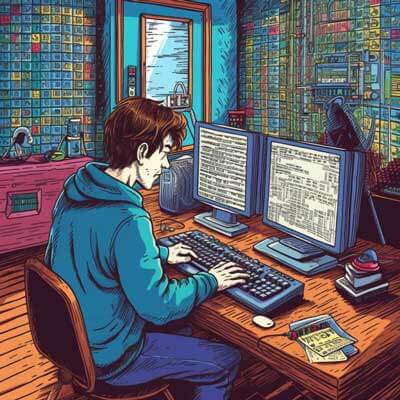
- Query Optimization Techniques
- Understanding Query Execution in PostgreSQL
- Maximizing Query Handling Capacity
- Improving PostgreSQL Query Performance
- Optimizing PostgreSQL Queries to Use Less Resources
- The Role of the Query Planner in PostgreSQL
- Creating Indexes for PostgreSQL Queries
- Setting Timeouts for PostgreSQL Queries
- Exploring Query Parallelism in PostgreSQL
- Analyzing PostgreSQL Query Cache
- Understanding PostgreSQL Query Limit
- Examining PostgreSQL Query Index
- Analyzing PostgreSQL Query Timeout
- Understanding PostgreSQL Query Parallelism
- Optimizing PostgreSQL Queries: Best Practices
- Analyzing PostgreSQL Query Handling Capacity
- Additional Resources
Query Optimization Techniques
When working with PostgreSQL, it is important to optimize your queries to ensure maximum query handling capacity. Query optimization involves making changes to your queries or the database structure to improve performance. Here are some techniques to consider:
1. Use Proper Indexing: Indexes play a crucial role in query performance. By creating indexes on columns used in WHERE clauses, JOIN conditions, and ORDER BY clauses, you can speed up query execution. For example, let’s say we have a table called “users” with columns “id”, “name”, and “email”. To create an index on the “name” column, you can use the following SQL command:
CREATE INDEX idx_users_name ON users(name);
2. Avoid Full Table Scans: Full table scans can be slow and resource-intensive. Instead, try to use indexes to retrieve only the necessary data. For example, if you want to retrieve all users with the name “John”, you can use the following query:
SELECT * FROM users WHERE name = 'John';
3. Minimize Joins: Joins can be expensive operations, especially when dealing with large tables. Try to minimize the number of joins in your queries by denormalizing your data or using subqueries. For example, instead of joining two tables, you can use a subquery to retrieve the necessary data:
SELECT * FROM users WHERE id IN (SELECT user_id FROM orders WHERE total > 100);
4. Use Query Rewriting: PostgreSQL allows you to rewrite queries to optimize their execution. You can use techniques such as query flattening, subquery flattening, and query simplification to achieve better performance. For example, you can rewrite a complex query using CTEs (Common Table Expressions) to improve readability and performance:
WITH products_in_stock AS ( SELECT product_id, SUM(quantity) AS total_quantity FROM inventory GROUP BY product_id HAVING SUM(quantity) > 0 ) SELECT p.name, p.price, s.total_quantity FROM products p JOIN products_in_stock s ON p.id = s.product_id;
5. Analyze Query Plans: PostgreSQL provides the EXPLAIN command, which allows you to analyze the execution plan of a query. By understanding the query plan, you can identify potential performance bottlenecks and optimize your queries accordingly. For example, you can run the following command to analyze the query plan of a SELECT query:
EXPLAIN SELECT * FROM users WHERE name = 'John';
These are just a few query optimization techniques you can use to improve the performance of your PostgreSQL queries. Remember to measure the impact of your optimizations and continuously monitor and fine-tune your queries for optimal performance.
Related Article: Detecting Optimization Issues in PostgreSQL Query Plans
Understanding Query Execution in PostgreSQL
To understand how PostgreSQL handles queries, it’s important to have a basic understanding of its query execution process. Here is a high-level overview of the steps involved in executing a query in PostgreSQL:
1. Parsing: When a query is received by PostgreSQL, it first goes through the parsing phase. During this phase, the query is analyzed to ensure its syntax is correct and to create an internal representation of the query, known as the parse tree.
