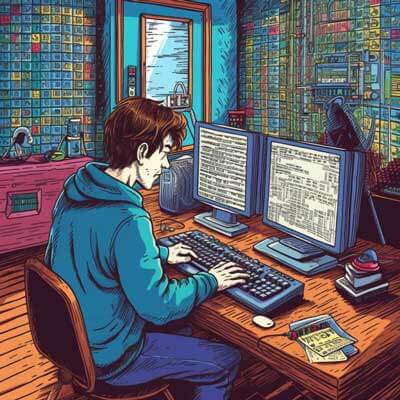
To generate random string characters in JavaScript, you can use a combination of built-in JavaScript functions and methods. There are multiple approaches you can take to accomplish this task, and I will outline two possible solutions below.
Solution 1: Using Math.random() and String.fromCharCode()
One way to generate random string characters is by utilizing the Math.random() function and the String.fromCharCode() method. The Math.random() function returns a random floating-point number between 0 and 1, and the String.fromCharCode() method converts Unicode values into characters.
Here’s an example code snippet that generates a random string of specified length:
function generateRandomString(length) { let result = ''; const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; const charactersLength = characters.length; for (let i = 0; i < length; i++) { const randomIndex = Math.floor(Math.random() * charactersLength); result += characters.charAt(randomIndex); } return result; } const randomString = generateRandomString(10); console.log(randomString);
In this example, the generateRandomString()
function takes a parameter length
which specifies the desired length of the random string. The function initializes an empty string result
and a string of allowed characters characters
. It then iterates length
number of times, generating a random index using Math.random()
and Math.floor()
to ensure the index falls within the range of charactersLength
. The character at the random index is appended to the result
string. Finally, the result
string is returned.
You can customize the characters
string to include any specific set of characters you desire.
Related Article: How to Work with Async Calls in JavaScript
Solution 2: Using Crypto.getRandomValues()
Another approach to generate random string characters is by using the Crypto.getRandomValues() function, which provides a more secure and random source of random numbers. This function is part of the Web Crypto API and is available in modern browsers.
Here’s an example code snippet that demonstrates how to use Crypto.getRandomValues() to generate a random string:
function generateRandomString(length) { const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; const charactersLength = characters.length; const randomValues = new Uint32Array(length); window.crypto.getRandomValues(randomValues); let result = ''; for (let i = 0; i < length; i++) { const randomIndex = randomValues[i] % charactersLength; result += characters.charAt(randomIndex); } return result; } const randomString = generateRandomString(10); console.log(randomString);
In this example, the generateRandomString()
function is similar to the previous solution, but instead of using Math.random()
, it uses crypto.getRandomValues()
to obtain a random set of values. The function creates a Uint32Array of length length
to store the random values. The crypto.getRandomValues()
function fills the array with cryptographically secure random values. The function then iterates over the array, calculates the random index using the modulo operator, and appends the corresponding character to the result
string. Finally, the result
string is returned.
Using crypto.getRandomValues()
provides a higher level of randomness compared to Math.random()
, making it a better choice when security is a concern.
Why generate random string characters in JavaScript?
The need to generate random string characters in JavaScript can arise in various scenarios. Some potential reasons include:
– Generating random passwords: When creating user accounts or generating temporary access tokens, it is often necessary to generate random strings as passwords or authentication tokens.
– Testing and simulations: In certain testing scenarios, generating random data, such as random string characters, can help simulate real-world scenarios and uncover potential issues or edge cases.
– Data obfuscation: Randomizing or obfuscating data by generating random string characters can be useful in certain encryption or data protection techniques.
Alternative ideas and suggestions
While the solutions provided above are effective for generating random string characters in JavaScript, there are alternative ideas and suggestions you can consider:
– Using a third-party library: If you require more advanced functionality or additional features, you may consider using a third-party library specifically designed for generating random strings. Libraries such as Chance.js (https://chancejs.com/) or Faker.js (https://fakerjsdocs.netlify.app/) provide comprehensive APIs for generating random data, including strings.
– Utilizing specific character sets: Depending on your requirements, you may need to generate random strings using specific character sets, such as only uppercase letters or numbers. You can modify the characters
string in the provided solutions to include or exclude specific characters as needed.
Related Article: Understanding JavaScript Execution Context and Hoisting
Best practices
When generating random string characters in JavaScript, it is important to keep the following best practices in mind:
– Use a cryptographically secure random number generator when security is a concern. In modern browsers, prefer using crypto.getRandomValues()
over Math.random()
.
– Avoid using predictable patterns or sources for randomization, as this can compromise the security and randomness of the generated strings.
– Consider the length and complexity of the generated strings based on your specific requirements. Longer strings with a mix of characters tend to provide a higher level of security.
– If generating random strings for passwords, consider enforcing additional password requirements, such as a minimum length or a mix of uppercase letters, lowercase letters, numbers, and special characters.