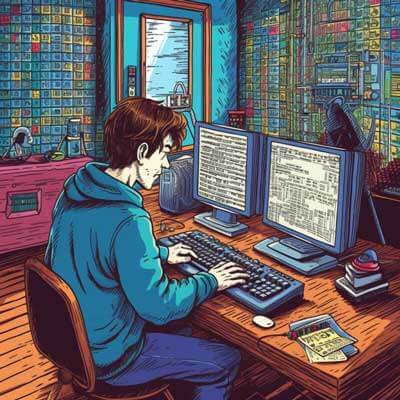
Table of Contents
Efficient Queries with JavaScript
When working with databases, efficient querying is essential for retrieving data quickly and optimizing performance. JavaScript provides various techniques and libraries that can help in writing efficient queries. Here, we will explore some of these techniques and how they can be implemented.
One of the most widely used libraries for working with databases in JavaScript is Sequelize. Sequelize is an Object-Relational Mapping (ORM) library that supports multiple databases, including MySQL, PostgreSQL, and SQLite. It provides a simple and intuitive way to define database models and perform queries.
Let's consider an example where we have a "users" table in a MySQL database and we want to retrieve all users with a specific age. With Sequelize, we can write the following code snippet:
const { Sequelize, DataTypes } = require('sequelize'); // Create a Sequelize instance and connect to the MySQL database const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); // Define the User model const User = sequelize.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, age: { type: DataTypes.INTEGER, allowNull: false, }, }); // Retrieve all users with age 25 const getUsersByAge = async (age) => { try { const users = await User.findAll({ where: { age: 25, }, }); console.log(users); } catch (error) { console.error(error); } }; getUsersByAge(25);
In this example, we first create a Sequelize instance and connect to the MySQL database. Then, we define the User model with the necessary attributes. Finally, we use the findAll
method to retrieve all users with the specified age.
Sequelize also provides various options for querying, such as sorting, pagination, and filtering based on multiple conditions. These options can be used to further optimize and customize the queries according to specific requirements.
Another way to write efficient queries in JavaScript is by using raw SQL queries. Although ORMs like Sequelize provide a convenient way to interact with databases, raw SQL queries can sometimes offer better performance and flexibility, especially for complex queries.
For example, if we want to retrieve the count of users grouped by age from a PostgreSQL database, we can use the pg
library and write the following code snippet:
const { Pool } = require('pg'); const pool = new Pool({ user: 'username', host: 'localhost', database: 'database', password: 'password', port: 5432, }); const getUsersCountByAge = async () => { try { const query = ` SELECT age, COUNT(*) as count FROM users GROUP BY age `; const result = await pool.query(query); console.log(result.rows); } catch (error) { console.error(error); } }; getUsersCountByAge();
In this example, we create a connection pool using the pg
library and define a function to execute a raw SQL query. The query retrieves the count of users grouped by age from the "users" table.
Using raw SQL queries gives us more control over the query execution and allows us to leverage the full power of the underlying database. However, it also requires proper handling of query parameters to prevent SQL injection attacks.
Related Article: How to Remove Duplicate Values From a JavaScript Array
Efficient Queries with Node.js
Node.js is a popular runtime environment for running JavaScript on the server-side. When it comes to efficient querying with Node.js, many of the techniques and libraries mentioned in the previous section can be applied.
One of the key advantages of using Node.js for database integration is its non-blocking and event-driven nature. This allows for asynchronous execution of queries, which can significantly improve the overall performance of an application.
Let's consider an example where we have a MongoDB database and we want to retrieve all documents from a collection using the official MongoDB Node.js driver:
const { MongoClient } = require('mongodb'); const uri = 'mongodb+srv://:@cluster0.mongodb.net/test?retryWrites=true&w=majority'; const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true }); const getDocuments = async () => { try { await client.connect(); const collection = client.db('test').collection('documents'); const documents = await collection.find().toArray(); console.log(documents); } catch (error) { console.error(error); } finally { await client.close(); } }; getDocuments();
In this example, we create a MongoClient instance and connect to a MongoDB database using the connection string. Then, we retrieve the "documents" collection and use the find
method to retrieve all documents. Finally, we log the retrieved documents to the console.
Node.js also provides various libraries and frameworks for working with databases, such as Knex.js and Sequelize, which were mentioned in the previous section. These libraries offer additional features and abstractions that can simplify database integration and improve query efficiency.
For example, using Knex.js, we can rewrite the previous example with a more expressive and concise syntax:
const knex = require('knex')({ client: 'mysql', connection: { host: 'localhost', user: 'username', password: 'password', database: 'database', }, }); const getDocuments = async () => { try { const documents = await knex('documents').select(); console.log(documents); } catch (error) { console.error(error); } finally { await knex.destroy(); } }; getDocuments();
In this example, we create a Knex.js instance and define the database connection details. Then, we use the select
method to retrieve all documents from the "documents" table. Finally, we log the retrieved documents to the console and destroy the Knex.js instance.
In addition to ORMs and query builders, Node.js also offers the flexibility to execute raw SQL queries or use the native database drivers directly. This allows developers to fine-tune and optimize queries for specific use cases and performance requirements.