2. Planning: Once the query is parsed, PostgreSQL moves on to the planning phase. In this phase, the query planner analyzes the parse tree and generates a query plan. The query plan is a detailed set of instructions on how to execute the query, including which tables to access, which indexes to use, and the order of operations.
3. Optimization: After the query plan is generated, PostgreSQL performs query optimization to improve the plan’s efficiency. This involves considering various factors, such as available indexes, statistics about the data, and the configuration settings of the database. The optimizer’s goal is to find the most efficient way to execute the query.
4. Execution: Once the query plan is optimized, PostgreSQL executes the plan and retrieves the requested data. During the execution phase, the database engine performs various operations, such as accessing disk blocks, applying filters, joining tables, and aggregating data.
5. Result Retrieval: Finally, PostgreSQL retrieves the results of the query and returns them to the client.
Understanding the query execution process in PostgreSQL can help you identify potential performance bottlenecks and optimize your queries accordingly. By analyzing the query plan and considering factors such as indexes, statistics, and configuration settings, you can improve the efficiency of your queries and maximize PostgreSQL’s query handling capacity.
Maximizing Query Handling Capacity
To maximize the query handling capacity of PostgreSQL, it is important to consider various factors that can affect performance. Here are some key considerations:
1. Hardware Resources: PostgreSQL’s query handling capacity is influenced by the hardware resources available to the database server. Factors such as CPU speed, memory size, disk I/O performance, and network bandwidth can impact the server’s ability to handle concurrent queries. To maximize query handling capacity, ensure that your server has sufficient resources to handle the expected workload.
2. Configuration Settings: PostgreSQL provides various configuration settings that can be tuned to optimize query performance. These settings control parameters such as memory allocation, parallelism, disk buffers, and query timeouts. By optimizing these settings based on your specific workload, you can improve the query handling capacity of PostgreSQL.
3. Query Optimization: As discussed earlier, optimizing your queries is crucial for maximizing query handling capacity. By following query optimization techniques, such as proper indexing, minimizing joins, and rewriting queries, you can improve the efficiency of your queries and reduce the overall load on the database server.
4. Connection Pooling: Connection pooling allows multiple client applications to share a pool of database connections, reducing the overhead of establishing new connections for each query. By using connection pooling, you can increase the number of concurrent queries that PostgreSQL can handle.
5. Load Balancing: If you have a high volume of queries, distributing the workload across multiple PostgreSQL servers using load balancing can help maximize query handling capacity. Load balancing ensures that queries are evenly distributed among servers, preventing any single server from becoming a bottleneck.
Improving PostgreSQL Query Performance
Improving the performance of PostgreSQL queries is essential for maximizing query handling capacity. Here are some techniques to improve PostgreSQL query performance:
1. Use Appropriate Indexes: Indexes play a crucial role in query performance. Analyze your queries and identify the columns used in WHERE clauses, JOIN conditions, and ORDER BY clauses. Create indexes on these columns to speed up query execution. However, keep in mind that adding too many indexes can negatively impact write performance, so strike a balance between read and write performance.
Example: Let’s say we have a table called “users” with columns “id”, “name”, and “email”. To create an index on the “name” column, you can use the following SQL command:
CREATE INDEX idx_users_name ON users(name);
2. Optimize Query Execution Plans: PostgreSQL’s query planner generates query execution plans based on available statistics and configuration settings. Analyze the query plans generated for your queries and look for opportunities to optimize them. You can use the EXPLAIN command to analyze query plans and identify potential performance bottlenecks.
Example: To analyze the query plan of a SELECT query, you can use the following SQL command:
EXPLAIN SELECT * FROM users WHERE name = 'John';
3. Denormalize Data: Normalized data models are essential for data integrity, but they can sometimes lead to complex queries and performance issues. Consider denormalizing your data by duplicating certain columns or aggregating related data into a single table. This can simplify queries and improve performance, especially for complex JOIN operations.
4. Use Query Rewriting: PostgreSQL allows you to rewrite queries to optimize their execution. Techniques such as query flattening, subquery flattening, and query simplification can improve performance.
Example: You can rewrite a complex query using CTEs (Common Table Expressions) to improve readability and performance.
