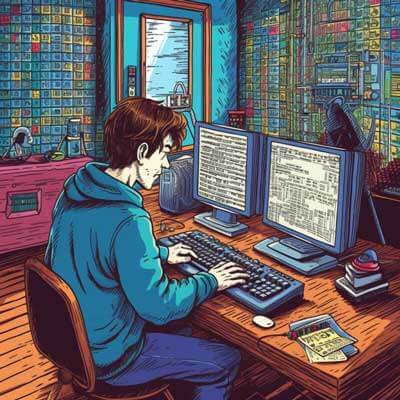
Table of Contents
The Concept of Greedy Algorithms
In the world of computer programming and algorithm design, the term "greedy" refers to a specific approach that is often used to solve optimization problems. Greedy algorithms are a class of algorithms that make locally optimal choices at each step with the hope of finding a global optimum solution. These algorithms are called "greedy" because they prioritize immediate gains without considering the long-term consequences.
To understand the concept of greedy algorithms, let's consider an example. Suppose you are given a set of tasks, each with a certain value and deadline. The goal is to maximize the total value of completed tasks while ensuring that no task exceeds its deadline. A greedy approach to solving this problem would involve selecting the tasks with the highest value-to-deadline ratio at each step.
Here's an example implementation of a greedy algorithm to solve this task scheduling problem in Python:
def schedule_tasks(tasks): tasks = sorted(tasks, key=lambda x: x[0] / x[1], reverse=True) schedule = [] deadline_count = max(task[1] for task in tasks) for task in tasks: deadline = min(deadline_count, task[1]) schedule.append(task) deadline_count -= 1 return schedule
In this example, the tasks
parameter represents a list of tuples, where each tuple consists of the value and deadline of a task. The algorithm sorts the tasks in descending order of their value-to-deadline ratio and then iterates over the sorted list, adding each task to the schedule until the deadline count is exhausted.
Related Article: How to Use Embedded JavaScript (EJS) in Node.js
The Optimization Problem and Greedy Algorithms
Greedy algorithms are particularly useful for solving optimization problems. An optimization problem involves finding the best solution from a set of possible solutions that satisfies certain constraints. The objective is to maximize or minimize an objective function.
In the context of greedy algorithms, the optimization problem can be thought of as finding the best solution by making locally optimal choices at each step. The algorithm aims to find a solution that is as close to the global optimum as possible, but it does not guarantee the optimal solution in all cases.
Let's consider the classic "knapsack problem" as an example of an optimization problem. In this problem, you are given a set of items, each with a weight and value, and a knapsack with a maximum weight capacity. The goal is to determine the most valuable combination of items that can be packed into the knapsack without exceeding its weight capacity.
A greedy algorithm for the knapsack problem would involve selecting items with the highest value-to-weight ratio at each step until the knapsack is full. Here's an example implementation in Python:
def knapsack(items, capacity): items = sorted(items, key=lambda x: x[1] / x[0], reverse=True) knapsack = [] weight_count = capacity for item in items: if weight_count >= item[0]: knapsack.append(item) weight_count -= item[0] return knapsack
In this example, the items
parameter represents a list of tuples, where each tuple consists of the weight and value of an item. The algorithm sorts the items in descending order of their value-to-weight ratio and then iterates over the sorted list, adding each item to the knapsack as long as it does not exceed the weight capacity.
Heuristics in Greedy Algorithms
Heuristics play a crucial role in the design and implementation of greedy algorithms. Heuristics are rules or strategies that guide the decision-making process of the algorithm. They help determine which choice is the best at each step based on the available information.
In the context of greedy algorithms, heuristics are used to make locally optimal choices. These choices are not guaranteed to be the best overall, but they are made with the hope that they will lead to a globally optimal solution.
Let's consider an example to understand the role of heuristics in greedy algorithms. Suppose you are given a set of cities, and you need to find the shortest possible route that visits each city exactly once and returns to the starting city. This is known as the "traveling salesman problem."
