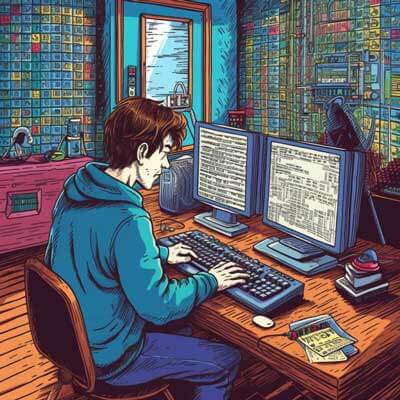
- Defining a ‘Group By’ Function in JavaScript
- Purpose of ‘Group By’ in JavaScript
- Example of Using ‘Group By’ in JavaScript
- Built-In Methods for Grouping Data
- Use Case: Grouping Elements for Aggregation
- Use Case: Grouping Elements for Object Creation
- Best Practice: Handling Empty Arrays with ‘Group By’
- Best Practice: Mapping Grouped Data to Objects
- Performance Consideration: Efficiency of ‘Group By’
- Performance Consideration: Memory Usage with Large Datasets
- Advanced Technique: Nesting ‘Group By’ Functions
Defining a ‘Group By’ Function in JavaScript
To use the ‘Group By’ function in JavaScript, we need to define a key or property by which we want to group our elements. This key can be any valid JavaScript expression that yields a value. The ‘Group By’ function takes two parameters: an array of elements and a callback function that determines the grouping key.
Here’s an example of how to use the ‘Group By’ function in JavaScript:
function groupBy(arr, callback) { return arr.reduce((acc, curr) => { const key = callback(curr); acc[key] = acc[key] || []; acc[key].push(curr); return acc; }, {}); } const numbers = [1, 2, 3, 4, 5]; const groupedNumbers = groupBy(numbers, (num) => num % 2 === 0 ? 'even' : 'odd'); console.log(groupedNumbers);
In this example, we have an array of numbers [1, 2, 3, 4, 5]
. We use the groupBy
function to group these numbers based on whether they are even or odd. The callback function (num) => num % 2 === 0 ? 'even' : 'odd'
determines the grouping key. The resulting groupedNumbers
object will have two properties: 'even'
and 'odd'
, each containing the corresponding numbers.
Related Article: How To Disable & Enable a Submit Button With jQuery
Purpose of ‘Group By’ in JavaScript
The ‘Group By’ function in JavaScript serves several purposes and offers various advantages in data manipulation and analysis. Here are some of the main purposes of using ‘Group By’:
1. Aggregation: Grouping elements allows us to perform aggregation operations on specific subsets of data. We can calculate sums, averages, counts, or other aggregate functions on grouped elements effortlessly.
2. Object Creation: Grouping elements can be used to create new objects or data structures based on specific criteria. By grouping elements, we can transform raw data into a more organized and meaningful format.
3. Data Analysis: Grouping elements helps in analyzing patterns and relationships within the data. It enables us to gain insights into the distribution or characteristics of different groups.
4. Efficiency: Grouping elements reduces the complexity of working with large datasets by organizing them into smaller, manageable groups. It simplifies data manipulation tasks and improves performance.
Overall, the ‘Group By’ function provides a useful tool for organizing, analyzing, and manipulating data effectively in JavaScript.
Example of Using ‘Group By’ in JavaScript
Let’s consider a practical example to illustrate how to use the ‘Group By’ function in JavaScript. Suppose we have an array of objects representing books with properties like title, author, and genre. We want to group these books by their genres to get a better understanding of their distribution.
const books = [ { title: "The Great Gatsby", author: "F. Scott Fitzgerald", genre: "Classic" }, { title: "To Kill a Mockingbird", author: "Harper Lee", genre: "Classic" }, { title: "1984", author: "George Orwell", genre: "Dystopian" }, { title: "The Catcher in the Rye", author: "J.D. Salinger", genre: "Coming-of-age" }, { title: "Pride and Prejudice", author: "Jane Austen", genre: "Classic" }, ]; const groupedBooks = groupBy(books, (book) => book.genre); console.log(groupedBooks);
In this example, we have an array of books with different genres. We use the ‘Group By’ function to group these books by their genres. The callback function (book) => book.genre
specifies that we want to group the books based on their genre
property. The resulting groupedBooks
object will have three properties: 'Classic'
, 'Dystopian'
, and 'Coming-of-age'
, each containing the corresponding books.