Indexing with JavaScript
Indexing plays a crucial role in optimizing database query performance by allowing for faster data retrieval and reducing the need for full table scans. JavaScript provides various techniques and libraries for creating and managing indexes in databases. In this section, we will explore some of these techniques and how they can be implemented.
When working with relational databases like MySQL or PostgreSQL, creating indexes on the appropriate columns can significantly improve query performance. One popular library for managing indexes in JavaScript with relational databases is Sequelize.
Let's consider an example where we have a "users" table in a MySQL database and we want to create an index on the "age" column to optimize queries that involve age-based filtering. With Sequelize, we can define the index as part of the User model definition:
const { Sequelize, DataTypes } = require('sequelize'); const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); const User = sequelize.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, age: { type: DataTypes.INTEGER, allowNull: false, }, }, { indexes: [ { name: 'age_index', fields: ['age'], }, ], });
In this example, we define the User model with the necessary attributes. Additionally, we specify an index called "age_index" on the "age" column using the indexes
option. This index will improve the performance of queries involving the "age" column.
Sequelize also provides options for creating composite indexes on multiple columns, specifying index types (e.g., B-tree, Hash), and customizing index properties like uniqueness and sorting order.
When working with NoSQL databases like MongoDB, indexing is also crucial for optimizing query performance. In MongoDB, indexes can be created on specific fields to speed up queries that involve those fields.
Let's consider an example where we have a MongoDB collection called "users" and we want to create an index on the "age" field. We can use the official MongoDB Node.js driver to create the index as follows:
const { MongoClient } = require('mongodb'); const uri = 'mongodb+srv://:@cluster0.mongodb.net/test?retryWrites=true&w=majority'; const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true }); const createIndex = async () => { try { await client.connect(); const collection = client.db('test').collection('users'); await collection.createIndex({ age: 1 }); console.log('Index created successfully'); } catch (error) { console.error(error); } finally { await client.close(); } }; createIndex();
In this example, we create a MongoClient instance and connect to a MongoDB database using the connection string. Then, we retrieve the "users" collection and use the createIndex
method to create an index on the "age" field with an ascending sort order (1). Finally, we log a success message to the console.
Indexing with Node.js
Indexing is a critical aspect of database management as it significantly improves query performance by allowing for faster data retrieval. In this section, we will explore how indexing can be implemented using Node.js and various database management systems.
When working with relational databases like MySQL or PostgreSQL, creating indexes on the appropriate columns can greatly enhance query performance. Node.js provides several libraries and frameworks that facilitate index management. One such library is again Sequelize.
Let's consider an example where we have a MySQL database with a "users" table, and we want to create an index on the "age" column using Sequelize. The following code snippet demonstrates how to define the index in the Sequelize model:
const { Sequelize, DataTypes } = require('sequelize'); const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); const User = sequelize.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, age: { type: DataTypes.INTEGER, allowNull: false, }, }, { indexes: [ { name: 'age_index', fields: ['age'], }, ], });
In this example, we create a Sequelize instance and connect to the MySQL database. We then define the User model, including the "name" and "age" columns. To create an index on the "age" column, we use the indexes
option and specify the name and fields of the index.
Sequelize also supports creating composite indexes on multiple columns, specifying index types (e.g., B-tree, Hash), and customizing index properties such as uniqueness and sorting order.
When working with NoSQL databases like MongoDB, indexing is equally important for query optimization. Node.js provides native MongoDB drivers and libraries that enable index management.
Consider a scenario where we have a MongoDB collection named "users" and we want to create an index on the "age" field. The following code snippet demonstrates how to create the index using the official MongoDB Node.js driver:
const { MongoClient } = require('mongodb'); const uri = 'mongodb+srv://:@cluster0.mongodb.net/test?retryWrites=true&w=majority'; const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true }); const createIndex = async () => { try { await client.connect(); const collection = client.db('test').collection('users'); await collection.createIndex({ age: 1 }); console.log('Index created successfully'); } catch (error) { console.error(error); } finally { await client.close(); } }; createIndex();
In this example, we create a MongoClient instance and connect to a MongoDB database using the connection string. We then retrieve the "users" collection and use the createIndex
method to create an index on the "age" field with an ascending sort order (1). Finally, we log a success message to the console.
Related Article: JavaScript Prototype Inheritance Explained (with Examples)
SQL Database Management
SQL (Structured Query Language) databases are widely used for storing and managing structured data. Node.js provides several libraries and frameworks for interacting with SQL databases, enabling efficient database management. In this section, we will explore some of these libraries and how they can be utilized.