A common heuristic used in greedy algorithms for the traveling salesman problem is the "nearest neighbor" heuristic. This heuristic involves selecting the nearest unvisited city at each step. Here's an example implementation in Python:
import math def nearest_neighbor(cities, start_city): route = [start_city] unvisited_cities = set(cities) unvisited_cities.remove(start_city) while unvisited_cities: current_city = route[-1] nearest_city = min(unvisited_cities, key=lambda city: distance(current_city, city)) route.append(nearest_city) unvisited_cities.remove(nearest_city) return route def distance(city1, city2): x1, y1 = city1 x2, y2 = city2 return math.sqrt((x2 - x1)<strong>2 + (y2 - y1)</strong>2)
In this example, the cities
parameter represents a list of tuples, where each tuple consists of the x and y coordinates of a city. The algorithm starts with the start_city
and iteratively selects the nearest unvisited city at each step until all cities have been visited.
Local Optimum in Greedy Algorithms
In the context of greedy algorithms, a local optimum refers to a solution that is optimal within a specific neighborhood or subset of the problem space. It is the best solution that can be obtained by making locally optimal choices at each step.
However, it is important to note that a local optimum may not necessarily be the best overall solution. Greedy algorithms prioritize immediate gains without considering the long-term consequences, which means that they may overlook better solutions that require sacrificing some immediate gains.
Let's consider an example to understand the concept of local optimum in greedy algorithms. Suppose you are given a set of coins with different denominations, and you need to make change for a given amount of money using the fewest possible number of coins.
A greedy algorithm for the change-making problem would involve selecting the largest denomination coin that is less than or equal to the remaining amount at each step. Here's an example implementation in Python:
def make_change(coins, amount): coins = sorted(coins, reverse=True) change = [] for coin in coins: while amount >= coin: change.append(coin) amount -= coin return change
In this example, the coins
parameter represents a list of coin denominations in descending order, and the amount
parameter represents the target amount of money to make change for. The algorithm iterates over the sorted list of coins, selecting the largest denomination coin that is less than or equal to the remaining amount.
While this greedy algorithm provides a locally optimal solution for most cases, it may fail to find the best overall solution in certain scenarios. For example, consider the case where the available coin denominations are [1, 5, 10, 25] and the target amount is 30. The greedy algorithm would select the coins [25, 5] to make change for 30, resulting in a total of 2 coins. However, a better solution would be to use three coins of denomination 10, resulting in a total of 3 coins.
Related Article: The most common wastes of software development (and how to reduce them)
Global Optimum in Greedy Algorithms
In the context of greedy algorithms, a global optimum refers to the best possible solution that can be obtained for a given problem instance. It is the solution that maximizes or minimizes the objective function of the optimization problem.
While greedy algorithms may not always find the global optimum, they often provide solutions that are close to the optimal solution. The greedy approach aims to find a solution that is as close to the global optimum as possible by making locally optimal choices at each step.
Let's consider an example to understand the concept of global optimum in greedy algorithms. Suppose you are given a set of intervals, each with a start and end time, and you need to find the maximum number of non-overlapping intervals.
A greedy algorithm for this interval scheduling problem would involve selecting the interval with the earliest end time at each step. Here's an example implementation in Python:
def schedule_intervals(intervals): intervals = sorted(intervals, key=lambda x: x[1]) schedule = [] end_time = float('-inf') for interval in intervals: if interval[0] >= end_time: schedule.append(interval) end_time = interval[1] return schedule
In this example, the intervals
parameter represents a list of tuples, where each tuple consists of the start and end time of an interval. The algorithm sorts the intervals in ascending order of their end times and then iterates over the sorted list, selecting intervals that do not overlap with previously selected intervals.
While this greedy algorithm does not guarantee the optimal solution in all cases, it often provides a solution that is close to the maximum number of non-overlapping intervals.
The Greedy Approach in Problem Solving
The greedy approach in problem solving involves making locally optimal choices at each step with the hope of finding a globally optimal solution. Greedy algorithms prioritize immediate gains without considering the long-term consequences, which makes them particularly useful for solving optimization problems.
The greedy approach can be applied to a wide range of problem domains, including scheduling, routing, graph algorithms, and combinatorial optimization. It offers a simple and intuitive way to tackle complex problems by breaking them down into smaller subproblems and making incremental progress towards the desired solution.
