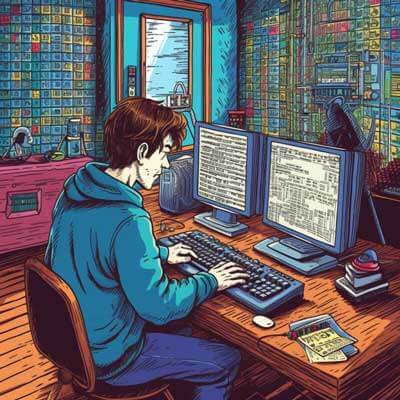
Table of Contents
There are several ways to enable and disable an HTML input button using JavaScript. In this answer, we will explore two common methods: using the disabled property and manipulating the button's CSS class.
Method 1: Using the disabled Property
One way to enable and disable an HTML input button is by using the disabled property. The disabled property is a boolean attribute that, when present, prevents the button from being interacted with.
To enable or disable a button using the disabled property, you can access the button element in JavaScript and set its disabled property to true or false.
Here's an example of how to enable and disable a button using the disabled property:
<button id="myButton">Click Me</button> // Disable the button document.getElementById("myButton").disabled = true; // Enable the button document.getElementById("myButton").disabled = false;
In the above example, the button starts off disabled. When document.getElementById("myButton").disabled = true;
is called, the button becomes disabled and cannot be interacted with. Conversely, when document.getElementById("myButton").disabled = false;
is called, the button becomes enabled again.
Related Article: Integrating JavaScript Functions in React Components
Method 2: Manipulating the CSS Class
Another way to enable and disable an HTML input button is by manipulating the button's CSS class. You can define different CSS styles for the enabled and disabled states of the button, and then add or remove the corresponding CSS class to enable or disable the button.
Here's an example of how to enable and disable a button by manipulating the CSS class:
.disabled { opacity: 0.5; pointer-events: none; } <button id="myButton" class="disabled">Click Me</button> // Enable the button document.getElementById("myButton").classList.remove("disabled"); // Disable the button document.getElementById("myButton").classList.add("disabled");
In the above example, the button starts off with the "disabled" class, which sets the opacity to 0.5 and disables pointer events. When document.getElementById("myButton").classList.remove("disabled");
is called, the "disabled" class is removed, enabling the button. Conversely, when document.getElementById("myButton").classList.add("disabled");
is called, the "disabled" class is added back, disabling the button.
Alternative Ideas and Best Practices
Related Article: How to Copy to the Clipboard in Javascript
- Instead of directly accessing the button element using document.getElementById
, you can also use other DOM traversal methods like querySelector
or getElementsByClassName
.
- If you have multiple buttons that need to be enabled or disabled together, consider using a loop to iterate over the buttons and apply the desired state.
- It is good practice to provide visual feedback to users when a button is disabled. You can modify the button's appearance using CSS to make it visually distinct from enabled buttons.
- When disabling a button, you may also want to consider disabling any associated form fields or performing additional actions to prevent unintended interactions.