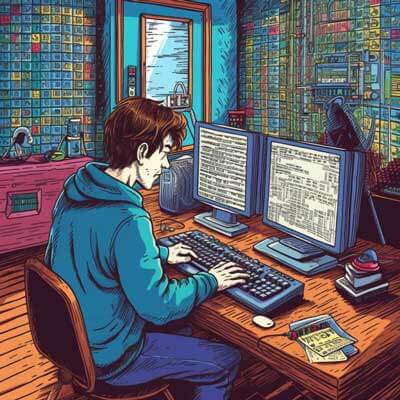
Validating an email address in JavaScript is a common requirement for web developers. It ensures that users enter a valid email address in a form or input field. In this article, we will explore different approaches to validate an email address using JavaScript.
Why is email validation important?
Email validation is important for several reasons. First, it ensures that the email address provided by the user is in the correct format. This helps to prevent user errors and improves the overall user experience. Second, email validation helps to protect your application from malicious activity such as spam or phishing attacks. By validating email addresses, you can verify the authenticity of user input and prevent potential security vulnerabilities.
Related Article: How To Check If a Key Exists In a Javascript Object
Approach 1: Regular Expressions
One of the most common approaches to validate an email address in JavaScript is to use regular expressions. Regular expressions provide a powerful and flexible way to match patterns in strings. Here is an example of how to use a regular expression to validate an email address:
function validateEmail(email) { const re = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; return re.test(email); }
In this example, we define a regular expression pattern /^[^\s@]+@[^\s@]+\.[^\s@]+$/
, which matches a string that starts with one or more characters that are not whitespace or “@” (^[^\s@]+
), followed by an “@” symbol (@
), followed by one or more characters that are not whitespace or “@” ([^\s@]+
), followed by a dot (\.
), and ends with one or more characters that are not whitespace or “@” ([^\s@]+$
). The test()
function then checks if the email matches the regular expression pattern and returns true
or false
accordingly.
Approach 2: HTML5 Input Type
Another approach to validate an email address is to use the HTML5 input type email
. When this input type is used, the browser automatically performs basic email validation. Here is an example of how to use the email
input type:
<button>Submit</button> function validateEmail() { const emailInput = document.getElementById('emailInput'); if (emailInput.checkValidity()) { // Email is valid console.log('Email is valid'); } else { // Email is not valid console.log('Email is not valid'); } }
In this example, we define an input field with the email
type and an id of emailInput
. We also define a button with an onclick
event that calls the validateEmail()
function. Inside the function, we use the checkValidity()
method on the emailInput
element to check if the email is valid. If it is valid, we log a message to the console.
Common Mistakes to Avoid
When validating an email address in JavaScript, there are some common mistakes to avoid:
– Not using server-side validation: JavaScript validation is performed on the client-side, which means it can be bypassed by malicious users. It is important to always perform server-side validation to ensure the email address is valid and safe to use.
– Overly strict or lenient validation: Regular expressions can be complex and it is important to strike a balance between strict and lenient validation. Being too strict may result in rejecting valid email addresses, while being too lenient may allow invalid email addresses to pass through.
– Not considering international email addresses: Email addresses can have international characters and domain names. Make sure to account for these when validating email addresses.
Related Article: How To Use the Javascript Void(0) Operator