WITH products_in_stock AS ( SELECT product_id, SUM(quantity) AS total_quantity FROM inventory GROUP BY product_id HAVING SUM(quantity) > 0 ) SELECT p.name, p.price, s.total_quantity FROM products p JOIN products_in_stock s ON p.id = s.product_id;
5. Tune Configuration Settings: PostgreSQL provides various configuration settings that can be tuned to optimize query performance. Parameters such as shared_buffers, work_mem, effective_cache_size, and max_connections can significantly impact performance. Experiment with different settings based on your workload and hardware resources to find the optimal configuration for your system.
These are just a few techniques to improve PostgreSQL query performance. It’s important to analyze your specific workload, monitor query performance, and continuously optimize your queries for maximum query handling capacity.
Related Article: Examining Query Execution Speed on Dates in PostgreSQL
Optimizing PostgreSQL Queries to Use Less Resources
Optimizing PostgreSQL queries to use fewer resources can help improve query handling capacity and overall performance. Here are some techniques to consider:
1. Limit Result Set Size: When querying large tables, it’s important to limit the number of rows returned to reduce resource usage. Use the LIMIT clause to retrieve only the necessary rows. Additionally, consider using pagination techniques, such as OFFSET and FETCH, to retrieve data in smaller chunks.
Example: To retrieve the first 10 rows from a table, you can use the following query:
SELECT * FROM users LIMIT 10;
2. Use Filter Conditions: Use filter conditions in your queries to reduce the amount of data processed. By applying filters early in the query execution process, you can minimize resource usage.
Example: Instead of retrieving all users and then filtering by name, you can apply the filter condition directly in the query:
SELECT * FROM users WHERE name = 'John';
3. Avoid Unnecessary Joins: Joins can be resource-intensive operations, especially when dealing with large tables. Avoid unnecessary joins by carefully analyzing your queries and eliminating unnecessary tables or conditions.
Example: If you only need data from a single table, avoid unnecessary joins:
SELECT * FROM users;
4. Use Aggregation Functions: When performing calculations or aggregating data, use built-in aggregation functions such as SUM, AVG, MIN, MAX, and COUNT. These functions are optimized for performance and can reduce resource usage compared to manual calculations.
Example: Instead of retrieving all rows and calculating the total manually, you can use the SUM function:
SELECT SUM(quantity) FROM orders;
5. Optimize Index Usage: Indexes can improve query performance, but they also consume resources. Avoid using excessive indexes and ensure that indexes are properly designed and selective. Consider creating multi-column indexes to cover multiple conditions in a single index.
Example: If you frequently query the “name” and “email” columns together, you can create a multi-column index:
CREATE INDEX idx_users_name_email ON users(name, email);
The Role of the Query Planner in PostgreSQL
The query planner is a crucial component of the PostgreSQL database system that plays a key role in query optimization and execution. The query planner is responsible for generating the most efficient query execution plan based on the available statistics, indexes, and configuration settings. Here are some key aspects of the query planner in PostgreSQL:
1. Cost-Based Optimization: PostgreSQL’s query planner uses a cost-based optimization approach to evaluate different query execution plans and choose the most efficient one. The planner assigns costs to various operations, such as table scans, index scans, and joins, based on statistics and estimates. It then compares the costs of different plans and selects the one with the lowest cost.
2. Query Rewriting: The query planner can rewrite queries to optimize their execution. It performs various transformations, such as query flattening, subquery flattening, and query simplification, to improve performance. The planner considers factors such as available indexes, statistics about the data, and the configuration settings of the database to determine the most efficient plan.
3. Statistics Collection: The query planner relies on accurate statistics about the data in order to make informed decisions. PostgreSQL collects statistics about table sizes, column distributions, and index selectivity to estimate the cost of different query plans. It uses these statistics to determine the most efficient access methods, join strategies, and sorting algorithms.
4. Index Selection: The query planner analyzes the query and the available indexes to determine the most efficient index to use. It considers factors such as selectivity, uniqueness, and the cost of index access to make informed decisions. The planner can also combine multiple indexes using bitmap indexes or index-only scans to further optimize query performance.
5. Parallel Query Execution: PostgreSQL supports parallel query execution, where multiple processes work together to execute a single query. The query planner determines when and how to parallelize a query based on factors such as the available hardware resources, query complexity, and configuration settings. Parallel query execution can significantly improve performance for queries that can be divided into smaller tasks.