This example demonstrates how the ‘Group By’ function can help us organize and analyze data based on specific criteria, in this case, the genre of books.
Built-In Methods for Grouping Data
JavaScript provides built-in methods that can be used for grouping data efficiently. These methods offer a more concise and readable way of achieving similar results to the ‘Group By’ function.
One such method is the reduce()
method, which can be utilized to perform grouping operations by combining elements into groups based on a specified key or property. Here’s an example:
const numbers = [1, 2, 3, 4, 5]; const groupedNumbers = numbers.reduce((acc, curr) => { const key = curr % 2 === 0 ? 'even' : 'odd'; acc[key] = acc[key] || []; acc[key].push(curr); return acc; }, {}); console.log(groupedNumbers);
In this example, we use the reduce()
method to group the numbers array based on whether they are even or odd. The callback function (acc, curr)
is responsible for accumulating the grouped elements into the acc
object. The resulting groupedNumbers
object will have two properties: 'even'
and 'odd'
, each containing the corresponding numbers.
Another built-in method that can be used for grouping data is the map()
method. The map()
method allows us to transform each element of an array into a new value, which can be used as a grouping key. Here’s an example:
const books = [ { title: "The Great Gatsby", author: "F. Scott Fitzgerald", genre: "Classic" }, { title: "To Kill a Mockingbird", author: "Harper Lee", genre: "Classic" }, { title: "1984", author: "George Orwell", genre: "Dystopian" }, { title: "The Catcher in the Rye", author: "J.D. Salinger", genre: "Coming-of-age" }, { title: "Pride and Prejudice", author: "Jane Austen", genre: "Classic" }, ]; const groupedBooks = books.reduce((acc, book) => { const key = book.genre; acc[key] = [...(acc[key] || []), book]; return acc; }, {}); console.log(groupedBooks);
In this example, we use the reduce()
method along with the spread operator (...
) to group the books array by their genres. The callback function (acc, book)
accumulates the grouped books into the acc
object. The resulting groupedBooks
object will have three properties: 'Classic'
, 'Dystopian'
, and 'Coming-of-age'
, each containing the corresponding books.
These built-in methods provide alternative approaches to grouping data in JavaScript, offering flexibility and convenience depending on the specific requirements of your use case.
Related Article: How to Autofill a Textarea Element with VueJS
Use Case: Grouping Elements for Aggregation
One common use case for grouping elements in JavaScript is for aggregation purposes. Aggregation involves combining or summarizing data within specific groups to derive meaningful insights or calculations. By grouping elements based on certain criteria, we can apply aggregate functions like sum, average, count, or maximum/minimum values to each group.
Let’s consider an example where we have an array of sales transactions and we want to calculate the total sales amount for each month. Each transaction object has properties like date and amount. We can use the ‘Group By’ function to group these transactions by month and calculate the total sales amount for each month.
const transactions = [ { date: "2021-01-14", amount: 100 }, { date: "2021-01-22", amount: 150 }, { date: "2021-02-05", amount: 200 }, { date: "2021-02-18", amount: 120 }, { date: "2021-03-10", amount: 180 }, ]; const groupedTransactions = groupBy(transactions, (transaction) => transaction.date.slice(0, 7)); const monthlySales = Object.entries(groupedTransactions).map(([month, transactions]) => ({ month, totalSales: transactions.reduce((acc, curr) => acc + curr.amount, 0), })); console.log(monthlySales);
In this example, we first group the transactions by extracting the year and month from the date
property using the callback function (transaction) => transaction.date.slice(0, 7)
. The resulting groupedTransactions
object will have properties for each month, with each property containing an array of transactions for that month.
