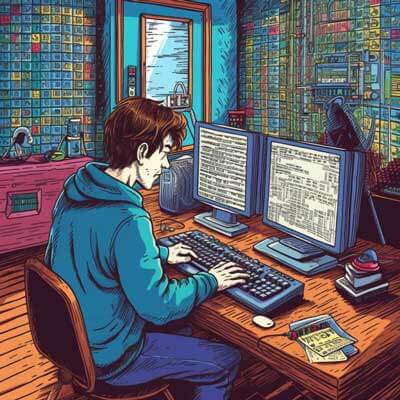
Creating a countdown timer with JavaScript is a common task in web development. It can be used for various purposes, such as displaying the remaining time for a sale, counting down to a specific event, or showing the time left for a task to be completed. In this guide, we will explore two different approaches to create a countdown timer using JavaScript.
Approach 1: Using setInterval()
One way to create a countdown timer is by utilizing the setInterval() function in JavaScript. This function allows you to repeatedly execute a given function at a specified interval. Here’s how you can use it to create a countdown timer:
// <a href="https://www.squash.io/how-to-set-time-delay-in-javascript/">Set the target date and time</a> for the countdown const targetDate = new Date("2022-12-31T23:59:59"); // Get the current date and time const currentDate = new Date(); // Calculate the time difference between the target date and the current date const timeDifference = targetDate.getTime() - currentDate.getTime(); // Convert the time difference to seconds const totalSeconds = Math.floor(timeDifference / 1000); // Define a function to update the countdown timer function updateCountdown() { // Calculate the remaining hours, minutes, and seconds const hours = Math.floor(totalSeconds / 3600); const minutes = Math.floor((totalSeconds % 3600) / 60); const seconds = Math.floor(totalSeconds % 60); // Display the countdown timer console.log(`${hours} hours, ${minutes} minutes, ${seconds} seconds remaining`); // Decrease the totalSeconds by 1 totalSeconds--; // Check if the countdown has reached zero if (totalSeconds < 0) { console.log("Countdown has ended"); clearInterval(intervalId); } } // Call the updateCountdown function every second const intervalId = setInterval(updateCountdown, 1000);
In this code snippet, we first set the target date and time for the countdown. Then, we calculate the time difference between the target date and the current date. By converting the time difference to seconds, we can easily calculate the remaining hours, minutes, and seconds. The updateCountdown() function is responsible for displaying the countdown timer and decreasing the totalSeconds by 1. We also check if the countdown has reached zero and clear the interval if it has.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Approach 2: Using setTimeout()
Another approach to create a countdown timer is by utilizing the setTimeout() function in JavaScript. This function allows you to execute a given function after a specified delay. Here’s how you can use it to create a countdown timer:
// Set the target date and time for the countdown const targetDate = new Date("2022-12-31T23:59:59"); // Define a function to update the countdown timer function updateCountdown() { // Get the current date and time const currentDate = new Date(); // Calculate the time difference between the target date and the current date const timeDifference = targetDate.getTime() - currentDate.getTime(); // Convert the time difference to seconds const totalSeconds = Math.floor(timeDifference / 1000); // Calculate the remaining hours, minutes, and seconds const hours = Math.floor(totalSeconds / 3600); const minutes = Math.floor((totalSeconds % 3600) / 60); const seconds = Math.floor(totalSeconds % 60); // Display the countdown timer console.log(`${hours} hours, ${minutes} minutes, ${seconds} seconds remaining`); // Check if the countdown has reached zero if (totalSeconds > 0) { // Call the updateCountdown function again after 1 second setTimeout(updateCountdown, 1000); } else { console.log("Countdown has ended"); } } // Call the updateCountdown function initially updateCountdown();
In this code snippet, we set the target date and time for the countdown and define the updateCountdown() function. Inside the function, we get the current date and time, calculate the time difference, and convert it to seconds. We then calculate the remaining hours, minutes, and seconds and display the countdown timer. If the countdown has not reached zero, we call the updateCountdown() function again after 1 second using setTimeout(). If the countdown has reached zero, we log the appropriate message.
Best Practices
When creating a countdown timer with JavaScript, it’s important to keep a few best practices in mind:
– Use the built-in Date object in JavaScript to handle dates and times effectively.
– Consider using a library like Moment.js for more advanced date and time manipulation.
– Format the countdown timer output to provide a clear and user-friendly display.
– Test your countdown timer thoroughly to ensure it works correctly in different scenarios.
– Optimize the performance of your countdown timer code by minimizing unnecessary calculations and updates.
Alternative Ideas
While the approaches mentioned above are commonly used for creating countdown timers, there are alternative ideas worth exploring:
– Utilize a front-end framework like React or Vue.js to build a more interactive and dynamic countdown timer component.
– Customize the visual appearance of the countdown timer to match the design of your website or application.
– Add additional functionality to the countdown timer, such as pausing, resetting, or restarting the timer.
– Implement sound effects or animations to enhance the user experience of the countdown timer.
These alternative ideas can help you create unique and engaging countdown timers tailored to your specific needs.
Related Article: How to Use the forEach Loop with JavaScript