Let's consider an example to understand the greedy approach in problem solving. Suppose you are given a set of jobs, each with a start time, end time, and profit. The goal is to find the maximum profit that can be obtained by scheduling non-overlapping jobs.
A greedy algorithm for this job scheduling problem would involve selecting the job with the highest profit-to-end time ratio at each step. Here's an example implementation in Python:
def schedule_jobs(jobs): jobs = sorted(jobs, key=lambda x: x[2] / x[1], reverse=True) schedule = [] end_time = float('-inf') total_profit = 0 for job in jobs: if job[0] >= end_time: schedule.append(job) end_time = job[1] total_profit += job[2] return total_profit
In this example, the jobs
parameter represents a list of tuples, where each tuple consists of the start time, end time, and profit of a job. The algorithm sorts the jobs in descending order of their profit-to-end time ratio and then iterates over the sorted list, selecting jobs that do not overlap with previously selected jobs.
This greedy algorithm provides a locally optimal solution for the job scheduling problem and often finds a solution that is close to the maximum profit.
The Greedy Strategy in Algorithm Design
The greedy strategy is a useful tool in algorithm design that can be applied to a wide range of problems. It involves making locally optimal choices at each step with the hope of finding a globally optimal solution. The greedy strategy is particularly useful for solving optimization problems where the goal is to maximize or minimize an objective function.
The key to applying the greedy strategy in algorithm design is identifying the right set of choices to make at each step. These choices should be based on heuristics or rules that guide the decision-making process. While the greedy strategy does not guarantee the optimal solution in all cases, it often provides solutions that are close to the global optimum.
Let's consider an example to understand the greedy strategy in algorithm design. Suppose you are given a set of activities, each with a start time and end time, and you need to find the maximum number of non-overlapping activities.
A greedy algorithm using the greedy strategy for this activity selection problem would involve selecting the activity with the earliest end time at each step. Here's an example implementation in Python:
def select_activities(activities): activities = sorted(activities, key=lambda x: x[1]) selected_activities = [] end_time = float('-inf') for activity in activities: if activity[0] >= end_time: selected_activities.append(activity) end_time = activity[1] return selected_activities
In this example, the activities
parameter represents a list of tuples, where each tuple consists of the start time and end time of an activity. The algorithm sorts the activities in ascending order of their end times and then iterates over the sorted list, selecting activities that do not overlap with previously selected activities.
The greedy strategy in algorithm design provides a simple and efficient way to solve complex optimization problems by making locally optimal choices at each step.
Greediness in Greedy Algorithms
Greediness is a fundamental characteristic of greedy algorithms. It refers to the tendency of these algorithms to prioritize immediate gains without considering the long-term consequences. Greedy algorithms make locally optimal choices at each step with the hope of finding a globally optimal solution.
The greedy nature of these algorithms can be both an advantage and a limitation. On the one hand, greediness allows for simplicity and efficiency in solving optimization problems. Greedy algorithms often provide solutions that are close to the global optimum and can be implemented with relatively low computational resources.
On the other hand, the greedy nature of these algorithms can lead to suboptimal solutions in certain scenarios. By prioritizing immediate gains, greedy algorithms may overlook better solutions that require sacrificing some immediate gains. It is important to carefully analyze the problem and the specific constraints before deciding to use a greedy algorithm.
Let's consider an example to understand the concept of greediness in greedy algorithms. Suppose you are given a set of intervals, each with a start and end time, and you need to find the maximum number of non-overlapping intervals.
A greedy algorithm for this interval scheduling problem would involve selecting the interval with the earliest end time at each step. While this greedy algorithm provides a locally optimal solution for most cases, it may fail to find the best overall solution in certain scenarios.
For example, consider the case where there are three intervals: A=[1,4], B=[2,3], and C=[3,5]. The greedy algorithm would select intervals A and C, resulting in a total of 2 non-overlapping intervals. However, a better solution would be to select intervals B and C, resulting in a total of 2 non-overlapping intervals.
The concept of greediness in greedy algorithms highlights the trade-off between immediate gains and long-term consequences, and the need to carefully analyze the problem and its specific constraints before deciding on the use of a greedy algorithm.
