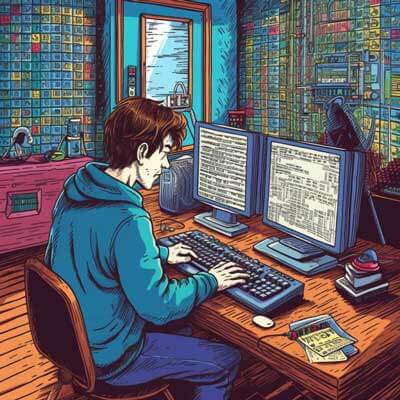
- What is a web worker and how does it work in JavaScript?
- What is Next.js and how does it differ from regular JavaScript?
- Why would I want to use web workers in Next.js?
- What are the benefits of using web workers in Next.js?
- How can I implement web workers in Next.js?
- Are web workers supported in all browsers?
- How do web workers help with concurrency in JavaScript?
- Can web workers be used for both frontend and backend development?
- What is the difference between concurrency and parallelism in JavaScript?
- Additional Resources
What is a web worker and how does it work in JavaScript?
A web worker is a JavaScript script that runs in the background, separate from the main browser thread. It allows you to perform time-consuming tasks without blocking the user interface or making the website unresponsive. Web workers are designed to handle complex computations, heavy calculations, or any other tasks that can be done independently of the main thread.
Web workers operate by running scripts in the background on a separate thread. This thread is completely isolated from the main thread, which means it doesn’t have access to the DOM or other web APIs directly. Instead, it communicates with the main thread using the postMessage() and onmessage event handlers.
To create and use a web worker in JavaScript, you need to follow these steps:
1. Create a new worker: You can create a new web worker by calling the Worker() constructor and passing the URL of the JavaScript file that will be executed in the worker thread.
const worker = new Worker('worker.js');
2. Handle messages from the worker: To receive messages from the worker, you need to attach an event listener to the worker’s onmessage property. This event listener will be triggered whenever the worker sends a message using the postMessage() method.
worker.onmessage = function(event) { const message = event.data; console.log('Message received from worker:', message); };
3. Send messages to the worker: To send messages to the worker, you can use the postMessage() method. This method accepts a single argument, which can be any JSON-serializable data.
worker.postMessage('Hello from the main thread!');
4. Handle messages in the worker: Inside the worker script, you can listen for messages from the main thread using the onmessage event handler. When a message is received, you can perform any necessary computations and send the result back to the main thread using the postMessage() method.
self.onmessage = function(event) { const message = event.data; console.log('Message received from main thread:', message); const result = performComplexCalculation(message); self.postMessage(result); };
Web workers provide a useful way to offload CPU-intensive tasks to separate threads, improving the overall performance and responsiveness of web applications. However, it’s important to note that web workers have some limitations, such as the inability to directly access the DOM or interact with certain web APIs.
Related Article: 25 Handy Javascript Code Snippets for Everyday Use
What is Next.js and how does it differ from regular JavaScript?
Next.js is a popular framework for building server-side rendered (SSR) React applications. It is built on top of React and provides many features and optimizations out of the box, making it easier to develop scalable and performant web applications.
Next.js differs from regular JavaScript in several ways:
1. Server-side rendering: Next.js allows you to render React components on the server before sending them to the client. This means that the initial page load is faster, as the server can pre-render the HTML and CSS, instead of relying on the client to do it. This is particularly useful for improving SEO and providing a better user experience.
2. Automatic code splitting: With Next.js, you can automatically split your JavaScript code into smaller chunks, which are loaded on-demand. This helps reduce the initial load time and improves the performance of your application. Next.js uses a technique called “lazy loading” to only load the JavaScript code that is needed for a particular page.
3. Routing: Next.js provides a built-in routing system that allows you to define your application’s routes using a file-based approach. You can create a file with the same name as the route, and Next.js will automatically generate the corresponding route for you. This makes it easier to organize and maintain your application’s routes.
4. API routes: Next.js allows you to define serverless API endpoints within your application by creating special files in the /pages/api
directory. These API routes can handle HTTP requests and return JSON data, making it easy to build backend functionality directly into your Next.js application.