Let's consider an example where we have a MySQL database and we want to create a "users" table using Sequelize. The following code snippet demonstrates how to define the table and perform basic CRUD (Create, Read, Update, Delete) operations:
const { Sequelize, DataTypes } = require('sequelize'); const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); const User = sequelize.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, email: { type: DataTypes.STRING, allowNull: false, unique: true, validate: { isEmail: true, }, }, }); const createUser = async (name, email) => { try { const user = await User.create({ name, email }); console.log('User created:', user.toJSON()); } catch (error) { console.error('Error creating user:', error); } }; const getUsers = async () => { try { const users = await User.findAll(); console.log('Users:', users.map((user) => user.toJSON())); } catch (error) { console.error('Error retrieving users:', error); } }; const updateUser = async (id, name) => { try { const user = await User.findByPk(id); if (user) { user.name = name; await user.save(); console.log('User updated:', user.toJSON()); } else { console.log('User not found'); } } catch (error) { console.error('Error updating user:', error); } }; const deleteUser = async (id) => { try { const user = await User.findByPk(id); if (user) { await user.destroy(); console.log('User deleted'); } else { console.log('User not found'); } } catch (error) { console.error('Error deleting user:', error); } }; (async () => { try { await sequelize.sync({ force: true }); await createUser('John Doe', 'john@example.com'); await createUser('Jane Smith', 'jane@example.com'); await getUsers(); await updateUser(1, 'John Smith'); await deleteUser(2); } catch (error) { console.error('Error:', error); } finally { await sequelize.close(); } })();
In this example, we create a Sequelize instance and connect to the MySQL database. We then define the User model with the necessary attributes, including data types and validations. We utilize the model to perform CRUD operations such as creating a user, retrieving all users, updating a user, and deleting a user.
Sequelize offers various querying options, including filtering, sorting, pagination, and association handling. It also provides support for database migrations, which simplifies the process of managing database schema changes over time.
Another popular library for SQL database management in Node.js is Knex.js. Knex.js is a query builder that supports multiple SQL databases and provides a fluent and convenient way to construct SQL queries.
Let's consider an example where we have a PostgreSQL database and we want to create a "products" table using Knex.js. The following code snippet demonstrates how to define the table and perform basic CRUD operations:
const knex = require('knex')({ client: 'pg', connection: { host: 'localhost', user: 'username', password: 'password', database: 'database', }, }); const createProduct = async (name, price) => { try { const [productId] = await knex('products').insert({ name, price }).returning('id'); console.log('Product created with ID:', productId); } catch (error) { console.error('Error creating product:', error); } }; const getProducts = async () => { try { const products = await knex('products').select(); console.log('Products:', products); } catch (error) { console.error('Error retrieving products:', error); } }; const updateProduct = async (id, price) => { try { const updatedCount = await knex('products').where({ id }).update({ price }); console.log('Updated', updatedCount, 'product(s)'); } catch (error) { console.error('Error updating product:', error); } }; const deleteProduct = async (id) => { try { const deletedCount = await knex('products').where({ id }).del(); console.log('Deleted', deletedCount, 'product(s)'); } catch (error) { console.error('Error deleting product:', error); } }; (async () => { try { await knex.schema.dropTableIfExists('products'); await knex.schema.createTable('products', (table) => { table.increments('id').primary(); table.string('name').notNullable(); table.decimal('price').notNullable(); }); await createProduct('Product 1', 10.99); await createProduct('Product 2', 19.99); await getProducts(); await updateProduct(1, 14.99); await deleteProduct(2); } catch (error) { console.error('Error:', error); } finally { await knex.destroy(); } })();
In this example, we create a Knex.js instance and define the database connection details. We then use the instance to perform CRUD operations on the "products" table. We utilize the query builder methods provided by Knex.js to construct SQL queries for creating, retrieving, updating, and deleting products.
Knex.js supports various advanced querying capabilities, including joins, aggregates, subqueries, and transactions. It also offers built-in support for database migrations, making it easier to manage schema changes.
NoSQL Database Management
NoSQL (Not Only SQL) databases have gained popularity due to their flexibility, scalability, and ability to handle unstructured and semi-structured data. Node.js provides various libraries and frameworks for managing NoSQL databases, enabling efficient database management. In this section, we will explore some of these libraries and how they can be utilized.
When working with NoSQL databases like MongoDB, Node.js offers the official MongoDB Node.js driver, which provides a native and efficient way to interact with MongoDB databases.
Let's consider an example where we have a MongoDB database and we want to perform basic CRUD (Create, Read, Update, Delete) operations on a collection using the official MongoDB Node.js driver:
const { MongoClient } = require('mongodb'); const uri = 'mongodb+srv://:@cluster0.mongodb.net/test?retryWrites=true&w=majority'; const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true }); const createDocument = async (collectionName, document) => { try { await client.connect(); const collection = client.db('test').collection(collectionName); const result = await collection.insertOne(document); console.log('Document created with ID:', result.insertedId); } catch (error) { console.error('Error creating document:', error); } finally { await client.close(); } }; const getDocuments = async (collectionName) => { try { await client.connect(); const collection = client.db('test').collection(collectionName); const documents = await collection.find().toArray(); console.log('Documents:', documents); } catch (error) { console.error('Error retrieving documents:', error); } finally { await client.close(); } }; const updateDocument = async (collectionName, filter, update) => { try { await client.connect(); const collection = client.db('test').collection(collectionName); const result = await collection.updateOne(filter, update); console.log('Updated', result.modifiedCount, 'document(s)'); } catch (error) { console.error('Error updating document:', error); } finally { await client.close(); } }; const deleteDocument = async (collectionName, filter) => { try { await client.connect(); const collection = client.db('test').collection(collectionName); const result = await collection.deleteOne(filter); console.log('Deleted', result.deletedCount, 'document(s)'); } catch (error) { console.error('Error deleting document:', error); } finally { await client.close(); } }; (async () => { try { await createDocument('users', { name: 'John Doe', age: 25 }); await createDocument('users', { name: 'Jane Smith', age: 30 }); await getDocuments('users'); await updateDocument('users', { name: 'John Doe' }, { $set: { age: 26 } }); await deleteDocument('users', { name: 'Jane Smith' }); } catch (error) { console.error('Error:', error); } finally { await client.close(); } })();
In this example, we create a MongoClient instance and connect to a MongoDB database using the connection string. We then define functions to perform CRUD operations on the "users" collection. We use methods provided by the MongoDB Node.js driver, such as insertOne
, find
, updateOne
, and deleteOne
, to create, retrieve, update, and delete documents in the collection.
