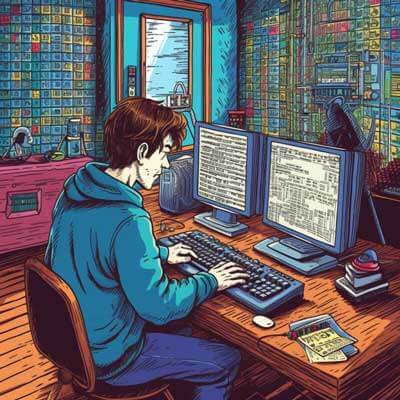
Table of Contents
Introduction to Generics in Go
Generics in Go allow us to write functions and data structures that can work with different types without sacrificing type safety. While Go has been known for its simplicity and minimalism, the lack of generics has sometimes made it challenging to write reusable and flexible code. However, with the release of Go 1.18, generics have been introduced as a new feature, opening up new possibilities for Go developers.
Related Article: Comparing GraphQL vs REST & How To Manage APIs
The Syntax of Generics in Go
The syntax of generics in Go involves using type parameters within angle brackets "<>". These type parameters can represent any valid Go type, including basic types, structs, and interfaces. The type parameters are then used within the function or data structure definition to specify the desired behavior.
Here's an example of a generic function that swaps two values of any type:
func Swap[T any](a, b T) (T, T) { return b, a }
In this example, the type parameter T
is used to represent the type of the input values a
and b
, as well as the return type of the function. The any
constraint ensures that T
can be any type.
Types of Generics in Go
Go generics support various types of type parameters, enabling developers to write highly flexible code. Some of the commonly used types of generics in Go include:
- Type Parameters with Constraints: Type parameters can be constrained to specific interfaces or types using the interface{}
or a specific interface name.
- Type Parameters with Bounds: Type parameters can be bounded to a specific set of types using the type
keyword and a set of valid types.
- Type Parameters with Type Lists: Type parameters can be specified as a list of types using the ...
syntax, allowing functions and data structures to work with multiple types simultaneously.
Writing Your First Generic Function in Go
To write your first generic function in Go, you can follow these steps:
1. Define the function using the func
keyword followed by the function name and the type parameter in angle brackets.
2. Specify the function parameters and return types using the type parameter.
3. Implement the function logic, making use of the type parameter as needed.
Here's an example of a generic function that concatenates two slices of any type:
func Concat[T any](a, b []T) []T { return append(a, b...) }
In this example, the type parameter T
represents the type of the slices a
and b
as well as the return type. The function uses the append
function to concatenate the two slices.
Related Article: How To Distinguish Between POST And PUT In HTTP
Code Snippet: Implementing a Generic Swap Function
Here's a code snippet that demonstrates how to implement a generic swap function in Go:
func Swap[T any](a, b *T) { *a, *b = *b, *a }
In this code snippet, the function Swap
takes two pointers a
and b
of any type T
. It swaps the values pointed to by a
and b
using a temporary variable.
Code Snippet: Creating a Generic Stack
Here's a code snippet that shows how to create a generic stack data structure in Go:
type Stack[T any] []T func (s *Stack[T]) Push(value T) { *s = append(*s, value) } func (s *Stack[T]) Pop() T { if len(*s) == 0 { panic("Stack is empty") } index := len(*s) - 1 value := (*s)[index] *s = (*s)[:index] return value }
In this code snippet, the Stack
type is defined as a slice of any type T
. The Push
method adds a value of type T
to the stack, and the Pop
method removes and returns the topmost value from the stack.
Code Snippet: Designing a Generic Queue
Here's a code snippet that demonstrates how to design a generic queue data structure in Go:
type Queue[T any] []T func (q *Queue[T]) Enqueue(value T) { *q = append(*q, value) } func (q *Queue[T]) Dequeue() T { if len(*q) == 0 { panic("Queue is empty") } value := (*q)[0] *q = (*q)[1:] return value }
In this code snippet, the Queue
type is defined as a slice of any type T
. The Enqueue
method adds a value of type T
to the end of the queue, and the Dequeue
method removes and returns the first value from the queue.
Code Snippet: Building a Generic Set
Here's a code snippet that shows how to build a generic set data structure in Go:
type Set[T any] map[T]bool func (s Set[T]) Add(value T) { s[value] = true } func (s Set[T]) Contains(value T) bool { return s[value] } func (s Set[T]) Remove(value T) { delete(s, value) }
In this code snippet, the Set
type is defined as a map with keys of any type T
and boolean values. The Add
method adds a value of type T
to the set, the Contains
method checks if a value exists in the set, and the Remove
method removes a value from the set.
Related Article: Tutorial: Working with Stacks in C
Code Snippet: Crafting a Generic Map
Here's a code snippet that demonstrates how to craft a generic map data structure in Go:
type Map[K comparable, V any] map[K]V func (m Map[K, V]) Put(key K, value V) { m[key] = value } func (m Map[K, V]) Get(key K) V { return m[key] } func (m Map[K, V]) Remove(key K) { delete(m, key) }
In this code snippet, the Map
type is defined as a map with keys of a comparable type K
and values of any type V
. The Put
method adds a key-value pair to the map, the Get
method retrieves the value associated with a key, and the Remove
method removes a key-value pair from the map.