Creating Indexes for PostgreSQL Queries
Creating indexes is an essential aspect of optimizing PostgreSQL queries. Indexes allow the database to quickly locate and retrieve data based on specific columns, improving query performance. Here are some key considerations when creating indexes for PostgreSQL queries:
1. Identify Columns for Indexing: Analyze your queries and identify the columns used in WHERE clauses, JOIN conditions, and ORDER BY clauses. These columns are good candidates for indexing, as they are frequently used for filtering, joining, or sorting data.
Example: Let’s say we have a table called “users” with columns “id”, “name”, and “email”. If you frequently query the “name” column, it is a good candidate for indexing.
2. Choose the Right Index Type: PostgreSQL supports different types of indexes, including B-tree, hash, GiST, SP-GiST, GIN, and BRIN. Each index type has its own strengths and weaknesses, depending on the nature of the data and the query patterns. Consider the characteristics of your data and the specific requirements of your queries when choosing the index type.
Example: For most cases, the B-tree index is a good choice, as it provides efficient access for equality and range queries.
3. Create Single-Column Indexes: Single-column indexes are the simplest form of indexes and are suitable for queries that filter or sort based on a single column. Create single-column indexes on the columns used in WHERE clauses, JOIN conditions, and ORDER BY clauses.
Example: To create an index on the “name” column of the “users” table, you can use the following SQL command:
CREATE INDEX idx_users_name ON users(name);
4. Create Multi-Column Indexes: Multi-column indexes can improve query performance for queries that involve multiple columns. They allow the database to efficiently filter and sort data based on multiple conditions.
Example: If you frequently query the “name” and “email” columns together, you can create a multi-column index:
CREATE INDEX idx_users_name_email ON users(name, email);
5. Consider Partial Indexes: Partial indexes are indexes that only cover a subset of the table rows, based on a specified condition. They can be useful for queries that only access a specific subset of data.
Example: To create a partial index on the “users” table for rows where the “active” column is true, you can use the following SQL command:
CREATE INDEX idx_users_active ON users(name) WHERE active = true;
6. Regularly Update Indexes: Indexes should be updated whenever there are changes to the underlying data. This includes inserts, updates, and deletes. PostgreSQL provides the autovacuum feature, which automatically updates indexes and reclaims disk space. However, it’s important to monitor and tune autovacuum settings to ensure optimal index maintenance.
Creating appropriate indexes for your PostgreSQL queries is essential for improving query performance. By identifying the columns for indexing, choosing the right index type, and regularly updating indexes, you can maximize query handling capacity and optimize query execution.
Related Article: Evaluating Active Connections to a PostgreSQL Query
Setting Timeouts for PostgreSQL Queries
Setting timeouts for PostgreSQL queries can help manage query execution and prevent long-running queries from impacting the overall performance of the system. By setting query timeouts, you can limit the amount of time a query can run and ensure that resources are not tied up indefinitely. Here are some ways to set timeouts for PostgreSQL queries:
1. SET statement_timeout: The SET statement_timeout command allows you to set a timeout for the current session. This timeout value applies to all subsequent queries executed in the session, unless overridden.
Example: To set a query timeout of 5 seconds for the current session, you can use the following SQL command:
SET statement_timeout = '5s';
2. SET LOCAL statement_timeout: The SET LOCAL statement_timeout command allows you to set a timeout for the current transaction block. This timeout value applies only to queries executed within the transaction block and does not affect queries executed outside the block.
Example: To set a query timeout of 10 seconds for the current transaction block, you can use the following SQL command:
SET LOCAL statement_timeout = '10s';
3. ALTER ROLE: You can set a default statement_timeout value for a specific PostgreSQL user or role using the ALTER ROLE command. This timeout value will be applied to all queries executed by the user or role, unless overridden.
Example: To set a default query timeout of 15 seconds for a user named “myuser”, you can use the following SQL command:
ALTER ROLE myuser SET statement_timeout = '15s';
4. Configuring the PostgreSQL Server: You can set a default statement_timeout value for the entire PostgreSQL server by modifying the configuration file (postgresql.conf). Locate the “statement_timeout” parameter and set it to the desired timeout value.