The official MongoDB Node.js driver provides various options for querying, filtering, sorting, and aggregating data. It also supports advanced features like transactions, change streams, and geospatial queries.
Another popular library for NoSQL database management in Node.js is Mongoose. Mongoose is an Object Data Modeling (ODM) library that provides a higher-level abstraction over the MongoDB Node.js driver. It offers schema-based modeling, validation, and query building capabilities.
Let's consider an example where we have a MongoDB database and we want to define a schema for the "users" collection using Mongoose. The following code snippet demonstrates how to define the schema and perform basic CRUD operations:
const mongoose = require('mongoose'); mongoose.connect('mongodb://localhost/test', { useNewUrlParser: true, useUnifiedTopology: true }); const userSchema = new mongoose.Schema({ name: { type: String, required: true, }, age: { type: Number, required: true, }, }); const User = mongoose.model('User', userSchema); const createUser = async (name, age) => { try { const user = await User.create({ name, age }); console.log('User created:', user.toJSON()); } catch (error) { console.error('Error creating user:', error); } }; const getUsers = async () => { try { const users = await User.find(); console.log('Users:', users); } catch (error) { console.error('Error retrieving users:', error); } }; const updateUser = async (id, age) => { try { const user = await User.findById(id); if (user) { user.age = age; await user.save(); console.log('User updated:', user.toJSON()); } else { console.log('User not found'); } } catch (error) { console.error('Error updating user:', error); } }; const deleteUser = async (id) => { try { const result = await User.deleteOne({ _id: id }); console.log('Deleted', result.deletedCount, 'user(s)'); } catch (error) { console.error('Error deleting user:', error); } }; (async () => { try { await User.deleteMany(); await createUser('John Doe', 25); await createUser('Jane Smith', 30); await getUsers(); await updateUser('60f081e34e2f3c3368c6b7f9', 26); await deleteUser('60f081e34e2f3c3368c6b7fa'); } catch (error) { console.error('Error:', error); } finally { await mongoose.disconnect(); } })();
In this example, we connect to a local MongoDB database using the connect
method provided by Mongoose. We define a schema for the "users" collection using the Schema
constructor and specify the fields and their types. We then create a User model from the schema using the model
method.
We define functions to perform CRUD operations on the User model. We use methods provided by Mongoose, such as create
, find
, findById
, save
, and deleteOne
, to create, retrieve, update, and delete documents in the collection.
Mongoose also provides features like middleware, population, and validation, which further simplify data modeling and management in MongoDB.
Data Integration Strategies
Data integration is a critical aspect of modern software systems that deal with multiple data sources and heterogeneous data formats. Node.js provides various strategies and libraries for integrating data from different sources and transforming it into a unified format. In this section, we will explore some of these strategies and how they can be implemented.
One common data integration scenario is combining data from multiple SQL databases. Node.js offers libraries like Sequelize and Knex.js, which support multiple SQL databases and provide convenient ways to interact with them.
Let's consider an example where we have two MySQL databases, "db1" and "db2", and we want to retrieve user data from both databases and combine it into a single result. We can use Sequelize to achieve this:
const { Sequelize, DataTypes } = require('sequelize'); const sequelizeDb1 = new Sequelize('db1', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); const sequelizeDb2 = new Sequelize('db2', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); const UserDb1 = sequelizeDb1.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, age: { type: DataTypes.INTEGER, allowNull: false, }, }); const UserDb2 = sequelizeDb2.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, age: { type: DataTypes.INTEGER, allowNull: false, }, }); const getUsersFromMultipleDatabases = async () => { try { await Promise.all([sequelizeDb1.sync(), sequelizeDb2.sync()]); const usersDb1 = await UserDb1.findAll(); const usersDb2 = await UserDb2.findAll(); const combinedUsers = [...usersDb1, ...usersDb2]; console.log('Combined Users:', combinedUsers); } catch (error) { console.error('Error retrieving users:', error); } finally { await Promise.all([sequelizeDb1.close(), sequelizeDb2.close()]); } }; getUsersFromMultipleDatabases();
In this example, we create two Sequelize instances, sequelizeDb1
and sequelizeDb2
, and connect to the "db1" and "db2" MySQL databases, respectively. We then define the User models for each database using the define
method provided by Sequelize.
