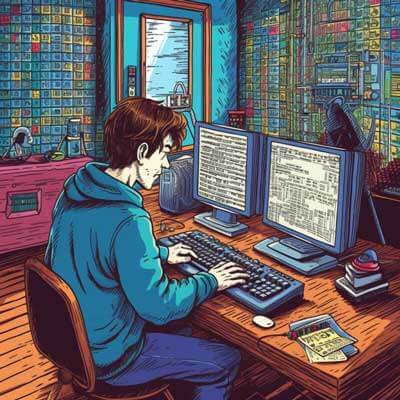
Table of Contents
Creating a Dropdown Menu in JavaScript
A dropdown menu is a common UI element that allows users to select an option from a list that is displayed when the user interacts with it. In JavaScript, we can create a dropdown menu by manipulating the DOM and handling events. Let's explore how to create a dropdown menu step by step.
To begin, we need an HTML structure to work with. Here's a simple example of a dropdown menu:
<button id="dropdownButton">Select an Option</button> <ul id="dropdownMenu" class="hidden"> <li>Option 1</li> <li>Option 2</li> <li>Option 3</li> </ul>
In this example, we have a <button>
element that serves as the dropdown button. When the user interacts with the button, a <ul>
element with multiple <li>
elements is displayed as the dropdown menu. The class hidden
is initially added to the dropdown menu to hide it.
Now, let's add some JavaScript code to handle the dropdown menu. We'll start by selecting the necessary elements using their id
:
const dropdownButton = document.getElementById('dropdownButton'); const dropdownMenu = document.getElementById('dropdownMenu');
Once we have references to the dropdown button and dropdown menu, we can add event listeners to show or hide the dropdown menu when the button is clicked:
dropdownButton.addEventListener('click', function() { dropdownMenu.classList.toggle('hidden'); });
In this example, we add a click
event listener to the dropdown button using the addEventListener()
method. When the button is clicked, the callback function is executed, and we toggle the hidden
class of the dropdown menu using the classList.toggle()
method. This means that if the dropdown menu is hidden, it will be shown, and if it is shown, it will be hidden.
To further enhance the dropdown menu, you can add additional event listeners to handle item selection or customize the appearance and behavior of the dropdown menu based on your requirements.
Related Article: How to Differentiate Between Js and Mjs Files in Javascript
Difference Between Dropdown Menu and Dropdown List in JavaScript
While the terms "dropdown menu" and "dropdown list" are often used interchangeably, there is a subtle difference in their functionality and purpose.
Dropdown Menu:
A dropdown menu is a UI element that provides a list of options that can be selected by the user. When the user interacts with the dropdown menu, a list of options is displayed, usually in a dropdown fashion, and the user can select an option by clicking on it or navigating through the options using keyboard inputs. The selected option is then displayed as the current selection in the dropdown menu. Dropdown menus are commonly used for navigation menus, form inputs, or any scenario where a selection needs to be made from a list of options.
Dropdown List:
A dropdown list, on the other hand, is a static list of options that is displayed when the user interacts with a dropdown button or a similar UI element. Unlike a dropdown menu, a dropdown list does not provide the ability to select an option directly from the list. Instead, it is used to present a predefined list of options to the user, and the user needs to take further action, such as clicking on an option or pressing a button, to make a selection. Dropdown lists are commonly used for scenarios where a user needs to view a list of options before making a selection, such as a list of available sorting options or a list of filter criteria.
Manipulating Arrays in JavaScript
Arrays are a fundamental data structure in JavaScript that allow us to store multiple values in a single variable. With arrays, we can easily manipulate and work with collections of data. Let's explore some common operations to manipulate arrays in JavaScript.
1. Adding Elements to an Array:
To add elements to an array, we can use the push()
method. Here's an example:
const fruits = ['apple', 'banana', 'orange']; fruits.push('mango'); console.log(fruits); // Output: ['apple', 'banana', 'orange', 'mango']
In this example, we add the value 'mango'
to the end of the fruits
array using the push()
method.
