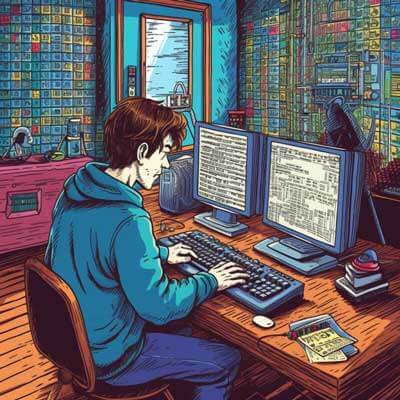
Table of Contents
Introduction
Validating passwords is an essential part of web application development to ensure the security and integrity of user data. Regular expressions, also known as regex, provide a useful tool for performing pattern matching and validation in JavaScript. In this answer, we will explore how to use regex for password validation in JavaScript.
Related Article: How to Reverse an Array in Javascript Without Libraries
Using Regex for Password Validation
When using regex for password validation, you can define a pattern that specifies the rules for a valid password. Here are some common requirements for a strong password:
Length Requirement
One common requirement is to enforce a minimum length for the password. For example, you may want to require passwords to be at least 8 characters long. You can use the following regex pattern to enforce this requirement:
const passwordRegex = /^.{8,}$/;
This pattern uses the ^
and $
anchors to match the entire input string and the .
character to match any character except for a newline. The {8,}
quantifier specifies that the previous token (in this case, the .
character) must occur at least 8 times.
Character Set Requirements
You may also want to enforce specific character set requirements for the password, such as requiring at least one uppercase letter, one lowercase letter, one digit, and one special character. You can use lookahead assertions to achieve this:
const passwordRegex = /^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[!@#$%^&*()\-_=+[{\]};:'",/?]).{8,}$/;
This pattern uses lookahead assertions (?!...)
to ensure that the password contains at least one lowercase letter ((?=.*[a-z])
), one uppercase letter ((?=.*[A-Z])
), one digit ((?=.*\d)
), and one special character ((?=.*[!@#$%^&*()\-_=+[{\]};:'",/?])
). The .{8,}
quantifier is used to enforce a minimum length of 8 characters.
Additional Requirements
In addition to the length and character set requirements, you may have additional rules for password validation, such as disallowing common passwords or enforcing a maximum length. You can customize the regex pattern to include these requirements as well.
Best Practices
Here are some best practices when using regex for password validation in JavaScript:
1. Use clear error messages: When a password fails validation, provide clear and specific error messages to help users understand the requirements and fix any issues.
2. Allow special characters: Special characters can significantly increase the strength of a password. Instead of restricting special characters, consider allowing a wide range of them.
3. Consider usability: While strong passwords are important, overly complex requirements can make it difficult for users to remember their passwords. Strike a balance between security and usability.
4. Test thoroughly: Regular expressions can be complex, so it's crucial to thoroughly test your regex pattern with various inputs to ensure it behaves as expected.
Alternative Approaches
While regex is a useful tool for password validation, there are alternative approaches you can consider:
1. Use a dedicated password validation library: Instead of writing your own regex pattern, you can use a library specifically designed for password validation, such as zxcvbn (https://github.com/dropbox/zxcvbn). These libraries often provide more advanced features, such as password strength estimation.
2. Combine regex with other validation techniques: Regex can be used in combination with other validation techniques, such as checking against a list of common passwords, enforcing a maximum length, or using a scoring system to evaluate password strength.