To retrieve user data from both databases, we use the findAll
method on each User model and combine the results into a single array. Finally, we log the combined users to the console.
Another data integration scenario is combining data from multiple NoSQL databases. Node.js provides libraries like the official MongoDB Node.js driver and Mongoose, which can be used to interact with different NoSQL databases.
Let's consider an example where we have two MongoDB databases, "db1" and "db2", and we want to retrieve user data from both databases and combine it into a single result. We can use the official MongoDB Node.js driver to accomplish this:
const { MongoClient } = require('mongodb'); const uriDb1 = 'mongodb+srv://:@cluster0.mongodb.net/db1?retryWrites=true&w=majority'; const uriDb2 = 'mongodb+srv://:@cluster0.mongodb.net/db2?retryWrites=true&w=majority'; const clientDb1 = new MongoClient(uriDb1, { useNewUrlParser: true, useUnifiedTopology: true }); const clientDb2 = new MongoClient(uriDb2, { useNewUrlParser: true, useUnifiedTopology: true }); const getUsersFromMultipleDatabases = async () => { try { await Promise.all([clientDb1.connect(), clientDb2.connect()]); const collectionDb1 = clientDb1.db('db1').collection('users'); const collectionDb2 = clientDb2.db('db2').collection('users'); const usersDb1 = await collectionDb1.find().toArray(); const usersDb2 = await collectionDb2.find().toArray(); const combinedUsers = [...usersDb1, ...usersDb2]; console.log('Combined Users:', combinedUsers); } catch (error) { console.error('Error retrieving users:', error); } finally { await Promise.all([clientDb1.close(), clientDb2.close()]); } }; getUsersFromMultipleDatabases();
In this example, we create two MongoClient instances, clientDb1
and clientDb2
, and connect to the "db1" and "db2" MongoDB databases, respectively, using the respective connection URIs.
We then retrieve the "users" collections from each database and use the find
method to retrieve all users from each collection. We combine the results into a single array and log the combined users to the console.
Data integration can also involve combining data from both SQL and NoSQL databases. In such cases, a combination of the techniques mentioned earlier can be used.
For example, let's consider a scenario where we have a MySQL database and a MongoDB database, and we want to retrieve user data from both databases and combine it into a single result. We can use Sequelize and the official MongoDB Node.js driver to achieve this:
const { Sequelize, DataTypes } = require('sequelize'); const { MongoClient } = require('mongodb'); const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); const client = new MongoClient('mongodb://localhost:27017', { useNewUrlParser: true, useUnifiedTopology: true, }); const User = sequelize.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, age: { type: DataTypes.INTEGER, allowNull: false, }, }); const getUsersFromMultipleDatabases = async () => { try { await Promise.all([sequelize.sync(), client.connect()]); const usersDb1 = await User.findAll(); const collectionDb2 = client.db('db2').collection('users'); const usersDb2 = await collectionDb2.find().toArray(); const combinedUsers = [...usersDb1, ...usersDb2]; console.log('Combined Users:', combinedUsers); } catch (error) { console.error('Error retrieving users:', error); } finally { await Promise.all([sequelize.close(), client.close()]); } }; getUsersFromMultipleDatabases();
In this example, we create a Sequelize instance and connect to the MySQL database. We also create a MongoClient instance and connect to the MongoDB database.
We define the User model using Sequelize and retrieve user data from the MySQL database using the findAll
method. We retrieve user data from the MongoDB database using the find
method provided by the MongoDB Node.js driver.
We combine the results from both databases into a single array and log the combined users to the console.
Query Optimization Techniques
Query optimization plays a crucial role in improving the performance and efficiency of database queries. Node.js provides several techniques and libraries that can be used to optimize queries and minimize their execution time. In this section, we will explore some of these techniques and how they can be implemented.
One common technique for query optimization is indexing. Indexes are data structures that improve the speed of data retrieval operations on database tables. By creating indexes on frequently queried columns, developers can significantly reduce query execution time.
Let's consider an example where we have a MySQL database with a "users" table, and we want to retrieve all users with a specific age. By creating an index on the "age" column, we can optimize the query execution:
const { Sequelize, DataTypes } = require('sequelize'); const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); const User = sequelize.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, age: { type: DataTypes.INTEGER, allowNull: false, }, }, { indexes: [ { name: 'age_index', fields: ['age'], }, ], }); const getUsersByAge = async (age) => { try { const users = await User.findAll({ where: { age: age, }, }); console.log(users); } catch (error) { console.error(error); } }; getUsersByAge(25);
In this example, we define the User model using Sequelize and create an index on the "age" column using the indexes
option. This index improves the performance of queries that involve age-based filtering.
Another technique for query optimization is query caching. Caching involves storing the results of a query in memory or a separate cache store, such as Redis, to avoid redundant database queries.
