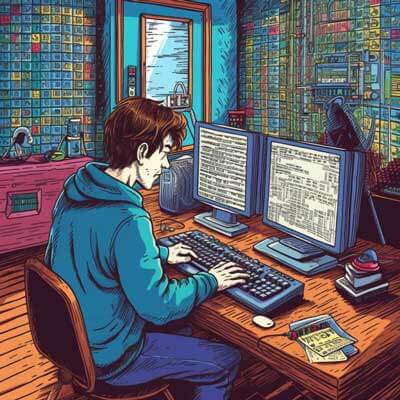
- Querying in Elasticsearch
- Using Match Query with Multiple Fields
- Filtering in Elasticsearch
- Combining Match and Range Queries
- Aggregations in Elasticsearch
- Using Aggregations with Filters
- Indexing in Elasticsearch
- Bulk Indexing
- Document Management in Elasticsearch
- Updating Documents
- Field Mapping in Elasticsearch
- Dynamic Mapping
- Analyzers in Elasticsearch
- Custom Analyzers
- Tokens in Elasticsearch
- Token Filters
- Advanced Querying Techniques
- Data Manipulation in Elasticsearch
- Scaling and Performance Optimization
- Monitoring and Troubleshooting Elasticsearch
- Additional Resources
Querying in Elasticsearch
Elasticsearch is a distributed, scalable, and highly available search engine built on top of the Apache Lucene library. It provides a useful query API that allows users to perform complex searches on large datasets in near real-time. The querying capabilities of Elasticsearch are one of its key features and are essential for retrieving relevant data from the index.
To perform a basic query in Elasticsearch, you can use the match
query. This query type analyzes the input text and retrieves documents that contain the specified terms. Here is an example of using the match
query to search for documents that contain the term “apple”:
GET /my_index/_search { "query": { "match": { "description": "apple" } } }
In this example, we are searching for the term “apple” in the “description” field of the “my_index” index. The match
query analyzes the input text and retrieves documents that contain the term “apple” in the specified field.
Related Article: 16 Amazing Python Libraries You Can Use Now
Using Match Query with Multiple Fields
The match
query can also be used to search for documents that contain the specified terms in multiple fields. You can specify multiple fields in the match
query by using an array of field names. Here is an example:
GET /my_index/_search { "query": { "match": { "title": "apple", "description": "fruit" } } }
In this example, we are searching for documents that contain the term “apple” in the “title” field and the term “fruit” in the “description” field. The match
query will retrieve documents that match either of the specified terms in the specified fields.
Filtering in Elasticsearch
In addition to querying, Elasticsearch also provides filtering capabilities that allow you to narrow down the search results based on specific criteria. Filters are generally faster and more efficient than queries because they do not involve scoring and relevance calculations.
One commonly used filter in Elasticsearch is the range
filter, which allows you to filter documents based on a range of values in a numeric or date field. Here is an example of using the range
filter to retrieve documents that have a price between $10 and $100:
GET /my_index/_search { "query": { "bool": { "filter": { "range": { "price": { "gte": 10, "lte": 100 } } } } } }
In this example, we are using the range
filter to filter documents based on the “price” field. The gte
parameter specifies the minimum value (greater than or equal to), and the lte
parameter specifies the maximum value (less than or equal to). The range
filter will retrieve documents that have a price between $10 and $100.
Combining Match and Range Queries
In Elasticsearch, you can combine the match
query and the range
filter to perform more complex searches. For example, you may want to retrieve documents that contain certain terms and also have a specific range of values in a numeric field. Here is an example:
GET /my_index/_search { "query": { "bool": { "must": [ { "match": { "title": "apple" } }, { "range": { "price": { "gte": 10, "lte": 100 } } } ] } } }
In this example, we are combining the match
query and the range
filter using a bool
query. The bool
query allows you to specify multiple query and filter clauses and control the logical relationship between them. The must
clause specifies that both the match
query and the range
filter must match for a document to be retrieved.
This example will retrieve documents that contain the term “apple” in the “title” field and have a price between $10 and $100.
Related Article: 24 influential books programmers should read
Aggregations in Elasticsearch
Aggregations in Elasticsearch are used to perform data analysis and generate summary statistics on the search results. They allow you to group, filter, and calculate metrics on the data in the index. Aggregations are useful and flexible, providing a wide range of options for analyzing your data.
One commonly used aggregation in Elasticsearch is the terms
aggregation, which calculates the frequency of terms in a specific field. Here is an example of using the terms
aggregation to calculate the number of documents for each value in the “category” field:
GET /my_index/_search { "aggs": { "category_count": { "terms": { "field": "category" } } } }
In this example, we are using the terms
aggregation to calculate the frequency of terms in the “category” field. The result of the aggregation will be a list of terms and their corresponding document counts.