2. Removing Elements from an Array:
To remove elements from an array, we can use the pop()
method to remove the last element, or the splice()
method to remove elements at specific positions. Here are some examples:
const fruits = ['apple', 'banana', 'orange']; fruits.pop(); console.log(fruits); // Output: ['apple', 'banana'] const numbers = [1, 2, 3, 4, 5]; numbers.splice(2, 1); console.log(numbers); // Output: [1, 2, 4, 5]
In the first example, we use the pop()
method to remove the last element from the fruits
array. In the second example, we use the splice()
method to remove one element at index 2 from the numbers
array.
3. Accessing Elements in an Array:
To access elements in an array, we can use square brackets []
along with the index of the element. Remember that array indices start from 0. Here's an example:
const fruits = ['apple', 'banana', 'orange']; console.log(fruits[1]); // Output: 'banana'
In this example, we access the element at index 1 (which is 'banana'
) from the fruits
array.
4. Iterating over an Array:
To iterate over the elements of an array, we can use a loop, such as for
or forEach
. Here are some examples:
const fruits = ['apple', 'banana', 'orange']; // Using a for loop for (let i = 0; i < fruits.length; i++) { console.log(fruits[i]); } // Using the forEach method fruits.forEach(function(fruit) { console.log(fruit); });
In the first example, we use a for
loop to iterate over the elements of the fruits
array. In the second example, we use the forEach()
method, which takes a callback function as an argument and executes it for each element in the array.
These are just a few examples of how to manipulate arrays in JavaScript. Arrays offer many more methods and operations to work with data efficiently.
Basics of JavaScript Objects
In JavaScript, an object is a complex data type that allows us to store multiple values as properties. Objects are useful for representing real-world entities, such as a person, a car, or a book. Let's explore how to create and work with JavaScript objects.
1. Creating Objects:
There are multiple ways to create objects in JavaScript. One common way is to use object literals, which allow us to define key-value pairs within curly braces {}
. Here's an example:
const person = { name: 'John', age: 30, profession: 'Software Engineer' };
In this example, we create an object named person
with three properties: name
, age
, and profession
. The property names are specified as keys, followed by a colon :
, and the corresponding values.
2. Accessing Object Properties:
To access properties of an object, we can use dot notation or square brackets. Here's an example:
console.log(person.name); // Output: 'John' console.log(person['age']); // Output: 30
In this example, we access the name
property of the person
object using dot notation and the age
property using square brackets.
3. Modifying Object Properties:
We can modify object properties by assigning new values to them. Here's an example:
person.age = 35; person['profession'] = 'Senior Software Engineer'; console.log(person); // Output: { name: 'John', age: 35, profession: 'Senior Software Engineer' }
In this example, we update the age
and profession
properties of the person
object with new values.
4. Adding and Removing Object Properties:
To add new properties to an object, we can simply assign a value to a new property name. To remove properties, we can use the delete
keyword. Here's an example:
person.location = 'New York'; delete person.profession; console.log(person); // Output: { name: 'John', age: 35, location: 'New York' }
In this example, we add a new location
property to the person
object and then remove the profession
property.
5. Working with Object Methods:
Objects can also have methods, which are functions defined as properties. Here's an example:
const person = { name: 'John', age: 30, greet: function() { console.log('Hello, my name is ' + this.name); } }; person.greet(); // Output: 'Hello, my name is John'
In this example, we define a greet
method within the person
object, which logs a greeting message that includes the name
property.
JavaScript objects are versatile and can be used to represent various entities and their behaviors. They provide a flexible way to organize and manipulate data in your applications.
Related Article: How to Reverse a String In-Place in JavaScript
Working with JSON in JavaScript
JSON (JavaScript Object Notation) is a popular data format that is used to store and exchange data between a server and a web application. In JavaScript, we can parse JSON data into objects or stringify objects into JSON. Let's explore how to work with JSON in JavaScript.
1. Parsing JSON:
To parse JSON data into a JavaScript object, we can use the JSON.parse()
method. Here's an example:
const json = '{"name":"John","age":30,"city":"New York"}'; const obj = JSON.parse(json); console.log(obj); // Output: { name: 'John', age: 30, city: 'New York' }
In this example, we use the JSON.parse()
method to convert the json
string into a JavaScript object.
