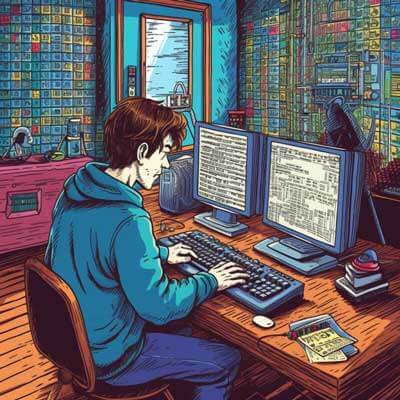
Table of Contents
What are response fields in Elasticsearch?
In Elasticsearch, response fields refer to the fields that are returned in the search results or query responses. When you perform a query in Elasticsearch, it returns a JSON document containing the search results. This document includes various metadata and the actual data matching the query. The response fields are the specific fields from the indexed documents that are included in the response.
Related Article: Detecting High-Cost Queries in Elasticsearch via Kibana
How to modify response fields in an Elasticsearch query?
To modify response fields in an Elasticsearch query, you can use the _source
parameter. The _source
parameter allows you to specify which fields to include or exclude from the response.
Here's an example of how to include specific fields in the response using the _source
parameter:
GET /index/_search { "_source": ["field1", "field2"], "query": { "match_all": {} } }
In the above example, the _source
parameter is set to an array of field names. Only the specified fields (field1
and field2
) will be included in the response, while all other fields will be excluded.
Similarly, you can exclude specific fields from the response by using the _source
parameter with the excludes
option:
GET /index/_search { "_source": { "excludes": ["field3", "field4"] }, "query": { "match_all": {} } }
In this example, the _source
parameter is set to an object with the excludes
option. The specified fields (field3
and field4
) will be excluded from the response, while all other fields will be included.
The purpose of modifying response fields in an Elasticsearch query
Modifying response fields in an Elasticsearch query serves several purposes:
1. Reducing network bandwidth: By including only the necessary fields in the response, you can reduce the amount of data transferred over the network. This can be especially important when dealing with large datasets or when the network bandwidth is limited.
2. Improving query performance: Including only the required fields in the response can significantly improve the performance of your queries. By reducing the size of the response, Elasticsearch can process and return the results more quickly.
3. Enhancing security: Excluding sensitive or unnecessary fields from the response can help improve the security of your application. By controlling the visibility of certain fields, you can prevent unauthorized access to sensitive information.
4. Simplifying data handling: Modifying response fields allows you to extract only the relevant information from the search results. This can simplify data handling and make it easier to process and analyze the returned data.
Different programming languages for Elasticsearch
Elasticsearch provides official clients for several programming languages, making it easy to interact with Elasticsearch from different platforms. Some of the popular programming languages with official Elasticsearch clients include:
1. Java: Elasticsearch provides a Java client that allows you to interact with Elasticsearch from Java applications. The Java client provides a high-level API for performing various operations, such as indexing, searching, and aggregating data.
2. Python: Elasticsearch offers an official Python client called elasticsearch-py
. It provides a comprehensive and flexible API for interacting with Elasticsearch from Python. The Python client supports all major Elasticsearch features and allows you to easily perform CRUD operations, execute queries, and handle search results.
Here's an example of using the Python client to perform a simple search query:
from elasticsearch import Elasticsearch # Connect to Elasticsearch es = Elasticsearch() # Perform a search query response = es.search( index="my_index", body={ "query": { "match": { "field": "value" } } } ) # Process the search results for hit in response["hits"]["hits"]: print(hit["_source"])
3. JavaScript: Elasticsearch provides an official JavaScript client called elasticsearch.js
. It allows you to interact with Elasticsearch from JavaScript applications running in the browser or on the server. The JavaScript client provides a simple and intuitive API for performing CRUD operations, executing queries, and handling search results.