Example (postgresql.conf):
statement_timeout = 30s
After modifying the configuration file, you need to restart the PostgreSQL server for the changes to take effect.
Setting timeouts for PostgreSQL queries can help prevent long-running queries from impacting the overall performance of the system. By using the SET statement_timeout or SET LOCAL statement_timeout commands, or by configuring the PostgreSQL server, you can manage query execution and ensure that resources are not tied up indefinitely.
Exploring Query Parallelism in PostgreSQL
Query parallelism in PostgreSQL allows multiple processes to work together to execute a single query, improving query performance and reducing query execution time. By dividing the work across multiple worker processes, parallel query execution can leverage the available hardware resources to process data more efficiently. Here are some key aspects of query parallelism in PostgreSQL:
1. Parallel Workers: PostgreSQL uses parallel workers to execute a query in parallel. These workers are additional processes that perform the actual work of executing the query. The number of parallel workers used for a query is determined by the configuration settings and the available hardware resources.
2. Parallel Aware Operators: PostgreSQL supports various parallel-aware operators that can be used in parallel query execution. These operators are designed to work efficiently in a parallel environment and can significantly improve performance for parallel queries. Examples of parallel-aware operators include parallel table scans, parallel hash joins, and parallel aggregations.
3. Configuration Settings: PostgreSQL provides various configuration settings that control parallel query execution. These settings include max_parallel_workers, max_parallel_workers_per_gather, and max_parallel_degree. By tuning these settings based on your hardware resources and workload, you can optimize parallel query execution.
4. Query Planning: The query planner in PostgreSQL determines when and how to parallelize a query based on various factors, such as the available hardware resources, query complexity, and configuration settings. The planner considers the cost of parallel execution compared to the cost of sequential execution and decides whether parallel execution is beneficial.
5. Monitoring and Tuning: Monitoring and tuning the performance of parallel queries is important to ensure optimal query handling capacity. You can use tools such as the pg_stat_progress_create_index view and the pg_stat_progress_analyze view to monitor the progress of parallel queries. Additionally, you can analyze the query plans and adjust the configuration settings to optimize parallel query execution.
Analyzing PostgreSQL Query Cache
PostgreSQL does not have a built-in query cache like some other database systems. Instead, it relies on its efficient query planning and execution process to provide fast query performance. However, PostgreSQL does have some caching mechanisms that can affect query performance. Here are some aspects of the PostgreSQL query cache:
1. Shared Buffer Cache: PostgreSQL uses a shared buffer cache to cache frequently accessed data pages in memory. This cache helps reduce disk I/O and improve query performance by keeping frequently accessed data in memory. The size of the shared buffer cache is controlled by the shared_buffers configuration parameter.
2. Disk Block Cache: PostgreSQL caches disk blocks in memory to reduce disk I/O. This cache is separate from the shared buffer cache and is controlled by the effective_cache_size configuration parameter. The disk block cache helps improve query performance by reducing the need for disk reads.
3. Execution Plan Cache: PostgreSQL caches query execution plans to avoid the overhead of repeated query planning. When a query is executed, PostgreSQL checks if the query has been executed before and if a cached execution plan exists. If a cached plan exists, it is reused, saving the time and resources required for planning the query again. The execution plan cache is managed by the PostgreSQL backend process and is limited by the configuration parameter plan_cache_mode.
4. Query Result Cache: PostgreSQL does not have a built-in query result cache. However, you can implement caching at the application level using tools such as Redis or Memcached. These tools allow you to cache query results and avoid executing the same query multiple times. By caching query results, you can further improve query performance and reduce the load on the database server.
When analyzing the performance of PostgreSQL queries, it’s important to consider the caching mechanisms that can impact query performance. By optimizing the shared buffer cache and disk block cache settings, monitoring the execution plan cache, and implementing application-level query result caching, you can improve query performance and maximize query handling capacity.
