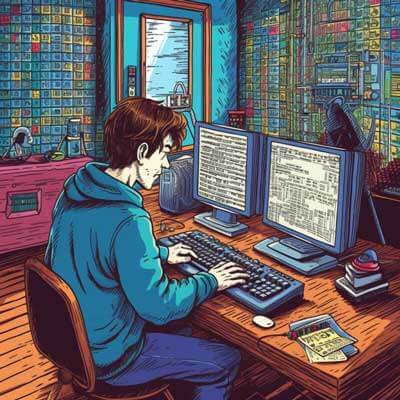
Table of Contents
The Purpose of a Natural Join in SQL
In SQL, a natural join is used to combine rows from two or more tables based on their related columns. It matches the values in these columns and returns a result set that contains only the rows with matching values. The natural join is a useful tool for querying databases and retrieving specific information that is spread across multiple tables.
To understand the purpose of a natural join, let's consider an example. Suppose we have two tables: "Employees" and "Departments". The "Employees" table contains information about the employees in a company, such as their names, IDs, and departments. The "Departments" table contains information about the departments in the company, such as their names and IDs.
Here's an example of a natural join query:
SELECT Employees.Name, Departments.Name FROM Employees NATURAL JOIN Departments;
This query will return a result set with two columns: "Name" from the "Employees" table and "Name" from the "Departments" table, where the "Department ID" matches.
Using a natural join in SQL can simplify complex queries that involve multiple tables and reduce the need for explicit join conditions. However, it's important to note that natural joins can also introduce duplicate records in the result set, which may need to be eliminated.
Related Article: How to Update Records in MySQL with a Select Query
Eliminating Duplicate Records in a SQL Database
Duplicate records in a SQL database can occur when there are multiple entries with the same values for the columns involved in the join condition. These duplicates can lead to data inconsistencies and inaccuracies in query results. Therefore, it is important to have mechanisms in place to eliminate duplicate records in a SQL database.
One common approach to eliminating duplicate records is to use the DISTINCT keyword in SQL queries. The DISTINCT keyword allows you to retrieve only the unique records from a result set, effectively removing any duplicates.
Here's an example that demonstrates how to use the DISTINCT keyword to eliminate duplicates:
SELECT DISTINCT Column1, Column2 FROM Table;
In this example, the SELECT statement retrieves only the unique combinations of values from "Column1" and "Column2" in the specified table, eliminating any duplicate records.
While using the DISTINCT keyword is a straightforward way to eliminate duplicates, it may not always be suitable for complex queries involving multiple tables and join conditions. In such cases, more advanced techniques like GROUP BY and HAVING clauses can be used to achieve the desired results.
Common Techniques for Deleting Duplicate Entries in SQL
In addition to eliminating duplicate records in a SQL database, there may be cases where you need to delete duplicate entries altogether. Deleting duplicate entries can help maintain data integrity and improve query performance.
Here are some common techniques for deleting duplicate entries in SQL:
1. Using the DELETE statement with subqueries: This technique involves using subqueries to identify the duplicate entries and then using the DELETE statement to remove them from the table. Here's an example:
DELETE FROM Table WHERE Column IN ( SELECT Column FROM Table GROUP BY Column HAVING COUNT(*) > 1 );
In this example, the subquery identifies the duplicate entries by grouping the rows based on the specified column and counting the number of occurrences. The DELETE statement then removes the duplicate entries from the table.
2. Using the ROW_NUMBER() function: The ROW_NUMBER() function assigns a unique number to each row in a result set. By using this function in combination with the DELETE statement, you can delete duplicate entries based on specific criteria. Here's an example:
DELETE FROM ( SELECT Column1, Column2, ROW_NUMBER() OVER (PARTITION BY Column1, Column2 ORDER BY Column1) AS RowNumber FROM Table ) AS T WHERE T.RowNumber > 1;
In this example, the ROW_NUMBER() function is used to assign a unique number to each row based on the specified columns. The DELETE statement then removes the rows where the assigned row number is greater than 1, effectively deleting the duplicate entries.
These are just a few examples of the common techniques for deleting duplicate entries in SQL. The approach you choose will depend on the specific requirements of your database and the complexity of your data.
Understanding the DISTINCT Keyword in SQL
The DISTINCT keyword in SQL is used to retrieve only the unique records from a result set, eliminating any duplicate entries. It is a useful tool for data analysis and query optimization when working with databases.
The DISTINCT keyword can be used in conjunction with the SELECT statement to specify which columns to consider for uniqueness. It examines the values in the specified columns and returns only the distinct combinations of those values.
Here's an example that demonstrates how to use the DISTINCT keyword:
SELECT DISTINCT Column1, Column2 FROM Table;
In this example, the SELECT statement retrieves only the unique combinations of values from "Column1" and "Column2" in the specified table, eliminating any duplicate records.