Here's an example of using the JavaScript client to perform a simple search query:
const { Client } = require('@elastic/elasticsearch'); // Create a client instance const client = new Client({ node: 'http://localhost:9200' }); // Perform a search query async function search() { const { body } = await client.search({ index: 'my_index', body: { query: { match: { field: 'value' } } } }); // Process the search results body.hits.hits.forEach(hit => { console.log(hit._source); }); } search();
4. Ruby: Elasticsearch provides an official Ruby client called elasticsearch-ruby
. It allows you to interact with Elasticsearch from Ruby applications. The Ruby client provides a comprehensive API for performing various operations, such as indexing, searching, and aggregating data.
Here's an example of using the Ruby client to perform a simple search query:
require 'elasticsearch' # Create a client instance client = Elasticsearch::Client.new # Perform a search query response = client.search( index: 'my_index', body: { query: { match: { field: 'value' } } } ) # Process the search results response['hits']['hits'].each do |hit| puts hit['_source'] end
These are just a few examples of the official Elasticsearch clients available for different programming languages. Depending on your preferred language, you can choose the appropriate client to interact with Elasticsearch.
Related Article: How To Distinguish Between POST And PUT In HTTP
Dynamically modifying response fields in an Elasticsearch query
In addition to statically modifying response fields using the _source
parameter, Elasticsearch also allows you to dynamically modify the response fields at query time. This can be useful when you need to conditionally include or exclude certain fields based on the query parameters or other runtime conditions.
One way to dynamically modify response fields is by using script fields. Script fields allow you to define custom fields based on a script that is executed for each document in the search results. The script can access and manipulate the document fields, allowing you to modify the response fields on the fly.
Here's an example of using a script field to dynamically modify response fields:
GET /index/_search { "query": { "match_all": {} }, "script_fields": { "modified_field": { "script": { "source": "doc['field'].value.toUpperCase()", "lang": "painless" } } } }
In this example, we define a script field called modified_field
that executes a script for each document in the search results. The script uses the toUpperCase()
function to convert the value of the field
field to uppercase. The modified field will be included in the response along with the other fields.
Another way to dynamically modify response fields is by using the stored_fields
parameter. The stored_fields
parameter allows you to specify a list of fields to be returned in the response. Unlike the _source
parameter, which only operates on indexed fields, the stored_fields
parameter can include both indexed and stored fields.
Here's an example of using the stored_fields
parameter to dynamically modify response fields:
GET /index/_search { "query": { "match_all": {} }, "stored_fields": ["field1", "field2"] }
In this example, we specify that only field1
and field2
should be returned in the response. All other fields will be excluded. This allows you to dynamically control which fields are included in the response based on your specific requirements.
Limitations and restrictions in modifying response fields
While modifying response fields in an Elasticsearch query provides flexibility and control over the returned data, there are certain limitations and restrictions to keep in mind:
1. The _source
field: The _source
field is stored separately from the indexed fields and is retrieved by default. Modifying response fields using the _source
parameter only affects the fields stored in the _source
field. If you want to modify non-indexed fields or fields stored in a different manner, you need to use other methods like script fields or the stored_fields
parameter.
2. Field data types: Modifying response fields may be limited by the data types of the fields. For example, if a field is of type text
, you may not be able to perform certain operations or transformations on it. It's important to understand the data types of the fields you are working with and the operations that are supported for each type.
3. Performance impact: Modifying response fields can have an impact on the performance of your queries, especially when dealing with large datasets or complex transformations. It's important to consider the performance implications and carefully test and optimize your queries to ensure efficient execution.
4. Security considerations: Modifying response fields may have security implications, especially when dealing with sensitive data. It's important to properly secure your Elasticsearch cluster and ensure that only authorized users have access to the necessary fields. Additionally, be cautious when using script fields, as they can introduce potential security risks if not handled properly.