5. CSS-in-JS support: Next.js has built-in support for CSS-in-JS libraries like styled-components and CSS modules. This allows you to write CSS styles directly in your JavaScript code, making it easier to encapsulate styles and avoid naming conflicts.
Overall, Next.js provides a more opinionated and streamlined approach to building React applications, with built-in support for server-side rendering, code splitting, routing, and more.
Why would I want to use web workers in Next.js?
Web workers can be particularly useful in Next.js applications for offloading CPU-intensive tasks and improving the overall performance and responsiveness of the application. Here are a few reasons why you might want to use web workers in Next.js:
1. Improved user experience: By using web workers to perform heavy computations or time-consuming tasks, you can prevent the main thread from being blocked, ensuring that the user interface remains responsive and smooth. This can greatly enhance the user experience, especially when dealing with complex calculations or data processing.
2. Faster page load times: Next.js already provides server-side rendering, which helps improve the initial page load time. However, there might still be cases where you need to perform additional computations or data processing on the client-side. By using web workers, you can parallelize these tasks and speed up the overall page load time.
3. Efficient resource utilization: Web workers run on separate threads, which means they can utilize additional CPU cores and resources available on the user’s device. By distributing the workload across multiple threads, you can make better use of the available resources and improve the overall performance of your Next.js application.
4. Scalability: When building large-scale Next.js applications, it’s important to consider scalability. By offloading CPU-intensive tasks to web workers, you can distribute the workload across multiple threads or even multiple devices, allowing your application to handle larger workloads without sacrificing performance.
What are the benefits of using web workers in Next.js?
Using web workers in Next.js can provide several benefits, including:
1. Improved performance: Web workers allow you to perform CPU-intensive tasks in the background without blocking the main thread. This can greatly improve the performance of your Next.js application, as the user interface remains responsive and smooth even when performing complex computations or heavy calculations.
2. Enhanced user experience: By offloading time-consuming tasks to web workers, you can prevent the user interface from freezing or becoming unresponsive. This helps provide a better user experience, as the application remains interactive and responsive, even when performing computationally intensive operations.
3. Parallel processing: Web workers run on separate threads, which means they can execute tasks in parallel. This can be especially useful for Next.js applications that require performing multiple computations or data processing operations simultaneously. By utilizing multiple threads, you can speed up the overall processing time and improve the application’s responsiveness.
4. Scalability: Next.js applications can benefit from using web workers for scalability purposes. By distributing the workload across multiple threads or even multiple devices, you can handle larger workloads and improve the overall scalability of your application.
5. Code organization and maintainability: By separating computationally intensive tasks into web workers, you can keep your main thread codebase clean and focused on handling user interactions and UI updates. This can improve code organization and maintainability, as the logic for complex computations is encapsulated within the web workers, making it easier to understand and maintain.
Overall, using web workers in Next.js can help improve performance, enhance the user experience, enable parallel processing, and enhance the scalability and maintainability of your application.
Related Article: Accessing Parent State from Child Components in Next.js
How can I implement web workers in Next.js?
To implement web workers in a Next.js application, you can follow these steps:
1. Create a new web worker file: In your Next.js project, create a new JavaScript file that will serve as your web worker. This file should contain the logic for the computations or tasks that you want to offload to the web worker. For example, let’s create a file named “worker.js” in the root of our project.
2. Define the logic in the web worker file: Inside the “worker.js” file, define the logic for the computations or tasks that you want the web worker to perform. This can include complex calculations, data processing, or any other time-consuming operation that can be done independently of the main thread. For example, let’s define a simple function that calculates the factorial of a given number.
// worker.js self.onmessage = function(event) { const number = event.data; const result = calculateFactorial(number); self.postMessage(result); }; function calculateFactorial(number) { let result = 1; for (let i = 2; i { const worker = new Worker('/worker.js'); worker.onmessage = function(event) { const result = event.data; setFactorial(result); worker.terminate(); }; worker.postMessage(number); }; return ( <div> setNumber(Number(e.target.value))} /> <button>Calculate Factorial</button> <p>Factorial: {factorial !== null ? factorial : 'N/A'}</p> </div> ); }
In this example, we create a Next.js component called “FactorialCalculator” that allows the user to enter a number and calculate its factorial. When the user clicks the “Calculate Factorial” button, we create a new web worker using the Worker() constructor and pass the URL of the web worker file. We then send the user-entered number to the web worker using the postMessage() method. When the web worker finishes the computation, it sends the result back using the onmessage event handler, where we update the state with the calculated factorial value.