Let's consider an example where we have a MongoDB database and we want to retrieve user data from a collection. By implementing query caching using the node-cache
library, we can reduce the number of database queries:
const { MongoClient } = require('mongodb'); const NodeCache = require('node-cache'); const uri = 'mongodb+srv://:@cluster0.mongodb.net/test?retryWrites=true&w=majority'; const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true }); const cache = new NodeCache(); const getUsers = async () => { try { await client.connect(); const collection = client.db('test').collection('users'); // Check if the data is already cached const cachedData = cache.get('users'); if (cachedData) { console.log('Retrieved from cache:', cachedData); } else { // Retrieve data from the database const users = await collection.find().toArray(); console.log('Retrieved from database:', users); // Cache the data for future use cache.set('users', users); } } catch (error) { console.error('Error retrieving users:', error); } finally { await client.close(); } }; getUsers();
In this example, we create a MongoClient instance and connect to a MongoDB database using the connection string. We also create a NodeCache instance for caching the query results.
Before querying the database, we check if the data is already cached using the get
method. If the data is found in the cache, we retrieve it and log a message. Otherwise, we retrieve the data from the database, log it, and cache it using the set
method.
Query optimization can also involve rewriting queries to leverage database-specific optimization features. For example, in PostgreSQL, the EXPLAIN
statement can be used to analyze query execution plans and identify potential performance bottlenecks.
Let's consider an example where we have a PostgreSQL database and we want to retrieve all users with a specific age. By using the EXPLAIN
statement, we can analyze the query execution plan and optimize the query if needed:
const { Pool } = require('pg'); const pool = new Pool({ user: 'username', host: 'localhost', database: 'database', password: 'password', port: 5432, }); const getUsersByAge = async (age) => { try { const query = ` EXPLAIN ANALYZE SELECT * FROM users WHERE age = $1 `; const result = await pool.query(query, [age]); console.log(result.rows[0]); } catch (error) { console.error(error); } }; getUsersByAge(25);
In this example, we create a connection pool using the pg
library and define a function to execute the query. The query includes the EXPLAIN ANALYZE
statement, which provides detailed information about the query execution plan and the time taken by each step.
Related Article: How To Downgrade To A Previous Node Version In Nodejs
Real-time Data Fetching
Real-time data fetching is a critical requirement for many applications that deal with constantly changing data. Node.js provides various strategies and libraries for efficiently fetching real-time data from databases and other data sources. In this section, we will explore some of these strategies and how they can be implemented.
One common strategy for real-time data fetching is using database triggers and change streams. Triggers are database objects that automatically execute a specified action in response to specific events, such as data modifications. Change streams, on the other hand, provide a stream of change events that occur in a database.
Let's consider an example where we have a MySQL database and we want to fetch real-time updates for a specific table. We can use the mysql
library and database triggers to achieve this:
const mysql = require('mysql2'); const connection = mysql.createConnection({ host: 'localhost', user: 'username', password: 'password', database: 'database', }); const createTrigger = async () => { try { await connection.promise().execute(` CREATE TRIGGER user_insert_trigger AFTER INSERT ON users FOR EACH ROW BEGIN SELECT * FROM users WHERE id = NEW.id; END `); console.log('Trigger created successfully'); } catch (error) { console.error('Error creating trigger:', error); } }; const getRealTimeUpdates = () => { connection.on('trigger', (table, action, row) => { console.log('Real-time update:', table, action, row); }); }; (async () => { try { await createTrigger(); getRealTimeUpdates(); } catch (error) { console.error('Error:', error); } })();
In this example, we create a MySQL connection using the mysql2
library and define a function to create a trigger. The trigger is created using the CREATE TRIGGER
statement and specifies the event (AFTER INSERT), the table (users), and the action (SELECT * FROM users).
The getRealTimeUpdates
function sets up an event listener for the 'trigger' event emitted by the connection. Whenever the trigger is executed (i.e., a new row is inserted into the "users" table), the event listener logs the table name, action, and row to the console.
Another strategy for real-time data fetching is utilizing the change streams feature provided by NoSQL databases like MongoDB. Change streams provide a way to listen for changes in a database collection and receive real-time updates.
Let's consider an example where we have a MongoDB database and we want to fetch real-time updates for a specific collection. We can use the official MongoDB Node.js driver and change streams to achieve this:
const { MongoClient } = require('mongodb'); const uri = 'mongodb+srv://:@cluster0.mongodb.net/test?retryWrites=true&w=majority'; const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true }); const getRealTimeUpdates = async () => { try { await client.connect(); const collection = client.db('test').collection('users'); const changeStream = collection.watch(); changeStream.on('change', (change) => { console.log('Real-time update:', change); }); } catch (error) { console.error('Error:', error); } }; getRealTimeUpdates();
In this example, we create a MongoClient instance and connect to a MongoDB database using the connection string. We retrieve the "users" collection and create a change stream using the watch
method provided by the MongoDB Node.js driver.
We set up an event listener for the 'change' event emitted by the change stream. Whenever a change occurs in the collection, the event listener logs the change object to the console.