Using Aggregations with Filters
Aggregations can also be combined with filters to calculate metrics on a subset of the search results. This is useful when you want to analyze a specific subset of the data based on certain criteria. Here is an example of using the terms
aggregation with a filter to calculate the number of documents for each value in the “category” field, but only for documents that have a price between $10 and $100:
GET /my_index/_search { "query": { "bool": { "filter": { "range": { "price": { "gte": 10, "lte": 100 } } } } }, "aggs": { "category_count": { "terms": { "field": "category" } } } }
In this example, we have added a bool
query with a range
filter to the query to filter documents based on the price range. The aggs
section remains the same, and the terms
aggregation will now calculate the frequency of terms in the “category” field only for the filtered subset of documents.
Indexing in Elasticsearch
Indexing is the process of adding documents to an Elasticsearch index. Elasticsearch uses a distributed architecture to store and retrieve data, and indexing is a key component of this architecture. When a document is indexed, it is stored in one or more shards, which are distributed across different nodes in the Elasticsearch cluster.
To index a document in Elasticsearch, you need to specify the index, type, and document ID. Here is an example of indexing a document in the “my_index” index, with the “my_type” type, and the document ID “1”:
PUT /my_index/my_type/1 { "title": "Document 1", "description": "This is the first document" }
In this example, we are using the PUT
API to index a document in Elasticsearch. The URL specifies the index, type, and document ID. The request body contains the JSON document to be indexed.
Related Article: 7 Shared Traits of Ineffective Engineering Teams
Bulk Indexing
When indexing a large number of documents, it is more efficient to use the bulk API. The bulk API allows you to index multiple documents in a single request, reducing the overhead of network communication. Here is an example of bulk indexing three documents in the “my_index” index:
POST /my_index/my_type/_bulk {"index": {"_id": "1"}} {"title": "Document 1", "description": "This is the first document"} {"index": {"_id": "2"}} {"title": "Document 2", "description": "This is the second document"} {"index": {"_id": "3"}} {"title": "Document 3", "description": "This is the third document"}
In this example, we are using the POST
API to perform a bulk request. Each line in the request body consists of two JSON objects: the first object specifies the index operation with the document ID, and the second object contains the document to be indexed.
Document Management in Elasticsearch
Elasticsearch provides various APIs for managing documents in the index, such as creating, updating, deleting, and retrieving documents. These APIs allow you to perform CRUD (Create, Read, Update, Delete) operations on individual documents.
To create a new document in Elasticsearch, you can use the index API. Here is an example of creating a new document in the “my_index” index with the document ID “1”:
PUT /my_index/my_type/1 { "title": "New Document", "description": "This is a new document" }
In this example, we are using the PUT
API to create a new document in Elasticsearch. The URL specifies the index, type, and document ID. The request body contains the JSON document to be created.
Updating Documents
To update an existing document in Elasticsearch, you can use the update API. The update API allows you to modify specific fields of a document without having to reindex the entire document. Here is an example of updating the “description” field of the document with the ID “1” in the “my_index” index:
POST /my_index/my_type/1/_update { "doc": { "description": "Updated description" } }
In this example, we are using the POST
API with the update operation to update the document. The URL specifies the index, type, and document ID. The request body contains the JSON object with the fields to be updated.
Related Article: Agile Shortfalls and What They Mean for Developers
Field Mapping in Elasticsearch
Field mapping in Elasticsearch is the process of defining the data type and characteristics of each field in the index. Field mapping is important because it determines how Elasticsearch analyzes, indexes, and searches the data. By default, Elasticsearch tries to automatically detect the data type of each field, but it is recommended to define explicit mappings for fields to ensure consistency and control over the data.
To define a field mapping in Elasticsearch, you can use the put mapping API. Here is an example of defining a field mapping for the “title” field in the “my_index” index:
PUT /my_index/_mapping { "properties": { "title": { "type": "text", "analyzer": "english" } } }
In this example, we are using the PUT
API to define the field mapping for the “title” field. The request body contains the JSON object with the field properties. In this case, we are specifying the data type as “text” and the analyzer as “english”. The analyzer determines how the text is analyzed and tokenized during indexing and searching.
Dynamic Mapping
Elasticsearch also supports dynamic mapping, which allows fields to be automatically added to the mapping when new documents are indexed. Dynamic mapping is useful when you have a flexible data schema and want to automatically adapt the mapping to new fields. However, it is important to be aware of the potential pitfalls of dynamic mapping, such as mapping conflicts and incorrect field types.
Analyzers in Elasticsearch
Analyzers in Elasticsearch are responsible for processing text data during indexing and searching. They perform tasks such as tokenization, stemming, and case normalization to ensure accurate and relevant search results. Elasticsearch provides a variety of built-in analyzers, each designed for specific use cases and languages.
