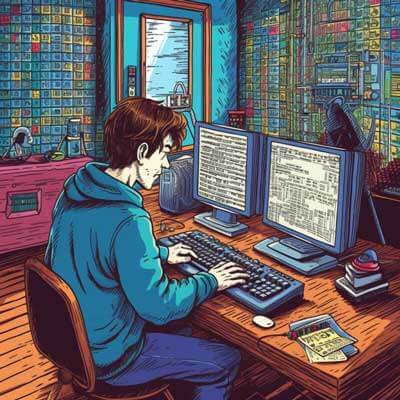
Table of Contents
Converting a string to an integer is a common task in javascript. There are several methods available for converting a string to an integer in javascript. In this answer, we will explore two popular methods: using the parseInt()
function and using the unary plus operator.
Method 1: Using the parseInt() function
The parseInt()
function in javascript is used to parse a string and return an integer. This function takes two parameters: the string to be parsed and the radix (optional).
Here is an example of using the parseInt()
function to convert a string to an integer:
var stringNumber = "42"; var integerNumber = parseInt(stringNumber); console.log(integerNumber); // Output: 42
In the above example, the parseInt()
function is used to convert the string "42" to an integer. The resulting integer is then stored in the variable integerNumber
.
If the radix parameter is not specified, parseInt()
assumes a radix of 10. However, it is considered a best practice to always specify the radix explicitly to avoid unexpected results. For example:
var stringNumber = "10"; var integerNumber = parseInt(stringNumber, 10); console.log(integerNumber); // Output: 10
In this example, the radix parameter is explicitly set to 10, indicating that the string should be interpreted as a decimal number.
Related Article: How To Check If a Key Exists In a Javascript Object
Method 2: Using the unary plus operator
Another way to convert a string to an integer in javascript is by using the unary plus operator (+
). The unary plus operator can convert a string containing a numeric value to a number data type.
Here is an example of using the unary plus operator to convert a string to an integer:
var stringNumber = "42"; var integerNumber = +stringNumber; console.log(integerNumber); // Output: 42
In the above example, the unary plus operator is used to convert the string "42" to an integer.
It is important to note that if the string cannot be converted to a valid number, the unary plus operator will return NaN
(Not-a-Number). For example:
var stringNumber = "Hello"; var integerNumber = +stringNumber; console.log(integerNumber); // Output: NaN
In this example, the string "Hello" cannot be converted to a valid number, so the unary plus operator returns NaN
.
Why is the conversion of string to integer needed?
The need to convert a string to an integer often arises when working with user input or when performing calculations that require numerical values. For example, when validating a form field that accepts numbers, it is necessary to convert the user's input from a string to an integer before performing any calculations or comparisons.
Another common use case is when working with APIs that return data in string format. In such cases, it may be necessary to convert the string data to an integer in order to perform mathematical operations on the data.
Best practices and considerations
When converting a string to an integer in javascript, there are a few best practices and considerations to keep in mind:
1. Always specify the radix parameter: When using the parseInt()
function, it is considered a best practice to always specify the radix parameter explicitly. This helps avoid unexpected results, especially when working with strings that start with a leading zero (which could be interpreted as an octal number) or when working with non-decimal radix values.
2. Handle invalid input: When converting a string to an integer, it is important to handle cases where the string cannot be converted to a valid number. Both the parseInt()
function and the unary plus operator will return NaN
if the string cannot be converted. It is a good practice to check for this condition and handle it appropriately in your code.
3. Consider using other conversion methods: In addition to the parseInt()
function and the unary plus operator, javascript provides other methods for converting strings to numbers, such as the Number()
constructor and the parseFloat()
function. Depending on your specific use case, these methods may be more suitable.
Related Article: How to Read an External Local JSON File in Javascript
Alternative ideas
Apart from the parseInt()
function and the unary plus operator, there are alternative ways to convert a string to an integer in javascript:
1. Using the Number()
constructor: The Number()
constructor can be used to convert a string to a number. It works similarly to the unary plus operator, but with some subtle differences. For example:
var stringNumber = "42"; var integerNumber = Number(stringNumber); console.log(integerNumber); // Output: 42
2. Using the parseFloat()
function: The parseFloat()
function can be used to convert a string to a floating-point number. If you specifically need an integer, you can use the Math.floor()
or Math.ceil()
functions to round the result. For example:
var stringNumber = "42.5"; var integerNumber = Math.floor(parseFloat(stringNumber)); console.log(integerNumber); // Output: 42
Both of these alternative methods have their own advantages and considerations, so it is important to choose the method that best suits your specific use case.