Next, we use the Object.entries()
method to iterate over the grouped transactions and calculate the total sales amount for each month. The resulting monthlySales
array of objects contains the month and its corresponding total sales amount.
This use case demonstrates how grouping elements can be utilized to aggregate data based on specific criteria, enabling us to derive meaningful insights or perform calculations efficiently.
Use Case: Grouping Elements for Object Creation
Another common use case for grouping elements in JavaScript is for creating new objects or data structures based on specific criteria. By grouping elements, we can transform raw data into a more organized and meaningful format that suits our needs.
Let’s consider an example where we have an array of products with properties like name, category, and price. We want to create a new object where the categories are grouped together as properties, and each property contains an array of products belonging to that category.
const products = [ { name: "iPhone", category: "Electronics", price: 999 }, { name: "Macbook Pro", category: "Electronics", price: 1999 }, { name: "T-shirt", category: "Clothing", price: 20 }, { name: "Jeans", category: "Clothing", price: 50 }, ]; const groupedProducts = groupBy(products, (product) => product.category); console.log(groupedProducts);
In this example, we group the products by their categories using the ‘Group By’ function. The resulting groupedProducts
object will have properties for each category ('Electronics'
and 'Clothing'
), with each property containing an array of products belonging to that category.
This use case demonstrates how grouping elements can be used to create new objects or data structures, providing a more organized representation of the data based on specific criteria.
Best Practice: Handling Empty Arrays with ‘Group By’
When using the ‘Group By’ function, it’s essential to handle empty arrays or scenarios where no elements match the grouping criteria. Failure to handle these cases correctly can result in unexpected behavior or errors in your code.
One common approach to handling empty arrays is by providing a default value for non-existent groups. By using the logical OR operator (||
), we can specify a default value when initializing the group within the ‘Group By’ function.
function groupBy(arr, callback) { return arr.reduce((acc, curr) => { const key = callback(curr); acc[key] = acc[key] || []; acc[key].push(curr); return acc; }, {}); } const numbers = []; const groupedNumbers = groupBy(numbers, (num) => num % 2 === 0 ? 'even' : 'odd'); console.log(groupedNumbers);
In this example, we have an empty array numbers
. When using the ‘Group By’ function, we ensure that empty groups are initialized as empty arrays by using acc[key] = acc[key] || []
. This ensures that even if no elements match the grouping criteria, an empty array will be created for that group in the resulting object.
Best Practice: Mapping Grouped Data to Objects
When working with grouped data, it’s often necessary to transform the grouped result into a more structured format, such as an array of objects. This allows for easier consumption and manipulation of the data. By mapping the grouped data to objects, we can provide meaningful keys and values that suit our needs.
Let’s consider an example where we have an array of books with properties like title, author, and genre. We want to group these books by genre and transform the grouped result into an array of objects where each object represents a genre and includes the number of books in that genre.
const books = [ { title: "The Great Gatsby", author: "F. Scott Fitzgerald", genre: "Classic" }, { title: "To Kill a Mockingbird", author: "Harper Lee", genre: "Classic" }, { title: "1984", author: "George Orwell", genre: "Dystopian" }, { title: "The Catcher in the Rye", author: "J.D. Salinger", genre: "Coming-of-age" }, { title: "Pride and Prejudice", author: "Jane Austen", genre: "Classic" }, ]; const groupedBooks = groupBy(books, (book) => book.genre); const genres = Object.entries(groupedBooks).map(([genre, books]) => ({ genre, count: books.length, })); console.log(genres);
In this example, we use the ‘Group By’ function to group the books by their genres. We then use the Object.entries()
method to iterate over the grouped result and map it into an array of objects. Each object has properties for genre
and count
, representing the genre name and the number of books in that genre, respectively.