One commonly used analyzer in Elasticsearch is the standard
analyzer, which performs basic text analysis by splitting the text into individual terms. Here is an example of using the standard
analyzer in a field mapping:
PUT /my_index { "mappings": { "properties": { "title": { "type": "text", "analyzer": "standard" } } } }
In this example, we are using the standard
analyzer in the field mapping for the “title” field. The standard
analyzer is the default analyzer in Elasticsearch and is suitable for most use cases.
Related Article: Altering Response Fields in an Elasticsearch Query
Custom Analyzers
In addition to the built-in analyzers, Elasticsearch also allows you to create custom analyzers by combining different tokenizers and token filters. Custom analyzers can be tailored to specific requirements and can improve the accuracy and relevance of search results.
Here is an example of creating a custom analyzer that uses the whitespace
tokenizer and the lowercase
token filter:
PUT /my_index { "settings": { "analysis": { "analyzer": { "custom_analyzer": { "type": "custom", "tokenizer": "whitespace", "filter": ["lowercase"] } } } }, "mappings": { "properties": { "title": { "type": "text", "analyzer": "custom_analyzer" } } } }
In this example, we are creating a custom analyzer called “custom_analyzer”. The whitespace
tokenizer splits the text into terms based on whitespace, and the lowercase
token filter converts the terms to lowercase. The custom analyzer is then used in the field mapping for the “title” field.
Tokens in Elasticsearch
Tokens in Elasticsearch are the individual units of text that are generated during the tokenization process. Tokenization is the process of splitting the text into individual terms, which are then used for indexing and searching. Each token represents a single term and is associated with a specific position and offset within the original text.
To analyze a text string and generate tokens in Elasticsearch, you can use the analyze API. Here is an example of analyzing the text “Hello World” using the standard
analyzer:
GET /_analyze { "analyzer": "standard", "text": "Hello World" }
In this example, we are using the GET
API with the _analyze
endpoint to analyze the text. The request body specifies the analyzer to be used and the text to be analyzed. The response will contain a list of tokens generated by the analyzer.
Token Filters
Token filters in Elasticsearch are used to modify the tokens generated during the tokenization process. They can perform tasks such as stemming, stopword removal, and synonym expansion. Token filters are applied after the tokens have been generated by the tokenizer and can modify or remove tokens based on specific criteria.
Here is an example of using the lowercase
token filter to convert the tokens to lowercase:
GET /_analyze { "tokenizer": "standard", "filter": ["lowercase"], "text": "Hello World" }
In this example, we are using the GET
API with the _analyze
endpoint to analyze the text. The request body specifies the tokenizer to be used, the token filter to be applied, and the text to be analyzed. The response will contain the lowercase tokens generated by the analyzer.
Related Article: BFS/DFS: Breadth First Search & Depth First Search Tutorial
Advanced Querying Techniques
Elasticsearch provides a wide range of advanced querying techniques that enable you to perform complex searches and retrieve relevant data from the index. These techniques include query types, aggregations, filters, and more. Here are a few examples of advanced querying techniques in Elasticsearch:
– Fuzzy Query: The fuzzy query allows you to search for terms that are similar to a specified term, taking into account possible misspellings and variations. Here is an example:
GET /my_index/_search { "query": { "fuzzy": { "title": { "value": "appl", "fuzziness": "AUTO" } } } }
In this example, we are using the fuzzy
query to search for documents that have a similar term to “appl” in the “title” field. The fuzziness
parameter specifies the degree of fuzziness allowed in the search.
– Match Phrase Query: The match phrase query allows you to search for documents that contain a specified phrase in the exact order. Here is an example:
GET /my_index/_search { "query": { "match_phrase": { "description": "red apple" } } }
In this example, we are using the match_phrase
query to search for documents that contain the phrase “red apple” in the “description” field. The match_phrase
query analyzes the input text and retrieves documents that have the exact phrase in the specified field.
– Multi-match Query: The multi-match query allows you to search for a term in multiple fields. Here is an example:
GET /my_index/_search { "query": { "multi_match": { "query": "apple", "fields": ["title", "description"] } } }
In this example, we are using the multi_match
query to search for the term “apple” in the “title” and “description” fields. The multi_match
query analyzes the input text and retrieves documents that contain the term in any of the specified fields.
Data Manipulation in Elasticsearch
Elasticsearch provides various APIs and features for manipulating data in the index. These include bulk operations, updating documents, deleting documents, and more. Here are a few examples of data manipulation in Elasticsearch:
– Bulk API: The bulk API allows you to perform multiple create, update, delete, or index operations in a single request, reducing the overhead of network communication. Here is an example of using the bulk API to index multiple documents:
POST /my_index/_bulk {"index": {"_id": "1"}} {"title": "Document 1", "description": "This is the first document"} {"index": {"_id": "2"}} {"title": "Document 2", "description": "This is the second document"}
In this example, we are using the POST
API with the _bulk
endpoint to perform a bulk request. Each line in the request body consists of two JSON objects: the first object specifies the operation (index in this case) and the document ID, and the second object contains the document to be indexed.
