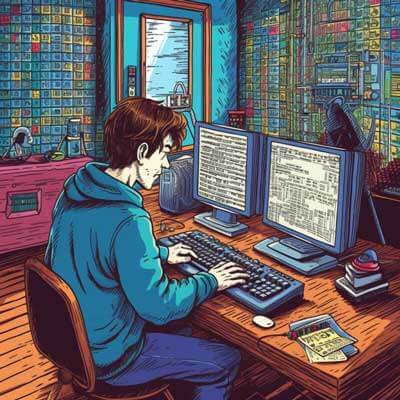
Table of Contents
To convert a list to a string in Python, you can use the built-in join()
function or a list comprehension. In this answer, we'll explore both methods and provide examples to illustrate their usage.
Using the join() function
The join()
function is a convenient way to concatenate the elements of a list into a single string. It takes a delimiter as an argument and returns a string where the elements of the list are joined by that delimiter.
Here's the syntax for using the join()
function:
string = delimiter.join(list)
Let's see an example:
fruits = ['apple', 'banana', 'orange'] delimiter = ', ' result = delimiter.join(fruits) print(result)
Output:
apple, banana, orange
In the example above, we have a list of fruits and we want to convert it into a string where the fruits are separated by a comma and a space. We use the join()
function with a comma and a space as the delimiter, and it returns the desired string.
Related Article: How to Work with Encoding & Multiple Languages in Django
Using a list comprehension
Another approach to convert a list to a string is by using a list comprehension. A list comprehension allows you to iterate over a list and perform an operation on each element to create a new list. In this case, we can iterate over the elements of the list and convert them to strings, and then join them together using the join()
function.
Here's an example:
fruits = ['apple', 'banana', 'orange'] result = ', '.join([str(fruit) for fruit in fruits]) print(result)
Output:
apple, banana, orange
In the example above, we use a list comprehension to iterate over each fruit in the list and convert it to a string using the str()
function. Then, we use the join()
function with a comma and a space as the delimiter to join the elements of the new list into a string.
Reasons for converting a list to a string
There are several reasons why you might need to convert a list to a string in Python. Some common use cases include:
- Displaying the elements of a list as a single string for output or logging purposes.
- Saving a list of values as a string in a file or a database.
- Passing a list of values as a string argument to a function or an API.
Converting a list to a string allows you to manipulate and work with the list data in a more flexible and convenient way.
Best practices and suggestions
When converting a list to a string in Python, keep the following best practices in mind:
- Choose a suitable delimiter: The delimiter you use to join the elements of the list into a string should be chosen based on your specific requirements. Common choices include a comma and a space (,
), a newline character (\n
), or a tab character (\t
).
- Convert elements to strings if necessary: If the elements of your list are not already strings, you may need to convert them using the str()
function before joining them together. This ensures that all elements can be concatenated into a single string.
- Handle special characters: If your list contains elements that include special characters, such as quotes or commas, you may need to handle them properly to avoid any issues when converting to a string. For example, you can escape special characters or enclose each element in quotes.
- Consider performance implications: If you're dealing with large lists or performance is a concern, be mindful of the performance implications of converting a list to a string. List comprehensions and the join()
function are generally efficient, but it's always a good idea to measure and optimize if necessary.