Real-time data fetching can also involve subscribing to external data sources or using WebSocket-based communication for real-time updates. Node.js provides several libraries and frameworks for implementing real-time data fetching using these strategies, such as Socket.IO, GraphQL subscriptions, and MQTT.js.
Caching Strategies for Databases
Caching is a common technique used to improve the performance and scalability of applications by storing frequently accessed data in memory or a separate cache store. Node.js provides several strategies and libraries for implementing caching with databases. In this section, we will explore some of these strategies and how they can be implemented.
One common caching strategy is storing query results in memory to avoid redundant database queries. Node.js provides various in-memory caching libraries, such as node-cache
, memory-cache
, and lru-cache
, which can be used to implement this strategy.
Let's consider an example where we have a MongoDB database and we want to cache the results of a query using the node-cache
library:
const { MongoClient } = require('mongodb'); const NodeCache = require('node-cache'); const uri = 'mongodb+srv://:@cluster0.mongodb.net/test?retryWrites=true&w=majority'; const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true }); const cache = new NodeCache(); const getUsers = async () => { try { const cachedUsers = cache.get('users'); if (cachedUsers) { console.log('Retrieved from cache:', cachedUsers); } else { await client.connect(); const collection = client.db('test').collection('users'); const users = await collection.find().toArray(); console.log('Retrieved from database:', users); cache.set('users', users); } } catch (error) { console.error('Error retrieving users:', error); } finally { await client.close(); } }; getUsers();
In this example, we create a MongoClient instance and connect to a MongoDB database using the connection string. We also create a NodeCache instance for caching the query results.
Before querying the database, we check if the data is already cached using the get
method. If the data is found in the cache, we retrieve it and log a message. Otherwise, we retrieve the data from the database, log it, and cache it using the set
method.
Another caching strategy is using a separate cache store, such as Redis or Memcached, to store frequently accessed data. Node.js provides libraries like ioredis
, redis
, and memcached
that can be used to implement this strategy.
Let's consider an example where we have a MySQL database and we want to cache query results using Redis as the cache store. We can use the ioredis
library to achieve this:
const mysql = require('mysql2'); const Redis = require('ioredis'); const connection = mysql.createConnection({ host: 'localhost', user: 'username', password: 'password', database: 'database', }); const redis = new Redis(); const getUsers = async () => { try { const cachedUsers = await redis.get('users'); if (cachedUsers) { console.log('Retrieved from cache:', JSON.parse(cachedUsers)); } else { const [users] = await connection.promise().query('SELECT * FROM users'); console.log('Retrieved from database:', users); await redis.set('users', JSON.stringify(users)); } } catch (error) { console.error('Error retrieving users:', error); } }; getUsers();
In this example, we create a MySQL connection using the mysql2
library and define a function to retrieve user data from the database. We also create a Redis instance using the ioredis
library for caching the query results.
Before querying the database, we check if the data is already cached using the get
method provided by Redis. If the data is found in the cache, we retrieve it, parse it, and log a message. Otherwise, we retrieve the data from the database, log it, and cache it using the set
method provided by Redis.
Caching can also involve caching the rendered output of dynamic web pages or API responses. Node.js provides libraries like express
and fastify
that support built-in caching mechanisms, such as HTTP response caching and template-based page caching.
Let's consider an example where we have an Express.js application and we want to cache the rendered output of a dynamic web page using the express-cache-middleware
library:
const express = require('express'); const cacheMiddleware = require('express-cache-middleware'); const app = express(); const cache = new cacheMiddleware(); app.use(cache.route()); app.get('/users', async (req, res) => { try { const users = await getUsersFromDatabase(); res.json(users); } catch (error) { console.error('Error retrieving users:', error); res.status(500).json({ error: 'Internal Server Error' }); } }); app.listen(3000, () => { console.log('Server listening on port 3000'); }); async function getUsersFromDatabase() { // Simulated function to retrieve users from the database return new Promise((resolve, reject) => { setTimeout(() => { const users = [{ name: 'John Doe', age: 25 }, { name: 'Jane Smith', age: 30 }]; resolve(users); }, 1000); }); }
In this example, we create an Express.js application and create a cacheMiddleware instance using the express-cache-middleware
library. We use the route
method provided by the middleware to add caching to specific routes.
We define a route for the "/users" endpoint and implement a function to retrieve user data from the database. The function is wrapped in a Promise with a simulated delay to mimic the database retrieval.
Search Operations with JavaScript
Search operations are a common requirement in many applications, where users need to find specific data based on certain criteria. Node.js provides various techniques and libraries for implementing search operations efficiently. In this section, we will explore some of these techniques and how they can be implemented.
When working with SQL databases, search operations can be performed using SQL queries with appropriate conditions and operators. Node.js provides libraries like Sequelize and Knex.js, which offer useful querying capabilities for SQL databases.