It is important to note that the DISTINCT keyword operates on the entire row, not just on individual columns. This means that if you specify multiple columns in the SELECT statement, the DISTINCT keyword will consider the combination of values in those columns to determine uniqueness.
While the DISTINCT keyword is a simple and effective way to eliminate duplicates in a result set, it may have performance implications when dealing with large datasets. The database engine needs to compare all the values in the specified columns, which can be time-consuming for large tables. In such cases, alternative techniques like GROUP BY and HAVING clauses may be more efficient.
Related Article: Tutorial: Installing PostgreSQL on Amazon Linux
Difference Between Inner Join and Natural Join in SQL
In SQL, both the inner join and natural join are used to combine rows from two or more tables based on related columns. However, there are some key differences between these two types of joins.
An inner join is a type of join that returns only the rows with matching values in the specified columns of the joined tables. It uses a comparison operator, usually an equal sign (=), to match the values and retrieve the desired result set. Here's an example of an inner join query:
SELECT * FROM Table1 INNER JOIN Table2 ON Table1.Column = Table2.Column;
In this example, the inner join combines the rows from "Table1" and "Table2" based on the matching values in the specified columns, resulting in a result set that contains only the rows with matching values.
On the other hand, a natural join is a type of join that combines rows from two or more tables based on the columns with the same name and data type. It automatically matches the columns with the same name in the joined tables and retrieves the result set. Here's an example of a natural join query:
SELECT * FROM Table1 NATURAL JOIN Table2;
In this example, the natural join combines the rows from "Table1" and "Table2" based on the columns with the same name, resulting in a result set that contains only the rows with matching values in those columns.
The main difference between an inner join and a natural join is that an inner join requires an explicit join condition using the ON keyword, while a natural join automatically matches the columns with the same name. Additionally, the natural join may not be suitable in all cases, especially when the columns with the same name are not intended to be used for joining.
It's important to carefully consider the requirements of your query and the structure of your data when choosing between an inner join and a natural join in SQL.
Example of Using Inner Join in a SQL Query
To demonstrate the usage of an inner join in a SQL query, let's consider an example involving two tables: "Customers" and "Orders. The "Customers" table contains information about the customers, such as their IDs and names. The "Orders" table contains information about the orders placed by the customers, such as the order IDs, customer IDs, and order dates.
Here's an example of an inner join query:
SELECT Orders.OrderID, Customers.CustomerName, Orders.OrderDate FROM Orders INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
In this example, the inner join combines the rows from the "Orders" and "Customers" tables based on the matching values in the "CustomerID" column, resulting in a result set that contains the order ID, customer name, and order date for all orders placed by customers.
The inner join is a useful tool for querying databases and retrieving specific information that is spread across multiple tables. By specifying the appropriate join condition, you can combine the relevant data from different tables and obtain a result set that meets your requirements.
What is an Outer Join in SQL and How Does it Differ from a Natural Join
In SQL, an outer join is a type of join that combines rows from two or more tables based on the related columns, just like a natural join. However, the key difference is that an outer join also includes rows that do not have matching values in the joined tables.
An outer join retrieves all the rows from one table and the matching rows from the other table(s), including any unmatched rows. This allows you to include information from one table even if there are no matching values in the other table(s).
There are three types of outer joins in SQL: left outer join, right outer join, and full outer join.
A left outer join includes all the rows from the left table and the matching rows from the right table(s). If there are no matching values in the right table(s), NULL values are included in the result set for the columns of the right table(s).
A right outer join includes all the rows from the right table(s) and the matching rows from the left table. If there are no matching values in the left table, NULL values are included in the result set for the columns of the left table.
A full outer join includes all the rows from both the left and right tables, regardless of whether there are matching values. If there are no matching values, NULL values are included in the result set for the columns of the table(s) without a match.
Here's an example of a left outer join query:
SELECT Customers.CustomerName, Orders.OrderID FROM Customers LEFT OUTER JOIN Orders ON Customers.CustomerID = Orders.CustomerID;
In this example, the left outer join combines the rows from the "Customers" table and the "Orders" table based on the matching values in the "CustomerID" column. It includes all the rows from the "Customers" table and the matching rows from the "Orders" table, but also includes NULL values for the columns of the "Orders" table if there are no matching values.
The outer join is a useful tool when you want to include all the rows from one table, even if there are no matching values in the other table(s). It allows you to retrieve a comprehensive result set that includes all the relevant information from both tables.
Deleting Duplicate Records from Multiple Tables Using a Single SQL Query
In SQL, you can delete duplicate records from multiple tables using a single SQL query by using subqueries and the DELETE statement. This approach allows you to identify and remove duplicate entries across multiple tables efficiently.