Related Article: How to Use the aria-label Attribute in HTML
The Greedy Choice Property in Algorithm Design
The greedy choice property is a key characteristic of greedy algorithms. It refers to the property that making a locally optimal choice at each step leads to a globally optimal solution. In other words, the greedy choice property ensures that the greedy algorithm always selects the best possible choice at each step, given the current state of the problem.
The greedy choice property is what allows greedy algorithms to make incremental progress towards the desired solution. By making locally optimal choices, these algorithms aim to find a solution that is as close to the global optimum as possible.
Let's consider an example to understand the concept of the greedy choice property in algorithm design. Suppose you are given a set of activities, each with a start time and end time, and you need to find the maximum number of non-overlapping activities.
A greedy algorithm using the greedy choice property for this activity selection problem would involve selecting the activity with the earliest end time at each step. This choice ensures that the selected activities do not overlap with each other and maximizes the number of non-overlapping activities.
The greedy choice property guarantees that this algorithm will always find a solution with the maximum number of non-overlapping activities. By selecting the activity with the earliest end time at each step, the algorithm ensures that no other activity can be selected without overlapping with previously selected activities.
The greedy choice property is a useful property that allows greedy algorithms to make locally optimal choices that lead to globally optimal solutions.
The Greedy Paradigm in Problem Solving
The greedy paradigm is a problem-solving approach that involves making locally optimal choices at each step with the hope of finding a globally optimal solution. It is a fundamental concept in algorithm design and is particularly useful for solving optimization problems.
The greedy paradigm can be thought of as a strategy that guides the decision-making process of the algorithm. It helps determine which choice is the best at each step based on the available information. Greedy algorithms prioritize immediate gains without considering the long-term consequences, which makes them efficient and intuitive for solving certain types of problems.
While the greedy paradigm does not guarantee the optimal solution in all cases, it often provides solutions that are close to the global optimum. It is important to carefully analyze the problem and its specific constraints before deciding to use a greedy algorithm.
Let's consider an example to understand the concept of the greedy paradigm in problem solving. Suppose you are given a set of tasks, each with a certain value and deadline. The goal is to maximize the total value of completed tasks while ensuring that no task exceeds its deadline.
A greedy algorithm using the greedy paradigm for this task scheduling problem would involve selecting the tasks with the highest value-to-deadline ratio at each step. This choice ensures that the selected tasks have the highest possible value given their respective deadlines.
The greedy paradigm allows the algorithm to make incremental progress towards the desired solution by selecting the locally optimal choice at each step. By prioritizing tasks with higher value-to-deadline ratios, the algorithm aims to maximize the total value of completed tasks.
The greedy paradigm is a useful problem-solving approach that provides a simple and intuitive way to tackle complex optimization problems.
Understanding Greedy Algorithms
Greedy algorithms are a class of algorithms that make locally optimal choices at each step with the hope of finding a globally optimal solution. They are particularly useful for solving optimization problems, where the goal is to maximize or minimize an objective function.
The main characteristic of greedy algorithms is their greediness, which refers to their tendency to prioritize immediate gains without considering the long-term consequences. Greedy algorithms make decisions based on heuristics or rules that guide the decision-making process. These heuristics help determine which choice is the best at each step based on the available information.
While greedy algorithms provide simple and efficient solutions to many optimization problems, they may not always find the optimal solution. By prioritizing immediate gains, these algorithms may overlook better solutions that require sacrificing some immediate gains. It is important to carefully analyze the problem and its specific constraints before deciding to use a greedy algorithm.
Understanding the concept of greediness, the role of heuristics, and the greedy choice property is key to understanding how greedy algorithms work and how to appropriately use them in problem solving. By understanding these concepts, software engineers can effectively apply greedy algorithms to solve a wide range of optimization problems.
How Greedy Algorithms Work
Greedy algorithms work by making locally optimal choices at each step with the hope of finding a globally optimal solution. They prioritize immediate gains without considering the long-term consequences. Greedy algorithms make decisions based on heuristics or rules that guide the decision-making process.
The general steps involved in implementing a greedy algorithm are as follows:
1. Define the problem: Clearly define the problem and its specific constraints. Understand the objective function that needs to be maximized or minimized.