Use Case: Generics in Data Structures
Generics in Go can greatly enhance the flexibility and reusability of data structures. With generics, we can create data structures such as stacks, queues, sets, and maps that can work with different types seamlessly. This allows us to write cleaner and more concise code, as we don't need to duplicate data structure implementations for different types.
Here's an example of using a generic stack to store integers:
stack := new(Stack[int]) stack.Push(42) stack.Push(24) fmt.Println(stack.Pop()) // Output: 24
In this example, we create a new stack of integers using the Stack
type defined earlier. We push two integers onto the stack and then pop the topmost value, which is 24
.
Use Case: Generics in Algorithms
Generics in Go can also be applied to algorithms, allowing us to write generic functions that can operate on different types. This enables us to write reusable and efficient algorithms without sacrificing type safety.
Here's an example of using a generic sorting algorithm:
func Sort[T comparable](slice []T) { sort.Slice(slice, func(i, j int) bool { return slice[i] < slice[j] }) }
In this example, the Sort
function takes a slice of a comparable type T
and uses the sort.Slice
function from the standard library to sort the slice in ascending order.
Use Case: Generics in Network Programming
Generics in Go can be useful in network programming scenarios where we need to handle different types of data. For example, we can use generics to create a generic server that can handle requests and responses of different types.
Here's an example of a generic server:
type Request[T any] struct { Data T } type Response[T any] struct { Result T } func HandleRequest[T any](req Request[T]) Response[T] { // Handle the request and return a response return Response[T]{Result: req.Data} }
In this example, we define a Request
and Response
struct with a generic type parameter T
. The HandleRequest
function takes a Request
with any type T
and returns a Response
with the same type. This allows us to handle requests and responses of different types in a single server implementation.
Related Article: SOLID Principles: Object-Oriented Design Tutorial
Real World Example: Generics in Database Access
Generics in Go can be particularly useful in the context of database access, where we often need to work with different types of data. With generics, we can write generic functions or libraries that can handle database operations for different types of entities.
Here's an example of a generic database access library:
type Repository[T any] struct { db *sql.DB } func (r *Repository[T]) Create(entity T) error { // Insert the entity into the database return nil } func (r *Repository[T]) GetByID(id int) (T, error) { // Retrieve the entity from the database by ID var entity T return entity, nil } func (r *Repository[T]) Update(entity T) error { // Update the entity in the database return nil } func (r *Repository[T]) Delete(entity T) error { // Delete the entity from the database return nil }
In this example, the Repository
struct represents a generic repository that can handle database operations for any type T
. The Create
, GetByID
, Update
, and Delete
methods provide generic implementations for creating, retrieving, updating, and deleting entities of any type from the database.
Real World Example: Generics in Web Services
Generics in Go can also be applied to web services, allowing us to write generic handlers that can handle requests and responses of different types. This can be particularly useful in scenarios where we have multiple endpoints that handle different types of data.
Here's an example of a generic web service handler:
type Handler[T any] struct { // Dependencies and configuration } func (h *Handler[T]) HandleRequest(req Request[T]) Response[T] { // Handle the request and return a response return Response[T]{Result: req.Data} }
In this example, the Handler
struct represents a generic web service handler that can handle requests and responses of any type T
. The HandleRequest
method takes a request of type Request[T]
and returns a response of type Response[T]
, allowing us to handle different types of requests and responses in a single handler.
Real World Example: Generics in File I/O Operations
Generics in Go can be beneficial in file I/O operations, where we often work with different types of data. With generics, we can write generic functions or libraries that can handle reading from and writing to files of different types.
Here's an example of a generic file I/O library:
func ReadFromFile[T any](filename string) (T, error) { // Read data from the file and return it var data T return data, nil } func WriteToFile[T any](filename string, data T) error { // Write data to the file return nil }
In this example, the ReadFromFile
function reads data of any type T
from a file, and the WriteToFile
function writes data of any type T
to a file. This allows us to read and write different types of data using a generic file I/O library.
Performance Consideration: Generics and Memory Usage
While generics in Go provide powerful abstractions, it's essential to consider their impact on memory usage. Generics can lead to increased memory usage, especially when working with complex data structures or large amounts of data.
To mitigate excessive memory usage when using generics, it's important to carefully manage the lifecycle of objects and minimize unnecessary allocations. Reusing objects and avoiding unnecessary copying can help reduce memory usage and improve overall performance.
Related Article: The very best software testing tools
Performance Consideration: Generics and Execution Speed
Generics in Go can impact execution speed due to the additional type checks and indirection introduced by generic code. While the Go compiler's optimizations can help mitigate the performance impact, it's important to be aware of potential performance bottlenecks when working with generics.
To improve execution speed when using generics, consider specializing generic code for frequently used types or critical performance-sensitive sections. By specializing specific cases, you can reduce the overhead introduced by generic code and potentially improve performance.