Related Article: Identifying the Query Holding the Lock in Postgres
Understanding PostgreSQL Query Limit
The LIMIT clause in PostgreSQL allows you to limit the number of rows returned by a query. The LIMIT clause is typically used in combination with the ORDER BY clause to retrieve a specific subset of rows based on a specified sorting order. Here are some key aspects of the LIMIT clause in PostgreSQL:
1. Syntax: The LIMIT clause is used at the end of a SELECT query and is followed by an integer value that specifies the maximum number of rows to be returned. The syntax is as follows:
SELECT column1, column2, ... FROM table ORDER BY column LIMIT n;
2. Retrieving a Subset of Rows: The LIMIT clause allows you to retrieve a specific subset of rows from a table. By specifying the maximum number of rows to be returned, you can control the size of the result set.
Example: To retrieve the first 10 rows from a table, you can use the following query:
SELECT * FROM users LIMIT 10;
3. Combining with OFFSET: The LIMIT clause can be combined with the OFFSET clause to retrieve a specific range of rows. The OFFSET clause specifies the number of rows to skip before starting to return rows. By combining the LIMIT and OFFSET clauses, you can implement pagination and retrieve data in smaller chunks.
Example: To retrieve rows 11-20 from a table, you can use the following query:
SELECT * FROM users OFFSET 10 LIMIT 10;
4. Using with ORDER BY: The LIMIT clause is commonly used in combination with the ORDER BY clause to retrieve a specific subset of rows based on a specified sorting order. By sorting the rows and applying the LIMIT clause, you can retrieve the top or bottom rows based on the sorting criteria.
Example: To retrieve the top 5 users with the highest scores, you can use the following query:
SELECT * FROM users ORDER BY score DESC LIMIT 5;
The LIMIT clause in PostgreSQL is a useful tool for controlling the number of rows returned by a query. By combining the LIMIT clause with other clauses such as ORDER BY and OFFSET, you can implement pagination, retrieve specific subsets of rows, and optimize query performance.
Examining PostgreSQL Query Index
Indexes play a crucial role in optimizing query performance in PostgreSQL. By creating appropriate indexes on columns used in WHERE clauses, JOIN conditions, and ORDER BY clauses, you can speed up query execution and improve overall performance. Here are some key aspects of examining query indexes in PostgreSQL:
1. Index Types: PostgreSQL supports various index types, including B-tree, hash, GiST, SP-GiST, GIN, and BRIN. Each index type has its own strengths and weaknesses, depending on the nature of the data and the query patterns. When examining query indexes, consider the index type and its suitability for the specific query.
2. Index Structure: The structure of an index determines how the data is organized and stored. For example, B-tree indexes store data in a balanced tree structure, while hash indexes use a hash table. Understanding the structure of an index can help you analyze its performance characteristics and make informed decisions when creating or modifying indexes.
3. Index Selectivity: Index selectivity refers to the uniqueness of values in an index column. A highly selective index has a large number of unique values, while a non-selective index has a small number of unique values. Highly selective indexes are more effective in reducing the number of rows that need to be accessed during query execution.
4. Index Usage: When examining query indexes, it’s important to analyze their usage in query execution plans. PostgreSQL provides the EXPLAIN command, which allows you to analyze the query plan of a SELECT query and understand how indexes are used. By analyzing the query plan, you can identify potential performance bottlenecks and optimize your indexes accordingly.
Example: To analyze the query plan of a SELECT query, you can use the following SQL command:
EXPLAIN SELECT * FROM users WHERE name = 'John';
5. Index Maintenance: Indexes require regular maintenance to ensure optimal performance. PostgreSQL provides the autovacuum feature, which automatically updates indexes and reclaims disk space. It’s important to monitor and tune autovacuum settings to ensure that index maintenance is performed efficiently.
When examining query indexes in PostgreSQL, consider the index type, structure, selectivity, usage, and maintenance requirements. By understanding these aspects, you can create appropriate indexes, analyze query performance, and optimize query handling capacity.
Analyzing PostgreSQL Query Timeout
Query timeouts in PostgreSQL allow you to control the maximum execution time of a query. By setting a query timeout, you can prevent long-running queries from impacting the overall performance of the system and ensure that resources are not tied up indefinitely. Here are some aspects of analyzing query timeouts in PostgreSQL:
1. Setting Query Timeouts: Query timeouts can be set using the statement_timeout configuration parameter or by using the SET statement_timeout or SET LOCAL statement_timeout commands. The timeout value is specified as an interval, such as ‘5s’ for 5 seconds or ‘1h30m’ for 1 hour and 30 minutes.