2. Identify the choices: Identify the set of choices available at each step. These choices should be based on the problem's constraints and the available information.
3. Define the heuristic: Define the heuristic or rule that guides the decision-making process. This heuristic should help determine which choice is the best at each step based on the available information.
4. Make the choice: Make the locally optimal choice according to the defined heuristic. This choice should be the best choice at the current step based on the available information.
5. Update the solution: Update the solution by incorporating the chosen element or removing elements that are no longer feasible.
6. Repeat steps 2-5: Repeat steps 2-5 until a solution is found or the problem is solved.
Let's consider an example to understand how greedy algorithms work. Suppose you are given a set of activities, each with a start time and end time, and you need to find the maximum number of non-overlapping activities.
A greedy algorithm for this activity selection problem would involve selecting the activity with the earliest end time at each step. This choice ensures that the selected activities do not overlap with each other and maximizes the number of non-overlapping activities.
The key to the success of a greedy algorithm lies in the choice of the heuristic and the ability to make locally optimal choices that lead to a globally optimal solution.
Related Article: Visualizing Binary Search Trees: Deep Dive
Appropriate Use of Greedy Algorithms
Greedy algorithms are a useful tool in algorithm design and can be used to solve a wide range of optimization problems. However, it is important to carefully analyze the problem and its specific constraints before deciding to use a greedy algorithm.
Greedy algorithms are most appropriate for problems that exhibit the following characteristics:
1. Greedy choice property: The problem has the property that making a locally optimal choice at each step leads to a globally optimal solution. The greedy algorithm can make incremental progress towards the desired solution by making locally optimal choices.
2. Overlapping subproblems: The problem can be broken down into smaller subproblems, and the solution to each subproblem can be combined to form the overall solution. Greedy algorithms often work well for problems that can be solved in a "greedy" manner, without needing to consider the entire problem space.
3. Optimal substructure: The problem exhibits optimal substructure, which means that an optimal solution to the problem contains optimal solutions to its subproblems. Greedy algorithms can exploit this property by making locally optimal choices that contribute to the overall optimal solution.
4. Efficiency: Greedy algorithms are often more efficient than other approaches, such as dynamic programming or backtracking. They provide simple and intuitive solutions to many optimization problems and can be implemented with relatively low computational resources.
However, it is important to note that greedy algorithms may not always find the optimal solution. By prioritizing immediate gains, these algorithms may overlook better solutions that require sacrificing some immediate gains. It is crucial to carefully analyze the problem and its specific constraints before deciding on the use of a greedy algorithm.
Advantages of Using Greedy Algorithms
Greedy algorithms offer several advantages that make them a popular choice for solving optimization problems:
1. Simplicity: Greedy algorithms provide simple and intuitive solutions to many optimization problems. They involve making locally optimal choices at each step, which can be easily implemented and understood.
2. Efficiency: Greedy algorithms are often more efficient than other approaches, such as dynamic programming or backtracking. They provide solutions that can be computed in a relatively short amount of time and with relatively low computational resources.
3. Incremental progress: Greedy algorithms make incremental progress towards the desired solution by making locally optimal choices. This allows them to solve problems that can be solved in a "greedy" manner, without needing to consider the entire problem space.
4. Subproblem optimization: Greedy algorithms often exhibit optimal substructure, which means that an optimal solution to the problem contains optimal solutions to its subproblems. This allows the algorithm to exploit the subproblem optimization property and make locally optimal choices.
5. Wide applicability: Greedy algorithms can be applied to a wide range of problem domains, including scheduling, routing, graph algorithms, and combinatorial optimization. They offer a versatile approach to solving optimization problems and can be adapted to suit various problem constraints.
While greedy algorithms have their limitations and may not always find the optimal solution, their advantages make them a valuable tool in algorithm design. By understanding the problem and its specific constraints, software engineers can effectively apply greedy algorithms to solve a wide range of optimization problems.
Limitations of Greedy Algorithms
While greedy algorithms offer simplicity and efficiency in solving optimization problems, they have certain limitations that need to be considered before deciding to use them:
1. Suboptimal solutions: Greedy algorithms do not always find the optimal solution. By prioritizing immediate gains, these algorithms may overlook better solutions that require sacrificing some immediate gains. It is important to carefully analyze the problem and its specific constraints before deciding on the use of a greedy algorithm.