Best Practices: Designing with Generics in Go
When designing with generics in Go, consider the following best practices:
1. Keep Generics Simple: Aim for simplicity and clarity when using generics. Avoid overly complex type constraints or nested generics that can make code harder to understand and maintain.
2. Prioritize Type Safety: Generics in Go are designed to provide type safety. Ensure that your generic code handles type errors gracefully and provides clear error messages to aid debugging and maintenance.
3. Test Generics Thoroughly: Test your generic code with various types to ensure it behaves correctly and handles all possible scenarios. Pay special attention to edge cases and boundary conditions.
4. Document Type Constraints: When using type constraints in generics, document the expected behavior and requirements of the types involved. This helps other developers understand and utilize your code correctly.
5. Consider Performance Impact: Be mindful of the potential impact on memory usage and execution speed when using generics. Optimize critical sections of code and consider specializing generic code for frequently used types.
Best Practices: Testing with Generics in Go
When testing code that uses generics in Go, consider the following best practices:
1. Test with Various Types: Ensure that your tests cover a wide range of types to validate the behavior of your generic code. Test with basic types, structs, and custom types to verify correctness and compatibility.
2. Test Edge Cases: Test with edge cases and boundary conditions to validate that your generic code handles all possible scenarios correctly. Test with empty collections, nil values, and extreme input values.
3. Test Error Handling: Test error handling scenarios to verify that your generic code handles type errors and constraints appropriately. Test with invalid types and verify that the correct errors are returned.
4. Test Performance: Measure the performance of your generic code using different types and data sizes. Benchmark critical sections and compare the performance against specialized code to identify potential bottlenecks.
5. Use Property-Based Testing: Consider using property-based testing libraries like quick
or gopter
to generate random inputs for your generic code. This can help uncover edge cases and ensure the correctness of your code across a wide range of inputs.
Error Handling: Dealing with Type Mismatch in Generics
When working with generics in Go, type mismatch errors can occur if the provided type does not satisfy the required constraints. To handle type mismatch errors, it's important to provide clear error messages and gracefully handle such situations.
Here's an example of handling a type mismatch error in a generic function:
func Double[T numeric](value T) (T, error) { switch value := value.(type) { case int: return value * 2, nil case float32: return value * 2, nil case float64: return value * 2, nil default: return 0, fmt.Errorf("unsupported type: %T", value) } }
In this example, the Double
function accepts a generic type T
with the constraint numeric
, which represents numeric types. If the provided type does not satisfy the numeric
constraint, an error is returned with a clear message indicating the unsupported type.
Related Article: 16 Amazing Python Libraries You Can Use Now
Error Handling: Handling Null Values in Generics
When working with generics in Go, it's important to handle null values appropriately, especially when dealing with generic types that can be nil. Proper null value handling ensures that your code behaves correctly and avoids runtime errors.
Here's an example of handling null values in a generic function:
func PrintNotNil[T any](value T) { if value != nil { fmt.Println(value) } }
In this example, the PrintNotNil
function accepts a generic type T
and checks if the value is not nil before printing it. This ensures that only non-nil values are printed, avoiding potential runtime errors.
Advanced Technique: Nesting Generics
In Go, it's possible to nest generics, allowing for even more powerful and flexible code. Nesting generics involves using one or more generic types as the type parameters of another generic type or function.
Here's an example of nesting generics:
type Pair[T1 any, T2 any] struct { First T1 Second T2 } type Stack[T any] []T type StackOfPairs[T1 any, T2 any] Stack[Pair[T1, T2]]
In this example, the Pair
type is defined as a struct with two generic type parameters. The Stack
type is a generic stack, and the StackOfPairs
type is a stack of pairs, where the elements of the stack are of type Pair[T1, T2]
.
Advanced Technique: Type Constraints in Generics
In Go generics, type constraints allow you to restrict the types that can be used as type parameters. Type constraints help ensure that the provided types satisfy certain requirements, such as implementing specific interfaces or having specific capabilities.
Here's an example of using type constraints in generics:
type Comparable interface { LessThan(other interface{}) bool } func FindMin[T Comparable](slice []T) T { min := slice[0] for _, value := range slice { if value.LessThan(min) { min = value } } return min }
In this example, the Comparable
interface defines a LessThan
method, which is used as a type constraint in the FindMin
function. The FindMin
function finds the minimum value in a slice of any type T
that satisfies the Comparable
constraint.
Advanced Technique: Generics and Concurrency
Generics in Go can be used in conjunction with concurrency features to write generic code that can be executed concurrently. By combining generics with goroutines and channels, you can create flexible and reusable concurrent code.
Here's an example of using generics with concurrency:
func ConcurrentMap[T any, U any](input []T, mapper func(T) U) []U { output := make([]U, len(input)) var wg sync.WaitGroup for i, value := range input { wg.Add(1) go func(i int, value T) { defer wg.Done() output[i] = mapper(value) }(i, value) } wg.Wait() return output }
In this example, the ConcurrentMap
function applies a mapping function mapper
to each element of the input slice concurrently using goroutines. The function returns a new slice with the mapped values.