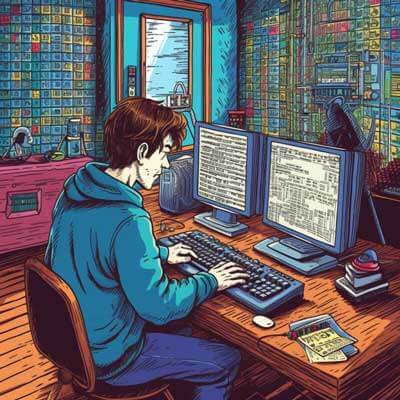
Table of Contents
Can I Autofill a Textarea in Vue.js?
Yes, it is possible to autofill a textarea in Vue.js. You can achieve this by setting the initial value of the textarea to the predefined content that you want to autofill. This can be done by assigning the desired value to the data property that is bound to the textarea using the v-model directive.
Here's an example of how to autofill a textarea in Vue.js:
<div> <textarea></textarea> </div> export default { data() { return { message: "This is the predefined content to autofill", }; }, };
In the above example, the message
data property is initialized with the predefined content that you want to autofill. This content will be displayed in the textarea when the component is rendered.
Related Article: How to Convert a Number to a String in Javascript
What is the Difference Between v-model and value in Textarea?
In Vue.js, the v-model
directive and the value
attribute are used to bind data to form input elements, including textareas. However, there is a difference between the two:
- v-model
: The v-model
directive is a two-way data binding directive in Vue.js. It allows you to bind the value of a form input element to a data property in the Vue instance. Any changes made to the form input element will update the data property, and any changes to the data property will update the form input element.
- value
: The value
attribute is a one-way binding attribute in HTML. It allows you to set the initial value of a form input element, including textareas, but it does not create a two-way data binding. Any changes made to the form input element will not update the data property, and any changes to the data property will not update the form input element.
In the context of autofilling a textarea, you would typically use v-model
to create a two-way data binding between the textarea and a data property, allowing the content of the textarea to be dynamically updated based on the data property's value.
How Can I Dynamically Update the Value of a Textarea in Vue.js?
To dynamically update the value of a textarea in Vue.js, you can modify the associated data property that is bound to the textarea using the v-model
directive. Any changes made to the data property will be automatically reflected in the textarea.
Here's an example of how to dynamically update the value of a textarea in Vue.js:
<div> <textarea></textarea> <button>Update Message</button> </div> export default { data() { return { message: "", }; }, methods: { updateMessage() { this.message = "New message"; }, }, };
In the above example, the message
data property is bound to the value of the textarea using the v-model
directive. When the "Update Message" button is clicked, the updateMessage
method is called, which sets the value of the message
property to "New message". This will dynamically update the value of the textarea.
Is it Possible to Auto-populate a Textarea with Predefined Content in Vue.js?
Yes, it is possible to auto-populate a textarea with predefined content in Vue.js. You can achieve this by setting the initial value of the textarea to the predefined content in the associated data property that is bound to the textarea using the v-model
directive.
Here's an example of how to auto-populate a textarea with predefined content in Vue.js:
<div> <textarea></textarea> <button>Auto-fill Message</button> </div> export default { data() { return { message: "", }; }, methods: { autofillMessage() { this.message = "Predefined content to auto-fill"; }, }, };
In the above example, the message
data property is bound to the value of the textarea using the v-model
directive. When the "Auto-fill Message" button is clicked, the autofillMessage
method is called, which sets the value of the message
property to the predefined content. This will auto-populate the textarea with the predefined content.
Related Article: How to Set Checked for a Checkbox with jQuery
Additional Resources
- How to Bind a Textarea Value in Vue.js
- Autofilling a Textarea in Vue.js
- Difference Between v-model and Value in Textarea in Vue.js