2. Lack of backtracking: Greedy algorithms do not consider the consequences of their choices beyond the current step. Once a choice is made, it cannot be undone. This lack of backtracking can lead to suboptimal solutions in certain scenarios where sacrificing immediate gains can lead to a better overall solution.
3. Problem dependency: Greedy algorithms are problem-dependent and may not be suitable for all types of problems. The problem must exhibit the greedy choice property, overlapping subproblems, and optimal substructure for a greedy algorithm to be effective.
4. Complexity analysis: The complexity of a greedy algorithm can be difficult to analyze. While greedy algorithms are often more efficient than other approaches, such as dynamic programming or backtracking, their performance can vary depending on the problem constraints and the chosen heuristic.
5. Trade-offs: Greedy algorithms involve trade-offs between immediate gains and long-term consequences. By prioritizing immediate gains, these algorithms may sacrifice better solutions that require sacrificing some immediate gains. The choice of the heuristic and the analysis of the problem constraints are crucial in striking the right balance.
Despite these limitations, greedy algorithms remain a valuable tool in algorithm design. By understanding the problem and its specific constraints, software engineers can effectively apply greedy algorithms and leverage their advantages while mitigating their limitations.
The Greedy Choice Property Explained
The greedy choice property is a key characteristic of greedy algorithms. It refers to the property that making a locally optimal choice at each step leads to a globally optimal solution. In other words, the greedy choice property ensures that the greedy algorithm always selects the best possible choice at each step, given the current state of the problem.
The greedy choice property is what allows greedy algorithms to make incremental progress towards the desired solution. By making locally optimal choices, these algorithms aim to find a solution that is as close to the global optimum as possible.
To understand the greedy choice property, let's consider an example. Suppose you are given a set of activities, each with a start time and end time, and you need to find the maximum number of non-overlapping activities.
A greedy algorithm using the greedy choice property for this activity selection problem would involve selecting the activity with the earliest end time at each step. This choice ensures that the selected activities do not overlap with each other and maximizes the number of non-overlapping activities.
The greedy choice property guarantees that this algorithm will always find a solution with the maximum number of non-overlapping activities. By selecting the activity with the earliest end time at each step, the algorithm ensures that no other activity can be selected without overlapping with previously selected activities.
The greedy choice property is a useful property that allows greedy algorithms to make locally optimal choices that lead to globally optimal solutions.
Related Article: How to Implement HTML Select Multiple As a Dropdown
Finding the Optimal Solution with Greedy Algorithms
Finding the optimal solution with greedy algorithms can be challenging, as these algorithms do not always guarantee the optimal solution. However, there are certain strategies and techniques that can be employed to increase the likelihood of finding the optimal solution using a greedy approach.
1. Define the problem: Clearly define the problem and its specific constraints. Understand the objective function that needs to be maximized or minimized.
2. Identify the choices: Identify the set of choices available at each step. These choices should be based on the problem's constraints and the available information.
3. Define the heuristic: Define the heuristic or rule that guides the decision-making process. This heuristic should help determine which choice is the best at each step based on the available information.
4. Analyze the problem constraints: Carefully analyze the problem constraints to identify any specific patterns or properties that can be exploited. Look for properties such as the greedy choice property, overlapping subproblems, or optimal substructure.
5. Optimize the heuristic: Fine-tune the heuristic based on the problem constraints and the available information. Consider the trade-offs between immediate gains and long-term consequences.
6. Test and validate: Test the algorithm with different inputs and validate the results against known optimal solutions or upper bounds. Iterate on the algorithm design and fine-tune the heuristic if necessary.
Difference Between Greedy and Dynamic Programming Algorithms
Greedy and dynamic programming algorithms are two different approaches to solving optimization problems. While both approaches aim to find the optimal solution, they differ in their strategies and decision-making processes.
The main difference between greedy and dynamic programming algorithms lies in their choice of subproblems and their approach to solving these subproblems.
Greedy algorithms make locally optimal choices at each step, without considering the long-term consequences. They prioritize immediate gains and aim to find a globally optimal solution by making the best possible choice at each step. Greedy algorithms are often more efficient than dynamic programming algorithms and provide simple and intuitive solutions to many optimization problems.