Example: To set a query timeout of 10 seconds for the current session, you can use the following SQL command:
SET statement_timeout = '10s';
2. Handling Query Timeout Errors: When a query exceeds the specified timeout value, PostgreSQL raises an error and terminates the query. The error message includes information about the query, the timeout value, and the time at which the query was canceled. It’s important to handle these errors gracefully in your application code.
3. Query Cancellation: When a query is canceled due to a timeout, PostgreSQL tries to cancel the query as quickly as possible. However, depending on the query’s progress, it may take some time for the query to be fully canceled. It’s important to be aware of this behavior and consider the potential impact on other queries and system performance.
4. Monitoring Query Execution: PostgreSQL provides various system views and functions that allow you to monitor query execution and analyze query timeouts. For example, the pg_stat_activity view provides information about currently executing queries, including the start time and query duration. By monitoring query execution, you can identify long-running queries and take appropriate actions.
Example: To view currently executing queries and their durations, you can use the following SQL command:
SELECT query, now() - query_start AS duration FROM pg_stat_activity WHERE state = 'active';
Analyzing query timeouts in PostgreSQL is important to ensure optimal query handling capacity and prevent long-running queries from impacting system performance. By setting query timeouts, handling timeout errors, monitoring query execution, and taking appropriate actions, you can manage query execution and resource usage effectively.
Related Article: Determining if Your PostgreSQL Query Utilizes an Index
Understanding PostgreSQL Query Parallelism
Parallelism in PostgreSQL allows multiple processes to work together to execute a single query, improving query performance and reducing query execution time. By dividing the work across multiple parallel workers, PostgreSQL can leverage the available hardware resources to process data more efficiently. Here are some key aspects of query parallelism in PostgreSQL:
1. Parallel Workers: PostgreSQL uses parallel workers to execute a query in parallel. These workers are additional processes that perform the actual work of executing the query. The number of parallel workers used for a query is determined by the configuration settings and the available hardware resources.
2. Parallel Aware Operators: PostgreSQL supports various parallel-aware operators that can be used in parallel query execution. These operators are designed to work efficiently in a parallel environment and can significantly improve performance for parallel queries. Examples of parallel-aware operators include parallel table scans, parallel hash joins, and parallel aggregations.
3. Configuration Settings: PostgreSQL provides various configuration settings that control parallel query execution. These settings include max_parallel_workers, max_parallel_workers_per_gather, and max_parallel_degree. By tuning these settings based on your hardware resources and workload, you can optimize parallel query execution.
4. Query Planning: The query planner in PostgreSQL determines when and how to parallelize a query based on various factors, such as the available hardware resources, query complexity, and configuration settings. The planner considers the cost of parallel execution compared to the cost of sequential execution and decides whether parallel execution is beneficial.
5. Monitoring and Tuning: Monitoring and tuning the performance of parallel queries is important to ensure optimal query handling capacity. You can use tools such as the pg_stat_progress_create_index view and the pg_stat_progress_analyze view to monitor the progress of parallel queries. Additionally, you can analyze the query plans and adjust the configuration settings to optimize parallel query execution.
Optimizing PostgreSQL Queries: Best Practices
Optimizing PostgreSQL queries is essential for maximizing query handling capacity and improving overall performance. Here are some best practices to consider when optimizing PostgreSQL queries:
1. Use Proper Indexing: Indexes play a crucial role in query performance. Analyze your queries and identify the columns used in WHERE clauses, JOIN conditions, and ORDER BY clauses. Create indexes on these columns to speed up query execution. However, avoid creating excessive indexes, as they can negatively impact write performance.
2. Minimize Joins: Joins can be expensive operations, especially when dealing with large tables. Minimize the number of joins in your queries by denormalizing your data or using subqueries. Consider using techniques such as query flattening and subquery flattening to optimize join operations.
3. Avoid Full Table Scans: Full table scans can be slow and resource-intensive. Instead of scanning the entire table, use indexes to retrieve only the necessary data. Analyze your queries and identify opportunities to use indexes effectively.