Best practices for modifying response fields in Elasticsearch
When modifying response fields in Elasticsearch queries, it's important to follow some best practices to ensure efficient and reliable operation:
1. Understand your data: Before modifying response fields, make sure you have a good understanding of your data and the fields you are working with. Be aware of the data types, indexing options, and any limitations or restrictions that may apply.
2. Plan your modifications: Carefully plan the modifications you want to make to the response fields. Consider the specific requirements of your application and the data you need to retrieve. Avoid unnecessary modifications or transformations that can impact query performance.
3. Test and optimize: Always test your modified queries and measure their performance. Use tools like the Elasticsearch Profile API to analyze the execution time and resource usage of your queries. Optimize your queries based on the performance analysis to achieve the best possible results.
4. Secure your cluster: Ensure that your Elasticsearch cluster is properly secured to prevent unauthorized access to sensitive data. Implement authentication and authorization mechanisms, and restrict access to the necessary fields based on user roles and permissions.
5. Monitor and maintain: Regularly monitor the performance of your Elasticsearch cluster and the impact of modifying response fields. Keep an eye on resource usage, query latency, and other relevant metrics. Perform regular maintenance tasks like index optimization and data cleanup to keep your cluster running smoothly.
Optimizing performance when modifying response fields
When modifying response fields in an Elasticsearch query, there are several techniques you can use to optimize the performance of your queries:
1. Selective retrieval: Only retrieve the fields that are necessary for your application. Avoid retrieving unnecessary fields, especially if they contain large amounts of data. This can significantly reduce the network bandwidth and improve query performance.
2. Indexing options: Configure the indexing options for your fields to optimize their retrieval. Use appropriate analyzers, index settings, and mappings to ensure efficient indexing and searching. Consider enabling field data caching for frequently accessed fields to improve query performance.
3. Query optimizations: Optimize your query structure and use appropriate query types to improve performance. Avoid unnecessary nested queries, excessive filtering, or complex aggregations that can slow down the query execution. Use the Elasticsearch Profile API to diagnose and optimize your queries.
4. Caching: Take advantage of Elasticsearch's caching mechanisms to improve query performance. Enable query and filter caching for frequently executed queries to avoid unnecessary computation. Use field data caching for fields that are accessed frequently to speed up retrieval.
5. Scaling and sharding: If you have a large dataset or high query load, consider scaling your Elasticsearch cluster and distributing the data across multiple shards. This can improve query performance by parallelizing the search operations and reducing the load on individual nodes.
Related Article: OAuth 2 Tutorial: Introduction & Basics
Modifying response fields without reindexing in Elasticsearch
In some cases, you may need to modify the response fields in Elasticsearch without reindexing the entire dataset. Elasticsearch provides several options to achieve this:
1. Dynamic mapping: Elasticsearch automatically creates mappings for fields based on the data it receives during indexing. You can modify the dynamic mapping settings to control how new fields are created and mapped. This allows you to add or remove fields dynamically without reindexing the existing documents.
2. Update by query: Elasticsearch's Update By Query API allows you to update documents in the index based on a query. You can use this API to modify the values of specific fields in the existing documents. This approach allows you to make targeted changes to the response fields without reindexing the entire dataset.
3. Field aliasing: Elasticsearch supports field aliasing, which allows you to create virtual fields that reference existing fields. You can use field aliases to modify the response fields without changing the underlying data. This can be useful when you want to rename fields or apply transformations to the response fields without reindexing.
4. Scripting: Elasticsearch provides useful scripting capabilities that allow you to manipulate the response fields at query time. You can use scripts to dynamically modify the values of the response fields, perform calculations, or apply transformations. Scripts can be executed using script fields, script filters, or scripting aggregations.
These options provide flexibility and allow you to modify the response fields without the need for a full reindexing. However, it's important to carefully consider the implications and limitations of each approach, as they may have performance and security implications. Test and benchmark your modifications to ensure optimal performance and reliability.
Additional Resources
- Elasticsearch: The Definitive Guide