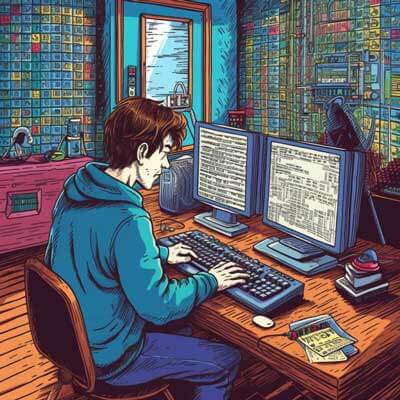
- What is Next.js?
- Is Next.js a backend or frontend framework?
- What is the difference between backend and frontend development?
- How does Next.js handle server-side rendering?
- Can Next.js be used as a standalone backend framework?
- Does Next.js require knowledge of JavaScript?
- Can Next.js work with other frontend frameworks like Angular or Vue?
- What is the role of React in Next.js?
- What is the role of Node.js in Next.js?
- How does Next.js handle API calls?
What is Next.js?
Next.js is a popular framework for building React applications that combines the best of both frontend and backend development. It provides a robust and efficient solution for server-side rendering (SSR) and static site generation (SSG), making it easier to create fast and SEO-friendly websites. With Next.js, developers can build modern web applications that are optimized for performance and user experience.
Related Article: How to Use the forEach Loop with JavaScript
Is Next.js a backend or frontend framework?
Next.js can be considered both a frontend and a backend framework. It allows developers to build the frontend of their applications using React components, while also providing server-side rendering capabilities that are traditionally associated with backend development. This unique combination makes Next.js a useful tool for building full-stack web applications.
What is the difference between backend and frontend development?
Backend development refers to the process of building the server-side logic and infrastructure that powers a web application. It involves working with databases, handling server requests, and implementing business logic. On the other hand, frontend development focuses on building the user interface and client-side functionality of a web application. It involves writing HTML, CSS, and JavaScript code to create an interactive and visually appealing user experience.
How does Next.js handle server-side rendering?
Next.js excels at server-side rendering, which allows the initial rendering of a web page to happen on the server before it is sent to the client. This provides several benefits, including improved performance and SEO optimization. Next.js achieves server-side rendering by pre-rendering pages at build time or on-demand using the getServerSideProps or getStaticProps functions.
Here’s an example of how Next.js handles server-side rendering using the getServerSideProps function:
// pages/index.js import React from 'react'; const HomePage = ({ data }) => { return ( <div> <h1>Welcome to my Next.js website!</h1> <p>{data}</p> </div> ); }; export async function getServerSideProps() { const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data, }, }; } export default HomePage;
In this example, the getServerSideProps function fetches data from an external API and passes it as a prop to the HomePage component. The page is then rendered on the server with the fetched data and sent to the client.
Related Article: How to Use Javascript Substring, Splice, and Slice
Can Next.js be used as a standalone backend framework?
While Next.js provides server-side rendering capabilities, it is primarily designed as a frontend framework. It is not meant to replace traditional backend frameworks like Express or Django, which are better suited for handling complex server logic and database operations. However, Next.js can be used in conjunction with a backend framework to create a full-stack web application. It can handle the frontend rendering and routing, while the backend framework handles the server logic and data management.
Does Next.js require knowledge of JavaScript?
Yes, Next.js is built on top of React and JavaScript, so a strong understanding of JavaScript is necessary to work effectively with Next.js. Knowledge of React is also beneficial, as Next.js uses React components for building the frontend of applications. However, if you are already familiar with React, transitioning to Next.js should be relatively straightforward.
Can Next.js work with other frontend frameworks like Angular or Vue?
Next.js is primarily designed to work with React, and it provides seamless integration with React components and libraries. While it is technically possible to use Next.js with other frontend frameworks like Angular or Vue, it may require additional configuration and customization. The benefits of using Next.js, such as server-side rendering and routing, are optimized for React applications.
Related Article: Conditional Flow in JavaScript: Understand the 'if else' and 'else if' Syntax and More
What is the role of React in Next.js?
React plays a central role in Next.js. Next.js is built on top of React and leverages its component-based architecture to create reusable UI components. React components are used to build the frontend of Next.js applications, and Next.js provides additional features like server-side rendering and routing to enhance the React development experience.
Here’s an example of a simple React component in Next.js:
// components/HelloWorld.js import React from 'react'; const HelloWorld = () => { return <h1>Hello, World!</h1>; }; export default HelloWorld;
In this example, the HelloWorld component renders a simple “Hello, World!” message. This component can be used in any Next.js page to display the greeting.
What is the role of Node.js in Next.js?
Node.js is the runtime environment that powers Next.js. Next.js is built on top of Node.js, which allows it to take advantage of its server-side capabilities. Node.js enables Next.js to handle server-side rendering, API routes, and other backend-related tasks. It provides a useful and efficient runtime for running Next.js applications.
How does Next.js handle API calls?
Next.js provides a built-in API routes feature that allows developers to define serverless API endpoints within their Next.js applications. These API routes can be used to handle HTTP requests and perform server-side logic.
Here’s an example of an API route in Next.js:
// pages/api/hello.js export default function handler(req, res) { res.status(200).json({ message: 'Hello, API!' }); }
In this example, the API route responds with a JSON object containing a simple greeting message. The API route can be accessed at /api/hello
.