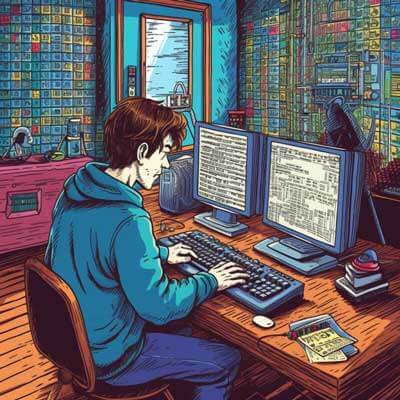
Table of Contents
In JavaScript, the forEach()
method is a convenient way to iterate over arrays. It allows you to execute a provided function once for each element in the array. This method is commonly used when you want to perform an operation on each item in an array without the need for an explicit loop.
Here's how you can use the forEach()
method to loop over an array in JavaScript:
Syntax:
array.forEach(function(currentValue, index, array) { // Your code here });
The forEach()
method takes a callback function as an argument. This callback function is executed for each element in the array and is passed three arguments:
- currentValue
: The current element being processed in the array.
- index
(optional): The index of the current element being processed in the array.
- array
(optional): The array that forEach()
is being applied to.
Related Article: Conditional Flow in JavaScript: Understand the 'if else' and 'else if' Syntax and More
Example:
Let's say we have an array of numbers and we want to print each number to the console:
const numbers = [1, 2, 3, 4, 5]; numbers.forEach(function(number) { console.log(number); });
Output:
1 2 3 4 5
In the above example, the callback function takes a single parameter number
, which represents the current element being processed. The function simply logs the number
to the console.
Best Practices:
Related Article: How to Parse a String to a Date in JavaScript
- When using the forEach()
method, it's generally recommended to use a named function instead of an anonymous function. This makes the code easier to read and allows for better reusability. Here's an example:
function printNumber(number) { console.log(number); } numbers.forEach(printNumber);
- The forEach()
method does not mutate the original array. If you want to modify the elements of an array, you can directly update them within the callback function. However, if you want to create a new array based on the original, you should consider using map()
instead.
const numbers = [1, 2, 3, 4, 5]; const squaredNumbers = []; numbers.forEach(function(number) { squaredNumbers.push(number * number); }); console.log(squaredNumbers); // [1, 4, 9, 16, 25]
- Unlike traditional for
loops, the forEach()
method cannot be stopped or breaked out of. If you need to break out of a loop early, you can use for...of
or Array.some()
instead.
const numbers = [1, 2, 3, 4, 5]; let foundNumber = false; numbers.some(function(number) { if (number === 3) { foundNumber = true; return true; // Stops the iteration } }); console.log(foundNumber); // true
- The forEach()
method is not available in older versions of Internet Explorer (prior to IE9). If you need to support older browsers, consider using a polyfill or transpiling your code to a compatible version.
Overall, the forEach()
method provides a concise and readable way to iterate over arrays in JavaScript. It's a useful tool in your JavaScript toolbox when you need to perform operations on each element of an array.