Let's consider an example where we have a MySQL database and we want to search for users with a specific name. We can use Sequelize to achieve this:
const { Sequelize, DataTypes } = require('sequelize'); const sequelize = new Sequelize('database', 'username', 'password', { host: 'localhost', dialect: 'mysql', }); const User = sequelize.define('User', { name: { type: DataTypes.STRING, allowNull: false, }, age: { type: DataTypes.INTEGER, allowNull: false, }, }); const searchUsersByName = async (name) => { try { const users = await User.findAll({ where: { name: { [Sequelize.Op.like]: `%${name}%`, }, }, }); console.log(users); } catch (error) { console.error(error); } }; searchUsersByName('John');
In this example, we define the User model using Sequelize and use the findAll
method to search for users with a name that matches a specific pattern. We use the Sequelize operator Op.like
to perform a case-insensitive partial match on the "name" column.
Sequelize provides various other operators and options for performing complex search operations, such as combining multiple conditions and sorting the results.
Another approach for implementing search operations is using full-text search capabilities provided by certain databases. Full-text search allows for efficient searching of text-based data using indexing techniques.
Let's consider an example where we have a PostgreSQL database and we want to perform a full-text search on a "products" table. We can use the pg
library and the full-text search capabilities of PostgreSQL to achieve this:
const { Pool } = require('pg'); const pool = new Pool({ user: 'username', host: 'localhost', database: 'database', password: 'password', port: 5432, }); const searchProducts = async (query) => { try { const result = await pool.query(` SELECT * FROM products WHERE to_tsvector('english', name) @@ to_tsquery('english', $1) `, [query]); console.log(result.rows); } catch (error) { console.error(error); } }; searchProducts('apple');
In this example, we create a connection pool using the pg
library and define a function to perform a full-text search on the "products" table. We use the to_tsvector
and to_tsquery
functions provided by PostgreSQL to convert the search query and the indexed text into the appropriate format for full-text search.
Full-text search capabilities may vary depending on the database system used. Some databases, like Elasticsearch, provide dedicated search engines that offer more advanced search functionalities, such as relevance scoring and fuzzy matching.
When working with Elasticsearch, Node.js provides libraries like elasticsearch
and elasticsearch.js
that can be used to perform efficient search operations. Elasticsearch supports advanced search features, including full-text search, filtering, aggregation, and relevance scoring.
Let's consider an example where we have an Elasticsearch cluster and we want to perform a full-text search on an "articles" index. We can use the elasticsearch
library to achieve this:
const { Client } = require('@elastic/elasticsearch'); const client = new Client({ node: 'http://localhost:9200' }); const searchArticles = async (query) => { try { const { body } = await client.search({ index: 'articles', body: { query: { match: { title: query, }, }, highlight: { fields: { title: {}, content: {}, }, }, }, }); console.log(body.hits.hits); } catch (error) { console.error(error); } }; searchArticles('Node.js');
In this example, we create an Elasticsearch client using the @elastic/elasticsearch
library and define a function to perform a full-text search on the "articles" index. We use the match
query to search for articles with a title that matches the specified query.
We also include the highlight
option to retrieve highlighted snippets that match the search query in the title and content fields.
Node.js Benefits for Databases
Node.js offers several benefits for integrating with and managing databases. Its asynchronous and event-driven nature, combined with its rich ecosystem of libraries and frameworks, makes it an excellent choice for building efficient and scalable database-driven applications. In this section, we will explore some of the benefits of using Node.js for databases.
One of the key benefits of Node.js for databases is its non-blocking and asynchronous I/O model. Node.js uses an event loop to handle I/O operations, allowing it to handle a large number of concurrent requests efficiently. This is particularly beneficial for database operations, which often involve I/O operations that can be time-consuming.
For example, when performing database queries, Node.js can initiate the query and continue executing other operations while waiting for the query result. This enables high concurrency and efficient resource utilization, resulting in improved performance and scalability.
Node.js also provides a rich ecosystem of libraries and frameworks for integrating with various databases and data stores. These libraries offer abstractions, query builders, and Object-Relational Mapping (ORM) capabilities that simplify database integration and data modeling.
For relational databases, libraries like Sequelize and Knex.js provide useful querying capabilities, transaction support, and database migrations. These libraries enable developers to interact with databases using JavaScript or TypeScript, without the need to write raw SQL queries.
For NoSQL databases, Node.js offers libraries like the official MongoDB Node.js driver and Mongoose. These libraries provide native or high-level abstractions for interacting with NoSQL databases, performing CRUD operations, and managing data models.
In addition to libraries, Node.js also offers built-in support for various database protocols and data formats. For example, the http
and https
modules enable easy integration with RESTful APIs and web services. The fs
module allows for seamless interaction with file-based databases and data formats.
Another benefit of using Node.js for databases is its ability to easily integrate with other systems and technologies. Node.js provides excellent support for networking, allowing developers to build distributed systems and microservices architectures. This enables seamless communication and data exchange between different components of an application.
Node.js also offers robust support for asynchronous messaging patterns, which can be beneficial for building real-time, event-driven systems. Libraries like Socket.IO and MQTT.js enable efficient communication between clients and servers, allowing for real-time updates and notifications.
Furthermore, Node.js can be easily combined with other tools and technologies commonly used in database management and operations. For example, Docker and Kubernetes can be used to containerize and orchestrate database instances, while monitoring and logging tools can be integrated to gain insights into database performance and health.