On the other hand, dynamic programming algorithms break down the problem into overlapping subproblems and solve each subproblem only once. Unlike greedy algorithms, dynamic programming algorithms evaluate all possible choices and select the best one based on the optimal substructure property. Dynamic programming algorithms are often more time-consuming and memory-intensive than greedy algorithms, but they guarantee the optimal solution.
Let's consider an example to understand the difference between greedy and dynamic programming algorithms. Suppose you are given a set of intervals, each with a start and end time, and you need to find the maximum number of non-overlapping intervals.
A greedy algorithm for this interval scheduling problem would involve selecting the interval with the earliest end time at each step. This choice ensures that the selected intervals do not overlap with each other and maximizes the number of non-overlapping intervals.
A dynamic programming algorithm for the same problem would involve evaluating all possible combinations of intervals and selecting the combination that maximizes the number of non-overlapping intervals. This approach guarantees the optimal solution but requires evaluating a large number of combinations.
Greedy Algorithms and the Global Optimum Solution
Greedy algorithms do not always find the global optimum solution, but they often provide solutions that are close to the global optimum. The greedy approach is characterized by making locally optimal choices at each step, without considering the long-term consequences.
While greedy algorithms prioritize immediate gains, they may overlook better solutions that require sacrificing some immediate gains. This limitation can prevent them from finding the global optimum solution in certain scenarios.
To illustrate this, let's consider an example. Suppose you are given a set of intervals, each with a start and end time, and you need to find the maximum number of non-overlapping intervals.
A greedy algorithm for this interval scheduling problem would involve selecting the interval with the earliest end time at each step. This choice ensures that the selected intervals do not overlap with each other and maximizes the number of non-overlapping intervals.
However, consider the case where there are three intervals: A=[1,4], B=[2,3], and C=[3,5]. The greedy algorithm would select intervals A and C, resulting in a total of 2 non-overlapping intervals. However, a better solution would be to select intervals B and C, resulting in a total of 2 non-overlapping intervals.
This example demonstrates that the greedy algorithm, while providing a locally optimal solution, fails to find the global optimum solution. It is important to carefully analyze the problem and its specific constraints before deciding on the use of a greedy algorithm, as it may not always lead to the global optimum solution.
Suitability of Greedy Approach for Optimization Problems
The greedy approach is particularly suitable for solving optimization problems, where the goal is to maximize or minimize an objective function. Greedy algorithms make locally optimal choices at each step, without considering the long-term consequences. They prioritize immediate gains and aim to find a globally optimal solution by making the best possible choice at each step.
The suitability of the greedy approach for optimization problems can be attributed to several factors:
1. Simplicity: Greedy algorithms provide simple and intuitive solutions to many optimization problems. They involve making locally optimal choices at each step, which can be easily implemented and understood.
2. Efficiency: Greedy algorithms are often more efficient than other approaches, such as dynamic programming or backtracking. They provide solutions that can be computed in a relatively short amount of time and with relatively low computational resources.
3. Incremental progress: Greedy algorithms make incremental progress towards the desired solution by making locally optimal choices. This allows them to solve problems that can be solved in a "greedy" manner, without needing to consider the entire problem space.
4. Subproblem optimization: Greedy algorithms often exhibit optimal substructure, which means that an optimal solution to the problem contains optimal solutions to its subproblems. This allows the algorithm to exploit the subproblem optimization property and make locally optimal choices.
5. Wide applicability: Greedy algorithms can be applied to a wide range of problem domains, including scheduling, routing, graph algorithms, and combinatorial optimization. They offer a versatile approach to solving optimization problems and can be adapted to suit various problem constraints.
However, it is important to note that the suitability of the greedy approach depends on the specific problem and its constraints. Greedy algorithms may not always find the optimal solution and may overlook better solutions that require sacrificing some immediate gains. It is crucial to carefully analyze the problem and its specific constraints before deciding on the use of a greedy algorithm.
Related Article: Comparing GraphQL and Elasticsearch
Additional Resources
- Greedy Algorithm - GeeksforGeeks