2. Stringifying Objects:
To convert a JavaScript object into a JSON string, we can use the JSON.stringify()
method. Here's an example:
const obj = { name: 'John', age: 30, city: 'New York' }; const json = JSON.stringify(obj); console.log(json); // Output: '{"name":"John","age":30,"city":"New York"}'
In this example, we use the JSON.stringify()
method to convert the obj
object into a JSON string.
3. Handling JSON Arrays:
JSON can also represent arrays. We can parse JSON arrays into JavaScript arrays and stringify JavaScript arrays into JSON arrays. Here's an example:
const json = '[1, 2, 3, 4, 5]'; const arr = JSON.parse(json); console.log(arr); // Output: [1, 2, 3, 4, 5] const numbers = [1, 2, 3, 4, 5]; const json = JSON.stringify(numbers); console.log(json); // Output: '[1,2,3,4,5]'
In the first example, we parse the json
string into a JavaScript array using JSON.parse()
. In the second example, we stringify the numbers
array into a JSON string using JSON.stringify()
.
4. Working with Nested Objects:
JSON can represent nested objects, where objects are nested within other objects. We can access nested properties using dot notation or square brackets. Here's an example:
const json = '{"person":{"name":"John","age":30},"city":"New York"}'; const obj = JSON.parse(json); console.log(obj.person.name); // Output: 'John' console.log(obj['city']); // Output: 'New York'
In this example, we parse the json
string into a JavaScript object, which contains a nested person
object and a city
property. We access the nested name
property using dot notation and the city
property using square brackets.
Working with JSON in JavaScript allows us to easily exchange data between different systems and perform operations on the data within our applications.
DOM Manipulation with JavaScript
The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the structure of a web page as a tree-like structure, where each node represents an HTML element. In JavaScript, we can manipulate the DOM to dynamically update the content and appearance of a web page. Let's explore how to manipulate the DOM with JavaScript.
1. Accessing DOM Elements:
To manipulate DOM elements, we first need to select them. We can use various methods provided by the browser's JavaScript API, such as getElementById()
, getElementsByClassName()
, or querySelector()
. Here's an example:
<div id="myDiv">Hello, World!</div>
const myDiv = document.getElementById('myDiv'); console.log(myDiv.textContent); // Output: 'Hello, World!'
In this example, we use the getElementById()
method to select the <div>
element with the id
attribute of 'myDiv'
. We then access the text content of the <div>
using the textContent
property.
2. Modifying DOM Elements:
Once we have selected a DOM element, we can modify its properties, attributes, or content. Here's an example:
<button id="myButton">Click Me</button>
const myButton = document.getElementById('myButton'); myButton.textContent = 'Click Me Now'; myButton.setAttribute('class', 'btn btn-primary');
In this example, we select the <button>
element with the id
attribute of 'myButton'
. We then update its text content using the textContent
property and add a CSS class using the setAttribute()
method.
3. Creating New DOM Elements:
We can also create new DOM elements dynamically using JavaScript. Here's an example:
const newDiv = document.createElement('div'); newDiv.textContent = 'This is a new div'; document.body.appendChild(newDiv);
In this example, we create a new <div>
element using the createElement()
method. We set its text content using the textContent
property and append it to the element using the
appendChild()
method.
4. Removing DOM Elements:
To remove a DOM element, we can use the remove()
method. Here's an example:
<div id="myDiv">Hello, World!</div>
const myDiv = document.getElementById('myDiv'); myDiv.remove();
In this example, we select the <div>
element with the id
attribute of 'myDiv'
and remove it from the DOM using the remove()
method.
DOM manipulation with JavaScript allows us to create dynamic and interactive web pages by modifying the structure and content of HTML elements.
Handling Events in JavaScript
Events are actions or occurrences that happen in the browser, such as a button click, a form submission, or a page load. In JavaScript, we can handle these events by attaching event listeners to DOM elements. Let's explore how to handle events in JavaScript.
1. Adding Event Listeners:
To handle an event, we first need to select the DOM element that triggers the event. We can then add an event listener to the element using the addEventListener()
method. Here's an example:
<button id="myButton">Click Me</button>
const myButton = document.getElementById('myButton'); myButton.addEventListener('click', function() { console.log('Button clicked'); });
In this example, we select the <button>
element with the id
attribute of 'myButton'
. We then add a click
event listener to the button using the addEventListener()
method. When the button is clicked, the associated callback function is executed, and the message 'Button clicked'
is logged to the console.