Here's an example that demonstrates how to delete duplicate records from multiple tables:
DELETE FROM Table1 WHERE (Column1, Column2) IN ( SELECT Column1, Column2 FROM Table1 GROUP BY Column1, Column2 HAVING COUNT(*) > 1 ) AND (Column1, Column2) IN ( SELECT Column1, Column2 FROM Table2 GROUP BY Column1, Column2 HAVING COUNT(*) > 1 );
In this example, the subqueries identify the duplicate entries in each table by grouping the rows based on the specified columns and counting the number of occurrences. The DELETE statement then removes the duplicate entries from both tables by using the IN operator to match the duplicate column combinations.
It's important to ensure that the column combinations specified in the subqueries are unique and accurately represent the duplicate entries across the tables. Additionally, you can expand this approach to include more tables by adding additional subqueries and conditions to the DELETE statement.
Deleting duplicate records from multiple tables using a single SQL query can help maintain data integrity and consistency in your database. However, it's essential to carefully review the query and backup your data before executing it to avoid unintended consequences.
Related Article: How to Convert Columns to Rows in PostgreSQL
Best Practices for Handling Duplicate Data in a Database
Handling duplicate data in a database is a common challenge that requires careful consideration and implementation of best practices. Here are some best practices for effectively managing duplicate data:
1. Data validation and normalization: Implementing data validation rules and normalizing your database can help prevent the introduction of duplicate data. By defining constraints and relationships between tables, you can ensure the integrity of your data and minimize the occurrence of duplicates.
2. Use unique constraints and indexes: Enforce uniqueness by adding unique constraints or creating unique indexes on the columns that should contain unique values. This will prevent the insertion of duplicate records and improve query performance.
3. Regular data cleansing: Periodically review and clean your data to identify and remove duplicate entries. This can be done using SQL queries or dedicated data cleansing tools. Regular data cleansing helps maintain data accuracy and ensures the reliability of your database.
4. Implement deduplication processes: Deduplication processes involve identifying and merging duplicate records based on specific criteria. These processes can be automated using SQL scripts or data integration tools. Implementing deduplication processes helps streamline data management and improve data quality.
5. Use appropriate join types: When querying your database, choose the appropriate join types (such as inner join, outer join, or natural join) based on your requirements. Be aware that some join types, like natural join, can introduce duplicate records, so it's important to handle them appropriately.
6. Regular backups: Regularly back up your database to safeguard against data loss or corruption. Backups provide a recovery option in case duplicate data causes issues or errors in your database.
7. Monitor and analyze data quality: Implement monitoring and analysis processes to identify patterns and trends in duplicate data. This can help you understand the root causes of duplicates and take proactive measures to prevent them in the future.
Performance Implications when Dealing with Large Datasets and Duplicate Records in SQL
When dealing with large datasets and duplicate records in SQL, there can be performance implications that need to be considered. Here are some factors to keep in mind:
1. Indexing: Indexes can significantly improve query performance by allowing the database engine to quickly locate the relevant data. However, when dealing with large datasets and duplicate records, indexing can become more challenging. Indexes may need to be carefully designed and maintained to ensure optimal performance.
2. Query optimization: Optimizing your SQL queries can help improve performance when dealing with large datasets and duplicate records. This involves analyzing the query execution plans, identifying bottlenecks, and making appropriate adjustments. Techniques such as using appropriate join types and reducing unnecessary operations can enhance query performance.
3. Data partitioning: Partitioning large tables can improve query performance by dividing the data into smaller, more manageable chunks. This allows the database engine to process queries more efficiently, especially when dealing with duplicate records that may span multiple partitions.
4. Database maintenance: Regularly maintaining your database, including tasks such as index rebuilding, statistics updates, and data purging, can help keep the performance of your database at an optimal level. This is particularly important when dealing with large datasets and duplicate records, as they can impact query execution times.
5. Hardware considerations: Large datasets and complex queries may require useful hardware configurations to handle the increased processing demands. Consider factors such as memory, CPU, and disk I/O when dealing with performance issues related to large datasets and duplicate records.
6. Query caching: Caching query results can significantly improve performance, especially when dealing with repetitive queries. By caching the results of frequently executed queries, you can reduce the processing overhead and improve response times.
7. Database design and schema optimization: Properly designing your database schema and optimizing table structures can have a significant impact on performance. This includes considerations such as data types, primary key selection, and normalization. By carefully planning the database schema, you can minimize the occurrence of duplicate records and improve overall performance.
When dealing with large datasets and duplicate records in SQL, it's important to carefully analyze the specific requirements and performance characteristics of your database. By implementing appropriate techniques and optimizations, you can mitigate the performance implications and ensure efficient query execution.