Mapping grouped data to objects allows us to structure the data in a way that is more meaningful for our use case, providing a clear representation of each group with relevant information.
Performance Consideration: Efficiency of ‘Group By’
When working with large datasets or performing complex grouping operations, the efficiency of the ‘Group By’ function becomes crucial. Proper optimization can significantly improve the performance and responsiveness of your code.
One important consideration is to ensure that the callback function used for grouping is efficient. The callback function should be designed to execute quickly and avoid unnecessary computations or operations. Complex calculations or operations within the callback can slow down the grouping process, especially when applied to a large number of elements.
Additionally, using built-in methods like reduce()
or map()
can provide performance benefits over custom implementation when grouping data. These built-in methods are highly optimized and offer better efficiency compared to manually iterating over the elements and performing grouping operations.
It’s also worth noting that JavaScript engines like V8 (used in Node.js and Chrome) have advanced optimizations that can improve the performance of common array operations, including grouping. These optimizations take advantage of techniques like inline caching, hidden classes, and just-in-time compilation.
To ensure optimal performance when using the ‘Group By’ function, consider the following best practices:
1. Optimize the callback function by minimizing unnecessary computations or operations.
2. Utilize built-in methods like reduce()
or map()
for grouping whenever possible.
3. Take advantage of advanced optimizations provided by JavaScript engines by using up-to-date versions of Node.js or modern browsers.
Performance Consideration: Memory Usage with Large Datasets
When working with large datasets, memory usage becomes an important consideration. Grouping elements in JavaScript can potentially consume a significant amount of memory if not optimized properly.
One approach to optimize memory usage is to use streaming or incremental processing techniques instead of loading all data into memory at once. Streaming allows you to process data in smaller chunks, reducing the overall memory footprint. This can be achieved by using techniques like generators or reading data from streams directly.
Another optimization technique is to avoid unnecessary copying or duplication of data during the grouping process. For example, when using the ‘Group By’ function, ensure that you are not creating unnecessary copies of grouped elements or intermediate data structures.
It’s also important to release any unused memory or resources after the grouping operation is completed. Explicitly freeing up memory can help mitigate memory leaks and improve overall performance.
To optimize memory usage when grouping elements in JavaScript, consider the following best practices:
1. Use streaming or incremental processing techniques to reduce memory consumption.
2. Avoid unnecessary copying or duplication of data during grouping.
3. Release unused memory or resources after the grouping operation is completed.
Advanced Technique: Nesting ‘Group By’ Functions
In some scenarios, it may be necessary to perform complex grouping operations by applying multiple levels of grouping criteria. This can be achieved by nesting ‘Group By’ functions, where the result of one grouping operation becomes the input for another.
Let’s consider an example where we have an array of sales transactions with properties like date, category, and amount. We want to group these transactions first by month and then by category to analyze sales performance at a more granular level.
const transactions = [ { date: "2021-01-14", category: "Electronics", amount: 100 }, { date: "2021-01-22", category: "Clothing", amount: 150 }, { date: "2021-02-05", category: "Electronics", amount: 200 }, { date: "2021-02-18", category: "Clothing", amount: 120 }, { date: "2021-03-10", category: "Electronics", amount: 180 }, ]; const groupedTransactions = groupBy(transactions, (transaction) => transaction.date.slice(0, 7)); const nestedGroupedTransactions = Object.entries(groupedTransactions).map(([month, transactions]) => ({ month, categories: groupBy(transactions, (transaction) => transaction.category), })); console.log(nestedGroupedTransactions);
In this example, we first group the transactions by month using the ‘Group By’ function. The resulting groupedTransactions
object will have properties for each month, with each property containing an array of transactions for that month.
Next, we use the Object.entries()
method to iterate over the grouped transactions and apply another level of grouping by category within each month. This is achieved by nesting another ‘Group By’ function within the mapping process. The resulting nestedGroupedTransactions
object contains properties for each month and sub-properties for each category within that month.