2. Event Object:
When an event is triggered, an event object is created and passed to the event listener. This object contains information about the event, such as the target element or the event type. Here's an example:
myButton.addEventListener('click', function(event) { console.log('Button clicked by:', event.target); });
In this example, we modify the callback function to accept an event
parameter. The event.target
property gives us a reference to the element that triggered the event.
3. Event Propagation:
Events in JavaScript follow a concept called event propagation, which means that an event can be triggered on a specific element and propagate through its parent elements. This is called event bubbling. We can also stop the propagation of an event using the stopPropagation()
method. Here's an example:
<div id="outer"> <div id="inner"> <button id="myButton">Click Me</button> </div> </div>
const myButton = document.getElementById('myButton'); const outer = document.getElementById('outer'); myButton.addEventListener('click', function(event) { console.log('Button clicked'); event.stopPropagation(); }); outer.addEventListener('click', function() { console.log('Outer div clicked'); });
In this example, we have an outer <div>
and an inner <div>
that contains a button. We add a click
event listener to the button and the outer <div>
. When the button is clicked, the event listener for the outer <div>
is not triggered because we call event.stopPropagation()
.
4. Event Delegation:
Event delegation is a technique where we attach a single event listener to a parent element, instead of attaching multiple event listeners to individual child elements. This can improve performance and simplify event handling. Here's an example:
<ul id="myList"> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul>
const myList = document.getElementById('myList'); myList.addEventListener('click', function(event) { if (event.target.tagName === 'LI') { console.log('Clicked on:', event.target.textContent); } });
In this example, we add a single click
event listener to the <ul>
element. When a <li>
element inside the <ul>
is clicked, the event listener is triggered. We can then access the text content of the clicked <li>
using event.target.textContent
.
Handling events in JavaScript allows us to create interactive web applications by responding to user actions and triggering appropriate behavior in our code.
Using Conditional Statements in JavaScript
Conditional statements in JavaScript allow us to execute different blocks of code based on specified conditions. They provide a way to control the flow of our programs and make decisions based on certain criteria. Let's explore how to use conditional statements in JavaScript.
1. if Statement:
The if
statement is used to execute a block of code if a specified condition is true. Here's an example:
const age = 18; if (age >= 18) { console.log('You are eligible to vote'); }
In this example, we use the if
statement to check if the age
variable is greater than or equal to 18. If the condition is true, the message 'You are eligible to vote'
is logged to the console.
2. if-else Statement:
The if-else
statement is used to execute one block of code if a condition is true, and another block of code if the condition is false. Here's an example:
const hour = new Date().getHours(); if (hour < 12) { console.log('Good morning'); } else { console.log('Good afternoon'); }
In this example, we check the current hour using the getHours()
method of the Date
object. If the hour is less than 12, the message 'Good morning'
is logged; otherwise, the message 'Good afternoon'
is logged.
3. if-else if-else Statement:
The if-else if-else
statement allows us to check multiple conditions and execute different blocks of code accordingly. Here's an example:
const score = 75; if (score >= 90) { console.log('A'); } else if (score >= 80) { console.log('B'); } else if (score >= 70) { console.log('C'); } else { console.log('F'); }
In this example, we check the value of the score
variable and assign a grade based on different score ranges. The appropriate grade is logged to the console.
4. Ternary Operator:
The ternary operator (? :
) provides a shorthand way to write simple conditional statements. It takes a condition, followed by a question mark, an expression to evaluate if the condition is true, a colon, and an expression to evaluate if the condition is false. Here's an example:
const age = 20; const message = age >= 18 ? 'You are an adult' : 'You are not an adult'; console.log(message); // Output: 'You are an adult'
In this example, if the age
variable is greater than or equal to 18, the message 'You are an adult'
is assigned to the message
variable; otherwise, the message 'You are not an adult'
is assigned.
Conditional statements in JavaScript allow us to control the flow of our programs and execute different blocks of code based on specified conditions.
Related Article: How to Integrate Redux with Next.js
Working with Loops in JavaScript
Loops in JavaScript allow us to repeat a block of code multiple times. They provide a way to iterate over arrays, perform operations on collections of data, and execute code based on certain conditions. Let's explore how to work with loops in JavaScript.