4. Add the necessary configuration: Next.js requires some additional configuration to allow importing the web worker file. In your Next.js project’s configuration file (usually next.config.js), add the following configuration to allow importing JavaScript files as web workers:
module.exports = { webpack: (config, { isServer }) => { if (!isServer) { config.output.globalObject = 'self'; } return config; }, };
With this configuration, Next.js will treat JavaScript files as web workers and allow you to import them using the Worker() constructor.
5. Run your Next.js application: Start your Next.js application using the appropriate command (e.g., “npm run dev” or “yarn dev”). The web worker will be loaded and executed in the background, allowing you to perform heavy computations or time-consuming tasks without blocking the main thread.
Are web workers supported in all browsers?
Web workers are supported in most modern browsers, but there are some exceptions and limitations to be aware of. Here is a breakdown of web worker support in different browsers:
– Chrome: Web workers are fully supported in Chrome, including support for SharedWorkers, which allow multiple browser tabs or windows to communicate with the same worker script.
– Firefox: Web workers are fully supported in Firefox, including support for SharedWorkers. Firefox also provides additional developer tools and debugging capabilities for working with web workers.
– Safari: Web workers are supported in Safari, but there are some limitations. Safari does not support SharedWorkers, and there are restrictions on the amount of data that can be transferred between the main thread and the web worker.
– Edge: Web workers are supported in Microsoft Edge, including support for SharedWorkers. However, Microsoft Edge is being replaced by the Chromium-based Microsoft Edge, which has full support for web workers.
– Internet Explorer: Web workers are partially supported in Internet Explorer, starting from version 10. However, there are some limitations and differences in behavior compared to other browsers, so it’s recommended to test your web worker code thoroughly in Internet Explorer.
– Mobile browsers: Most modern mobile browsers, including Chrome for Android and Safari for iOS, have support for web workers. However, it’s important to test your web worker code on different mobile devices and browsers to ensure compatibility.
Overall, web workers are widely supported in modern browsers, making them a reliable choice for offloading CPU-intensive tasks in web applications. However, it’s always a good idea to test your web worker code on different browsers and devices to ensure compatibility.
How do web workers help with concurrency in JavaScript?
In JavaScript, concurrency refers to the ability to execute multiple tasks or operations simultaneously. However, JavaScript is single-threaded, which means it can only execute one task at a time. This can lead to performance issues and a lack of responsiveness, especially when dealing with computationally intensive tasks.
Web workers help with concurrency in JavaScript by allowing you to offload CPU-intensive tasks to separate threads, which can run in parallel with the main thread. This enables concurrent execution of multiple tasks, improving performance and responsiveness.
Here’s how web workers help with concurrency in JavaScript:
1. Offloading tasks to separate threads: Web workers run on separate threads, which are independent of the main thread. By offloading CPU-intensive tasks to web workers, you can ensure that these tasks run concurrently with the main thread, without blocking the user interface or making the website unresponsive.
2. Parallel processing: Web workers enable parallel processing by running on separate threads. This means that multiple tasks can be executed simultaneously, utilizing the available CPU cores and resources more efficiently. By distributing the workload across multiple threads, you can achieve better performance and faster execution times.
3. Asynchronous communication: Web workers communicate with the main thread using asynchronous messaging. This means that the main thread can send messages to the web worker and continue executing other tasks without waiting for a response. Similarly, the web worker can perform computations or tasks independently and send the results back to the main thread asynchronously.
4. Improved performance and responsiveness: By offloading CPU-intensive tasks to web workers and allowing them to run in parallel, you can improve the overall performance and responsiveness of your JavaScript applications. The main thread remains free to handle user interactions, UI updates, and other tasks, ensuring a smooth and responsive user experience.
Web workers provide a useful mechanism for achieving concurrency in JavaScript, enabling concurrent execution of multiple tasks and improved performance. However, it’s important to note that web workers have some limitations, such as the inability to directly access the DOM or interact with certain web APIs. Nevertheless, they are a valuable tool for handling computationally intensive tasks and improving concurrency in JavaScript applications.
