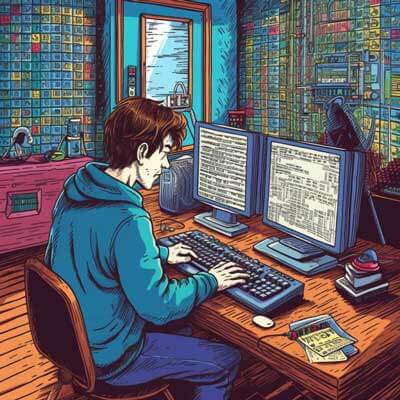
To fetch and post JSON data in Javascript, you can use the built-in Fetch API. This API provides a way to make HTTP requests, including fetching data from an external API and sending data to a server. Here are two possible ways to fetch and post JSON data in Javascript:
Using the Fetch API
The Fetch API provides a simple and flexible way to make HTTP requests. To fetch JSON data, you can use the fetch()
function and then use the .json()
method to parse the response:
fetch(url) .then(response => response.json()) .then(data => { // Handle the JSON data here }) .catch(error => { // Handle any errors here });
In this example, replace url
with the URL of the JSON API you want to fetch from. The fetch()
function returns a Promise that resolves to the response from the server. You can then use the .json()
method on the response object to parse the response as JSON data. The resulting JSON data will be available in the data
variable inside the second .then()
callback.
To post JSON data, you can use the fetch()
function with the method
option set to 'POST'
and the body
option set to the JSON data:
fetch(url, { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(data) }) .then(response => response.json()) .then(responseData => { // Handle the response data here }) .catch(error => { // Handle any errors here });
In this example, replace url
with the URL of the server you want to send the JSON data to, and replace data
with the JSON data you want to send. The fetch()
function takes an optional second argument that specifies the options for the request. In this case, we set the method
option to 'POST'
to indicate that we want to make a POST request, and we set the headers
option to specify that the request body contains JSON data. The body
option is set to the JSON data, which is first converted to a string using JSON.stringify()
.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Alternative Approach: Using XMLHttpRequest
Another way to fetch and post JSON data in Javascript is by using the XMLHttpRequest object. This is an older method but is still widely supported:
var xhr = new XMLHttpRequest(); xhr.open('GET', url, true); xhr.responseType = 'json'; xhr.onload = function() { if (xhr.status === 200) { var data = xhr.response; // Handle the JSON data here } else { // Handle errors here } }; xhr.send();
This example demonstrates how to fetch JSON data using a GET request. Replace url
with the URL of the JSON API you want to fetch from. The XMLHttpRequest
object is created using the new XMLHttpRequest()
constructor. The open()
method initializes the request, specifying the HTTP method (‘GET’ in this case), the URL, and whether the request should be asynchronous (set to true
in this example). The responseType
property is set to 'json'
to indicate that the response should be parsed as JSON. The onload
event handler is triggered when the request is complete, and you can access the JSON data from the response
property of the XMLHttpRequest
object.
To post JSON data using XMLHttpRequest, you can use a similar approach:
var xhr = new XMLHttpRequest(); xhr.open('POST', url, true); xhr.setRequestHeader('Content-Type', 'application/json'); xhr.onload = function() { if (xhr.status === 200) { var responseData = xhr.response; // Handle the response data here } else { // Handle errors here } }; xhr.send(JSON.stringify(data));
In this example, replace url
with the URL of the server you want to send the JSON data to, and replace data
with the JSON data you want to send. The open()
method is used to initialize the request, with the method
option set to 'POST'
, the URL, and the asynchronous flag set to true
. The setRequestHeader()
method is used to set the Content-Type
header to indicate that the request body contains JSON data. The onload
event handler is triggered when the request is complete, and you can access the response data from the response
property of the XMLHttpRequest
object.
Best Practices
When fetching and posting JSON data in Javascript, there are some best practices to keep in mind:
– Always handle errors and network failures. Use the .catch()
method or the onerror
event handler to handle any errors that occur during the request.
– Validate and sanitize user input before sending it to the server to prevent security vulnerabilities.
– Consider using a library or framework that provides a higher-level abstraction for making HTTP requests, such as Axios or jQuery’s AJAX functions. These libraries can simplify the process and provide additional features, such as automatic JSON parsing and error handling.
– Be mindful of cross-origin resource sharing (CORS) restrictions when making requests to a different domain. The server needs to be configured to allow requests from your domain.
– Use appropriate HTTP methods for your requests (GET, POST, PUT, DELETE, etc.) based on the intended action.
References:
– [Fetch API – MDN Web Docs](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API)
– [XMLHttpRequest – MDN Web Docs](https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest)
– [Axios – GitHub](https://github.com/axios/axios)
– [jQuery AJAX – jQuery API Documentation](https://api.jquery.com/category/ajax/)
Related Article: How to Use the forEach Loop with JavaScript