1. for Loop:
The for
loop is a common loop in JavaScript that allows us to execute a block of code a specified number of times. Here's an example:
for (let i = 0; i < 5; i++) { console.log(i); }
In this example, the loop variable i
is initialized to 0. The loop continues as long as i
is less than 5. After each iteration, the value of i
is incremented by 1. The loop body logs the value of i
to the console.
2. while Loop:
The while
loop is another type of loop in JavaScript that repeats a block of code as long as a specified condition is true. Here's an example:
let i = 0; while (i < 5) { console.log(i); i++; }
In this example, the loop starts with the variable i
initialized to 0. The loop continues as long as i
is less than 5. After each iteration, the value of i
is incremented by 1. The loop body logs the value of i
to the console.
3. do-while Loop:
The do-while
loop is similar to the while
loop, but it executes the loop body at least once before checking the condition. Here's an example:
let i = 0; do { console.log(i); i++; } while (i < 5);
In this example, the loop starts with the variable i
initialized to 0. The loop body is executed once, and then the condition i < 5
is checked. If the condition is true, the loop continues. After each iteration, the value of i
is incremented by 1. The loop body logs the value of i
to the console.
4. for...in Loop:
The for...in
loop is used to iterate over the properties of an object. It can be used to loop through the keys of an object or the indices of an array. Here's an example:
const person = { name: 'John', age: 30, profession: 'Software Engineer' }; for (const key in person) { console.log(key + ':', person[key]); }
In this example, we use the for...in
loop to iterate over the properties of the person
object. The loop assigns each key of the object to the key
variable, and we can access the corresponding value using person[key]
.
Loops in JavaScript provide a useful way to iterate over arrays, perform operations on collections of data, and automate repetitive tasks in your code.
Popular JavaScript Data Structures
JavaScript offers a variety of data structures that allow us to store, organize, and manipulate data efficiently. Each data structure has its own strengths and use cases. Let's explore some popular JavaScript data structures.
1. Arrays:
Arrays are one of the most commonly used data structures in JavaScript. They allow us to store multiple values in a single variable and provide various methods for manipulating and accessing the data. Here's an example:
const fruits = ['apple', 'banana', 'orange']; console.log(fruits[0]); // Output: 'apple' console.log(fruits.length); // Output: 3 fruits.push('mango'); // Add an element to the end fruits.pop(); // Remove the last element
In this example, we create an array named fruits
and access elements using square brackets. We can also use array methods like push()
to add elements or pop()
to remove elements.
2. Objects:
Objects are key-value pairs that allow us to represent complex data structures. They are useful for modeling real-world entities and provide an efficient way to access and manipulate data. Here's an example:
const person = { name: 'John', age: 30, profession: 'Software Engineer' }; console.log(person.name); // Output: 'John' person.age = 35; // Modify a property person.location = 'New York'; // Add a new property
In this example, we create an object named person
with properties like name
, age
, and profession
. We can access properties using dot notation and modify or add properties as needed.
3. Sets:
Sets are collections of unique values that provide methods for adding, removing, and checking the existence of elements. They are useful when we need to work with unique values or perform operations like set intersections or unions. Here's an example:
const set = new Set(); set.add('apple'); set.add('banana'); console.log(set.size); // Output: 2 console.log(set.has('banana')); // Output: true set.delete('apple');
In this example, we create a set and add elements using the add()
method. We can check if an element exists using the has()
method and remove elements using the delete()
method.
4. Maps:
Maps are key-value pairs similar to objects, but with some differences. They allow any type of key and provide methods for adding, removing, and iterating over entries. They are useful when we need to maintain the order of elements or perform operations like key lookups. Here's an example:
const map = new Map(); map.set('name', 'John'); map.set('age', 30); console.log(map.get('name')); // Output: 'John' console.log(map.size); // Output: 2 map.delete('age');
In this example, we create a map and add entries using the set()
method. We can retrieve values using the get()
method and remove entries using the delete()
method.
These are just a few examples of popular JavaScript data structures. Depending on the requirements of your application, you can choose the appropriate data structure to store and manipulate your data efficiently.
Additional Resources
- W3Schools - JavaScript Dropdowns