Related Article: Advanced DB Queries with Nodejs, Sequelize & Knex.js
Can web workers be used for both frontend and backend development?
Web workers are primarily designed for frontend development and are typically used in web browsers to offload CPU-intensive tasks from the main thread. However, they are not limited to frontend development and can be used for backend development as well, although with some limitations and considerations.
In frontend development, web workers are commonly used to perform computationally intensive tasks, such as complex calculations, data processing, or image manipulation. By offloading these tasks to web workers, the main thread remains free to handle user interactions and UI updates, ensuring a smooth and responsive user experience.
In backend development, web workers can also be used to handle computationally intensive tasks or parallelize processing. For example, in a Node.js application, you can use the “worker_threads” module to create and manage web workers. This allows you to distribute the workload across multiple threads, utilizing the available CPU cores and resources more efficiently.
However, there are some limitations and considerations when using web workers for backend development:
1. Compatibility: Web workers are primarily supported in web browsers, so if you’re using web workers in a backend environment, you need to ensure that the environment supports them. For example, Node.js has built-in support for web workers using the “worker_threads” module, but other backend environments may not have native support.
2. Inter-process communication: When using web workers in backend development, you may need to consider how to communicate between different processes or threads. For example, in a Node.js application, you can use message passing or other inter-process communication mechanisms to exchange data between web workers.
3. Shared state and resources: Web workers run in separate threads or processes, which means they have their own isolated state and resources. If you need to share state or resources between web workers, you need to consider synchronization and coordination mechanisms, such as locks, semaphores, or message queues.
4. Scalability and resource utilization: Web workers can help improve the scalability and resource utilization of backend applications by distributing the workload across multiple threads or processes. However, you need to carefully design your application to take full advantage of web workers and ensure that the workload can be effectively distributed across the available resources.
Overall, while web workers are primarily designed for frontend development, they can be used for backend development as well, with some limitations and considerations. They provide a mechanism for offloading CPU-intensive tasks, achieving parallel processing, and improving the performance and scalability of backend applications. However, the specific implementation and usage may vary depending on the backend environment and requirements.
What is the difference between concurrency and parallelism in JavaScript?
In JavaScript, concurrency and parallelism refer to different ways of achieving multiple tasks or operations simultaneously. While they are related concepts, there is a subtle difference between them:
Concurrency: Concurrency in JavaScript refers to the ability to execute multiple tasks or operations independently, without blocking each other. Concurrency is typically achieved through techniques such as asynchronous programming, event-driven architecture, or the use of web workers. In a concurrent system, tasks can be interleaved or executed in an overlapping manner, but not necessarily at the same time.
For example, in JavaScript, you can use asynchronous functions, promises, or callbacks to achieve concurrency. By making use of non-blocking I/O operations or timers, you can initiate multiple tasks and let them run concurrently, without waiting for each other to complete. This allows for efficient utilization of resources and improved responsiveness.
Parallelism: Parallelism in JavaScript refers to the ability to execute multiple tasks or operations at the same time, using multiple threads or processes. Parallelism is typically achieved through techniques such as web workers or the use of multi-threaded frameworks like Node.js with the “worker_threads” module. In a parallel system, tasks are executed simultaneously, utilizing multiple CPU cores or resources.
For example, in JavaScript, you can use web workers to achieve parallelism. Web workers allow you to offload CPU-intensive tasks to separate threads, which can run in parallel with the main thread. This enables concurrent execution of multiple tasks, improving performance and responsiveness.
The main difference between concurrency and parallelism is that concurrency focuses on independent task execution and non-blocking behavior, while parallelism focuses on simultaneous task execution using multiple threads or processes. Concurrency is about managing tasks effectively and allowing them to progress without blocking each other, while parallelism is about utilizing multiple resources to achieve faster execution times.
In JavaScript, both concurrency and parallelism can be valuable techniques for achieving better performance, responsiveness, and scalability. The choice between them depends on the specific requirements of your application and the available resources.
Additional Resources
– Introduction to Web Workers
– JavaScript Web Workers
– Concurrency in Web Workers