raise ValueError("Invalid value") # Raising a TypeError raise TypeError("Invalid type") # Raising a FileNotFoundError raise FileNotFoundError("File not found")
In the above examples, we are raising different built-in exceptions with custom error messages. These exceptions can be caught and handled by an appropriate exception handler.
Raising a custom exception
# Defining a custom exception class class CustomException(Exception): pass # Raising a custom exception raise CustomException("Custom error message")
In this example, we define a custom exception class called CustomException
that inherits from the base Exception
class. We can then raise an instance of this custom exception class with a custom error message.
Alternative ideas and suggestions
While manually raising exceptions is a powerful feature in Python, it should be used judiciously. Here are some alternative ideas and suggestions to consider:
1. Use built-in exceptions: Python provides a wide range of built-in exception types that cover common error scenarios. Before defining a custom exception, check if any of the existing built-in exceptions are suitable for your use case. Using built-in exceptions can make your code more readable and maintainable.
2. Use context managers: In some cases, it may be more appropriate to use context managers (with
statement) instead of manually raising exceptions. Context managers provide a convenient way to manage resources and handle exceptions in a controlled manner. They ensure that resources are properly released, even in the presence of exceptions.
3. Provide detailed error messages: When raising an exception, it is important to provide meaningful and detailed error messages. This helps in debugging and makes it easier to understand the cause of the error. Include relevant information such as variable values, file names, line numbers, etc., in the error message.
4. Handle exceptions gracefully: When raising exceptions, make sure to handle them gracefully in your code. Instead of letting the exception propagate up the call stack, consider catching the exception at an appropriate level and taking appropriate actions. This can include logging the error, displaying an error message to the user, or retrying the operation.
Best practices
When manually raising exceptions in Python, it is good practice to follow these guidelines:
1. Choose the right exception type: Use the most appropriate exception type that accurately describes the error condition. Python provides a wide range of built-in exception types that cover common error scenarios. Choosing the right exception type makes your code more readable and helps other developers understand the intent of the code.
2. Provide informative error messages: Include detailed error messages that provide enough information to understand the cause of the error. The error message should be clear, concise, and meaningful. Include relevant information such as variable values, file names, line numbers, etc., to aid in debugging.
3. Avoid catching generic exceptions: When catching exceptions, avoid using a generic except
block without specifying the type of exception to catch. Catching generic exceptions can make it difficult to diagnose and handle specific error conditions. Instead, catch specific exception types that you expect and handle them appropriately.
4. Use context managers when appropriate: In some cases, it may be more appropriate to use context managers (with
statement) instead of manually raising exceptions. Context managers provide a clean and structured way to manage resources and handle exceptions. They ensure that resources are properly released, even in the presence of exceptions.
5. Test exception handling code: As with any code, it is important to thoroughly test the exception handling code to ensure that it behaves as expected. Write test cases that cover different error scenarios and verify that the exceptions are raised and handled correctly.