4. Optimize Query Execution Plans: PostgreSQL’s query planner generates query execution plans based on available statistics and configuration settings. Analyze the query plans generated for your queries and look for opportunities to optimize them. Use the EXPLAIN command to analyze query plans and identify potential performance bottlenecks.
5. Monitor and Tune Configuration Settings: PostgreSQL provides various configuration settings that control query performance. Regularly monitor and tune these settings based on your workload and hardware resources. Parameters such as shared_buffers, work_mem, effective_cache_size, and max_connections can significantly impact performance.
6. Use Query Rewriting: PostgreSQL allows you to rewrite queries to optimize their execution. Use techniques such as query flattening, subquery flattening, and query simplification to improve performance. Consider rewriting complex queries using CTEs (Common Table Expressions) for better readability and performance.
7. Analyze and Optimize Query Statistics: PostgreSQL collects statistics about table sizes, column distributions, and index selectivity to estimate the cost of different query plans. Regularly analyze these statistics and update them as necessary. Use the ANALYZE command to update statistics for a table.
8. Implement Connection Pooling: Connection pooling allows multiple client applications to share a pool of database connections, reducing the overhead of establishing new connections for each query. Use connection pooling to increase the number of concurrent queries that PostgreSQL can handle.
9. Implement Query Result Caching: Implement application-level query result caching using tools such as Redis or Memcached. Caching query results can significantly improve query performance and reduce the load on the database server. Use caching selectively for queries that have a high cost of execution.
10. Regularly Monitor Query Performance: Regularly monitor the performance of your queries using tools such as the pg_stat_statements extension or third-party monitoring tools. Analyze query execution times, identify slow-running queries, and optimize them based on their impact on the overall system performance.
Analyzing PostgreSQL Query Handling Capacity
Analyzing the query handling capacity of PostgreSQL is crucial for ensuring optimal performance and scalability. By understanding the limitations and capabilities of PostgreSQL, you can make informed decisions about hardware resources, query optimization, and workload management. Here are some key aspects to consider when analyzing PostgreSQL query handling capacity:
1. Hardware Resources: The query handling capacity of PostgreSQL is influenced by the hardware resources available to the database server. Factors such as CPU speed, memory size, disk I/O performance, and network bandwidth can impact the server’s ability to handle concurrent queries. Ensure that your server has sufficient resources to handle the expected workload.
2. Configuration Settings: PostgreSQL provides various configuration settings that can be tuned to optimize query performance and handling capacity. Parameters such as max_connections, shared_buffers, work_mem, and maintenance_work_mem can significantly impact query handling capacity. Regularly monitor and adjust these settings based on your workload and hardware resources.
3. Query Optimization: Optimizing your queries is essential for maximizing query handling capacity. Use proper indexing, minimize joins, and rewrite complex queries to improve performance. Regularly analyze query execution plans, monitor query performance, and fine-tune your queries for optimal performance.
4. Connection Pooling: Connection pooling allows multiple client applications to share a pool of database connections, reducing the overhead of establishing new connections for each query. Use connection pooling to increase the number of concurrent queries that PostgreSQL can handle.
5. Load Balancing: If you have a high volume of queries, consider distributing the workload across multiple PostgreSQL servers using load balancing. Load balancing ensures that queries are evenly distributed among servers, preventing any single server from becoming a bottleneck.
6. Monitoring and Alerting: Regularly monitor the performance of your PostgreSQL server using tools such as pg_stat_activity, pg_stat_bgwriter, and pg_stat_database. Set up alerts to notify you of any performance issues or resource bottlenecks. Analyze query execution times, query plans, and system metrics to identify potential performance bottlenecks.
7. Benchmarking and Stress Testing: Benchmarking and stress testing can help you understand the maximum query handling capacity of your PostgreSQL server. Use tools such as pgbench or third-party load testing tools to simulate realistic workloads and measure the server’s performance under different scenarios. Identify any performance bottlenecks and adjust your configuration settings or query optimization strategies accordingly.
Related Article: Determining the Status of a Running Query in PostgreSQL
Additional Resources
– PostgreSQL – Official Website
– PostgreSQL – Wikipedia
– PostgreSQL Tutorial – Tutorialspoint