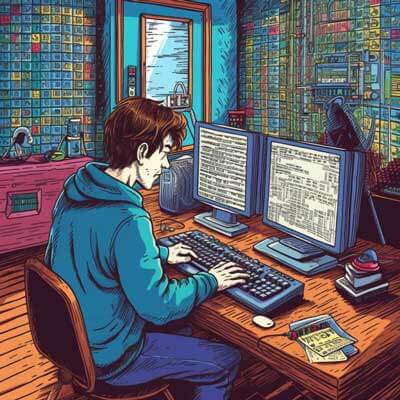
Converting a PHP object to an associative array is a common task when working with PHP. There are several reasons why you might need to convert an object to an array, such as:
– You want to pass the data to a function or method that expects an array.
– You need to manipulate or analyze the data in a format that is more convenient to work with.
– You want to convert the object to JSON for sending it over an API or storing it in a database.
In PHP, there are a few different ways to convert an object to an associative array. Let’s explore some of the most commonly used methods.
Method 1: Typecasting
One straightforward way to convert an object to an associative array is to use typecasting. PHP allows you to cast an object to an array, which will create an associative array where the object properties become the keys, and their values become the corresponding values of the array.
Here’s an example:
$obj = new stdClass(); $obj->name = 'John'; $obj->age = 30; $obj->city = 'New York'; $array = (array) $obj; print_r($array);
Output:
Array ( [name] => John [age] => 30 [city] => New York )
By using typecasting, you can quickly convert an object to an associative array. However, there are a few things to keep in mind:
– Typecasting can only be used on objects of the stdClass
class or classes that do not have any private or protected properties. If the object has private or protected properties, they will not be included in the resulting array.
– Typecasting may not preserve the data types of the object properties. For example, if the object property is an integer, it will be converted to a string in the array.
Related Article: How to Work with Arrays in PHP (with Advanced Examples)
Method 2: JSON Encoding and Decoding
Another way to convert an object to an associative array is by using JSON encoding and decoding. PHP provides built-in functions for encoding and decoding JSON, which can be used to convert objects to arrays and vice versa.
Here’s an example:
$obj = new stdClass(); $obj->name = 'John'; $obj->age = 30; $obj->city = 'New York'; $json = json_encode($obj); $array = json_decode($json, true); print_r($array);
Output:
Array ( [name] => John [age] => 30 [city] => New York )
In this example, we first encode the object to JSON using the json_encode
function. The resulting JSON string is then decoded using the json_decode
function with the second parameter set to true
. This tells PHP to decode the JSON string as an associative array.
Using JSON encoding and decoding provides more flexibility than typecasting because it can handle objects of any class, including those with private or protected properties. It also preserves the data types of the object properties in the resulting array.
Method 3: Iterating over Object Properties
If you need more control over the conversion process, you can manually iterate over the object properties and build the associative array yourself. This method allows you to filter or transform the object properties as needed.
Here’s an example:
$obj = new stdClass(); $obj->name = 'John'; $obj->age = 30; $obj->city = 'New York'; $array = []; foreach ($obj as $key => $value) { $array[$key] = $value; } print_r($array);
Output:
Array ( [name] => John [age] => 30 [city] => New York )
In this example, we use a foreach loop to iterate over the object properties. For each property, we assign the property name as the key and the property value as the value in the associative array.
Using this method, you have full control over the conversion process and can perform additional operations on the object properties if needed.
Related Article: How To Make A Redirect In PHP