– Updating Documents: To update an existing document in Elasticsearch, you can use the update API. The update API allows you to modify specific fields of a document without having to reindex the entire document. Here is an example of updating the “description” field of the document with the ID “1”:
POST /my_index/my_type/1/_update { "doc": { "description": "Updated description" } }
In this example, we are using the POST
API with the update operation to update the document. The URL specifies the index, type, and document ID. The request body contains the JSON object with the fields to be updated.
– Deleting Documents: To delete a document in Elasticsearch, you can use the delete API. Here is an example of deleting the document with the ID “1” in the “my_index” index:
DELETE /my_index/my_type/1
In this example, we are using the DELETE
API to delete the document. The URL specifies the index, type, and document ID.
Scaling and Performance Optimization
Scaling and performance optimization are crucial aspects of running Elasticsearch in production. As your data grows and the number of queries increases, you need to ensure that your Elasticsearch cluster can handle the load and provide fast response times. Here are some techniques for scaling and optimizing performance in Elasticsearch:
– Shard Allocation: Elasticsearch distributes data across multiple shards to achieve horizontal scalability. By default, an index is divided into five primary shards, but you can customize the number of shards based on your requirements. Increasing the number of shards allows for parallel processing and better query performance. However, it also increases the overhead of managing and replicating shards. You need to carefully balance the number of shards to avoid unnecessary overhead.
– Hardware Optimization: Elasticsearch performance heavily depends on the underlying hardware. To optimize performance, you should use SSDs for storage to reduce disk latency. Additionally, having a sufficient amount of RAM is critical for caching frequently accessed data and speeding up search operations. It is recommended to allocate at least half of the available RAM to Elasticsearch’s heap size.
– Query Optimization: Elasticsearch provides useful querying capabilities, but complex queries can be resource-intensive and impact performance. To optimize queries, you can use techniques such as query caching, filter caching, and query rewriting. You should also consider using filters instead of queries for non-scoring operations to improve performance.
– Indexing Optimization: Efficient indexing is essential for fast and accurate search operations. You can optimize indexing by reducing the number of indexed fields, disabling unnecessary features like text analysis or indexing, and using the bulk API for bulk indexing operations.
– Monitoring and Logging: To identify performance bottlenecks and troubleshoot issues, you need to monitor your Elasticsearch cluster and analyze the logs. Elasticsearch provides a monitoring API and various plugins for monitoring cluster health, resource usage, query performance, and more. You should also enable logging and analyze the logs to identify any warning or error messages.
Related Article: Comparing GraphQL and Elasticsearch
Monitoring and Troubleshooting Elasticsearch
Monitoring and troubleshooting Elasticsearch is crucial for maintaining a healthy and performant cluster. Elasticsearch provides various tools and APIs for monitoring and troubleshooting, allowing you to identify and resolve issues quickly. Here are some techniques for monitoring and troubleshooting Elasticsearch:
– Cluster Health API: The Cluster Health API provides information about the health of your Elasticsearch cluster. It can be used to check the status of nodes, indices, and shards, and monitor the overall health of the cluster. The API returns a detailed JSON response with information such as the number of nodes, active and inactive shards, and cluster status.
– Index Stats API: The Index Stats API provides statistics about the size, document count, and other metrics for each index in your Elasticsearch cluster. It can be used to monitor the growth of indices, track resource usage, and identify any indexing or search performance issues. The API returns a detailed JSON response with various statistics for each index.
– Slow Log: Elasticsearch has a slow log feature that records queries that take longer than a specified threshold to execute. The slow log can be useful for identifying slow queries and understanding the performance impact of different search operations. You can configure the slow log threshold and analyze the log entries to optimize query performance.
– Garbage Collection Logs: Elasticsearch runs on the JVM, and garbage collection (GC) is a critical aspect of its performance. Analyzing the garbage collection logs can help identify memory-related issues and optimize JVM settings. You can enable verbose GC logging in the Elasticsearch configuration and analyze the logs using tools like GCViewer or Elastic’s own Elasticsearch Service Console.
– Cluster Diagnostics: Elasticsearch provides a diagnostic tool called es-diagnostics
that can be used to collect diagnostic information about your cluster. The tool collects various metrics, logs, and configuration files from each node in the cluster and generates a comprehensive diagnostic report. The report can be useful for troubleshooting issues, identifying misconfigurations, and analyzing performance bottlenecks.
Additional Resources
– Official Elasticsearch Documentation
– Elasticsearch: The Definitive Guide
– Elasticsearch – Wikipedia