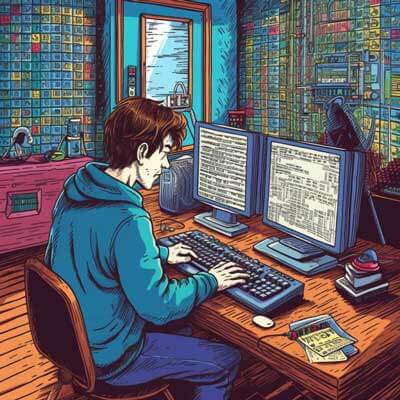
Reading JSON data from a file is a common task in Python, especially when working with APIs or when processing data stored in JSON format. In this guide, we will explore different methods to read JSON data from a file in Python.
Why is this question asked?
The question “How to read JSON from a file in Python?” is often asked by developers who are new to working with JSON data or those who are looking for an efficient way to read and process JSON files in their Python programs. JSON (JavaScript Object Notation) is a popular data interchange format, and Python provides built-in modules to handle JSON data.
Related Article: How to Convert JSON to CSV in Python
Potential reasons for reading JSON from a file:
1. Data processing: JSON files are commonly used to store structured data, such as configuration settings, user data, or API responses. Reading JSON from a file allows you to access and process this data in your Python program.
2. Data exchange: JSON is widely used as a data interchange format between different systems and programming languages. Reading JSON from a file enables you to retrieve data from another system or application and use it in your Python code.
Methods to read JSON from a file in Python:
Method 1: Using the built-in json module
Python provides a built-in json module that makes it easy to read, write, and manipulate JSON data. To read JSON from a file using this module, follow these steps:
1. Import the json module: Start by importing the json module in your Python script.
import json
2. Open the JSON file: Use the open()
function to open the JSON file in read mode. Specify the file path and mode as arguments.
with open('data.json', 'r') as file: data = json.load(file)
3. Read the JSON data: Use the json.load()
function to read the JSON data from the file. This function parses the JSON data and returns a Python dictionary or list, depending on the structure of the JSON.
4. Access the JSON data: Once the JSON data is loaded into the data
variable, you can access its elements using standard dictionary or list indexing.
print(data['name'])
5. Close the file: It’s good practice to close the file after reading the JSON data to free up system resources.
file.close()
Here’s an example that demonstrates how to read JSON data from a file using the built-in json module:
import json with open('data.json', 'r') as file: data = json.load(file) print(data['name'])
Method 2: Using the pandas library
The pandas library in Python provides powerful data manipulation and analysis tools. It also offers convenient functions to read and write JSON data. If you are already using pandas in your project, you can take advantage of its read_json()
function to read JSON data from a file.
To read JSON from a file using pandas, follow these steps:
1. Install pandas: If you don’t have pandas installed, you can install it using pip:
pip install pandas
2. Import the pandas module: Start by importing the pandas module in your Python script.
import pandas as pd
3. Read the JSON file: Use the read_json()
function from pandas to read the JSON file. Pass the file path as the argument.
data = pd.read_json('data.json')
4. Access the JSON data: Once the JSON data is loaded into the data
variable, you can access its elements using pandas data manipulation methods.
print(data['name'])
Here’s an example that demonstrates how to read JSON data from a file using the pandas library:
import pandas as pd data = pd.read_json('data.json') print(data['name'])
Best practices and suggestions:
– It’s a good practice to use the with
statement when reading files in Python, as it automatically takes care of closing the file after reading. This ensures that system resources are properly released.
– When working with large JSON files, consider using a streaming JSON parser instead of loading the entire file into memory at once. The ijson
library provides such a parser for Python.
– Validate the JSON data before reading it from a file to ensure its integrity. The json
module provides the json.JSONDecodeError
exception, which can be used to catch and handle JSON decoding errors.
– If you need to read multiple JSON objects from a file where each object is on a separate line (known as JSON Lines format), you can use the jsonlines
library. It provides an easy way to iterate over the objects in such files.
Related Article: How to Read Xlsx File Using Pandas Library in Python